
Example for updating the MTi-1's firmware. Uses a platform independent, retargetable pure C implementation of the firmware updater protocol.
mtinterface.h
00001 /*! 00002 * \file 00003 * \copyright Copyright (C) Xsens Technologies B.V., 2015. 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may not 00006 * use this file except in compliance with the License. You may obtain a copy 00007 * of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the 00014 * License for the specific language governing permissions and limitations 00015 * under the License. 00016 */ 00017 #ifndef MTINTERFACE_H 00018 #define MTINTERFACE_H 00019 00020 #include "mbed.h" 00021 #include "rtos.h" 00022 #include "xbusmessage.h " 00023 #include "xbusparser.h " 00024 00025 class MtInterface 00026 { 00027 public: 00028 MtInterface(); 00029 virtual ~MtInterface(); 00030 00031 /*! \brief This function must be periodically called from the main loop. Classes which inherid 00032 from MtInterface can place functionality which must run periodically in this function. 00033 */ 00034 virtual void process() = 0; 00035 00036 /*! \brief Interface for sending xbus messages to the module 00037 */ 00038 virtual void sendXbusMessage(XbusMessage const* xbusMessage) = 0; 00039 00040 /*! \brief Interface for requesting the low level format used by an MtInterface instance 00041 */ 00042 virtual XbusBusFormat busFormat() = 0; 00043 00044 XbusMessage* getXbusMessage(); 00045 void releaseXbusMessage(XbusMessage *xbusMessage); 00046 00047 XbusMessage* sendAndWait(XbusMessage const* xbusMessage); 00048 00049 protected: 00050 static const int m_rxBufferSize = 300; 00051 MemoryPool<uint8_t[m_rxBufferSize], 3> m_memoryPool; 00052 MemoryPool<XbusMessage, 6> m_xbusMessagePool; 00053 XbusParser *m_xbusParser; 00054 Queue<XbusMessage, 4> m_xbusRxQueue; 00055 00056 void *allocateMessageData(size_t bufferSize); 00057 void deallocateMessageData(void const *buffer); 00058 void xbusParserCallbackFunction(struct XbusMessage const *message); 00059 00060 friend void *allocateMessageDataWrapper(size_t bufferSize); 00061 friend void deallocateMessageDataWrapper(void const *buffer); 00062 friend void xbusParserCallbackFunctionWrapper(struct XbusMessage const *message); 00063 }; 00064 00065 #endif //MTINTERFACE_H
Generated on Wed Jul 13 2022 07:56:15 by
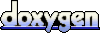