
BLE LED control example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include <events/mbed_events.h> 00018 #include <mbed.h> 00019 #include "ble/BLE.h" 00020 #include "LEDService.h" 00021 00022 DigitalOut alivenessLED(LED1, 0); 00023 DigitalOut actuatedLED(LED2, 0); 00024 00025 const static char DEVICE_NAME[] = "LED"; 00026 static const uint16_t uuid16_list[] = {LEDService::LED_SERVICE_UUID}; 00027 00028 static EventQueue eventQueue(/* event count */ 10 * EVENTS_EVENT_SIZE); 00029 00030 LEDService *ledServicePtr; 00031 00032 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *params) 00033 { 00034 (void) params; 00035 BLE::Instance().gap().startAdvertising(); 00036 } 00037 00038 void blinkCallback(void) 00039 { 00040 alivenessLED = !alivenessLED; /* Do blinky on LED1 to indicate system aliveness. */ 00041 } 00042 00043 /** 00044 * This callback allows the LEDService to receive updates to the ledState Characteristic. 00045 * 00046 * @param[in] params 00047 * Information about the characterisitc being updated. 00048 */ 00049 void onDataWrittenCallback(const GattWriteCallbackParams *params) { 00050 if ((params->handle == ledServicePtr->getValueHandle()) && (params->len == 1)) { 00051 actuatedLED = *(params->data); 00052 } 00053 } 00054 00055 /** 00056 * This function is called when the ble initialization process has failled 00057 */ 00058 void onBleInitError(BLE &ble, ble_error_t error) 00059 { 00060 /* Initialization error handling should go here */ 00061 } 00062 00063 /** 00064 * Callback triggered when the ble initialization process has finished 00065 */ 00066 void bleInitComplete(BLE::InitializationCompleteCallbackContext *params) 00067 { 00068 BLE& ble = params->ble; 00069 ble_error_t error = params->error; 00070 00071 if (error != BLE_ERROR_NONE) { 00072 /* In case of error, forward the error handling to onBleInitError */ 00073 onBleInitError(ble, error); 00074 return; 00075 } 00076 00077 /* Ensure that it is the default instance of BLE */ 00078 if(ble.getInstanceID() != BLE::DEFAULT_INSTANCE) { 00079 return; 00080 } 00081 00082 ble.gap().onDisconnection(disconnectionCallback); 00083 ble.gattServer().onDataWritten(onDataWrittenCallback); 00084 00085 bool initialValueForLEDCharacteristic = false; 00086 ledServicePtr = new LEDService(ble, initialValueForLEDCharacteristic); 00087 00088 /* setup advertising */ 00089 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); 00090 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, (uint8_t *)uuid16_list, sizeof(uuid16_list)); 00091 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); 00092 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00093 ble.gap().setAdvertisingInterval(1000); /* 1000ms. */ 00094 ble.gap().startAdvertising(); 00095 } 00096 00097 void scheduleBleEventsProcessing(BLE::OnEventsToProcessCallbackContext* context) { 00098 BLE &ble = BLE::Instance(); 00099 eventQueue.call(Callback<void()>(&ble, &BLE::processEvents)); 00100 } 00101 00102 int main() 00103 { 00104 eventQueue.call_every(500, blinkCallback); 00105 00106 BLE &ble = BLE::Instance(); 00107 ble.onEventsToProcess(scheduleBleEventsProcessing); 00108 ble.init(bleInitComplete); 00109 00110 eventQueue.dispatch_forever(); 00111 00112 return 0; 00113 }
Generated on Tue Jul 12 2022 15:33:03 by
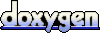