Create a UART to USB Serial bridge with the DipCortex
Fork of DipCortex-USB-CDC by
main.cpp
00001 /** 00002 * USB to UART Bridge 00003 */ 00004 00005 #include "mbed.h" 00006 #include "USBSerial.h" 00007 00008 // Serial TX Pin19, Serial RX Pin20 00009 // Using port and pin names as the mbed definitions pin defs for the M0 are incorrect 00010 Serial uart(P1_13, P1_14); 00011 USBSerial pc; 00012 00013 // Called by ISR 00014 void settingsChanged(int baud, int bits, int parity, int stop) 00015 { 00016 const Serial::Parity parityTable[] = {Serial::None, Serial::Odd, Serial::Even, Serial::Forced0, Serial::Forced1}; 00017 00018 if (stop != 2) { 00019 stop = 1; // stop bit(s) = 1 or 1.5 00020 } 00021 00022 uart.baud(baud); 00023 uart.format(bits, parityTable[parity], stop); 00024 } 00025 00026 int main() 00027 { 00028 pc.attach(settingsChanged); 00029 00030 while (1) { 00031 while (uart.readable()) { 00032 pc.putc(uart.getc()); 00033 } 00034 00035 while (pc.readable()) { 00036 uart.putc(pc.getc()); 00037 } 00038 } 00039 }
Generated on Sat Jul 16 2022 17:41:26 by
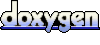