VL53L0X World smallest Time-of-Flight (ToF) ranging sensor
Dependencies: X_NUCLEO_COMMON ST_INTERFACES
Dependents: HelloWorld_ST_Sensors mbed-os-mqtt-client Multi_VL53L0X DISCO-IOT01_HomeEnv ... more
Fork of VL53L0X by
VL53L0X.h
00001 /******************************************************************************* 00002 Copyright © 2016, STMicroelectronics International N.V. 00003 All rights reserved. 00004 00005 Redistribution and use in source and binary forms, with or without 00006 modification, are permitted provided that the following conditions are met: 00007 * Redistributions of source code must retain the above copyright 00008 notice, this list of conditions and the following disclaimer. 00009 * Redistributions in binary form must reproduce the above copyright 00010 notice, this list of conditions and the following disclaimer in the 00011 documentation and/or other materials provided with the distribution. 00012 * Neither the name of STMicroelectronics nor the 00013 names of its contributors may be used to endorse or promote products 00014 derived from this software without specific prior written permission. 00015 00016 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00017 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00018 WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE, AND 00019 NON-INFRINGEMENT OF INTELLECTUAL PROPERTY RIGHTS ARE DISCLAIMED. 00020 IN NO EVENT SHALL STMICROELECTRONICS INTERNATIONAL N.V. BE LIABLE FOR ANY 00021 DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00022 (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00023 LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND 00024 ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00025 (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00026 SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00027 *****************************************************************************/ 00028 00029 #ifndef __VL53L0X_CLASS_H 00030 #define __VL53L0X_CLASS_H 00031 00032 00033 #ifdef _MSC_VER 00034 # ifdef VL53L0X_API_EXPORTS 00035 # define VL53L0X_API __declspec(dllexport) 00036 # else 00037 # define VL53L0X_API 00038 # endif 00039 #else 00040 # define VL53L0X_API 00041 #endif 00042 00043 00044 /* Includes ------------------------------------------------------------------*/ 00045 #include "mbed.h" 00046 #include "RangeSensor.h" 00047 #include "DevI2C.h" 00048 #include "PinNames.h" 00049 #include "VL53L0X_def.h" 00050 #include "VL53L0X_platform.h" 00051 #include "Stmpe1600.h" 00052 00053 00054 /** 00055 * The device model ID 00056 */ 00057 #define IDENTIFICATION_MODEL_ID 0x000 00058 00059 00060 #define STATUS_OK 0x00 00061 #define STATUS_FAIL 0x01 00062 00063 #define VL53L0X_OsDelay(...) wait_ms(2) // 2 msec delay. can also use wait(float secs)/wait_us(int) 00064 00065 #ifdef USE_EMPTY_STRING 00066 #define VL53L0X_STRING_DEVICE_INFO_NAME "" 00067 #define VL53L0X_STRING_DEVICE_INFO_NAME_TS0 "" 00068 #define VL53L0X_STRING_DEVICE_INFO_NAME_TS1 "" 00069 #define VL53L0X_STRING_DEVICE_INFO_NAME_TS2 "" 00070 #define VL53L0X_STRING_DEVICE_INFO_NAME_ES1 "" 00071 #define VL53L0X_STRING_DEVICE_INFO_TYPE "" 00072 00073 /* PAL ERROR strings */ 00074 #define VL53L0X_STRING_ERROR_NONE "" 00075 #define VL53L0X_STRING_ERROR_CALIBRATION_WARNING "" 00076 #define VL53L0X_STRING_ERROR_MIN_CLIPPED "" 00077 #define VL53L0X_STRING_ERROR_UNDEFINED "" 00078 #define VL53L0X_STRING_ERROR_INVALID_PARAMS "" 00079 #define VL53L0X_STRING_ERROR_NOT_SUPPORTED "" 00080 #define VL53L0X_STRING_ERROR_RANGE_ERROR "" 00081 #define VL53L0X_STRING_ERROR_TIME_OUT "" 00082 #define VL53L0X_STRING_ERROR_MODE_NOT_SUPPORTED "" 00083 #define VL53L0X_STRING_ERROR_BUFFER_TOO_SMALL "" 00084 #define VL53L0X_STRING_ERROR_GPIO_NOT_EXISTING "" 00085 #define VL53L0X_STRING_ERROR_GPIO_FUNCTIONALITY_NOT_SUPPORTED "" 00086 #define VL53L0X_STRING_ERROR_CONTROL_INTERFACE "" 00087 #define VL53L0X_STRING_ERROR_INVALID_COMMAND "" 00088 #define VL53L0X_STRING_ERROR_DIVISION_BY_ZERO "" 00089 #define VL53L0X_STRING_ERROR_REF_SPAD_INIT "" 00090 #define VL53L0X_STRING_ERROR_NOT_IMPLEMENTED "" 00091 00092 #define VL53L0X_STRING_UNKNOW_ERROR_CODE "" 00093 00094 00095 00096 /* Range Status */ 00097 #define VL53L0X_STRING_RANGESTATUS_NONE "" 00098 #define VL53L0X_STRING_RANGESTATUS_RANGEVALID "" 00099 #define VL53L0X_STRING_RANGESTATUS_SIGMA "" 00100 #define VL53L0X_STRING_RANGESTATUS_SIGNAL "" 00101 #define VL53L0X_STRING_RANGESTATUS_MINRANGE "" 00102 #define VL53L0X_STRING_RANGESTATUS_PHASE "" 00103 #define VL53L0X_STRING_RANGESTATUS_HW "" 00104 00105 00106 /* Range Status */ 00107 #define VL53L0X_STRING_STATE_POWERDOWN "" 00108 #define VL53L0X_STRING_STATE_WAIT_STATICINIT "" 00109 #define VL53L0X_STRING_STATE_STANDBY "" 00110 #define VL53L0X_STRING_STATE_IDLE "" 00111 #define VL53L0X_STRING_STATE_RUNNING "" 00112 #define VL53L0X_STRING_STATE_UNKNOWN "" 00113 #define VL53L0X_STRING_STATE_ERROR "" 00114 00115 00116 /* Device Specific */ 00117 #define VL53L0X_STRING_DEVICEERROR_NONE "" 00118 #define VL53L0X_STRING_DEVICEERROR_VCSELCONTINUITYTESTFAILURE "" 00119 #define VL53L0X_STRING_DEVICEERROR_VCSELWATCHDOGTESTFAILURE "" 00120 #define VL53L0X_STRING_DEVICEERROR_NOVHVVALUEFOUND "" 00121 #define VL53L0X_STRING_DEVICEERROR_MSRCNOTARGET "" 00122 #define VL53L0X_STRING_DEVICEERROR_SNRCHECK "" 00123 #define VL53L0X_STRING_DEVICEERROR_RANGEPHASECHECK "" 00124 #define VL53L0X_STRING_DEVICEERROR_SIGMATHRESHOLDCHECK "" 00125 #define VL53L0X_STRING_DEVICEERROR_TCC "" 00126 #define VL53L0X_STRING_DEVICEERROR_PHASECONSISTENCY "" 00127 #define VL53L0X_STRING_DEVICEERROR_MINCLIP "" 00128 #define VL53L0X_STRING_DEVICEERROR_RANGECOMPLETE "" 00129 #define VL53L0X_STRING_DEVICEERROR_ALGOUNDERFLOW "" 00130 #define VL53L0X_STRING_DEVICEERROR_ALGOOVERFLOW "" 00131 #define VL53L0X_STRING_DEVICEERROR_RANGEIGNORETHRESHOLD "" 00132 #define VL53L0X_STRING_DEVICEERROR_UNKNOWN "" 00133 00134 /* Check Enable */ 00135 #define VL53L0X_STRING_CHECKENABLE_SIGMA_FINAL_RANGE "" 00136 #define VL53L0X_STRING_CHECKENABLE_SIGNAL_RATE_FINAL_RANGE "" 00137 #define VL53L0X_STRING_CHECKENABLE_SIGNAL_REF_CLIP "" 00138 #define VL53L0X_STRING_CHECKENABLE_RANGE_IGNORE_THRESHOLD "" 00139 00140 /* Sequence Step */ 00141 #define VL53L0X_STRING_SEQUENCESTEP_TCC "" 00142 #define VL53L0X_STRING_SEQUENCESTEP_DSS "" 00143 #define VL53L0X_STRING_SEQUENCESTEP_MSRC "" 00144 #define VL53L0X_STRING_SEQUENCESTEP_PRE_RANGE "" 00145 #define VL53L0X_STRING_SEQUENCESTEP_FINAL_RANGE "" 00146 #else 00147 #define VL53L0X_STRING_DEVICE_INFO_NAME "VL53L0X cut1.0" 00148 #define VL53L0X_STRING_DEVICE_INFO_NAME_TS0 "VL53L0X TS0" 00149 #define VL53L0X_STRING_DEVICE_INFO_NAME_TS1 "VL53L0X TS1" 00150 #define VL53L0X_STRING_DEVICE_INFO_NAME_TS2 "VL53L0X TS2" 00151 #define VL53L0X_STRING_DEVICE_INFO_NAME_ES1 "VL53L0X ES1 or later" 00152 #define VL53L0X_STRING_DEVICE_INFO_TYPE "VL53L0X" 00153 00154 /* PAL ERROR strings */ 00155 #define VL53L0X_STRING_ERROR_NONE \ 00156 "No Error" 00157 #define VL53L0X_STRING_ERROR_CALIBRATION_WARNING \ 00158 "Calibration Warning Error" 00159 #define VL53L0X_STRING_ERROR_MIN_CLIPPED \ 00160 "Min clipped error" 00161 #define VL53L0X_STRING_ERROR_UNDEFINED \ 00162 "Undefined error" 00163 #define VL53L0X_STRING_ERROR_INVALID_PARAMS \ 00164 "Invalid parameters error" 00165 #define VL53L0X_STRING_ERROR_NOT_SUPPORTED \ 00166 "Not supported error" 00167 #define VL53L0X_STRING_ERROR_RANGE_ERROR \ 00168 "Range error" 00169 #define VL53L0X_STRING_ERROR_TIME_OUT \ 00170 "Time out error" 00171 #define VL53L0X_STRING_ERROR_MODE_NOT_SUPPORTED \ 00172 "Mode not supported error" 00173 #define VL53L0X_STRING_ERROR_BUFFER_TOO_SMALL \ 00174 "Buffer too small" 00175 #define VL53L0X_STRING_ERROR_GPIO_NOT_EXISTING \ 00176 "GPIO not existing" 00177 #define VL53L0X_STRING_ERROR_GPIO_FUNCTIONALITY_NOT_SUPPORTED \ 00178 "GPIO funct not supported" 00179 #define VL53L0X_STRING_ERROR_INTERRUPT_NOT_CLEARED \ 00180 "Interrupt not Cleared" 00181 #define VL53L0X_STRING_ERROR_CONTROL_INTERFACE \ 00182 "Control Interface Error" 00183 #define VL53L0X_STRING_ERROR_INVALID_COMMAND \ 00184 "Invalid Command Error" 00185 #define VL53L0X_STRING_ERROR_DIVISION_BY_ZERO \ 00186 "Division by zero Error" 00187 #define VL53L0X_STRING_ERROR_REF_SPAD_INIT \ 00188 "Reference Spad Init Error" 00189 #define VL53L0X_STRING_ERROR_NOT_IMPLEMENTED \ 00190 "Not implemented error" 00191 00192 #define VL53L0X_STRING_UNKNOW_ERROR_CODE \ 00193 "Unknown Error Code" 00194 00195 00196 00197 /* Range Status */ 00198 #define VL53L0X_STRING_RANGESTATUS_NONE "No Update" 00199 #define VL53L0X_STRING_RANGESTATUS_RANGEVALID "Range Valid" 00200 #define VL53L0X_STRING_RANGESTATUS_SIGMA "Sigma Fail" 00201 #define VL53L0X_STRING_RANGESTATUS_SIGNAL "Signal Fail" 00202 #define VL53L0X_STRING_RANGESTATUS_MINRANGE "Min Range Fail" 00203 #define VL53L0X_STRING_RANGESTATUS_PHASE "Phase Fail" 00204 #define VL53L0X_STRING_RANGESTATUS_HW "Hardware Fail" 00205 00206 00207 /* Range Status */ 00208 #define VL53L0X_STRING_STATE_POWERDOWN "POWERDOWN State" 00209 #define VL53L0X_STRING_STATE_WAIT_STATICINIT \ 00210 "Wait for staticinit State" 00211 #define VL53L0X_STRING_STATE_STANDBY "STANDBY State" 00212 #define VL53L0X_STRING_STATE_IDLE "IDLE State" 00213 #define VL53L0X_STRING_STATE_RUNNING "RUNNING State" 00214 #define VL53L0X_STRING_STATE_UNKNOWN "UNKNOWN State" 00215 #define VL53L0X_STRING_STATE_ERROR "ERROR State" 00216 00217 00218 /* Device Specific */ 00219 #define VL53L0X_STRING_DEVICEERROR_NONE "No Update" 00220 #define VL53L0X_STRING_DEVICEERROR_VCSELCONTINUITYTESTFAILURE \ 00221 "VCSEL Continuity Test Failure" 00222 #define VL53L0X_STRING_DEVICEERROR_VCSELWATCHDOGTESTFAILURE \ 00223 "VCSEL Watchdog Test Failure" 00224 #define VL53L0X_STRING_DEVICEERROR_NOVHVVALUEFOUND \ 00225 "No VHV Value found" 00226 #define VL53L0X_STRING_DEVICEERROR_MSRCNOTARGET \ 00227 "MSRC No Target Error" 00228 #define VL53L0X_STRING_DEVICEERROR_SNRCHECK \ 00229 "SNR Check Exit" 00230 #define VL53L0X_STRING_DEVICEERROR_RANGEPHASECHECK \ 00231 "Range Phase Check Error" 00232 #define VL53L0X_STRING_DEVICEERROR_SIGMATHRESHOLDCHECK \ 00233 "Sigma Threshold Check Error" 00234 #define VL53L0X_STRING_DEVICEERROR_TCC \ 00235 "TCC Error" 00236 #define VL53L0X_STRING_DEVICEERROR_PHASECONSISTENCY \ 00237 "Phase Consistency Error" 00238 #define VL53L0X_STRING_DEVICEERROR_MINCLIP \ 00239 "Min Clip Error" 00240 #define VL53L0X_STRING_DEVICEERROR_RANGECOMPLETE \ 00241 "Range Complete" 00242 #define VL53L0X_STRING_DEVICEERROR_ALGOUNDERFLOW \ 00243 "Range Algo Underflow Error" 00244 #define VL53L0X_STRING_DEVICEERROR_ALGOOVERFLOW \ 00245 "Range Algo Overlow Error" 00246 #define VL53L0X_STRING_DEVICEERROR_RANGEIGNORETHRESHOLD \ 00247 "Range Ignore Threshold Error" 00248 #define VL53L0X_STRING_DEVICEERROR_UNKNOWN \ 00249 "Unknown error code" 00250 00251 /* Check Enable */ 00252 #define VL53L0X_STRING_CHECKENABLE_SIGMA_FINAL_RANGE \ 00253 "SIGMA FINAL RANGE" 00254 #define VL53L0X_STRING_CHECKENABLE_SIGNAL_RATE_FINAL_RANGE \ 00255 "SIGNAL RATE FINAL RANGE" 00256 #define VL53L0X_STRING_CHECKENABLE_SIGNAL_REF_CLIP \ 00257 "SIGNAL REF CLIP" 00258 #define VL53L0X_STRING_CHECKENABLE_RANGE_IGNORE_THRESHOLD \ 00259 "RANGE IGNORE THRESHOLD" 00260 #define VL53L0X_STRING_CHECKENABLE_SIGNAL_RATE_MSRC \ 00261 "SIGNAL RATE MSRC" 00262 #define VL53L0X_STRING_CHECKENABLE_SIGNAL_RATE_PRE_RANGE \ 00263 "SIGNAL RATE PRE RANGE" 00264 00265 /* Sequence Step */ 00266 #define VL53L0X_STRING_SEQUENCESTEP_TCC "TCC" 00267 #define VL53L0X_STRING_SEQUENCESTEP_DSS "DSS" 00268 #define VL53L0X_STRING_SEQUENCESTEP_MSRC "MSRC" 00269 #define VL53L0X_STRING_SEQUENCESTEP_PRE_RANGE "PRE RANGE" 00270 #define VL53L0X_STRING_SEQUENCESTEP_FINAL_RANGE "FINAL RANGE" 00271 #endif /* USE_EMPTY_STRING */ 00272 00273 /* sensor operating modes */ 00274 typedef enum { 00275 range_single_shot_polling = 1, 00276 range_continuous_polling, 00277 range_continuous_interrupt, 00278 range_continuous_polling_low_threshold, 00279 range_continuous_polling_high_threshold, 00280 range_continuous_polling_out_of_window, 00281 range_continuous_interrupt_low_threshold, 00282 range_continuous_interrupt_high_threshold, 00283 range_continuous_interrupt_out_of_window, 00284 } OperatingMode; 00285 00286 /** default device address */ 00287 #define VL53L0X_DEFAULT_ADDRESS 0x52 /* (8-bit) */ 00288 00289 /* Classes -------------------------------------------------------------------*/ 00290 /** Class representing a VL53L0 sensor component 00291 */ 00292 class VL53L0X : public RangeSensor 00293 { 00294 public: 00295 /** Constructor 00296 * @param[in] &i2c device I2C to be used for communication 00297 * @param[in] &pin_gpio1 pin Mbed InterruptIn PinName to be used as component GPIO_1 INT 00298 * @param[in] dev_addr device address, 0x29 by default 00299 */ 00300 VL53L0X(DevI2C *i2c, DigitalOut *pin, PinName pin_gpio1, uint8_t dev_addr = VL53L0X_DEFAULT_ADDRESS) : _dev_i2c(i2c), 00301 _gpio0(pin) 00302 { 00303 _my_device.I2cDevAddr = dev_addr; 00304 _my_device.comms_type = 1; // VL53L0X_COMMS_I2C 00305 _my_device.comms_speed_khz = 400; 00306 _device = &_my_device; 00307 _expgpio0 = NULL; 00308 if (pin_gpio1 != NC) { 00309 _gpio1Int = new InterruptIn(pin_gpio1); 00310 } else { 00311 _gpio1Int = NULL; 00312 } 00313 } 00314 00315 /** Constructor 2 (STMPE1600DigiOut) 00316 * @param[in] i2c device I2C to be used for communication 00317 * @param[in] &pin Gpio Expander STMPE1600DigiOut pin to be used as component GPIO_0 CE 00318 * @param[in] pin_gpio1 pin Mbed InterruptIn PinName to be used as component GPIO_1 INT 00319 * @param[in] device address, 0x29 by default 00320 */ 00321 VL53L0X(DevI2C *i2c, Stmpe1600DigiOut *pin, PinName pin_gpio1, 00322 uint8_t dev_addr = VL53L0X_DEFAULT_ADDRESS) : _dev_i2c(i2c), _expgpio0(pin) 00323 { 00324 _my_device.I2cDevAddr = dev_addr; 00325 _my_device.comms_type = 1; // VL53L0X_COMMS_I2C 00326 _my_device.comms_speed_khz = 400; 00327 _device = &_my_device; 00328 _gpio0 = NULL; 00329 if (pin_gpio1 != NC) { 00330 _gpio1Int = new InterruptIn(pin_gpio1); 00331 } else { 00332 _gpio1Int = NULL; 00333 } 00334 } 00335 00336 /** Destructor 00337 */ 00338 virtual ~VL53L0X() 00339 { 00340 if (_gpio1Int != NULL) { 00341 delete _gpio1Int; 00342 } 00343 } 00344 00345 /*** Interface Methods ***/ 00346 /*** High level API ***/ 00347 /** 00348 * @brief PowerOn the sensor 00349 * @return void 00350 */ 00351 /* turns on the sensor */ 00352 void VL53L0X_on(void) 00353 { 00354 if (_gpio0) { 00355 *_gpio0 = 1; 00356 } else { 00357 if (_expgpio0) { 00358 *_expgpio0 = 1; 00359 } 00360 } 00361 wait_ms(10); 00362 } 00363 00364 /** 00365 * @brief PowerOff the sensor 00366 * @return void 00367 */ 00368 /* turns off the sensor */ 00369 void VL53L0X_off(void) 00370 { 00371 if (_gpio0) { 00372 *_gpio0 = 0; 00373 } else { 00374 if (_expgpio0) { 00375 *_expgpio0 = 0; 00376 } 00377 } 00378 wait_ms(10); 00379 } 00380 00381 00382 /** 00383 * @brief Initialize the sensor with default values 00384 * @return "0" on success 00385 */ 00386 int init_sensor(uint8_t new_addr); 00387 00388 /** 00389 * @brief Start the measure indicated by operating mode 00390 * @param[in] operating_mode specifies requested measure 00391 * @param[in] fptr specifies call back function must be !NULL in case of interrupt measure 00392 * @return "0" on success 00393 */ 00394 int start_measurement(OperatingMode operating_mode, void (*fptr)(void)); 00395 00396 /** 00397 * @brief Get results for the measure indicated by operating mode 00398 * @param[in] operating_mode specifies requested measure results 00399 * @param[out] p_data pointer to the MeasureData_t structure to read data in to 00400 * @return "0" on success 00401 */ 00402 int get_measurement(OperatingMode operating_mode, VL53L0X_RangingMeasurementData_t *p_data); 00403 00404 /** 00405 * @brief Stop the currently running measure indicate by operating_mode 00406 * @param[in] operating_mode specifies requested measure to stop 00407 * @return "0" on success 00408 */ 00409 int stop_measurement(OperatingMode operating_mode); 00410 00411 /** 00412 * @brief Interrupt handling func to be called by user after an INT is occourred 00413 * @param[in] opeating_mode indicating the in progress measure 00414 * @param[out] Data pointer to the MeasureData_t structure to read data in to 00415 * @return "0" on success 00416 */ 00417 int handle_irq(OperatingMode operating_mode, VL53L0X_RangingMeasurementData_t *data); 00418 00419 /** 00420 * @brief Enable interrupt measure IRQ 00421 * @return "0" on success 00422 */ 00423 void enable_interrupt_measure_detection_irq(void) 00424 { 00425 if (_gpio1Int != NULL) { 00426 _gpio1Int->enable_irq(); 00427 } 00428 } 00429 00430 /** 00431 * @brief Disable interrupt measure IRQ 00432 * @return "0" on success 00433 */ 00434 void disable_interrupt_measure_detection_irq(void) 00435 { 00436 if (_gpio1Int != NULL) { 00437 _gpio1Int->disable_irq(); 00438 } 00439 } 00440 00441 /** 00442 * @brief Attach a function to call when an interrupt is detected, i.e. measurement is ready 00443 * @param[in] fptr pointer to call back function to be called whenever an interrupt occours 00444 * @return "0" on success 00445 */ 00446 void attach_interrupt_measure_detection_irq(void (*fptr)(void)) 00447 { 00448 if (_gpio1Int != NULL) { 00449 _gpio1Int->rise(fptr); 00450 } 00451 } 00452 00453 /** Wrapper functions */ 00454 /** @defgroup api_init Init functions 00455 * @brief API init functions 00456 * @ingroup api_hl 00457 * @{ 00458 */ 00459 00460 /** 00461 * 00462 * @brief One time device initialization 00463 * 00464 * To be called once and only once after device is brought out of reset (Chip enable) and booted. 00465 * 00466 * @par Function Description 00467 * When not used after a fresh device "power up" or reset, it may return @a #CALIBRATION_WARNING 00468 * meaning wrong calibration data may have been fetched from device that can result in ranging offset error\n 00469 * If application cannot execute device reset or need to run VL53L0X_data_init multiple time 00470 * then it must ensure proper offset calibration saving and restore on its own 00471 * by using @a VL53L0X_get_offset_calibration_data_micro_meter() on first power up and then @a VL53L0X_set_offset_calibration_data_micro_meter() all all subsequent init 00472 * 00473 * @param void 00474 * @return "0" on success, @a #CALIBRATION_WARNING if failed 00475 */ 00476 virtual int init(void *init) 00477 { 00478 return VL53L0X_data_init(_device); 00479 } 00480 00481 /** 00482 * @brief Prepare device for operation 00483 * @par Function Description 00484 * Does static initialization and reprogram common default settings \n 00485 * Device is prepared for new measure, ready single shot ranging or ALS typical polling operation\n 00486 * After prepare user can : \n 00487 * @li Call other API function to set other settings\n 00488 * @li Configure the interrupt pins, etc... \n 00489 * @li Then start ranging or ALS operations in single shot or continuous mode 00490 * 00491 * @param void 00492 * @return "0" on success 00493 */ 00494 int prepare() 00495 { 00496 VL53L0X_Error status = VL53L0X_ERROR_NONE; 00497 uint32_t ref_spad_count; 00498 uint8_t is_aperture_spads; 00499 uint8_t vhv_settings; 00500 uint8_t phase_cal; 00501 00502 if (status == VL53L0X_ERROR_NONE) { 00503 //printf("Call of VL53L0X_StaticInit\r\n"); 00504 status = VL53L0X_static_init(_device); // Device Initialization 00505 } 00506 00507 if (status == VL53L0X_ERROR_NONE) { 00508 //printf("Call of VL53L0X_PerformRefCalibration\r\n"); 00509 status = VL53L0X_perform_ref_calibration(_device, 00510 &vhv_settings, &phase_cal); // Device Initialization 00511 } 00512 00513 if (status == VL53L0X_ERROR_NONE) { 00514 //printf("Call of VL53L0X_PerformRefSpadManagement\r\n"); 00515 status = VL53L0X_perform_ref_spad_management(_device, 00516 &ref_spad_count, &is_aperture_spads); // Device Initialization 00517 // printf ("refSpadCount = %d, isApertureSpads = %d\r\n", refSpadCount, isApertureSpads); 00518 } 00519 00520 return status; 00521 } 00522 00523 /** 00524 * @brief Start continuous ranging mode 00525 * 00526 * @details End user should ensure device is in idle state and not already running 00527 * @return "0" on success 00528 */ 00529 int range_start_continuous_mode() 00530 { 00531 int status; 00532 status = VL53L0X_set_device_mode(_device, VL53L0X_DEVICEMODE_CONTINUOUS_RANGING); 00533 00534 if (status == VL53L0X_ERROR_NONE) { 00535 //printf ("Call of VL53L0X_StartMeasurement\r\n"); 00536 status = VL53L0X_start_measurement(_device); 00537 } 00538 00539 return status; 00540 } 00541 00542 /** 00543 * @brief Get ranging result and only that 00544 * 00545 * @par Function Description 00546 * Unlike @a VL53L0X_get_ranging_measurement_data() this function only retrieves the range in millimeter \n 00547 * It does any required up-scale translation\n 00548 * It can be called after success status polling or in interrupt mode \n 00549 * @warning these function is not doing wrap around filtering \n 00550 * This function doesn't perform any data ready check! 00551 * 00552 * @param p_data Pointer to range distance 00553 * @return "0" on success 00554 */ 00555 virtual int get_distance(uint32_t *p_data) 00556 { 00557 int status = 0; 00558 VL53L0X_RangingMeasurementData_t p_ranging_measurement_data; 00559 00560 status = start_measurement(range_single_shot_polling, NULL); 00561 if (!status) { 00562 status = get_measurement(range_single_shot_polling, &p_ranging_measurement_data); 00563 } 00564 if (p_ranging_measurement_data.RangeStatus == 0) { 00565 // we have a valid range. 00566 *p_data = p_ranging_measurement_data.RangeMilliMeter; 00567 } else { 00568 *p_data = 0; 00569 status = VL53L0X_ERROR_RANGE_ERROR; 00570 } 00571 stop_measurement(range_single_shot_polling); 00572 return status; 00573 } 00574 00575 /** @} */ 00576 00577 /** 00578 * @brief Set new device i2c address 00579 * 00580 * After completion the device will answer to the new address programmed. 00581 * 00582 * @param new_addr The new i2c address (7bit) 00583 * @return "0" on success 00584 */ 00585 int set_device_address(int new_addr) 00586 { 00587 int status; 00588 00589 status = VL53L0X_set_device_address(_device, new_addr); 00590 if (!status) { 00591 _device->I2cDevAddr = new_addr; 00592 } 00593 return status; 00594 00595 } 00596 00597 /** 00598 * @brief Clear given system interrupt condition 00599 * 00600 * @par Function Description 00601 * Clear given interrupt cause by writing into register #SYSTEM_INTERRUPT_CLEAR register. 00602 * @param dev The device 00603 * @param int_clear Which interrupt source to clear. Use any combinations of #INTERRUPT_CLEAR_RANGING , #INTERRUPT_CLEAR_ALS , #INTERRUPT_CLEAR_ERROR. 00604 * @return "0" on success 00605 */ 00606 int clear_interrupt(uint8_t int_clear) 00607 { 00608 return VL53L0X_clear_interrupt_mask(_device, int_clear); 00609 } 00610 00611 /** 00612 * 00613 * @brief Get the 53L0 device 00614 * 00615 * To be called to retrive the internal device descriptor to allow usage of 00616 * low level API having device as parameter. To be called after set_device_address() 00617 * (if any). 00618 * 00619 * @par Function Description 00620 * To be called if low level API usage is needed as those functions requires 00621 * device as a parameter.TICINIT. 00622 * 00623 * @note This function return a pointer to an object internal structure 00624 * 00625 * @param dev ptr to ptr to Device Handle 00626 * @return VL53L0X_ERROR_NONE Success 00627 * @return "Other error code" See ::VL53L0X_Error 00628 */ 00629 VL53L0X_Error vl53l0x_get_device(VL53L0X_DEV *dev) 00630 { 00631 *dev = _device; 00632 return VL53L0X_ERROR_NONE; 00633 } 00634 00635 /** 00636 * 00637 * @brief One time device initialization 00638 * 00639 * To be called once and only once after device is brought out of reset 00640 * (Chip enable) and booted see @a VL53L0X_WaitDeviceBooted() 00641 * 00642 * @par Function Description 00643 * When not used after a fresh device "power up" or reset, it may return 00644 * @a #VL53L0X_ERROR_CALIBRATION_WARNING meaning wrong calibration data 00645 * may have been fetched from device that can result in ranging offset error\n 00646 * If application cannot execute device reset or need to run VL53L0X_DataInit 00647 * multiple time then it must ensure proper offset calibration saving and 00648 * restore on its own by using @a VL53L0X_GetOffsetCalibrationData() on first 00649 * power up and then @a VL53L0X_SetOffsetCalibrationData() in all subsequent init 00650 * This function will change the VL53L0X_State from VL53L0X_STATE_POWERDOWN to 00651 * VL53L0X_STATE_WAIT_STATICINIT. 00652 * 00653 * @note This function accesses to the device 00654 * 00655 * @param dev Device Handle 00656 * @return VL53L0X_ERROR_NONE Success 00657 * @return "Other error code" See ::VL53L0X_Error 00658 */ 00659 VL53L0X_Error VL53L0X_data_init(VL53L0X_DEV dev); 00660 00661 /** 00662 * @brief Do basic device init (and eventually patch loading) 00663 * This function will change the VL53L0X_State from 00664 * VL53L0X_STATE_WAIT_STATICINIT to VL53L0X_STATE_IDLE. 00665 * In this stage all default setting will be applied. 00666 * 00667 * @note This function Access to the device 00668 * 00669 * @param dev Device Handle 00670 * @return VL53L0X_ERROR_NONE Success 00671 * @return "Other error code" See ::VL53L0X_Error 00672 */ 00673 VL53L0X_Error VL53L0X_static_init(VL53L0X_DEV dev); 00674 00675 /** 00676 * @brief Perform Reference Calibration 00677 * 00678 * @details Perform a reference calibration of the Device. 00679 * This function should be run from time to time before doing 00680 * a ranging measurement. 00681 * This function will launch a special ranging measurement, so 00682 * if interrupt are enable an interrupt will be done. 00683 * This function will clear the interrupt generated automatically. 00684 * 00685 * @warning This function is a blocking function 00686 * 00687 * @note This function Access to the device 00688 * 00689 * @param dev Device Handle 00690 * @param p_vhv_settings Pointer to vhv settings parameter. 00691 * @param p_phase_cal Pointer to PhaseCal parameter. 