f
Dependencies: ST_INTERFACES X_NUCLEO_COMMON
Fork of X_NUCLEO_IHM01A1 by
L6474 Class Reference
Class representing an L6474 component. More...
#include <L6474.h>
Public Member Functions | |
L6474 (PinName flag_irq, PinName standby_reset, PinName direction, PinName pwm, PinName ssel, DevSPI &spi) | |
Constructor. | |
virtual | ~L6474 (void) |
Destructor. | |
virtual int | init (void *init=NULL) |
Initializing the component in 1/16 Microstepping mode. | |
virtual int | read_id (uint8_t *id=NULL) |
Getting the ID of the component. | |
virtual unsigned int | get_status (void) |
Getting the value of the Status Register. | |
virtual float | get_parameter (unsigned int parameter) |
Getting a parameter. | |
virtual signed int | get_position (void) |
Getting the position. | |
virtual signed int | get_mark (void) |
Getting the marked position. | |
virtual unsigned int | get_speed (void) |
Getting the current speed in pps. | |
virtual unsigned int | get_max_speed (void) |
Getting the maximum speed in pps. | |
virtual unsigned int | get_min_speed (void) |
Getting the minimum speed in pps. | |
virtual unsigned int | get_acceleration (void) |
Getting the acceleration in pps^2. | |
virtual unsigned int | get_deceleration (void) |
Getting the deceleration in pps^2. | |
virtual direction_t | get_direction (void) |
Getting the direction of rotation. | |
virtual void | set_parameter (unsigned int parameter, float value) |
Setting a parameter. | |
virtual void | set_home (void) |
Setting the current position to be the home position. | |
virtual void | set_mark (void) |
Setting the current position to be the marked position. | |
virtual bool | set_max_speed (unsigned int speed) |
Setting the maximum speed in pps. | |
virtual bool | set_min_speed (unsigned int speed) |
Setting the minimum speed in pps. | |
virtual bool | set_acceleration (unsigned int acceleration) |
Setting the acceleration in pps^2. | |
virtual bool | set_deceleration (unsigned int deceleration) |
Setting the deceleration in pps^2. | |
virtual void | go_to (signed int position) |
Going to a specified position. | |
virtual void | go_home (void) |
Going to the home position. | |
virtual void | go_mark (void) |
Going to the marked position. | |
virtual void | run (direction_t direction) |
Running the motor towards a specified direction. | |
virtual void | move (direction_t direction, unsigned int steps) |
Moving the motor towards a specified direction for a certain number of steps. | |
virtual void | soft_stop (void) |
Stopping the motor through an immediate deceleration up to zero speed. | |
virtual void | hard_stop (void) |
Stopping the motor through an immediate infinite deceleration. | |
virtual void | soft_hiz (void) |
Disabling the power bridge after performing a deceleration to zero. | |
virtual void | hard_hiz (void) |
Disabling the power bridge immediately. | |
virtual void | wait_while_active (void) |
Waiting while the motor is active. | |
virtual motorState_t | get_device_state (void) |
Getting the device state. | |
virtual uint16_t | read_status_register (void) |
Reading the Status Register. | |
virtual bool | set_step_mode (step_mode_t step_mode) |
Setting the Step Mode. | |
virtual void | attach_error_handler (void(*fptr)(uint16_t error)) |
Attaching an error handler. | |
virtual void | enable (void) |
Enabling the device. | |
virtual void | disable (void) |
Disabling the device. | |
virtual uint8_t | get_fw_version (void) |
Getting the version of the firmware. | |
void | attach_flag_irq (void(*fptr)(void)) |
Attaching an interrupt handler to the FLAG interrupt. | |
void | enable_flag_irq (void) |
Enabling the FLAG interrupt handling. | |
void | disable_flag_irq (void) |
Disabling the FLAG interrupt handling. | |
Protected Member Functions | |
status_t | Read (uint8_t *pBuffer, uint16_t NumBytesToRead) |
Utility function to read data from L6474. | |
status_t | Write (uint8_t *pBuffer, uint16_t NumBytesToWrite) |
Utility function to write data to L6474. | |
status_t | ReadWrite (uint8_t *pBufferToRead, uint8_t *pBufferToWrite, uint16_t NumBytes) |
Utility function to read and write data from/to L6474 at the same time. | |
void | L6474_Delay (uint32_t delay) |
Making the CPU wait. | |
void | L6474_EnableIrq (void) |
Enabling interrupts. | |
void | L6474_DisableIrq (void) |
Disabling interrupts. | |
void | L6474_PwmInit (void) |
Initialising the PWM. | |
void | L6474_PwmSetFreq (uint16_t frequency) |
Setting the frequency of PWM. | |
void | L6474_PwmStop (void) |
Stopping the PWM. | |
void | L6474_ReleaseReset (void) |
Putting the device in standby mode. | |
void | L6474_Reset (void) |
Putting the device in reset mode. | |
void | L6474_SetDirectionGpio (uint8_t gpioState) |
Setting the direction of rotation. | |
uint8_t | L6474_SpiWriteBytes (uint8_t *pByteToTransmit, uint8_t *pReceivedByte) |
Writing and reading bytes to/from the component through the SPI at the same time. |
Detailed Description
Class representing an L6474 component.
