Library to handle the X_NUCLEO_IHM02A1 Motor Control Expansion Board based on the L6470 component.
Dependencies: X_NUCLEO_COMMON ST_INTERFACES
Dependents: HelloWorld_IHM02A1 ConcorsoFinal HelloWorld_IHM02A1_mbedOS HelloWorld_IHM02A1-Serialinterpreter ... more
Fork of X_NUCLEO_IHM02A1 by
XNucleoIHM02A1.h
00001 /** 00002 ****************************************************************************** 00003 * @file XNucleoIHM02A1.h 00004 * @author AST / Software Platforms and Cloud 00005 * @version V1.0 00006 * @date November 3rd, 2015 00007 * @brief Class header file for the X-NUCLEO-IHM02A1 expansion board. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 00039 /* Generated with STM32CubeTOO -----------------------------------------------*/ 00040 00041 00042 /* Define to prevent recursive inclusion -------------------------------------*/ 00043 00044 #ifndef __X_NUCLEO_IHM02A1_CLASS_H 00045 #define __X_NUCLEO_IHM02A1_CLASS_H 00046 00047 00048 /* Includes ------------------------------------------------------------------*/ 00049 00050 /* ACTION 1 ------------------------------------------------------------------* 00051 * Include here platform specific header files. * 00052 *----------------------------------------------------------------------------*/ 00053 #include "mbed.h" 00054 #include "DevSPI.h" 00055 /* ACTION 2 ------------------------------------------------------------------* 00056 * Include here expansion board configuration's header files. * 00057 *----------------------------------------------------------------------------*/ 00058 #include "x_nucleo_ihm02a1_config.h" 00059 /* ACTION 3 ------------------------------------------------------------------* 00060 * Include here expansion board's components' header files. * 00061 * * 00062 * Example: * 00063 * #include "COMPONENT_1.h" * 00064 * #include "COMPONENT_2.h" * 00065 *----------------------------------------------------------------------------*/ 00066 #include "L6470.h" 00067 00068 00069 /* Classes -------------------------------------------------------------------*/ 00070 00071 /** Class representing a X-NUCLEO-IHM02A1 board. 00072 */ 00073 class XNucleoIHM02A1 00074 { 00075 public: 00076 00077 /*** Constructor, Destructor, and Initialization Methods ***/ 00078 00079 /** 00080 * @brief Constructor. 00081 * @param init_0 pointer to the initialization structure of the first motor. 00082 * @param init_1 pointer to the initialization structure of the second motor. 00083 * @param flag_irq pin name of the FLAG pin of the component. 00084 * @param busy_irq pin name of the BUSY pin of the component. 00085 * @param standby_reset pin name of the STBY\RST pin of the component. 00086 * @param ssel pin name of the SSEL pin of the SPI device to be used for communication. 00087 * @param spi SPI device to be used for communication. 00088 */ 00089 XNucleoIHM02A1(L6470_init_t *init_0, L6470_init_t *init_1, PinName flag_irq, PinName busy_irq, PinName standby_reset, PinName ssel, DevSPI *spi); 00090 00091 /** 00092 * @brief Constructor. 00093 * @param init_0 pointer to the initialization structure of the first motor. 00094 * @param init_1 pointer to the initialization structure of the second motor. 00095 * @param flag_irq pin name of the FLAG pin of the component. 00096 * @param busy_irq pin name of the BUSY pin of the component. 00097 * @param standby_reset pin name of the STBY\RST pin of the component. 00098 * @param ssel pin name of the SSEL pin of the SPI device to be used for communication. 00099 * @param mosi pin name of the MOSI pin of the SPI device to be used for communication. 00100 * @param miso pin name of the MISO pin of the SPI device to be used for communication. 00101 * @param sclk pin name of the SCLK pin of the SPI device to be used for communication. 00102 */ 00103 XNucleoIHM02A1(L6470_init_t *init_0, L6470_init_t *init_1, PinName flag_irq, PinName busy_irq, PinName standby_reset, PinName ssel, PinName mosi, PinName miso, PinName sclk); 00104 00105 /** 00106 * @brief Destructor. 