00692 * @return VL53L0X_ERROR_NONE Success 00693 * @return "Other error code" See ::VL53L0X_Error 00694 */ 00695 VL53L0X_Error VL53L0X_perform_ref_calibration(VL53L0X_DEV dev, uint8_t *p_vhv_settings, 00696 uint8_t *p_phase_cal); 00697 00698 /** 00699 * @brief Get Reference Calibration Parameters 00700 * 00701 * @par Function Description 00702 * Get Reference Calibration Parameters. 00703 * 00704 * @note This function Access to the device 00705 * 00706 * @param dev Device Handle 00707 * @param p_vhv_settings Pointer to VHV parameter 00708 * @param p_phase_cal Pointer to PhaseCal Parameter 00709 * @return VL53L0X_ERROR_NONE Success 00710 * @return "Other error code" See ::VL53L0X_Error 00711 */ 00712 VL53L0X_Error VL53L0X_get_ref_calibration(VL53L0X_DEV dev, 00713 uint8_t *p_vhv_settings, uint8_t *p_phase_cal); 00714 00715 VL53L0X_Error VL53L0X_set_ref_calibration(VL53L0X_DEV dev, 00716 uint8_t vhv_settings, uint8_t phase_cal); 00717 00718 /** 00719 * @brief Performs Reference Spad Management 00720 * 00721 * @par Function Description 00722 * The reference SPAD initialization procedure determines the minimum amount 00723 * of reference spads to be enables to achieve a target reference signal rate 00724 * and should be performed once during initialization. 00725 * 00726 * @note This function Access to the device 00727 * 00728 * @note This function change the device mode to 00729 * VL53L0X_DEVICEMODE_SINGLE_RANGING 00730 * 00731 * @param dev Device Handle 00732 * @param ref_spad_count Reports ref Spad Count 00733 * @param is_aperture_spads Reports if spads are of type 00734 * aperture or non-aperture. 00735 * 1:=aperture, 0:=Non-Aperture 00736 * @return VL53L0X_ERROR_NONE Success 00737 * @return VL53L0X_ERROR_REF_SPAD_INIT Error in the Ref Spad procedure. 00738 * @return "Other error code" See ::VL53L0X_Error 00739 */ 00740 VL53L0X_Error VL53L0X_perform_ref_spad_management(VL53L0X_DEV dev, 00741 uint32_t *ref_spad_count, uint8_t *is_aperture_spads); 00742 00743 /** 00744 * @brief Applies Reference SPAD configuration 00745 * 00746 * @par Function Description 00747 * This function applies a given number of reference spads, identified as 00748 * either Aperture or Non-Aperture. 00749 * The requested spad count and type are stored within the device specific 00750 * parameters data for access by the host. 00751 * 00752 * @note This function Access to the device 00753 * 00754 * @param dev Device Handle 00755 * @param refSpadCount Number of ref spads. 00756 * @param is_aperture_spads Defines if spads are of type 00757 * aperture or non-aperture. 00758 * 1:=aperture, 0:=Non-Aperture 00759 * @return VL53L0X_ERROR_NONE Success 00760 * @return VL53L0X_ERROR_REF_SPAD_INIT Error in the in the reference 00761 * spad configuration. 00762 * @return "Other error code" See ::VL53L0X_Error 00763 */ 00764 VL53L0X_Error VL53L0X_set_reference_spads(VL53L0X_DEV dev, 00765 uint32_t refSpadCount, uint8_t is_aperture_spads); 00766 00767 /** 00768 * @brief Retrieves SPAD configuration 00769 * 00770 * @par Function Description 00771 * This function retrieves the current number of applied reference spads 00772 * and also their type : Aperture or Non-Aperture. 00773 * 00774 * @note This function Access to the device 00775 * 00776 * @param dev Device Handle 00777 * @param p_spad_count Number ref Spad Count 00778 * @param p_is_aperture_spads Reports if spads are of type 00779 * aperture or non-aperture. 00780 * 1:=aperture, 0:=Non-Aperture 00781 * @return VL53L0X_ERROR_NONE Success 00782 * @return VL53L0X_ERROR_REF_SPAD_INIT Error in the in the reference 00783 * spad configuration. 00784 * @return "Other error code" See ::VL53L0X_Error 00785 */ 00786 VL53L0X_Error VL53L0X_get_reference_spads(VL53L0X_DEV dev, 00787 uint32_t *p_spad_count, uint8_t *p_is_aperture_spads); 00788 00789 /** 00790 * @brief Get part to part calibration offset 00791 * 00792 * @par Function Description 00793 * Should only be used after a successful call to @a VL53L0X_DataInit to backup 00794 * device NVM value 00795 * 00796 * @note This function Access to the device 00797 * 00798 * @param dev Device Handle 00799 * @param p_offset_calibration_data_micro_meter Return part to part 00800 * calibration offset from device (microns) 00801 * @return VL53L0X_ERROR_NONE Success 00802 * @return "Other error code" See ::VL53L0X_Error 00803 */ 00804 VL53L0X_Error VL53L0X_get_offset_calibration_data_micro_meter(VL53L0X_DEV dev, 00805 int32_t *p_offset_calibration_data_micro_meter); 00806 /** 00807 * Set or over-hide part to part calibration offset 00808 * \sa VL53L0X_DataInit() VL53L0X_GetOffsetCalibrationDataMicroMeter() 00809 * 00810 * @note This function Access to the device 00811 * 00812 * @param dev Device Handle 00813 * @param p_offset_calibration_data_micro_meter Offset (microns) 00814 * @return VL53L0X_ERROR_NONE Success 00815 * @return "Other error code" See ::VL53L0X_Error 00816 */ 00817 VL53L0X_Error VL53L0X_set_offset_calibration_data_micro_meter(VL53L0X_DEV dev, 00818 int32_t offset_calibration_data_micro_meter); 00819 00820 VL53L0X_Error VL53L0X_perform_offset_calibration(VL53L0X_DEV dev, 00821 FixPoint1616_t cal_distance_milli_meter, 00822 int32_t *p_offset_micro_meter); 00823 00824 VL53L0X_Error VL53L0X_perform_xtalk_calibration(VL53L0X_DEV dev, 00825 FixPoint1616_t xtalk_cal_distance, 00826 FixPoint1616_t *p_xtalk_compensation_rate_mega_cps); 00827 00828 /** 00829 * @brief Perform XTalk Measurement 00830 * 00831 * @details Measures the current cross talk from glass in front 00832 * of the sensor. 00833 * This functions performs a histogram measurement and uses the results 00834 * to measure the crosstalk. For the function to be successful, there 00835 * must be no target in front of the sensor. 00836 * 00837 * @warning This function is a blocking function 00838 * 00839 * @warning This function is not supported when the final range 00840 * vcsel clock period is set below 10 PCLKS. 00841 * 00842 * @note This function Access to the device 00843 * 00844 * @param dev Device Handle 00845 * @param timeout_ms Histogram measurement duration. 00846 * @param p_xtalk_per_spad Output parameter containing the crosstalk 00847 * measurement result, in MCPS/Spad. 00848 * Format fixpoint 16:16. 00849 * @param p_ambient_too_high Output parameter which indicate that 00850 * pXtalkPerSpad is not good if the Ambient 00851 * is too high. 00852 * @return VL53L0X_ERROR_NONE Success 00853 * @return VL53L0X_ERROR_INVALID_PARAMS vcsel clock period not supported 00854 * for this operation. 00855 * Must not be less than 10PCLKS. 00856 * @return "Other error code" See ::VL53L0X_Error 00857 */ 00858 VL53L0X_Error VL53L0X_perform_xtalk_measurement(VL53L0X_DEV dev, 00859 uint32_t timeout_ms, FixPoint1616_t *p_xtalk_per_spad, 00860 uint8_t *p_ambient_too_high); 00861 00862 /** 00863 * @brief Enable/Disable Cross talk compensation feature 00864 * 00865 * @note This function is not Implemented. 00866 * Enable/Disable Cross Talk by set to zero the Cross Talk value 00867 * by using @a VL53L0X_SetXTalkCompensationRateMegaCps(). 00868 * 00869 * @param dev Device Handle 00870 * @param x_talk_compensation_enable Cross talk compensation 00871 * to be set 0=disabled else = enabled 00872 * @return VL53L0X_ERROR_NOT_IMPLEMENTED Not implemented 00873 */ 00874 VL53L0X_Error VL53L0X_set_x_talk_compensation_enable(VL53L0X_DEV dev, 00875 uint8_t x_talk_compensation_enable); 00876 00877 /** 00878 * @brief Get Cross talk compensation rate 00879 * 00880 * @note This function is not Implemented. 00881 * Enable/Disable Cross Talk by set to zero the Cross Talk value by 00882 * using @a VL53L0X_SetXTalkCompensationRateMegaCps(). 00883 * 00884 * @param dev Device Handle 00885 * @param p_x_talk_compensation_enable Pointer to the Cross talk compensation 00886 * state 0=disabled or 1 = enabled 00887 * @return VL53L0X_ERROR_NOT_IMPLEMENTED Not implemented 00888 */ 00889 VL53L0X_Error VL53L0X_get_x_talk_compensation_enable(VL53L0X_DEV dev, 00890 uint8_t *p_x_talk_compensation_enable); 00891 /** 00892 * @brief Set Cross talk compensation rate 00893 * 00894 * @par Function Description 00895 * Set Cross talk compensation rate. 00896 * 00897 * @note This function Access to the device 00898 * 00899 * @param dev Device Handle 00900 * @param x_talk_compensation_rate_mega_cps Compensation rate in 00901 * Mega counts per second 00902 * (16.16 fix point) see 00903 * datasheet for details 00904 * @return VL53L0X_ERROR_NONE Success 00905 * @return "Other error code" See ::VL53L0X_Error 00906 */ 00907 VL53L0X_Error VL53L0X_set_x_talk_compensation_rate_mega_cps(VL53L0X_DEV dev, 00908 FixPoint1616_t x_talk_compensation_rate_mega_cps); 00909 00910 /** 00911 * @brief Get Cross talk compensation rate 00912 * 00913 * @par Function Description 00914 * Get Cross talk compensation rate. 00915 * 00916 * @note This function Access to the device 00917 * 00918 * @param dev Device Handle 00919 * @param p_xtalk_compensation_rate_mega_cps Pointer to Compensation rate 00920 * in Mega counts per second 00921 * (16.16 fix point) see 00922 * datasheet for details 00923 * @return VL53L0X_ERROR_NONE Success 00924 * @return "Other error code" See ::VL53L0X_Error 00925 */ 00926 VL53L0X_Error VL53L0X_get_x_talk_compensation_rate_mega_cps(VL53L0X_DEV dev, 00927 FixPoint1616_t *p_xtalk_compensation_rate_mega_cps); 00928 00929 /** 00930 * @brief Set a new device mode 00931 * @par Function Description 00932 * Set device to a new mode (ranging, histogram ...) 00933 * 00934 * @note This function doesn't Access to the device 00935 * 00936 * @param dev Device Handle 00937 * @param device_mode New device mode to apply 00938 * Valid values are: 00939 * VL53L0X_DEVICEMODE_SINGLE_RANGING 00940 * VL53L0X_DEVICEMODE_CONTINUOUS_RANGING 00941 * VL53L0X_DEVICEMODE_CONTINUOUS_TIMED_RANGING 00942 * VL53L0X_DEVICEMODE_SINGLE_HISTOGRAM 00943 * VL53L0X_HISTOGRAMMODE_REFERENCE_ONLY 00944 * VL53L0X_HISTOGRAMMODE_RETURN_ONLY 00945 * VL53L0X_HISTOGRAMMODE_BOTH 00946 * 00947 * 00948 * @return VL53L0X_ERROR_NONE Success 00949 * @return VL53L0X_ERROR_MODE_NOT_SUPPORTED This error occurs when 00950 * DeviceMode is not in the 00951 * supported list 00952 */ 00953 VL53L0X_Error VL53L0X_set_device_mode(VL53L0X_DEV dev, VL53L0X_DeviceModes device_mode); 00954 00955 /** 00956 * @brief Get current new device mode 00957 * @par Function Description 00958 * Get actual mode of the device(ranging, histogram ...) 00959 * 00960 * @note This function doesn't Access to the device 00961 * 00962 * @param dev Device Handle 00963 * @param p_device_mode Pointer to current apply mode value 00964 * Valid values are: 00965 * VL53L0X_DEVICEMODE_SINGLE_RANGING 00966 * VL53L0X_DEVICEMODE_CONTINUOUS_RANGING 00967 * VL53L0X_DEVICEMODE_CONTINUOUS_TIMED_RANGING 00968 * VL53L0X_DEVICEMODE_SINGLE_HISTOGRAM 00969 * VL53L0X_HISTOGRAMMODE_REFERENCE_ONLY 00970 * VL53L0X_HISTOGRAMMODE_RETURN_ONLY 00971 * VL53L0X_HISTOGRAMMODE_BOTH 00972 * 00973 * @return VL53L0X_ERROR_NONE Success 00974 * @return VL53L0X_ERROR_MODE_NOT_SUPPORTED This error occurs when 00975 * DeviceMode is not in the 00976 * supported list 00977 */ 00978 VL53L0X_Error VL53L0X_get_device_mode(VL53L0X_DEV dev, 00979 VL53L0X_DeviceModes *p_device_mode); 00980 00981 /** 00982 * @brief Get current configuration for GPIO pin for a given device 00983 * 00984 * @note This function Access to the device 00985 * 00986 * @param dev Device Handle 00987 * @param pin ID of the GPIO Pin 00988 * @param p_device_mode Pointer to Device Mode associated to the Gpio. 00989 * @param p_functionality Pointer to Pin functionality. 00990 * Refer to ::VL53L0X_GpioFunctionality 00991 * @param p_polarity Pointer to interrupt polarity. 00992 * Active high or active low see 00993 * ::VL53L0X_InterruptPolarity 00994 * @return VL53L0X_ERROR_NONE Success 00995 * @return VL53L0X_ERROR_GPIO_NOT_EXISTING Only Pin=0 is accepted. 00996 * @return VL53L0X_ERROR_GPIO_FUNCTIONALITY_NOT_SUPPORTED 00997 * This error occurs 00998 * when Funcionality programmed is not in the supported list: 00999 * Supported value are: 01000 * VL53L0X_GPIOFUNCTIONALITY_OFF, 01001 * VL53L0X_GPIOFUNCTIONALITY_THRESHOLD_CROSSED_LOW, 01002 * VL53L0X_GPIOFUNCTIONALITY_THRESHOLD_CROSSED_HIGH, 01003 * VL53L0X_GPIOFUNCTIONALITY_THRESHOLD_CROSSED_OUT, 01004 * VL53L0X_GPIOFUNCTIONALITY_NEW_MEASURE_READY 01005 * @return "Other error code" See ::VL53L0X_Error 01006 */ 01007 VL53L0X_Error VL53L0X_get_gpio_config(VL53L0X_DEV dev, uint8_t pin, 01008 VL53L0X_DeviceModes *p_device_mode, 01009 VL53L0X_GpioFunctionality *p_functionality, 01010 VL53L0X_InterruptPolarity *p_polarity); 01011 01012 /** 01013 * @brief Set the configuration of GPIO pin for a given device 01014 * 01015 * @note This function Access to the device 01016 * 01017 * @param dev Device Handle 01018 * @param pin ID of the GPIO Pin 01019 * @param functionality Select Pin functionality. 01020 * Refer to ::VL53L0X_GpioFunctionality 01021 * @param device_mode Device Mode associated to the Gpio. 01022 * @param polarity Set interrupt polarity. Active high 01023 * or active low see ::VL53L0X_InterruptPolarity 01024 * @return VL53L0X_ERROR_NONE Success 01025 * @return VL53L0X_ERROR_GPIO_NOT_EXISTING Only Pin=0 is accepted. 