
Programsko rješenje za uređaj koji mjeri nagib i udaljenost.
Embed:
(wiki syntax)
Show/hide line numbers
HCSR04.h
00001 #ifndef HCSR04_H 00002 #define HCSR04_H 00003 00004 /** A distance measurement class using ultrasonic sensor HC-SR04. 00005 * 00006 * Example of use: 00007 * @code 00008 * #include "mbed.h" 00009 * #include "HCSR04.h" 00010 * 00011 * Serial pc(USBTX, USBRX); 00012 * Timer timer; 00013 * 00014 * int main() { 00015 * HCSR04 sensor(p5, p7); 00016 * sensor.setRanges(10, 110); 00017 * pc.printf("Min. range = %g cm\n\rMax. range = %g cm\n\r", 00018 * sensor.getMinRange(), sensor.getMaxRange()); 00019 * while(true) { 00020 * timer.reset(); 00021 * timer.start(); 00022 * sensor.startMeasurement(); 00023 * while(!sensor.isNewDataReady()) { 00024 * // wait for new data 00025 * // waiting time depends on the distance 00026 * } 00027 * pc.printf("Distance: %5.1f mm\r", sensor.getDistance_mm()); 00028 * timer.stop(); 00029 * wait_ms(500 - timer.read_ms()); // time the loop 00030 * } 00031 * } 00032 * @endcode 00033 */ 00034 class HCSR04 { 00035 00036 public: 00037 00038 /** Receives two PinName variables. 00039 * @param echoPin mbed pin to which the echo signal is connected to 00040 * @param triggerPin mbed pin to which the trigger signal is connected to 00041 */ 00042 HCSR04(PinName echoPin, PinName triggerPin); 00043 00044 /** Start the measurement. Measurement time depends on the distance. 00045 * Maximum measurement time is limited to 25 ms (400 cm). 00046 */ 00047 void startMeasurement(); 00048 00049 /** Returns the distance in cm. Requires previous call of startMeasurement(). 00050 * @returns distance of the measuring object in cm. 00051 */ 00052 float getDistance_cm(); 00053 00054 /** Returns the distance in mm. Requires previous call of startMeasurement(). 00055 * @returns distance of the measuring object in mm. 00056 */ 00057 float getDistance_mm(); 00058 00059 /** Sets the minimum and maximum ranges between the factory values of 2 cm and 400 cm. 00060 * @param minRange Minimum range in cm. Must be between 2 cm and maxRange. 00061 * @param maxRange Maximum range in cm. Must be between minRange and 400 cm. 00062 */ 00063 void setRanges(float minRange, float maxRange); 00064 00065 /** Retreives the minimum sensor range set by the user. 00066 * @returns the minimum sensor range set by the user in cm. 00067 */ 00068 float getMinRange(); 00069 00070 /** Retreives the maximum sensor range set by the user. 00071 * @returns the maximum sensor range set by the user in cm. 00072 */ 00073 float getMaxRange(); 00074 00075 /** Checks if the new data is ready. 00076 * @returns true if new data is ready, false otherwise. 00077 */ 00078 bool isNewDataReady(); 00079 00080 private: 00081 00082 InterruptIn echo; // echo pin 00083 DigitalOut trigger; // trigger pin 00084 Timer timer; // echo pulsewidth measurement 00085 float distance; // store the distance in cm 00086 float minDistance; // minimum measurable distance 00087 float maxDistance; // maximum measurable distance 00088 Timeout triggerTimeout, echoTimeout; 00089 bool newDataReady, timerStarted; 00090 00091 /** Start the timer. */ 00092 void startTimer(); 00093 00094 /** Stop the timer. */ 00095 void stopTimer(); 00096 00097 /** Initialization. */ 00098 void init(); 00099 00100 void turnOffTrigger(); 00101 }; 00102 00103 #endif
Generated on Wed Jul 13 2022 08:57:40 by
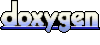