Code for controlling mbed hardware (LED's, motors), as well as code for the Raspberry Pi to run a Support Vector Machine that identifies objects using the Pi camera
Dependencies: mbed Motordriver mbed-rtos PololuLedStrip
Classifier.py
00001 #!/usr/bin/env python 00002 00003 import numpy as np 00004 import re 00005 import scipy.stats 00006 from sklearn import svm, metrics, model_selection 00007 from skimage import io, feature, filters, exposure, color 00008 from joblib import dump, load 00009 00010 00011 00012 class ImageClassifier: 00013 00014 def __init__(self): 00015 self.classifer = None 00016 00017 def imread_convert(self, f): 00018 return io.imread(f).astype(np.uint8) 00019 00020 def load_data_from_folder(self, dir): 00021 # read all images into an image collection 00022 ic = io.ImageCollection(dir + "*.bmp", load_func=self.imread_convert) 00023 00024 # create one large array of image data 00025 data = io.concatenate_images(ic) 00026 00027 # extract labels from image names 00028 labels = np.array(ic.files) 00029 for i, f in enumerate(labels): 00030 m = re.search("_", f) 00031 labels[i] = f[len(dir):m.start()] 00032 00033 return (data, labels) 00034 00035 def extract_image_features(self, data): 00036 # Please do not modify the header above 00037 00038 # extract feature vector from image data 00039 00040 ######################## 00041 ######## YOUR CODE HERE 00042 ######################## 00043 00044 feature_data = [] 00045 00046 for image in data: 00047 feature_data.append( 00048 feature.hog(image, pixels_per_cell=(25, 25), cells_per_block=(3, 3), feature_vector=True, 00049 block_norm="L2-Hys")) 00050 00051 # Please do not modify the return type below 00052 return (feature_data) 00053 00054 def train_classifier(self, train_data, train_labels): 00055 # Please do not modify the header above 00056 00057 # train model and save the trained model to self.classifier 00058 00059 ######################## 00060 ######## YOUR CODE HERE 00061 ######################## 00062 00063 # this param distribution dictionary was obtained from the sklearn documentation page 00064 param_distribution = {'C': scipy.stats.expon(scale=100), 'gamma': scipy.stats.expon(scale=.1), 00065 'kernel': ['rbf']} 00066 00067 model = model_selection.RandomizedSearchCV(svm.SVC(), param_distribution, cv=3, n_iter=6) 00068 00069 self.classifer = model.fit(train_data, train_labels) 00070 00071 def predict_labels(self, data): 00072 # Please do not modify the header 00073 00074 # predict labels of test data using trained model in self.classifier 00075 # the code below expects output to be stored in predicted_labels 00076 00077 ######################## 00078 ######## YOUR CODE HERE 00079 ######################## 00080 00081 predicted_labels = self.classifer.predict(data) 00082 00083 # Please do not modify the return type below 00084 return predicted_labels 00085 00086 00087 def main(): 00088 img_clf = ImageClassifier() 00089 00090 # load images 00091 print("Loading Images") 00092 (train_raw, train_labels) = img_clf.load_data_from_folder('./train/') 00093 (test_raw, test_labels) = img_clf.load_data_from_folder('./test/') 00094 00095 # convert images into features 00096 print("Extracting features from images") 00097 train_data = img_clf.extract_image_features(train_raw) 00098 test_data = img_clf.extract_image_features(test_raw) 00099 00100 # train model and test on training data 00101 print("Training Model") 00102 img_clf.train_classifier(train_data, train_labels) 00103 predicted_labels = img_clf.predict_labels(train_data) 00104 print("\nTraining results") 00105 print("=============================") 00106 print("Confusion Matrix:\n", metrics.confusion_matrix(train_labels, predicted_labels)) 00107 print("Accuracy: ", metrics.accuracy_score(train_labels, predicted_labels)) 00108 print("F1 score: ", metrics.f1_score(train_labels, predicted_labels, average='micro')) 00109 00110 # test model 00111 predicted_labels = img_clf.predict_labels(test_data) 00112 print("\nTest results") 00113 print("=============================") 00114 print("Confusion Matrix:\n", metrics.confusion_matrix(test_labels, predicted_labels)) 00115 print("Accuracy: ", metrics.accuracy_score(test_labels, predicted_labels)) 00116 print("F1 score: ", metrics.f1_score(test_labels, predicted_labels, average='micro')) 00117 00118 dump(img_clf, "model.joblib") 00119 00120 00121 if __name__ == "__main__": 00122 main()
Generated on Tue Jul 12 2022 17:58:52 by
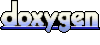