01026 * @return VL53L0X_ERROR_GPIO_FUNCTIONALITY_NOT_SUPPORTED This error occurs 01027 * when Functionality programmed is not in the supported list: 01028 * Supported value are: 01029 * VL53L0X_GPIOFUNCTIONALITY_OFF, 01030 * VL53L0X_GPIOFUNCTIONALITY_THRESHOLD_CROSSED_LOW, 01031 * VL53L0X_GPIOFUNCTIONALITY_THRESHOLD_CROSSED_HIGH, 01032 VL53L0X_GPIOFUNCTIONALITY_THRESHOLD_CROSSED_OUT, 01033 * VL53L0X_GPIOFUNCTIONALITY_NEW_MEASURE_READY 01034 * @return "Other error code" See ::VL53L0X_Error 01035 */ 01036 VL53L0X_Error VL53L0X_set_gpio_config(VL53L0X_DEV dev, uint8_t pin, 01037 VL53L0X_DeviceModes device_mode, VL53L0X_GpioFunctionality functionality, 01038 VL53L0X_InterruptPolarity polarity); 01039 01040 /** 01041 * @brief Start device measurement 01042 * 01043 * @details Started measurement will depend on device parameters set through 01044 * @a VL53L0X_SetParameters() 01045 * This is a non-blocking function. 01046 * This function will change the VL53L0X_State from VL53L0X_STATE_IDLE to 01047 * VL53L0X_STATE_RUNNING. 01048 * 01049 * @note This function Access to the device 01050 * 01051 01052 * @param dev Device Handle 01053 * @return VL53L0X_ERROR_NONE Success 01054 * @return VL53L0X_ERROR_MODE_NOT_SUPPORTED This error occurs when 01055 * DeviceMode programmed with @a VL53L0X_SetDeviceMode is not in the supported 01056 * list: 01057 * Supported mode are: 01058 * VL53L0X_DEVICEMODE_SINGLE_RANGING, 01059 * VL53L0X_DEVICEMODE_CONTINUOUS_RANGING, 01060 * VL53L0X_DEVICEMODE_CONTINUOUS_TIMED_RANGING 01061 * @return VL53L0X_ERROR_TIME_OUT Time out on start measurement 01062 * @return "Other error code" See ::VL53L0X_Error 01063 */ 01064 VL53L0X_Error VL53L0X_start_measurement(VL53L0X_DEV dev); 01065 01066 /** 01067 * @brief Stop device measurement 01068 * 01069 * @details Will set the device in standby mode at end of current measurement\n 01070 * Not necessary in single mode as device shall return automatically 01071 * in standby mode at end of measurement. 01072 * This function will change the VL53L0X_State from VL53L0X_STATE_RUNNING 01073 * to VL53L0X_STATE_IDLE. 01074 * 01075 * @note This function Access to the device 01076 * 01077 * @param dev Device Handle 01078 * @return VL53L0X_ERROR_NONE Success 01079 * @return "Other error code" See ::VL53L0X_Error 01080 */ 01081 VL53L0X_Error VL53L0X_stop_measurement(VL53L0X_DEV dev); 01082 01083 /** 01084 * @brief Return device stop completion status 01085 * 01086 * @par Function Description 01087 * Returns stop completiob status. 01088 * User shall call this function after a stop command 01089 * 01090 * @note This function Access to the device 01091 * 01092 * @param dev Device Handle 01093 * @param p_stop_status Pointer to status variable to update 01094 * @return VL53L0X_ERROR_NONE Success 01095 * @return "Other error code" See ::VL53L0X_Error 01096 */ 01097 VL53L0X_Error VL53L0X_get_stop_completed_status(VL53L0X_DEV dev, 01098 uint32_t *p_stop_status); 01099 01100 /** 01101 * @brief Return Measurement Data Ready 01102 * 01103 * @par Function Description 01104 * This function indicate that a measurement data is ready. 01105 * This function check if interrupt mode is used then check is done accordingly. 01106 * If perform function clear the interrupt, this function will not work, 01107 * like in case of @a VL53L0X_PerformSingleRangingMeasurement(). 01108 * The previous function is blocking function, VL53L0X_GetMeasurementDataReady 01109 * is used for non-blocking capture. 01110 * 01111 * @note This function Access to the device 01112 * 01113 * @param dev Device Handle 01114 * @param p_measurement_data_ready Pointer to Measurement Data Ready. 01115 * 0=data not ready, 1 = data ready 01116 * @return VL53L0X_ERROR_NONE Success 01117 * @return "Other error code" See ::VL53L0X_Error 01118 */ 01119 VL53L0X_Error VL53L0X_get_measurement_data_ready(VL53L0X_DEV dev, 01120 uint8_t *p_measurement_data_ready); 01121 01122 /** 01123 * @brief Retrieve the measurements from device for a given setup 01124 * 01125 * @par Function Description 01126 * Get data from last successful Ranging measurement 01127 * @warning USER should take care about @a VL53L0X_GetNumberOfROIZones() 01128 * before get data. 01129 * PAL will fill a NumberOfROIZones times the corresponding data 01130 * structure used in the measurement function. 01131 * 01132 * @note This function Access to the device 01133 * 01134 * @param dev Device Handle 01135 * @param p_ranging_measurement_data Pointer to the data structure to fill up. 01136 * @return VL53L0X_ERROR_NONE Success 01137 * @return "Other error code" See ::VL53L0X_Error 01138 */ 01139 VL53L0X_Error VL53L0X_get_ranging_measurement_data(VL53L0X_DEV dev, 01140 VL53L0X_RangingMeasurementData_t *p_ranging_measurement_data); 01141 01142 /** 01143 * @brief Clear given system interrupt condition 01144 * 01145 * @par Function Description 01146 * Clear given interrupt(s). 01147 * 01148 * @note This function Access to the device 01149 * 01150 * @param dev Device Handle 01151 * @param interrupt_mask Mask of interrupts to clear 01152 * @return VL53L0X_ERROR_NONE Success 01153 * @return VL53L0X_ERROR_INTERRUPT_NOT_CLEARED Cannot clear interrupts 01154 * 01155 * @return "Other error code" See ::VL53L0X_Error 01156 */ 01157 VL53L0X_Error VL53L0X_clear_interrupt_mask(VL53L0X_DEV dev, uint32_t interrupt_mask); 01158 01159 /** 01160 * @brief Return device interrupt status 01161 * 01162 * @par Function Description 01163 * Returns currently raised interrupts by the device. 01164 * User shall be able to activate/deactivate interrupts through 01165 * @a VL53L0X_SetGpioConfig() 01166 * 01167 * @note This function Access to the device 01168 * 01169 * @param dev Device Handle 01170 * @param p_interrupt_mask_status Pointer to status variable to update 01171 * @return VL53L0X_ERROR_NONE Success 01172 * @return "Other error code" See ::VL53L0X_Error 01173 */ 01174 VL53L0X_Error VL53L0X_get_interrupt_mask_status(VL53L0X_DEV dev, 01175 uint32_t *p_interrupt_mask_status); 01176 01177 /** 01178 * @brief Performs a single ranging measurement and retrieve the ranging 01179 * measurement data 01180 * 01181 * @par Function Description 01182 * This function will change the device mode to VL53L0X_DEVICEMODE_SINGLE_RANGING 01183 * with @a VL53L0X_SetDeviceMode(), 01184 * It performs measurement with @a VL53L0X_PerformSingleMeasurement() 01185 * It get data from last successful Ranging measurement with 01186 * @a VL53L0X_GetRangingMeasurementData. 01187 * Finally it clear the interrupt with @a VL53L0X_ClearInterruptMask(). 01188 * 01189 * @note This function Access to the device 01190 * 01191 * @note This function change the device mode to 01192 * VL53L0X_DEVICEMODE_SINGLE_RANGING 01193 * 01194 * @param dev Device Handle 01195 * @param p_ranging_measurement_data Pointer to the data structure to fill up. 01196 * @return VL53L0X_ERROR_NONE Success 01197 * @return "Other error code" See ::VL53L0X_Error 01198 */ 01199 VL53L0X_Error VL53L0X_perform_single_ranging_measurement(VL53L0X_DEV dev, 01200 VL53L0X_RangingMeasurementData_t *p_ranging_measurement_data); 01201 01202 /** 01203 * @brief Single shot measurement. 01204 * 01205 * @par Function Description 01206 * Perform simple measurement sequence (Start measure, Wait measure to end, 01207 * and returns when measurement is done). 01208 * Once function returns, user can get valid data by calling 01209 * VL53L0X_GetRangingMeasurement or VL53L0X_GetHistogramMeasurement 01210 * depending on defined measurement mode 01211 * User should Clear the interrupt in case this are enabled by using the 01212 * function VL53L0X_ClearInterruptMask(). 01213 * 01214 * @warning This function is a blocking function 01215 * 01216 * @note This function Access to the device 01217 * 01218 * @param dev Device Handle 01219 * @return VL53L0X_ERROR_NONE Success 01220 * @return "Other error code" See ::VL53L0X_Error 01221 */ 01222 VL53L0X_Error VL53L0X_perform_single_measurement(VL53L0X_DEV dev); 01223 01224 /** 01225 * @brief Read current status of the error register for the selected device 01226 * 01227 * @note This function Access to the device 01228 * 01229 * @param dev Device Handle 01230 * @param p_device_error_status Pointer to current error code of the device 01231 * @return VL53L0X_ERROR_NONE Success 01232 * @return "Other error code" See ::VL53L0X_Error 01233 */ 01234 VL53L0X_Error VL53L0X_get_device_error_status(VL53L0X_DEV dev, 01235 VL53L0X_DeviceError *p_device_error_status); 01236 01237 /** 01238 * @brief Human readable error string for a given Error Code 01239 * 01240 * @note This function doesn't access to the device 01241 * 01242 * @param error_code The error code as stored on ::VL53L0X_DeviceError 01243 * @param p_device_error_string The error string corresponding to the ErrorCode 01244 * @return VL53L0X_ERROR_NONE Success 01245 * @return "Other error code" See ::VL53L0X_Error 01246 */ 01247 VL53L0X_Error VL53L0X_get_device_error_string( 01248 VL53L0X_DeviceError error_code, char *p_device_error_string); 01249 01250 /** 01251 * @brief Human readable Range Status string for a given RangeStatus 01252 * 01253 * @note This function doesn't access to the device 01254 * 01255 * @param range_status The RangeStatus code as stored on 01256 * @a VL53L0X_RangingMeasurementData_t 01257 * @param p_range_status_string The returned RangeStatus string. 01258 * @return VL53L0X_ERROR_NONE Success 01259 * @return "Other error code" See ::VL53L0X_Error 01260 */ 01261 VL53L0X_Error VL53L0X_get_range_status_string(uint8_t range_status, 01262 char *p_range_status_string); 01263 01264 VL53L0X_Error VL53L0X_get_total_signal_rate(VL53L0X_DEV dev, 01265 VL53L0X_RangingMeasurementData_t *p_ranging_measurement_data, 01266 FixPoint1616_t *p_total_signal_rate_mcps); 01267 01268 VL53L0X_Error VL53L0X_get_total_xtalk_rate(VL53L0X_DEV dev, 01269 VL53L0X_RangingMeasurementData_t *p_ranging_measurement_data, 01270 FixPoint1616_t *p_total_xtalk_rate_mcps); 01271 01272 /** 01273 * @brief Get Ranging Timing Budget in microseconds 01274 * 01275 * @par Function Description 01276 * Returns the programmed the maximum time allowed by the user to the 01277 * device to run a full ranging sequence for the current mode 01278 * (ranging, histogram, ASL ...) 01279 * 01280 * @note This function Access to the device 01281 * 01282 * @param dev Device Handle 01283 * @param p_measurement_timing_budget_micro_seconds Max measurement time in 01284 * microseconds. 01285 * Valid values are: 01286 * >= 17000 microsecs when wraparound enabled 01287 * >= 12000 microsecs when wraparound disabled 01288 * @return VL53L0X_ERROR_NONE Success 01289 * @return "Other error code" See ::VL53L0X_Error 01290 */ 01291 VL53L0X_Error VL53L0X_get_measurement_timing_budget_micro_seconds(VL53L0X_DEV dev, 01292 uint32_t *p_measurement_timing_budget_micro_seconds); 01293 01294 /** 01295 * @brief Set Ranging Timing Budget in microseconds 01296 * 01297 * @par Function Description 01298 * Defines the maximum time allowed by the user to the device to run a 01299 * full ranging sequence for the current mode (ranging, histogram, ASL ...) 01300 * 01301 * @note This function Access to the device 01302 * 01303 * @param dev Device Handle 01304 * @param measurement_timing_budget_micro_seconds Max measurement time in 01305 * microseconds. 01306 * Valid values are: 01307 * >= 17000 microsecs when wraparound enabled 01308 * >= 12000 microsecs when wraparound disabled 01309 * @return VL53L0X_ERROR_NONE Success 01310 * @return VL53L0X_ERROR_INVALID_PARAMS This error is returned if 01311 MeasurementTimingBudgetMicroSeconds out of range 01312 * @return "Other error code" See ::VL53L0X_Error 01313 */ 01314 VL53L0X_Error VL53L0X_set_measurement_timing_budget_micro_seconds(VL53L0X_DEV dev, 01315 uint32_t measurement_timing_budget_micro_seconds); 01316 01317 /** 01318 * @brief Get specific limit check enable state 01319 * 01320 * @par Function Description 01321 * This function get the enable state of a specific limit check. 01322 * The limit check is identified with the LimitCheckId. 01323 * 01324 * @note This function Access to the device 01325 * 01326 * @param dev Device Handle 01327 * @param limit_check_id Limit Check ID 01328 * (0<= LimitCheckId < VL53L0X_GetNumberOfLimitCheck() ). 01329 * @param p_limit_check_enable Pointer to the check limit enable 01330 * value. 01331 * if 1 the check limit 01332 * corresponding to LimitCheckId is Enabled 01333 * if 0 the check limit 01334 * corresponding to LimitCheckId is disabled 01335 * @return VL53L0X_ERROR_NONE Success 01336 * @return VL53L0X_ERROR_INVALID_PARAMS This error is returned 01337 * when LimitCheckId value is out of range. 01338 * @return "Other error code" See ::VL53L0X_Error 01339 */ 01340 VL53L0X_Error VL53L0X_get_limit_check_enable(VL53L0X_DEV dev, uint16_t limit_check_id, 01341 uint8_t *p_limit_check_enable); 01342 01343 /** 01344 * @brief Enable/Disable a specific limit check 01345 * 01346 * @par Function Description 01347 * This function Enable/Disable a specific limit check. 