A distance measurement class using ultrasonic sensor HC-SR04.
Dependents: Esercitazione4_4 HC-SR04 Group10_slave Oled_Gus ... more
HCSR04 Class Reference
A distance measurement class using ultrasonic sensor HC-SR04. More...
#include <HCSR04.h>
Public Member Functions | |
HCSR04 (PinName echoPin, PinName triggerPin) | |
Receives two PinName variables. | |
void | startMeasurement () |
Start the measurement. | |
float | getDistance_cm () |
Returns the distance in cm. | |
float | getDistance_mm () |
Returns the distance in mm. | |
void | setRanges (float minRange, float maxRange) |
Sets the minimum and maximum ranges between the factory values of 2 cm and 400 cm. | |
float | getMinRange () |
Retreives the minimum sensor range set by the user. | |
float | getMaxRange () |
Retreives the maximum sensor range set by the user. | |
bool | isNewDataReady () |
Checks if the new data is ready. |
Detailed Description
A distance measurement class using ultrasonic sensor HC-SR04.
Example of use:
#include "mbed.h" #include "HCSR04.h" Serial pc(USBTX, USBRX); Timer timer; int main() { HCSR04 sensor(p5, p7); sensor.setRanges(10, 110); pc.printf("Min. range = %g cm\n\rMax. range = %g cm\n\r", sensor.getMinRange(), sensor.getMaxRange()); while(true) { timer.reset(); timer.start(); sensor.startMeasurement(); while(!sensor.isNewDataReady()) { // wait for new data // waiting time depends on the distance } pc.printf("Distance: %5.1f mm\r", sensor.getDistance_mm()); timer.stop(); wait_ms(500 - timer.read_ms()); // time the loop } }
Definition at line 34 of file HCSR04.h.
Constructor & Destructor Documentation
HCSR04 | ( | PinName | echoPin, |
PinName | triggerPin | ||
) |
Receives two PinName variables.
- Parameters:
-
echoPin mbed pin to which the echo signal is connected to triggerPin mbed pin to which the trigger signal is connected to
Definition at line 4 of file HCSR04.cpp.
Member Function Documentation
float getDistance_cm | ( | ) |
Returns the distance in cm.
Requires previous call of startMeasurement().
- Returns:
- distance of the measuring object in cm.
Definition at line 52 of file HCSR04.cpp.
float getDistance_mm | ( | ) |
Returns the distance in mm.
Requires previous call of startMeasurement().
- Returns:
- distance of the measuring object in mm.
Definition at line 57 of file HCSR04.cpp.
float getMaxRange | ( | ) |
Retreives the maximum sensor range set by the user.
- Returns:
- the maximum sensor range set by the user in cm.
Definition at line 79 of file HCSR04.cpp.
float getMinRange | ( | ) |
Retreives the minimum sensor range set by the user.
- Returns:
- the minimum sensor range set by the user in cm.
Definition at line 75 of file HCSR04.cpp.
bool isNewDataReady | ( | ) |
Checks if the new data is ready.
- Returns:
- true if new data is ready, false otherwise.
Definition at line 62 of file HCSR04.cpp.
void setRanges | ( | float | minRange, |
float | maxRange | ||
) |
Sets the minimum and maximum ranges between the factory values of 2 cm and 400 cm.
- Parameters:
-
minRange Minimum range in cm. Must be between 2 cm and maxRange. maxRange Maximum range in cm. Must be between minRange and 400 cm.
Definition at line 66 of file HCSR04.cpp.
void startMeasurement | ( | ) |
Start the measurement.
Measurement time depends on the distance. Maximum measurement time is limited to 25 ms (400 cm).
Definition at line 45 of file HCSR04.cpp.
Generated on Tue Jul 12 2022 13:21:01 by
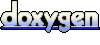