A distance measurement class using ultrasonic sensor HC-SR04.
Dependents: Esercitazione4_4 HC-SR04 Group10_slave Oled_Gus ... more
HCSR04.cpp
00001 #include "mbed.h" 00002 #include "HCSR04.h" 00003 00004 HCSR04::HCSR04(PinName echoPin, PinName triggerPin) : echo(echoPin), trigger(triggerPin) { 00005 init(); 00006 } 00007 00008 void HCSR04::init() { 00009 distance = -1; // initial distance 00010 minDistance = 2; 00011 maxDistance = 400; 00012 newDataReady = timerStarted = false; 00013 } 00014 00015 void HCSR04::startTimer() { 00016 if (!timerStarted) { 00017 timer.start(); // start the timer 00018 timerStarted = true; 00019 echoTimeout.attach_us(this, &HCSR04::stopTimer, 25000); // in case echo fall does not occur 00020 echo.fall(this, &HCSR04::stopTimer); 00021 echo.rise(NULL); 00022 } 00023 } 00024 00025 void HCSR04::stopTimer() { 00026 timer.stop(); // stop the timer 00027 if (timerStarted) { 00028 distance = timer.read() * 1e6 / 58; 00029 if (distance < minDistance) 00030 distance = minDistance; 00031 if (distance > maxDistance) 00032 distance = maxDistance; 00033 newDataReady = true; 00034 } 00035 timer.reset(); 00036 timerStarted = false; 00037 echoTimeout.detach(); 00038 echo.fall(NULL); 00039 } 00040 00041 void HCSR04::turnOffTrigger() { 00042 trigger = 0; 00043 } 00044 00045 void HCSR04::startMeasurement() { 00046 trigger = 1; 00047 triggerTimeout.attach_us(this, &HCSR04::turnOffTrigger, 10); 00048 echo.rise(this, &HCSR04::startTimer); 00049 newDataReady = false; 00050 } 00051 00052 float HCSR04::getDistance_cm() { 00053 newDataReady = false; 00054 return distance; 00055 } 00056 00057 float HCSR04::getDistance_mm() { 00058 newDataReady = false; 00059 return distance * 10; 00060 } 00061 00062 bool HCSR04::isNewDataReady() { 00063 return newDataReady; 00064 } 00065 00066 void HCSR04::setRanges(float minRange, float maxRange) { 00067 if (minRange < maxRange) { 00068 if (minRange >= 2 && minRange < 400) // bug from revs. 4 and 5 corrected 00069 minDistance = minRange; 00070 if (maxRange <= 400) 00071 maxDistance = maxRange; 00072 } 00073 } 00074 00075 float HCSR04::getMinRange() { 00076 return minDistance; 00077 } 00078 00079 float HCSR04::getMaxRange() { 00080 return maxDistance; 00081 }
Generated on Tue Jul 12 2022 13:21:01 by
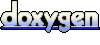