01348 * The limit check is identified with the LimitCheckId. 01349 * 01350 * @note This function doesn't Access to the device 01351 * 01352 * @param dev Device Handle 01353 * @param limit_check_id Limit Check ID 01354 * (0<= LimitCheckId < VL53L0X_GetNumberOfLimitCheck() ). 01355 * @param limit_check_enable if 1 the check limit 01356 * corresponding to LimitCheckId is Enabled 01357 * if 0 the check limit 01358 * corresponding to LimitCheckId is disabled 01359 * @return VL53L0X_ERROR_NONE Success 01360 * @return VL53L0X_ERROR_INVALID_PARAMS This error is returned 01361 * when LimitCheckId value is out of range. 01362 * @return "Other error code" See ::VL53L0X_Error 01363 */ 01364 VL53L0X_Error VL53L0X_set_limit_check_enable(VL53L0X_DEV dev, uint16_t limit_check_id, 01365 uint8_t limit_check_enable); 01366 01367 /** 01368 * @brief Get a specific limit check value 01369 * 01370 * @par Function Description 01371 * This function get a specific limit check value from device then it updates 01372 * internal values and check enables. 01373 * The limit check is identified with the LimitCheckId. 01374 * 01375 * @note This function Access to the device 01376 * 01377 * @param dev Device Handle 01378 * @param limit_check_id Limit Check ID 01379 * (0<= LimitCheckId < VL53L0X_GetNumberOfLimitCheck() ). 01380 * @param p_limit_check_value Pointer to Limit 01381 * check Value for a given LimitCheckId. 01382 * @return VL53L0X_ERROR_NONE Success 01383 * @return VL53L0X_ERROR_INVALID_PARAMS This error is returned 01384 * when LimitCheckId value is out of range. 01385 * @return "Other error code" See ::VL53L0X_Error 01386 */ 01387 VL53L0X_Error VL53L0X_get_limit_check_value(VL53L0X_DEV dev, uint16_t limit_check_id, 01388 FixPoint1616_t *p_limit_check_value); 01389 01390 /** 01391 * @brief Set a specific limit check value 01392 * 01393 * @par Function Description 01394 * This function set a specific limit check value. 01395 * The limit check is identified with the LimitCheckId. 01396 * 01397 * @note This function Access to the device 01398 * 01399 * @param dev Device Handle 01400 * @param limit_check_id Limit Check ID 01401 * (0<= LimitCheckId < VL53L0X_GetNumberOfLimitCheck() ). 01402 * @param limit_check_value Limit check Value for a given 01403 * LimitCheckId 01404 * @return VL53L0X_ERROR_NONE Success 01405 * @return VL53L0X_ERROR_INVALID_PARAMS This error is returned when either 01406 * LimitCheckId or LimitCheckValue value is out of range. 01407 * @return "Other error code" See ::VL53L0X_Error 01408 */ 01409 VL53L0X_Error VL53L0X_set_limit_check_value(VL53L0X_DEV dev, uint16_t limit_check_id, 01410 FixPoint1616_t limit_check_value); 01411 01412 /** 01413 * @brief Get the current value of the signal used for the limit check 01414 * 01415 * @par Function Description 01416 * This function get a the current value of the signal used for the limit check. 01417 * To obtain the latest value you should run a ranging before. 01418 * The value reported is linked to the limit check identified with the 01419 * LimitCheckId. 01420 * 01421 * @note This function Access to the device 01422 * 01423 * @param dev Device Handle 01424 * @param limit_check_id Limit Check ID 01425 * (0<= LimitCheckId < VL53L0X_GetNumberOfLimitCheck() ). 01426 * @param p_limit_check_current Pointer to current Value for a 01427 * given LimitCheckId. 01428 * @return VL53L0X_ERROR_NONE Success 01429 * @return VL53L0X_ERROR_INVALID_PARAMS This error is returned when 01430 * LimitCheckId value is out of range. 01431 * @return "Other error code" See ::VL53L0X_Error 01432 */ 01433 VL53L0X_Error VL53L0X_get_limit_check_current(VL53L0X_DEV dev, uint16_t limit_check_id, 01434 FixPoint1616_t *p_limit_check_current); 01435 01436 /** 01437 * @brief Return a the Status of the specified check limit 01438 * 01439 * @par Function Description 01440 * This function returns the Status of the specified check limit. 01441 * The value indicate if the check is fail or not. 01442 * The limit check is identified with the LimitCheckId. 01443 * 01444 * @note This function doesn't Access to the device 01445 * 01446 * @param dev Device Handle 01447 * @param limit_check_id Limit Check ID 01448 (0<= LimitCheckId < VL53L0X_GetNumberOfLimitCheck() ). 01449 * @param p_limit_check_status Pointer to the 01450 Limit Check Status of the given check limit. 01451 * LimitCheckStatus : 01452 * 0 the check is not fail 01453 * 1 the check if fail or not enabled 01454 * 01455 * @return VL53L0X_ERROR_NONE Success 01456 * @return VL53L0X_ERROR_INVALID_PARAMS This error is 01457 returned when LimitCheckId value is out of range. 01458 * @return "Other error code" See ::VL53L0X_Error 01459 */ 01460 VL53L0X_Error VL53L0X_get_limit_check_status(VL53L0X_DEV dev, 01461 uint16_t limit_check_id, uint8_t *p_limit_check_status); 01462 01463 /** 01464 * Get continuous mode Inter-Measurement period in milliseconds 01465 * 01466 * @par Function Description 01467 * When trying to set too short time return INVALID_PARAMS minimal value 01468 * 01469 * @note This function Access to the device 01470 * 01471 * @param dev Device Handle 01472 * @param p_inter_measurement_period_milli_seconds Pointer to programmed 01473 * Inter-Measurement Period in milliseconds. 01474 * @return VL53L0X_ERROR_NONE Success 01475 * @return "Other error code" See ::VL53L0X_Error 01476 */ 01477 VL53L0X_Error VL53L0X_get_inter_measurement_period_milli_seconds(VL53L0X_DEV dev, 01478 uint32_t *p_inter_measurement_period_milli_seconds); 01479 01480 /** 01481 * Program continuous mode Inter-Measurement period in milliseconds 01482 * 01483 * @par Function Description 01484 * When trying to set too short time return INVALID_PARAMS minimal value 01485 * 01486 * @note This function Access to the device 01487 * 01488 * @param dev Device Handle 01489 * @param inter_measurement_period_milli_seconds Inter-Measurement Period in ms. 01490 * @return VL53L0X_ERROR_NONE Success 01491 * @return "Other error code" See ::VL53L0X_Error 01492 */ 01493 VL53L0X_Error VL53L0X_set_inter_measurement_period_milli_seconds( 01494 VL53L0X_DEV dev, uint32_t inter_measurement_period_milli_seconds); 01495 01496 /** 01497 * @brief Set new device address 01498 * 01499 * After completion the device will answer to the new address programmed. 01500 * This function should be called when several devices are used in parallel 01501 * before start programming the sensor. 01502 * When a single device us used, there is no need to call this function. 01503 * 01504 * @note This function Access to the device 01505 * 01506 * @param dev Device Handle 01507 * @param device_address The new Device address 01508 * @return VL53L0X_ERROR_NONE Success 01509 * @return "Other error code" See ::VL53L0X_Error 01510 */ 01511 VL53L0X_Error VL53L0X_set_device_address(VL53L0X_DEV dev, uint8_t device_address); 01512 01513 /** 01514 * @brief Do an hard reset or soft reset (depending on implementation) of the 01515 * device \nAfter call of this function, device must be in same state as right 01516 * after a power-up sequence.This function will change the VL53L0X_State to 01517 * VL53L0X_STATE_POWERDOWN. 01518 * 01519 * @note This function Access to the device 01520 * 01521 * @param dev Device Handle 01522 * @return VL53L0X_ERROR_NONE Success 01523 * @return "Other error code" See ::VL53L0X_Error 01524 */ 01525 VL53L0X_Error VL53L0X_reset_device(VL53L0X_DEV dev); 01526 01527 /** 01528 * @brief Get setup of Wrap around Check 01529 * 01530 * @par Function Description 01531 * This function get the wrapAround check enable parameters 01532 * 01533 * @note This function Access to the device 01534 * 01535 * @param dev Device Handle 01536 * @param p_wrap_around_check_enable Pointer to the Wrap around Check state 01537 * 0=disabled or 1 = enabled 01538 * @return VL53L0X_ERROR_NONE Success 01539 * @return "Other error code" See ::VL53L0X_Error 01540 */ 01541 VL53L0X_Error VL53L0X_get_wrap_around_check_enable(VL53L0X_DEV dev, 01542 uint8_t *p_wrap_around_check_enable); 01543 01544 /** 01545 * @brief Enable (or disable) Wrap around Check 01546 * 01547 * @note This function Access to the device 01548 * 01549 * @param dev Device Handle 01550 * @param wrap_around_check_enable Wrap around Check to be set 01551 * 0=disabled, other = enabled 01552 * @return VL53L0X_ERROR_NONE Success 01553 * @return "Other error code" See ::VL53L0X_Error 01554 */ 01555 VL53L0X_Error VL53L0X_set_wrap_around_check_enable(VL53L0X_DEV dev, 01556 uint8_t wrap_around_check_enable); 01557 01558 /** 01559 * @brief Gets the VCSEL pulse period. 01560 * 01561 * @par Function Description 01562 * This function retrieves the VCSEL pulse period for the given period type. 01563 * 01564 * @note This function Accesses the device 01565 * 01566 * @param dev Device Handle 01567 * @param vcsel_period_type VCSEL period identifier (pre-range|final). 01568 * @param p_vcsel_pulse_period_pclk Pointer to VCSEL period value. 01569 * @return VL53L0X_ERROR_NONE Success 01570 * @return VL53L0X_ERROR_INVALID_PARAMS Error VcselPeriodType parameter not 01571 * supported. 01572 * @return "Other error code" See ::VL53L0X_Error 01573 */ 01574 VL53L0X_Error VL53L0X_get_vcsel_pulse_period(VL53L0X_DEV dev, 01575 VL53L0X_VcselPeriod vcsel_period_type, uint8_t *p_vcsel_pulse_period_pclk); 01576 01577 /** 01578 * @brief Sets the VCSEL pulse period. 01579 * 01580 * @par Function Description 01581 * This function retrieves the VCSEL pulse period for the given period type. 01582 * 01583 * @note This function Accesses the device 01584 * 01585 * @param dev Device Handle 01586 * @param vcsel_period_type VCSEL period identifier (pre-range|final). 01587 * @param vcsel_pulse_period VCSEL period value 01588 * @return VL53L0X_ERROR_NONE Success 01589 * @return VL53L0X_ERROR_INVALID_PARAMS Error VcselPeriodType parameter not 01590 * supported. 01591 * @return "Other error code" See ::VL53L0X_Error 01592 */ 01593 VL53L0X_Error VL53L0X_set_vcsel_pulse_period(VL53L0X_DEV dev, 01594 VL53L0X_VcselPeriod vcsel_period_type, uint8_t vcsel_pulse_period); 01595 01596 /** 01597 * @brief Set low and high Interrupt thresholds for a given mode 01598 * (ranging, ALS, ...) for a given device 01599 * 01600 * @par Function Description 01601 * Set low and high Interrupt thresholds for a given mode (ranging, ALS, ...) 01602 * for a given device 01603 * 01604 * @note This function Access to the device 01605 * 01606 * @note DeviceMode is ignored for the current device 01607 * 01608 * @param dev Device Handle 01609 * @param device_mode Device Mode for which change thresholds 01610 * @param threshold_low Low threshold (mm, lux ..., depending on the mode) 01611 * @param threshold_high High threshold (mm, lux ..., depending on the mode) 01612 * @return VL53L0X_ERROR_NONE Success 01613 * @return "Other error code" See ::VL53L0X_Error 01614 */ 01615 VL53L0X_Error VL53L0X_set_interrupt_thresholds(VL53L0X_DEV dev, 01616 VL53L0X_DeviceModes device_mode, FixPoint1616_t threshold_low, 01617 FixPoint1616_t threshold_high); 01618 01619 /** 01620 * @brief Get high and low Interrupt thresholds for a given mode 01621 * (ranging, ALS, ...) for a given device 01622 * 01623 * @par Function Description 01624 * Get high and low Interrupt thresholds for a given mode (ranging, ALS, ...) 01625 * for a given device 01626 * 01627 * @note This function Access to the device 01628 * 01629 * @note DeviceMode is ignored for the current device 01630 * 01631 * @param dev Device Handle 01632 * @param device_mode Device Mode from which read thresholds 01633 * @param p_threshold_low Low threshold (mm, lux ..., depending on the mode) 01634 * @param p_threshold_high High threshold (mm, lux ..., depending on the mode) 01635 * @return VL53L0X_ERROR_NONE Success 01636 * @return "Other error code" See ::VL53L0X_Error 01637 */ 01638 VL53L0X_Error VL53L0X_get_interrupt_thresholds(VL53L0X_DEV dev, 01639 VL53L0X_DeviceModes device_mode, FixPoint1616_t *p_threshold_low, 01640 FixPoint1616_t *p_threshold_high); 01641 01642 /** 01643 * @brief Reads the Device information for given Device 01644 * 01645 * @note This function Access to the device 01646 * 01647 * @param dev Device Handle 01648 * @param p_VL53L0X_device_info Pointer to current device info for a given 01649 * Device 01650 * @return VL53L0X_ERROR_NONE Success 01651 * @return "Other error code" See ::VL53L0X_Error 01652 */ 01653 VL53L0X_Error VL53L0X_get_device_info(VL53L0X_DEV dev, 01654 VL53L0X_DeviceInfo_t *p_VL53L0X_device_info); 01655 01656 /** 01657 * @brief Gets the (on/off) state of all sequence steps. 01658 * 01659 * @par Function Description 01660 * This function retrieves the state of all sequence step in the scheduler. 01661 * 01662 * @note This function Accesses the device 01663 * 01664 * @param dev Device Handle 01665 * @param p_scheduler_sequence_steps Pointer to struct containing result. 