00107 */ 00108 ~XNucleoIHM02A1(void) {} 00109 00110 /** 00111 * @brief Initializing the X-NUCLEO-IHM02A1 board. 00112 * @retval true if initialization is successful, false otherwise. 00113 */ 00114 bool init(void); 00115 00116 00117 /*** Other Public Expansion Board Related Methods ***/ 00118 00119 /** 00120 * @brief Getting the array of components. 00121 * @param None. 00122 * @retval The array of components. 00123 */ 00124 L6470 **get_components(void) 00125 { 00126 return components; 00127 } 00128 00129 /** 00130 * @brief Performing the actions set on the motors with calls to a number of 00131 * "Prepare<Action>()" methods, one for each motor of the daisy-chain. 00132 * @param None. 00133 * @retval A pointer to the results returned by the components, i.e. an 00134 * integer value for each of them. 00135 */ 00136 virtual uint32_t* perform_prepared_actions(void) 00137 { 00138 /* Performing pre-actions, if needed. */ 00139 for (int m = 0; m < L6470DAISYCHAINSIZE; m++) { 00140 /* 00141 "GetPosition()" is needed by "PrepareSetMark()" at the time when the 00142 prepared actions get performed. 00143 */ 00144 if (components[m]->get_prepared_action() == L6470::PREPARED_SET_MARK) { 00145 components[m]->prepare_set_mark((uint32_t) components[m]->get_position()); 00146 } 00147 } 00148 00149 /* Performing the prepared actions and getting back raw data. */ 00150 uint8_t *raw_data = components[0]->perform_prepared_actions(); 00151 00152 /* Processing raw data. */ 00153 for (int m = 0; m < L6470DAISYCHAINSIZE; m++) { 00154 results[m] = components[m]->get_result(raw_data); 00155 } 00156 00157 /* Returning results. */ 00158 return results; 00159 } 00160 00161 00162 /*** Public Expansion Board Related Attributes ***/ 00163 00164 /* ACTION 4 --------------------------------------------------------------* 00165 * Declare here a public attribute for each expansion board's component. * 00166 * You will have to call these attributes' public methods within your * 00167 * main program. * 00168 * * 00169 * Example: * 00170 * COMPONENT_1 *component_1; * 00171 * COMPONENT_2 *component_2; * 00172 *------------------------------------------------------------------------*/ 00173 L6470 *l6470_0; 00174 L6470 *l6470_1; 00175 00176 00177 protected: 00178 00179 /*** Protected Expansion Board Related Initialization Methods ***/ 00180 00181 /* ACTION 5 --------------------------------------------------------------* 00182 * Declare here a protected initialization method for each expansion * 00183 * board's component. * 00184 * * 00185 * Example: * 00186 * bool init_COMPONENT_1(void); * 00187 * bool init_COMPONENT_2(void); * 00188 *------------------------------------------------------------------------*/ 00189 bool init_L6470_0(void); 00190 bool init_L6470_1(void); 00191 00192 00193 /*** Component's Instance Variables ***/ 00194 00195 /* IO Device. */ 00196 DevSPI *dev_spi; 00197 00198 /* Components. */ 00199 L6470 *components[L6470DAISYCHAINSIZE]; 00200 00201 /* Components' initialization. */ 00202 L6470_init_t *init_components[L6470DAISYCHAINSIZE]; 00203 00204 /* Results of prepared actions. */ 00205 uint32_t results[L6470DAISYCHAINSIZE]; 00206 00207 /* ACTION 6 --------------------------------------------------------------* 00208 * Declare here the component's static and non-static data, one variable * 00209 * per line. * 00210 * * 00211 * Example: * 00212 * int instance_id; * 00213 * static int number_of_instances; * 00214 *------------------------------------------------------------------------*/ 00215 /* Data. */ 00216 uint8_t instance_id; 00217 00218 /* Static data. */ 00219 static uint8_t number_of_boards; 00220 }; 00221 00222 #endif /* __X_NUCLEO_IHM02A1_CLASS_H */ 00223 00224 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 22:48:07 by
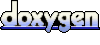