Definition at line 83 of file L6474.h.
Constructor & Destructor Documentation
L6474 | ( | PinName | flag_irq, |
PinName | standby_reset, | ||
PinName | direction, | ||
PinName | pwm, | ||
PinName | ssel, | ||
DevSPI & | spi | ||
) |
Constructor.
- Parameters:
-
flag_irq pin name of the FLAG pin of the component. standby_reset pin name of the STBY pin of the component. direction pin name of the DIR pin of the component. pwm pin name of the PWM pin of the component. ssel pin name of the SSEL pin of the SPI device to be used for communication. spi SPI device to be used for communication.
Member Function Documentation
virtual void attach_error_handler | ( | void(*)(uint16_t error) | fptr ) | [virtual] |
void attach_flag_irq | ( | void(*)(void) | fptr ) |
virtual void disable | ( | void | ) | [virtual] |
void disable_flag_irq | ( | void | ) |
virtual void enable | ( | void | ) | [virtual] |
void enable_flag_irq | ( | void | ) |
virtual unsigned int get_acceleration | ( | void | ) | [virtual] |
virtual unsigned int get_deceleration | ( | void | ) | [virtual] |
virtual motorState_t get_device_state | ( | void | ) | [virtual] |
virtual direction_t get_direction | ( | void | ) | [virtual] |
virtual uint8_t get_fw_version | ( | void | ) | [virtual] |
virtual signed int get_mark | ( | void | ) | [virtual] |
virtual unsigned int get_max_speed | ( | void | ) | [virtual] |
virtual unsigned int get_min_speed | ( | void | ) | [virtual] |
virtual float get_parameter | ( | unsigned int | parameter ) | [virtual] |
Getting a parameter.
- Parameters:
-
parameter A parameter's register address.
- Return values:
-
The parameter's value.
- Note:
- The Status Register's flags are cleared, contrary to the read_status_register() method. The parameter can be one of the following: + L6474_ABS_POS + L6474_EL_POS + L6474_MARK + L6474_RESERVED_REG01 + L6474_RESERVED_REG02 + L6474_RESERVED_REG03 + L6474_RESERVED_REG04 + L6474_RESERVED_REG05 + L6474_RESERVED_REG06 + L6474_TVAL : value in mA + L6474_RESERVED_REG07 + L6474_RESERVED_REG08 + L6474_RESERVED_REG09 + L6474_RESERVED_REG10 + L6474_T_FAST + L6474_TON_MIN : value in us + L6474_TOFF_MIN : value in us + L6474_RESERVED_REG11 + L6474_ADC_OUT + L6474_OCD_TH + L6474_RESERVED_REG12 + L6474_STEP_MODE + L6474_ALARM_EN + L6474_CONFIG + L6474_STATUS + L6474_RESERVED_REG13 + L6474_RESERVED_REG14 + L6474_INEXISTENT_REG
virtual signed int get_position | ( | void | ) | [virtual] |
virtual unsigned int get_speed | ( | void | ) | [virtual] |
virtual unsigned int get_status | ( | void | ) | [virtual] |
Getting the value of the Status Register.
- Parameters:
-
None.
- Return values:
-
None.
- Note:
- The Status Register's flags are cleared, contrary to the read_status_register() method.
virtual void go_home | ( | void | ) | [virtual] |
virtual void go_mark | ( | void | ) | [virtual] |
virtual void go_to | ( | signed int | position ) | [virtual] |
virtual void hard_hiz | ( | void | ) | [virtual] |
virtual void hard_stop | ( | void | ) | [virtual] |
virtual int init | ( | void * | init = NULL ) |
[virtual] |
void L6474_Delay | ( | uint32_t | delay ) | [protected] |
void L6474_DisableIrq | ( | void | ) | [protected] |
void L6474_EnableIrq | ( | void | ) | [protected] |
void L6474_PwmInit | ( | void | ) | [protected] |
void L6474_PwmSetFreq | ( | uint16_t | frequency ) | [protected] |
void L6474_PwmStop | ( | void | ) | [protected] |
void L6474_ReleaseReset | ( | void | ) | [protected] |
void L6474_Reset | ( | void | ) | [protected] |
void L6474_SetDirectionGpio | ( | uint8_t | gpioState ) | [protected] |
uint8_t L6474_SpiWriteBytes | ( | uint8_t * | pByteToTransmit, |
uint8_t * | pReceivedByte | ||
) | [protected] |
Writing and reading bytes to/from the component through the SPI at the same time.