01666 * @return VL53L0X_ERROR_NONE Success 01667 * @return "Other error code" See ::VL53L0X_Error 01668 */ 01669 VL53L0X_Error VL53L0X_get_sequence_step_enables(VL53L0X_DEV dev, 01670 VL53L0X_SchedulerSequenceSteps_t *p_scheduler_sequence_steps); 01671 01672 /** 01673 * @brief Sets the (on/off) state of a requested sequence step. 01674 * 01675 * @par Function Description 01676 * This function enables/disables a requested sequence step. 01677 * 01678 * @note This function Accesses the device 01679 * 01680 * @param dev Device Handle 01681 * @param sequence_step_id Sequence step identifier. 01682 * @param sequence_step_enabled Demanded state {0=Off,1=On} 01683 * is enabled. 01684 * @return VL53L0X_ERROR_NONE Success 01685 * @return VL53L0X_ERROR_INVALID_PARAMS Error SequenceStepId parameter not 01686 * supported. 01687 * @return "Other error code" See ::VL53L0X_Error 01688 */ 01689 VL53L0X_Error VL53L0X_set_sequence_step_enable(VL53L0X_DEV dev, 01690 VL53L0X_SequenceStepId sequence_step_id, uint8_t sequence_step_enabled); 01691 01692 /** 01693 * @brief Gets the fraction enable parameter indicating the resolution of 01694 * range measurements. 01695 * 01696 * @par Function Description 01697 * Gets the fraction enable state, which translates to the resolution of 01698 * range measurements as follows :Enabled:=0.25mm resolution, 01699 * Not Enabled:=1mm resolution. 01700 * 01701 * @note This function Accesses the device 01702 * 01703 * @param dev Device Handle 01704 * @param p_enabled Output Parameter reporting the fraction enable state. 01705 * 01706 * @return VL53L0X_ERROR_NONE Success 01707 * @return "Other error code" See ::VL53L0X_Error 01708 */ 01709 VL53L0X_Error VL53L0X_get_fraction_enable(VL53L0X_DEV dev, uint8_t *p_enabled); 01710 01711 /** 01712 * @brief Sets the resolution of range measurements. 01713 * @par Function Description 01714 * Set resolution of range measurements to either 0.25mm if 01715 * fraction enabled or 1mm if not enabled. 01716 * 01717 * @note This function Accesses the device 01718 * 01719 * @param dev Device Handle 01720 * @param enable Enable high resolution 01721 * 01722 * @return VL53L0X_ERROR_NONE Success 01723 * @return "Other error code" See ::VL53L0X_Error 01724 */ 01725 VL53L0X_Error VL53L0X_set_range_fraction_enable(VL53L0X_DEV dev, 01726 uint8_t enable); 01727 01728 /** 01729 * @brief Return the VL53L0X PAL Implementation Version 01730 * 01731 * @note This function doesn't access to the device 01732 * 01733 * @param p_version Pointer to current PAL Implementation Version 01734 * @return VL53L0X_ERROR_NONE Success 01735 * @return "Other error code" See ::VL53L0X_Error 01736 */ 01737 VL53L0X_Error VL53L0X_get_version(VL53L0X_Version_t *p_version); 01738 01739 /** 01740 * @brief Reads the Product Revision for a for given Device 01741 * This function can be used to distinguish cut1.0 from cut1.1. 01742 * 01743 * @note This function Access to the device 01744 * 01745 * @param dev Device Handle 01746 * @param p_product_revision_major Pointer to Product Revision Major 01747 * for a given Device 01748 * @param p_product_revision_minor Pointer to Product Revision Minor 01749 * for a given Device 01750 * @return VL53L0X_ERROR_NONE Success 01751 * @return "Other error code" See ::VL53L0X_Error 01752 */ 01753 VL53L0X_Error VL53L0X_get_product_revision(VL53L0X_DEV dev, 01754 uint8_t *p_product_revision_major, uint8_t *p_product_revision_minor); 01755 01756 /** 01757 * @brief Retrieve current device parameters 01758 * @par Function Description 01759 * Get actual parameters of the device 01760 * @li Then start ranging operation. 01761 * 01762 * @note This function Access to the device 01763 * 01764 * @param dev Device Handle 01765 * @param p_device_parameters Pointer to store current device parameters. 01766 * @return VL53L0X_ERROR_NONE Success 01767 * @return "Other error code" See ::VL53L0X_Error 01768 */ 01769 VL53L0X_Error VL53L0X_get_device_parameters(VL53L0X_DEV dev, 01770 VL53L0X_DeviceParameters_t *p_device_parameters); 01771 01772 /** 01773 * @brief Human readable error string for current PAL error status 01774 * 01775 * @note This function doesn't access to the device 01776 * 01777 * @param pal_error_code The error code as stored on @a VL53L0X_Error 01778 * @param p_pal_error_string The error string corresponding to the 01779 * PalErrorCode 01780 * @return VL53L0X_ERROR_NONE Success 01781 * @return "Other error code" See ::VL53L0X_Error 01782 */ 01783 VL53L0X_Error VL53L0X_get_pal_error_string(VL53L0X_Error pal_error_code, 01784 char *p_pal_error_string); 01785 01786 /** 01787 * @brief Return the PAL Specification Version used for the current 01788 * implementation. 01789 * 01790 * @note This function doesn't access to the device 01791 * 01792 * @param p_pal_spec_version Pointer to current PAL Specification Version 01793 * @return VL53L0X_ERROR_NONE Success 01794 * @return "Other error code" See ::VL53L0X_Error 01795 */ 01796 VL53L0X_Error VL53L0X_get_pal_spec_version( 01797 VL53L0X_Version_t *p_pal_spec_version); 01798 01799 /** 01800 * @brief Reads the internal state of the PAL for a given Device 01801 * 01802 * @note This function doesn't access to the device 01803 * 01804 * @param dev Device Handle 01805 * @param p_pal_state Pointer to current state of the PAL for a 01806 * given Device 01807 * @return VL53L0X_ERROR_NONE Success 01808 * @return "Other error code" See ::VL53L0X_Error 01809 */ 01810 VL53L0X_Error VL53L0X_get_pal_state(VL53L0X_DEV dev, 01811 VL53L0X_State *p_pal_state); 01812 01813 /** 01814 * @brief Human readable PAL State string 01815 * 01816 * @note This function doesn't access to the device 01817 * 01818 * @param pal_state_code The State code as stored on @a VL53L0X_State 01819 * @param p_pal_state_string The State string corresponding to the 01820 * PalStateCode 01821 * @return VL53L0X_ERROR_NONE Success 01822 * @return "Other error code" See ::VL53L0X_Error 01823 */ 01824 VL53L0X_Error VL53L0X_get_pal_state_string(VL53L0X_State pal_state_code, 01825 char *p_pal_state_string); 01826 01827 /*** End High level API ***/ 01828 private: 01829 /* api.h functions */ 01830 01831 /** 01832 * @brief Wait for device booted after chip enable (hardware standby) 01833 * This function can be run only when VL53L0X_State is VL53L0X_STATE_POWERDOWN. 01834 * 01835 * @note This function is not Implemented 01836 * 01837 * @param dev Device Handle 01838 * @return VL53L0X_ERROR_NOT_IMPLEMENTED Not implemented 01839 * 01840 */ 01841 VL53L0X_Error VL53L0X_wait_device_booted(VL53L0X_DEV dev); 01842 01843 01844 VL53L0X_Error sequence_step_enabled(VL53L0X_DEV dev, 01845 VL53L0X_SequenceStepId sequence_step_id, uint8_t sequence_config, 01846 uint8_t *p_sequence_step_enabled); 01847 01848 VL53L0X_Error VL53L0X_check_and_load_interrupt_settings(VL53L0X_DEV dev, 01849 uint8_t start_not_stopflag); 01850 01851 01852 /* api_core.h functions */ 01853 01854 VL53L0X_Error VL53L0X_get_info_from_device(VL53L0X_DEV dev, uint8_t option); 01855 01856 VL53L0X_Error VL53L0X_device_read_strobe(VL53L0X_DEV dev); 01857 01858 VL53L0X_Error wrapped_VL53L0X_get_measurement_timing_budget_micro_seconds(VL53L0X_DEV dev, 01859 uint32_t *p_measurement_timing_budget_micro_seconds); 01860 01861 VL53L0X_Error wrapped_VL53L0X_get_vcsel_pulse_period(VL53L0X_DEV dev, 01862 VL53L0X_VcselPeriod vcsel_period_type, uint8_t *p_vcsel_pulse_period_pclk); 01863 01864 uint8_t VL53L0X_decode_vcsel_period(uint8_t vcsel_period_reg); 01865 01866 uint32_t VL53L0X_decode_timeout(uint16_t encoded_timeout); 01867 01868 uint32_t VL53L0X_calc_timeout_us(VL53L0X_DEV dev, 01869 uint16_t timeout_period_mclks, 01870 uint8_t vcsel_period_pclks); 01871 01872 uint32_t VL53L0X_calc_macro_period_ps(VL53L0X_DEV dev, uint8_t vcsel_period_pclks); 01873 01874 VL53L0X_Error VL53L0X_measurement_poll_for_completion(VL53L0X_DEV dev); 01875 01876 VL53L0X_Error VL53L0X_load_tuning_settings(VL53L0X_DEV dev, 01877 uint8_t *p_tuning_setting_buffer); 01878 01879 VL53L0X_Error VL53L0X_get_pal_range_status(VL53L0X_DEV dev, 01880 uint8_t device_range_status, 01881 FixPoint1616_t signal_rate, 01882 uint16_t effective_spad_rtn_count, 01883 VL53L0X_RangingMeasurementData_t *p_ranging_measurement_data, 01884 uint8_t *p_pal_range_status); 01885 VL53L0X_Error VL53L0X_calc_sigma_estimate(VL53L0X_DEV dev, 01886 VL53L0X_RangingMeasurementData_t *p_ranging_measurement_data, 01887 FixPoint1616_t *p_sigma_estimate, 01888 uint32_t *p_dmax_mm); 01889 uint32_t VL53L0X_calc_timeout_mclks(VL53L0X_DEV dev, 01890 uint32_t timeout_period_us, 01891 uint8_t vcsel_period_pclks); 01892 uint32_t VL53L0X_isqrt(uint32_t num); 01893 01894 uint32_t VL53L0X_quadrature_sum(uint32_t a, uint32_t b); 01895 01896 VL53L0X_Error VL53L0X_calc_dmax( 01897 VL53L0X_DEV dev, 01898 FixPoint1616_t total_signal_rate_mcps, 01899 FixPoint1616_t total_corr_signal_rate_mcps, 01900 FixPoint1616_t pw_mult, 01901 uint32_t sigma_estimate_p1, 01902 FixPoint1616_t sigma_estimate_p2, 01903 uint32_t peak_vcsel_duration_us, 01904 uint32_t *pd_max_mm); 01905 VL53L0X_Error wrapped_VL53L0X_set_measurement_timing_budget_micro_seconds(VL53L0X_DEV dev, 01906 uint32_t measurement_timing_budget_micro_seconds); 01907 VL53L0X_Error get_sequence_step_timeout(VL53L0X_DEV dev, 01908 VL53L0X_SequenceStepId sequence_step_id, 01909 uint32_t *p_time_out_micro_secs); 01910 VL53L0X_Error set_sequence_step_timeout(VL53L0X_DEV dev, 01911 VL53L0X_SequenceStepId sequence_step_id, 01912 uint32_t timeout_micro_secs); 01913 uint16_t VL53L0X_encode_timeout(uint32_t timeout_macro_clks); 01914 VL53L0X_Error wrapped_VL53L0X_set_vcsel_pulse_period(VL53L0X_DEV dev, 01915 VL53L0X_VcselPeriod vcsel_period_type, uint8_t vcsel_pulse_period_pclk); 01916 uint8_t lv53l0x_encode_vcsel_period(uint8_t vcsel_period_pclks); 01917 01918 /* api_calibration.h functions */ 01919 VL53L0X_Error VL53L0X_apply_offset_adjustment(VL53L0X_DEV dev); 01920 VL53L0X_Error wrapped_VL53L0X_get_offset_calibration_data_micro_meter(VL53L0X_DEV dev, 01921 int32_t *p_offset_calibration_data_micro_meter); 01922 VL53L0X_Error wrapped_VL53L0X_set_offset_calibration_data_micro_meter(VL53L0X_DEV dev, 01923 int32_t offset_calibration_data_micro_meter); 01924 VL53L0X_Error wrapped_VL53L0X_perform_ref_spad_management(VL53L0X_DEV dev, 01925 uint32_t *ref_spad_count, 01926 uint8_t *is_aperture_spads); 01927 VL53L0X_Error VL53L0X_perform_ref_calibration(VL53L0X_DEV dev, 01928 uint8_t *p_vhv_settings, uint8_t *p_phase_cal, uint8_t get_data_enable); 01929 VL53L0X_Error VL53L0X_perform_vhv_calibration(VL53L0X_DEV dev, 01930 uint8_t *p_vhv_settings, const uint8_t get_data_enable, 01931 const uint8_t restore_config); 01932 VL53L0X_Error VL53L0X_perform_single_ref_calibration(VL53L0X_DEV dev, 01933 uint8_t vhv_init_byte); 01934 VL53L0X_Error VL53L0X_ref_calibration_io(VL53L0X_DEV dev, uint8_t read_not_write, 01935 uint8_t vhv_settings, uint8_t phase_cal, 01936 uint8_t *p_vhv_settings, uint8_t *p_phase_cal, 01937 const uint8_t vhv_enable, const uint8_t phase_enable); 01938 VL53L0X_Error VL53L0X_perform_phase_calibration(VL53L0X_DEV dev, 01939 uint8_t *p_phase_cal, const uint8_t get_data_enable, 01940 const uint8_t restore_config); 01941 VL53L0X_Error enable_ref_spads(VL53L0X_DEV dev, 01942 uint8_t aperture_spads, 01943 uint8_t good_spad_array[], 01944 uint8_t spad_array[], 01945 uint32_t size, 01946 uint32_t start, 01947 uint32_t offset, 01948 uint32_t spad_count, 01949 uint32_t *p_last_spad); 01950 void get_next_good_spad(uint8_t good_spad_array[], uint32_t size, 01951 uint32_t curr, int32_t *p_next); 01952 uint8_t is_aperture(uint32_t spad_index); 01953 VL53L0X_Error enable_spad_bit(uint8_t spad_array[], uint32_t size, 01954 uint32_t spad_index); 01955 VL53L0X_Error set_ref_spad_map(VL53L0X_DEV dev, uint8_t *p_ref_spad_array); 01956 VL53L0X_Error get_ref_spad_map(VL53L0X_DEV dev, uint8_t *p_ref_spad_array); 01957 VL53L0X_Error perform_ref_signal_measurement(VL53L0X_DEV dev, 01958 uint16_t *p_ref_signal_rate); 01959 VL53L0X_Error wrapped_VL53L0X_set_reference_spads(VL53L0X_DEV dev, 01960 uint32_t count, uint8_t is_aperture_spads); 01961 01962 /* api_strings.h functions */ 01963 VL53L0X_Error wrapped_VL53L0X_get_device_info(VL53L0X_DEV dev, 01964 VL53L0X_DeviceInfo_t *p_VL53L0X_device_info); 01965 VL53L0X_Error VL53L0X_check_part_used(VL53L0X_DEV dev, 01966 uint8_t *revision, 01967 VL53L0X_DeviceInfo_t *p_VL53L0X_device_info); 01968 01969 /* Read function of the ID device */ 01970 // virtual int read_id(); 01971 virtual int read_id(uint8_t *id); 01972 01973 VL53L0X_Error wait_measurement_data_ready(VL53L0X_DEV dev); 01974 01975 VL53L0X_Error wait_stop_completed(VL53L0X_DEV dev); 01976 01977 /* Write and read functions from I2C */ 01978 /** 01979 * Write single byte register 01980 * @param dev Device Handle 01981 * @param index The register index 01982 * @param data 8 bit register data 01983 * @return VL53L0X_ERROR_NONE Success 01984 * @return "Other error code" See ::VL53L0X_Error 01985 */ 01986 VL53L0X_Error VL53L0X_write_byte(VL53L0X_DEV dev, uint8_t index, uint8_t data); 01987 /** 01988 * Write word register 01989 * @param dev Device Handle 01990 * @param index The register index 01991 * @param data 16 bit register data 01992 * @return