
NFC EEPROM sample application
Embed:
(wiki syntax)
Show/hide line numbers
NfcControllerToEEPROMAdapter.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2018-2018 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef NFCCONTROLLER2EEPROMADAPTER_H_ 00018 #define NFCCONTROLLER2EEPROMADAPTER_H_ 00019 00020 #include <algorithm> 00021 00022 #include "nfc/NFCController.h" 00023 #include "nfc/NFCEEPROMDriver.h" 00024 #include "nfc/NFCRemoteInitiator.h" 00025 00026 namespace mbed { 00027 namespace nfc { 00028 00029 /** 00030 * Adapt an NFCController into an NFCEEPROMDriver. 00031 */ 00032 class ControllerToEEPROMDriverAdapter : 00033 public NFCEEPROMDriver, 00034 private NFCController::Delegate, 00035 private NFCRemoteInitiator::Delegate 00036 { 00037 public: 00038 ControllerToEEPROMDriverAdapter( 00039 NFCController &controller, 00040 const Span<uint8_t> &buffer 00041 ); 00042 00043 virtual ~ControllerToEEPROMDriverAdapter(); 00044 00045 /* ------------------------------------------------------------------------ 00046 * Implementation of NFCEEPROMDriver 00047 */ 00048 virtual void reset(); 00049 00050 virtual size_t read_max_size(); 00051 00052 virtual void start_session(bool force); 00053 00054 virtual void end_session(); 00055 00056 virtual void read_bytes(uint32_t address, uint8_t *bytes, size_t count); 00057 00058 virtual void write_bytes(uint32_t address, const uint8_t *bytes, size_t count); 00059 00060 virtual void read_size(); 00061 00062 virtual void write_size(size_t count); 00063 00064 virtual void erase_bytes(uint32_t address, size_t count); 00065 00066 private: 00067 /* ------------------------------------------------------------------------ 00068 * Implementation of NFCRemoteInitiator::Delegate 00069 */ 00070 virtual void on_connected(); 00071 00072 virtual void on_disconnected(); 00073 00074 virtual void parse_ndef_message(const Span<const uint8_t> &buffer); 00075 00076 virtual size_t build_ndef_message(const Span<uint8_t> &buffer); 00077 00078 /* ------------------------------------------------------------------------ 00079 * Implementation of NFCController::Delegate 00080 */ 00081 virtual void on_discovery_terminated( 00082 nfc_discovery_terminated_reason_t reason 00083 ); 00084 00085 virtual void on_nfc_initiator_discovered( 00086 const SharedPtr<NFCRemoteInitiator> &nfc_initiator 00087 ); 00088 00089 NFCController& _nfc_controller; 00090 Span<uint8_t> _eeprom_buffer; 00091 size_t _current_eeprom_size; 00092 bool _session_opened; 00093 SharedPtr<NFCRemoteInitiator> _nfc_remote_initiator; 00094 }; 00095 00096 } // namespace nfc 00097 } // namespace mbed 00098 00099 00100 #endif /* NFCCONTROLLER2EEPROMADAPTER_H_ */
Generated on Tue Jul 12 2022 15:46:04 by
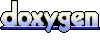