- Parameters:
-
[in] pByteToTransmit pointer to the buffer of data to send. [out] pReceivedByte pointer to the buffer to read data into.
- Return values:
-
0 in case of success, "1" otherwise.
virtual void move | ( | direction_t | direction, |
unsigned int | steps | ||
) | [virtual] |
status_t Read | ( | uint8_t * | pBuffer, |
uint16_t | NumBytesToRead | ||
) | [protected] |
virtual int read_id | ( | uint8_t * | id = NULL ) |
[virtual] |
virtual uint16_t read_status_register | ( | void | ) | [virtual] |
status_t ReadWrite | ( | uint8_t * | pBufferToRead, |
uint8_t * | pBufferToWrite, | ||
uint16_t | NumBytes | ||
) | [protected] |
Utility function to read and write data from/to L6474 at the same time.
- Parameters:
-
[out] pBufferToRead pointer to the buffer to read data into. [in] pBufferToWrite pointer to the buffer of data to send. [in] NumBytes number of bytes to read and write.
- Return values:
-
COMPONENT_OK in case of success, COMPONENT_ERROR otherwise.
virtual void run | ( | direction_t | direction ) | [virtual] |
virtual bool set_acceleration | ( | unsigned int | acceleration ) | [virtual] |
virtual bool set_deceleration | ( | unsigned int | deceleration ) | [virtual] |
virtual void set_home | ( | void | ) | [virtual] |
virtual void set_mark | ( | void | ) | [virtual] |
virtual bool set_max_speed | ( | unsigned int | speed ) | [virtual] |
virtual bool set_min_speed | ( | unsigned int | speed ) | [virtual] |
virtual void set_parameter | ( | unsigned int | parameter, |
float | value | ||
) | [virtual] |
Setting a parameter.
- Parameters:
-
parameter A parameter's register address. value The parameter's value.
- Return values:
-
None.
- Note:
- The parameter can be one of the following: + L6474_ABS_POS + L6474_EL_POS + L6474_MARK + L6474_RESERVED_REG01 + L6474_RESERVED_REG02 + L6474_RESERVED_REG03 + L6474_RESERVED_REG04 + L6474_RESERVED_REG05 + L6474_RESERVED_REG06 + L6474_TVAL : value in mA + L6474_RESERVED_REG07 + L6474_RESERVED_REG08 + L6474_RESERVED_REG09 + L6474_RESERVED_REG10 + L6474_T_FAST + L6474_TON_MIN : value in us + L6474_TOFF_MIN : value in us + L6474_RESERVED_REG11 + L6474_ADC_OUT + L6474_OCD_TH + L6474_RESERVED_REG12 + L6474_STEP_MODE + L6474_ALARM_EN + L6474_CONFIG + L6474_STATUS + L6474_RESERVED_REG13 + L6474_RESERVED_REG14 + L6474_INEXISTENT_REG
- Warning:
- Some registers can only be written in particular conditions (see L6474's datasheet). Any attempt to write one of those registers when the conditions are not satisfied causes the command to be ignored and the NOTPERF_CMD flag to rise at the end of the last argument byte. Any attempt to set an inexistent register (wrong address value) causes the command to be ignored and the WRONG_CMD flag to rise. For example, setting some parameters requires first to disable the power bridge; this can be done through the soft_hiz() method. They are the following: + L6474_EL_POS + L6474_T_FAST + L6474_TON_MIN : value in us + L6474_TOFF_MIN : value in us + L6474_ADC_OUT + L6474_STEP_MODE + L6474_CONFIG + L6474_STATUS
virtual bool set_step_mode | ( | step_mode_t | step_mode ) | [virtual] |
Setting the Step Mode.
- Parameters:
-
step_mode The Step Mode.
- Return values:
-
true in case of success, "false" otherwise.
- Warning:
- Setting the step mode implies first disabling the power bridge through the soft_hiz() method.
- Every time step mode is changed, the values of the home and mark positions lose meaning and are reset.
virtual void soft_hiz | ( | void | ) | [virtual] |
virtual void soft_stop | ( | void | ) | [virtual] |
virtual void wait_while_active | ( | void | ) | [virtual] |
status_t Write | ( | uint8_t * | pBuffer, |
uint16_t | NumBytesToWrite | ||
) | [protected] |
Generated on Wed Jul 20 2022 02:05:49 by
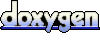