VL53L0X_ERROR_NONE Success 01993 * @return "Other error code" See ::VL53L0X_Error 01994 */ 01995 VL53L0X_Error VL53L0X_write_word(VL53L0X_DEV dev, uint8_t index, uint16_t data); 01996 /** 01997 * Write double word (4 byte) register 01998 * @param dev Device Handle 01999 * @param index The register index 02000 * @param data 32 bit register data 02001 * @return VL53L0X_ERROR_NONE Success 02002 * @return "Other error code" See ::VL53L0X_Error 02003 */ 02004 VL53L0X_Error VL53L0X_write_dword(VL53L0X_DEV dev, uint8_t index, uint32_t data); 02005 /** 02006 * Read single byte register 02007 * @param dev Device Handle 02008 * @param index The register index 02009 * @param data pointer to 8 bit data 02010 * @return VL53L0X_ERROR_NONE Success 02011 * @return "Other error code" See ::VL53L0X_Error 02012 */ 02013 VL53L0X_Error VL53L0X_read_byte(VL53L0X_DEV dev, uint8_t index, uint8_t *p_data); 02014 /** 02015 * Read word (2byte) register 02016 * @param dev Device Handle 02017 * @param index The register index 02018 * @param data pointer to 16 bit data 02019 * @return VL53L0X_ERROR_NONE Success 02020 * @return "Other error code" See ::VL53L0X_Error 02021 */ 02022 VL53L0X_Error VL53L0X_read_word(VL53L0X_DEV dev, uint8_t index, uint16_t *p_data); 02023 /** 02024 * Read dword (4byte) register 02025 * @param dev Device Handle 02026 * @param index The register index 02027 * @param data pointer to 32 bit data 02028 * @return VL53L0X_ERROR_NONE Success 02029 * @return "Other error code" See ::VL53L0X_Error 02030 */ 02031 VL53L0X_Error VL53L0X_read_dword(VL53L0X_DEV dev, uint8_t index, uint32_t *p_data); 02032 /** 02033 * Threat safe Update (read/modify/write) single byte register 02034 * 02035 * Final_reg = (Initial_reg & and_data) |or_data 02036 * 02037 * @param dev Device Handle 02038 * @param index The register index 02039 * @param and_data 8 bit and data 02040 * @param or_data 8 bit or data 02041 * @return VL53L0X_ERROR_NONE Success 02042 * @return "Other error code" See ::VL53L0X_Error 02043 */ 02044 VL53L0X_Error VL53L0X_update_byte(VL53L0X_DEV dev, uint8_t index, uint8_t and_data, uint8_t or_data); 02045 /** 02046 * Writes the supplied byte buffer to the device 02047 * @param dev Device Handle 02048 * @param index The register index 02049 * @param p_data Pointer to uint8_t buffer containing the data to be written 02050 * @param count Number of bytes in the supplied byte buffer 02051 * @return VL53L0X_ERROR_NONE Success 02052 * @return "Other error code" See ::VL53L0X_Error 02053 */ 02054 VL53L0X_Error VL53L0X_write_multi(VL53L0X_DEV dev, uint8_t index, uint8_t *p_data, uint32_t count); 02055 /** 02056 * Reads the requested number of bytes from the device 02057 * @param dev Device Handle 02058 * @param index The register index 02059 * @param p_data Pointer to the uint8_t buffer to store read data 02060 * @param count Number of uint8_t's to read 02061 * @return VL53L0X_ERROR_NONE Success 02062 * @return "Other error code" See ::VL53L0X_Error 02063 */ 02064 VL53L0X_Error VL53L0X_read_multi(VL53L0X_DEV dev, uint8_t index, uint8_t *p_data, uint32_t count); 02065 02066 /** 02067 * @brief Writes a buffer towards the I2C peripheral device. 02068 * @param dev Device Handle 02069 * @param p_data pointer to the byte-array data to send 02070 * @param number_of_bytes number of bytes to be written. 02071 * @retval 0 if ok, 02072 * @retval -1 if an I2C error has occured 02073 * @note On some devices if NumByteToWrite is greater 02074 * than one, the RegisterAddr must be masked correctly! 02075 */ 02076 VL53L0X_Error VL53L0X_i2c_write(uint8_t dev, uint8_t index, uint8_t *p_data, uint16_t number_of_bytes); 02077 02078 /** 02079 * @brief Reads a buffer from the I2C peripheral device. 02080 * @param dev Device Handle 02081 * @param p_data pointer to the byte-array to read data in to 02082 * @param number_of_bytes number of bytes to be read. 02083 * @retval 0 if ok, 02084 * @retval -1 if an I2C error has occured 02085 * @note On some devices if NumByteToWrite is greater 02086 * than one, the RegisterAddr must be masked correctly! 02087 */ 02088 VL53L0X_Error VL53L0X_i2c_read(uint8_t dev, uint8_t index, uint8_t *p_data, uint16_t number_of_bytes); 02089 02090 /** 02091 * @brief execute delay in all polling API call 02092 * 02093 * A typical multi-thread or RTOs implementation is to sleep the task for some 5ms (with 100Hz max rate faster polling is not needed) 02094 * if nothing specific is need you can define it as an empty/void macro 02095 * @code 02096 * #define VL53L0X_PollingDelay(...) (void)0 02097 * @endcode 02098 * @param dev Device Handle 02099 * @return VL53L0X_ERROR_NONE Success 02100 * @return "Other error code" See ::VL53L0X_Error 02101 */ 02102 VL53L0X_Error VL53L0X_polling_delay(VL53L0X_DEV dev); /* usually best implemented as a real function */ 02103 02104 int is_present() 02105 { 02106 int status; 02107 uint8_t id = 0; 02108 02109 status = read_id(&id); 02110 if (status) { 02111 VL53L0X_ErrLog("Failed to read ID device. Device not present!\n\r"); 02112 } 02113 return status; 02114 } 02115 02116 /////////////////////////////////////////////////////////////////////////////////////////////////////// 02117 //Added functions // 02118 /////////////////////////////////////////////////////////////////////////////////////////////////////// 02119 02120 /** 02121 * @brief Cycle Power to Device 02122 * 02123 * @return status - status 0 = ok, 1 = error 02124 * 02125 */ 02126 int32_t VL53L0X_cycle_power(void); 02127 02128 uint8_t VL53L0X_encode_vcsel_period(uint8_t vcsel_period_pclks); 02129 02130 VL53L0X_Error wrapped_VL53L0X_get_device_error_string(VL53L0X_DeviceError error_code, 02131 char *p_device_error_string); 02132 02133 VL53L0X_Error wrapped_VL53L0X_get_limit_check_info(VL53L0X_DEV dev, uint16_t limit_check_id, 02134 char *p_limit_check_string); 02135 02136 VL53L0X_Error wrapped_VL53L0X_get_pal_error_string(VL53L0X_Error pal_error_code, 02137 char *p_pal_error_string); 02138 02139 VL53L0X_Error wrapped_VL53L0X_get_pal_state_string(VL53L0X_State pal_state_code, 02140 char *p_pal_state_string); 02141 02142 VL53L0X_Error wrapped_VL53L0X_get_range_status_string(uint8_t range_status, 02143 char *p_range_status_string); 02144 02145 VL53L0X_Error wrapped_VL53L0X_get_ref_calibration(VL53L0X_DEV dev, 02146 uint8_t *p_vhv_settings, uint8_t *p_phase_cal); 02147 02148 02149 VL53L0X_Error count_enabled_spads(uint8_t spad_array[], 02150 uint32_t byte_count, uint32_t max_spads, 02151 uint32_t *p_total_spads_enabled, uint8_t *p_is_aperture); 02152 02153 VL53L0X_Error wrapped_VL53L0X_get_sequence_steps_info(VL53L0X_SequenceStepId sequence_step_id, 02154 char *p_sequence_steps_string); 02155 02156 02157 /** 02158 * @brief Gets the name of a given sequence step. 02159 * 02160 * @par Function Description 02161 * This function retrieves the name of sequence steps corresponding to 02162 * SequenceStepId. 02163 * 02164 * @note This function doesn't Accesses the device 02165 * 02166 * @param sequence_step_id Sequence step identifier. 02167 * @param p_sequence_steps_string Pointer to Info string 02168 * 02169 * @return VL53L0X_ERROR_NONE Success 02170 * @return "Other error code" See ::VL53L0X_Error 02171 */ 02172 VL53L0X_Error VL53L0X_get_sequence_steps_info(VL53L0X_SequenceStepId sequence_step_id, 02173 char *p_sequence_steps_string); 02174 02175 /** 02176 * @brief Get the frequency of the timer used for ranging results time stamps 02177 * 02178 * @param[out] p_timer_freq_hz : pointer for timer frequency 02179 * 02180 * @return status : 0 = ok, 1 = error 02181 * 02182 */ 02183 int32_t VL53L0X_get_timer_frequency(int32_t *p_timer_freq_hz); 02184 02185 /** 02186 * @brief Get the timer value in units of timer_freq_hz (see VL53L0X_get_timestamp_frequency()) 02187 * 02188 * @param[out] p_timer_count : pointer for timer count value 02189 * 02190 * @return status : 0 = ok, 1 = error 02191 * 02192 */ 02193 int32_t VL53L0X_get_timer_value(int32_t *p_timer_count); 02194 02195 /** 02196 * @brief Configure ranging interrupt reported to system 02197 * 02198 * @note This function is not Implemented 02199 * 02200 * @param dev Device Handle 02201 * @param interrupt_mask Mask of interrupt to Enable/disable 02202 * (0:interrupt disabled or 1: interrupt enabled) 02203 * @return VL53L0X_ERROR_NOT_IMPLEMENTED Not implemented 02204 */ 02205 VL53L0X_Error VL53L0X_enable_interrupt_mask(VL53L0X_DEV dev, 02206 uint32_t interrupt_mask); 02207 02208 /** 02209 * @brief Get Dmax Calibration Parameters for a given device 02210 * 02211 * 02212 * @note This function Access to the device 02213 * 02214 * @param dev Device Handle 02215 * @param p_range_milli_meter Pointer to Calibration Distance 02216 * @param p_signal_rate_rtn_mega_cps Pointer to Signal rate return 02217 * @return VL53L0X_ERROR_NONE Success 02218 * @return "Other error code" See ::VL53L0X_Error 02219 */ 02220 VL53L0X_Error VL53L0X_get_dmax_cal_parameters(VL53L0X_DEV dev, 02221 uint16_t *p_range_milli_meter, FixPoint1616_t *p_signal_rate_rtn_mega_cps); 02222 02223 /** 02224 * @brief Set Dmax Calibration Parameters for a given device 02225 * When one of the parameter is zero, this function will get parameter 02226 * from NVM. 02227 * @note This function doesn't Access to the device 02228 * 02229 * @param dev Device Handle 02230 * @param range_milli_meter Calibration Distance 02231 * @param signal_rate_rtn_mega_cps Signal rate return read at CalDistance 02232 * @return VL53L0X_ERROR_NONE Success 02233 * @return "Other error code" See ::VL53L0X_Error 02234 */ 02235 VL53L0X_Error VL53L0X_get_dmax_cal_parameters(VL53L0X_DEV dev, 02236 uint16_t range_milli_meter, FixPoint1616_t signal_rate_rtn_mega_cps); 02237 02238 /** 02239 * @brief Retrieve the measurements from device for a given setup 02240 * 02241 * @par Function Description 02242 * Get data from last successful Histogram measurement 02243 * @warning USER should take care about @a VL53L0X_GetNumberOfROIZones() 02244 * before get data. 02245 * PAL will fill a NumberOfROIZones times the corresponding data structure 02246 * used in the measurement function. 02247 * 02248 * @note This function is not Implemented 02249 * 02250 * @param dev Device Handle 02251 * @param p_histogram_measurement_data Pointer to the histogram data structure. 02252 * @return VL53L0X_ERROR_NOT_IMPLEMENTED Not implemented 02253 */ 02254 VL53L0X_Error VL53L0X_get_histogram_measurement_data(VL53L0X_DEV dev, 02255 VL53L0X_HistogramMeasurementData_t *p_histogram_measurement_data); 02256 02257 /** 02258 * @brief Get current new device mode 02259 * @par Function Description 02260 * Get current Histogram mode of a Device 02261 * 02262 * @note This function doesn't Access to the device 02263 * 02264 * @param dev Device Handle 02265 * @param p_histogram_mode Pointer to current Histogram Mode value 02266 * Valid values are: 02267 * VL53L0X_HISTOGRAMMODE_DISABLED 02268 * VL53L0X_DEVICEMODE_SINGLE_HISTOGRAM 02269 * VL53L0X_HISTOGRAMMODE_REFERENCE_ONLY 02270 * VL53L0X_HISTOGRAMMODE_RETURN_ONLY 02271 * VL53L0X_HISTOGRAMMODE_BOTH 02272 * @return VL53L0X_ERROR_NONE Success 02273 * @return "Other error code" See ::VL53L0X_Error 02274 */ 02275 VL53L0X_Error VL53L0X_get_histogram_mode(VL53L0X_DEV dev, 02276 VL53L0X_HistogramModes *p_histogram_mode); 02277 02278 /** 02279 * @brief Set a new Histogram mode 02280 * @par Function Description 02281 * Set device to a new Histogram mode 02282 * 02283 * @note This function doesn't Access to the device 02284 * 02285 * @param dev Device Handle 02286 * @param histogram_mode New device mode to apply 02287 * Valid values are: 02288 * VL53L0X_HISTOGRAMMODE_DISABLED 02289 * VL53L0X_DEVICEMODE_SINGLE_HISTOGRAM 02290 * VL53L0X_HISTOGRAMMODE_REFERENCE_ONLY 02291 * VL53L0X_HISTOGRAMMODE_RETURN_ONLY 02292 * VL53L0X_HISTOGRAMMODE_BOTH 02293 * 02294 * @return VL53L0X_ERROR_NONE Success 02295 * @return VL53L0X_ERROR_MODE_NOT_SUPPORTED This error occurs when 02296 * HistogramMode is not in the supported list 02297 * @return "Other error code" See ::VL53L0X_Error 02298 */ 02299 VL53L0X_Error VL53L0X_set_histogram_mode(VL53L0X_DEV dev, 02300 VL53L0X_HistogramModes histogram_mode); 02301 02302 /** 02303 * @brief Return a description string for a given limit check number 02304 * 02305 * @par Function Description 02306 * This function returns a description string for a given limit check number. 02307 * The limit check is identified with the LimitCheckId. 02308 * 02309 * @note This function doesn't Access to the device 02310 * 02311 * @param dev Device Handle 02312 * @param limit_check_id Limit Check ID 02313 (0<= LimitCheckId < VL53L0X_GetNumberOfLimitCheck() ). 02314 * @param p_limit_check_string Pointer to the 02315 description string of the given check limit. 02316 * @return VL53L0X_ERROR_NONE Success 02317 * @return VL53L0X_ERROR_INVALID_PARAMS This error is 02318 returned when LimitCheckId value is out of range. 02319 * @return "Other error code" See ::VL53L0X_Error 02320 */ 02321 VL53L0X_Error VL53L0X_get_limit_check_info(VL53L0X_DEV dev, 02322 uint16_t limit_check_id, char *p_limit_check_string); 02323 02324 /** 02325 * @brief Get the linearity corrective gain 02326 * 02327 * @par Function Description 02328 * Should only be used after a successful call to @a VL53L0X_DataInit to backup 02329 * device NVM value 02330 * 02331 * @note This function Access to the device 02332 * 02333 * @param dev Device Handle 02334 * @param p_linearity_corrective_gain Pointer to the linearity 02335 * corrective gain in x1000 02336 * if value is 1000 then no modification is applied. 02337 * @return VL53L0X_ERROR_NONE Success 02338 * @return "Other error code" See ::VL53L0X_Error 02339 */ 02340 VL53L0X_Error VL53L0X_get_linearity_corrective_gain(VL53L0X_DEV dev, 02341 uint16_t *p_linearity_corrective_gain); 02342 02343 /** 02344 * Set the linearity corrective gain 02345 * 02346 * @note This function Access to the device 02347 * 02348 * @param dev Device Handle 02349 * @param linearity_corrective_gain Linearity corrective 02350 * gain in x1000 02351 * if value is 1000 then no modification is applied. 02352 * @return VL53L0X_ERROR_NONE Success 02353 * @return "Other error code" See ::VL53L0X_Error 02354 */ 02355 VL53L0X_Error VL53L0X_set_linearity_corrective_gain(VL53L0X_DEV dev, 02356 int16_t linearity_corrective_gain); 02357 02358 /** 02359 * @brief Get the Maximum number of ROI Zones managed by the Device 02360 * 02361 * @par Function Description 02362 * Get Maximum number of ROI Zones managed by the Device. 02363 * 02364 * @note This function doesn't Access to the device 02365 * 02366 * @param dev Device Handle 02367 * @param p_max_number_of_roi_zones Pointer to the Maximum Number 02368 * of ROI Zones value. 02369 * @return VL53L0X_ERROR_NONE Success 02370 */ 02371 VL53L0X_Error VL53L0X_get_max_number_of_roi_zones(VL53L0X_DEV dev, 02372 uint8_t *p_max_number_of_roi_zones); 02373 02374 /** 02375 * @brief Retrieve the Reference Signal after a measurements 02376 * 02377 * @par Function Description 02378 * Get Reference Signal from last successful Ranging measurement 02379 * This function return a valid value after that you call the 02380 * @a VL53L0X_GetRangingMeasurementData(). 02381 * 02382 * @note This function Access to the device 02383 * 02384 * @param dev Device Handle 02385 * @param p_measurement_ref_signal Pointer to the Ref Signal to fill up. 02386 * @return VL53L0X_ERROR_NONE Success 02387 * @return "Other error code" See ::VL53L0X_Error 02388 */ 02389 VL53L0X_Error VL53L0X_get_measurement_ref_signal(VL53L0X_DEV dev, 02390 FixPoint1616_t *p_measurement_ref_signal); 02391 02392 /** 02393 * @brief Get the number of the check limit managed by a given Device 02394 * 02395 * @par Function Description 02396 * This function give the number of the check limit managed by the Device 02397 * 02398 * @note This function doesn't Access to the device 02399 * 02400 * @param p_number_of_limit_check Pointer to the number of check limit. 02401 * @return VL53L0X_ERROR_NONE Success 02402 * @return "Other error code" See ::VL53L0X_Error 02403 */ 02404 VL53L0X_Error VL53L0X_get_number_of_limit_check( 02405 uint16_t *p_number_of_limit_check); 02406 02407 /** 02408 * @brief Get the number of ROI Zones managed by the Device 02409 * 02410 * @par Function Description 02411 * Get number of ROI Zones managed by the Device 02412 * USER should take care about @a VL53L0X_GetNumberOfROIZones() 02413 * before get data after a perform measurement. 02414 * PAL will fill a NumberOfROIZones times the corresponding data 02415 * structure used in the measurement function. 02416 * 02417 * @note This function doesn't Access to the device 02418 * 02419 * @param dev Device Handle 02420 * @param p_number_of_roi_zones Pointer to the Number of ROI Zones value. 02421 * @return VL53L0X_ERROR_NONE Success 02422 */ 02423 VL53L0X_Error VL53L0X_get_number_of_roi_zones(VL53L0X_DEV dev, 02424 uint8_t *p_number_of_roi_zones); 02425 02426 /** 02427 * @brief Set the number of ROI Zones to be used for a specific Device 02428 * 02429 * @par Function Description 02430 * Set the number of ROI Zones to be used for a specific Device. 02431 * The programmed value should be less than the max number of ROI Zones given 02432 * with @a VL53L0X_GetMaxNumberOfROIZones(). 02433 * This version of API manage only one zone. 02434 * 02435 * @param dev Device Handle 02436 * @param number_of_roi_zones Number of ROI Zones to be used for a 02437 * specific Device. 02438 * @return VL53L0X_ERROR_NONE Success 02439 * @return VL53L0X_ERROR_INVALID_PARAMS This error is returned if 02440 * NumberOfROIZones != 1 02441 */ 02442 VL53L0X_Error VL53L0X_set_number_of_roi_zones(VL53L0X_DEV dev, 02443 uint8_t number_of_roi_zones); 02444 02445 /** 02446 * @brief Gets number of sequence steps managed by the API. 02447 * 02448 * @par Function Description 02449 * This function retrieves the number of sequence steps currently managed 02450 * by the API 02451 * 02452 * @note This function Accesses the device 02453 * 02454 * @param dev Device Handle 02455 * @param p_number_of_sequence_steps Out parameter reporting the number of 02456 * sequence steps. 02457 * @return VL53L0X_ERROR_NONE Success 02458 * @return "Other error code" See ::VL53L0X_Error 02459 */ 02460 VL53L0X_Error VL53L0X_get_number_of_sequence_steps(VL53L0X_DEV dev, 02461 uint8_t *p_number_of_sequence_steps); 02462 /** 02463 * @brief Get the power mode for a given Device 02464 * 02465 * @note This function Access to the device 02466 * 02467 * @param dev Device Handle 02468 * @param p_power_mode Pointer to the current value of the power 02469 * mode. see ::VL53L0X_PowerModes 02470 * Valid values are: 02471 * VL53L0X_POWERMODE_STANDBY_LEVEL1, 02472 * VL53L0X_POWERMODE_IDLE_LEVEL1 02473 * @return VL53L0X_ERROR_NONE Success 02474 * @return "Other error code" See ::VL53L0X_Error 02475 */ 02476 VL53L0X_Error VL53L0X_get_power_mode(VL53L0X_DEV dev, 02477 VL53L0X_PowerModes *p_power_mode); 02478 02479 /** 02480 * @brief Set the power mode for a given Device 02481 * The power mode can be Standby or Idle. Different level of both Standby and 02482 * Idle can exists. 02483 * This function should not be used when device is in Ranging state. 02484 * 02485 * @note This function Access to the device 02486 * 02487 * @param dev Device Handle 02488 * @param power_mode The value of the power mode to set. 02489 * see ::VL53L0X_PowerModes 02490 * Valid values are: 02491 * VL53L0X_POWERMODE_STANDBY_LEVEL1, 02492 * VL53L0X_POWERMODE_IDLE_LEVEL1 02493 * @return VL53L0X_ERROR_NONE Success 02494 * @return VL53L0X_ERROR_MODE_NOT_SUPPORTED This error occurs when PowerMode 02495 * is not in the supported list 02496 * @return "Other error code" See ::VL53L0X_Error 02497 */ 02498 VL53L0X_Error VL53L0X_set_power_mode(VL53L0X_DEV dev, 02499 VL53L0X_PowerModes power_mode); 02500 02501 /** 02502 * @brief Retrieves SPAD configuration 02503 * 02504 * @par Function Description 02505 * This function retrieves the current number of applied reference spads 02506 * and also their type : Aperture or Non-Aperture. 02507 * 02508 * @note This function Access to the device 02509 * 02510 * @param dev Device Handle 02511 * @param p_spad_count Number ref Spad Count 02512 * @param p_is_aperture_spads Reports if spads are of type 02513 * aperture or non-aperture. 02514 * 1:=aperture, 0:=Non-Aperture 02515 * @return VL53L0X_ERROR_NONE Success 02516 * @return VL53L0X_ERROR_REF_SPAD_INIT Error in the in the reference 02517 * spad configuration. 02518 * @return "Other error code" See ::VL53L0X_Error 02519 */ 02520 VL53L0X_Error wrapped_VL53L0X_get_reference_spads(VL53L0X_DEV dev, 02521 uint32_t *p_spad_count, uint8_t *p_is_aperture_spads); 02522 02523 /** 02524 * @brief Gets the (on/off) state of a requested sequence step. 02525 * 02526 * @par Function Description 02527 * This function retrieves the state of a requested sequence step, i.e. on/off. 02528 * 02529 * @note This function Accesses the device 02530 * 02531 * @param dev Device Handle 02532 * @param sequence_step_id Sequence step identifier. 02533 * @param p_sequence_step_enabled Out parameter reporting if the sequence step 02534 * is enabled {0=Off,1=On}. 02535 * @return VL53L0X_ERROR_NONE Success 02536 * @return VL53L0X_ERROR_INVALID_PARAMS Error SequenceStepId parameter not 02537 * supported. 02538 * @return "Other error code" See ::VL53L0X_Error 02539 */ 02540 VL53L0X_Error VL53L0X_get_sequence_step_enable(VL53L0X_DEV dev, 02541 VL53L0X_SequenceStepId sequence_step_id, uint8_t *p_sequence_step_enabled); 02542 02543 02544 /** 02545 * @brief Gets the timeout of a requested sequence step. 02546 * 02547 * @par Function Description 02548 * This function retrieves the timeout of a requested sequence step. 02549 * 02550 * @note This function Accesses the device 02551 * 02552 * @param dev Device Handle 02553 * @param sequence_step_id Sequence step identifier. 02554 * @param p_time_out_milli_secs Timeout value. 02555 * @return VL53L0X_ERROR_NONE Success 02556 * @return VL53L0X_ERROR_INVALID_PARAMS Error SequenceStepId parameter not 02557 * supported. 02558 * @return "Other error code" See ::VL53L0X_Error 02559 */ 02560 VL53L0X_Error VL53L0X_get_sequence_step_timeout(VL53L0X_DEV dev, 02561 VL53L0X_SequenceStepId sequence_step_id, 02562 FixPoint1616_t *p_time_out_milli_secs); 02563 02564 /** 02565 * @brief Sets the timeout of a requested sequence step. 02566 * 02567 * @par Function Description 02568 * This function sets the timeout of a requested sequence step. 02569 * 02570 * @note This function Accesses the device 02571 * 02572 * @param dev Device Handle 02573 * @param sequence_step_id Sequence step identifier. 02574 * @param time_out_milli_secs Demanded timeout 02575 * @return VL53L0X_ERROR_NONE Success 02576 * @return VL53L0X_ERROR_INVALID_PARAMS Error SequenceStepId parameter not 02577 * supported. 02578 * @return "Other error code" See ::VL53L0X_Error 02579 */ 02580 VL53L0X_Error VL53L0X_set_sequence_step_timeout(VL53L0X_DEV dev, 02581 VL53L0X_SequenceStepId sequence_step_id, FixPoint1616_t time_out_milli_secs); 02582 02583 /** 02584 * @brief Get the current SPAD Ambient Damper Factor value 02585 * 02586 * @par Function Description 02587 * This function get the SPAD Ambient Damper Factor value 02588 * 02589 * @note This function Access to the device 02590 * 02591 * @param dev Device Handle 02592 * @param p_spad_ambient_damper_factor Pointer to programmed SPAD Ambient 02593 * Damper Factor value 02594 * @return VL53L0X_ERROR_NONE Success 02595 * @return "Other error code" See ::VL53L0X_Error 02596 */ 02597 VL53L0X_Error VL53L0X_get_spad_ambient_damper_factor(VL53L0X_DEV dev, 02598 uint16_t *p_spad_ambient_damper_factor); 02599 /** 02600 * @brief Set the SPAD Ambient Damper Factor value 02601 * 02602 * @par Function Description 02603 * This function set the SPAD Ambient Damper Factor value 02604 * 02605 * @note This function Access to the device 02606 * 02607 * @param dev Device Handle 02608 * @param spad_ambient_damper_factor SPAD Ambient Damper Factor value 02609 * @return VL53L0X_ERROR_NONE Success 02610 * @return "Other error code" See ::VL53L0X_Error 02611 */ 02612 VL53L0X_Error VL53L0X_set_spad_ambient_damper_factor(VL53L0X_DEV dev, 02613 uint16_t spad_ambient_damper_factor); 02614 02615 /** 02616 * @brief Get the current SPAD Ambient Damper Threshold value 02617 * 02618 * @par Function Description 02619 * This function get the SPAD Ambient Damper Threshold value 02620 * 02621 * @note This function Access to the device 02622 * 02623 * @param dev Device Handle 02624 * @param p_spad_ambient_damper_threshold Pointer to programmed 02625 * SPAD Ambient Damper Threshold value 02626 * @return VL53L0X_ERROR_NONE Success 02627 * @return "Other error code" See ::VL53L0X_Error 02628 */ 02629 VL53L0X_Error VL53L0X_get_spad_ambient_damper_threshold(VL53L0X_DEV dev, 02630 uint16_t *p_spad_ambient_damper_threshold); 02631 02632 /** 02633 * @brief Set the SPAD Ambient Damper Threshold value 02634 * 02635 * @par Function Description 02636 * This function set the SPAD Ambient Damper Threshold value 02637 * 02638 * @note This function Access to the device 02639 * 02640 * @param dev Device Handle 02641 * @param spad_ambient_damper_threshold SPAD Ambient Damper Threshold value 02642 * @return VL53L0X_ERROR_NONE Success 02643 * @return "Other error code" See ::VL53L0X_Error 02644 */ 02645 VL53L0X_Error VL53L0X_set_spad_ambient_damper_threshold(VL53L0X_DEV dev, 02646 uint16_t spad_ambient_damper_threshold); 02647 02648 /** 02649 * @brief Get the maximal distance for actual setup 02650 * @par Function Description 02651 * Device must be initialized through @a VL53L0X_SetParameters() prior calling 02652 * this function. 02653 * 02654 * Any range value more than the value returned is to be considered as 02655 * "no target detected" or 02656 * "no target in detectable range"\n 02657 * @warning The maximal distance depends on the setup 02658 * 02659 * @note This function is not Implemented 02660 * 02661 * @param dev Device Handle 02662 * @param p_upper_limit_milli_meter The maximal range limit for actual setup 02663 * (in millimeter) 02664 * @return VL53L0X_ERROR_NOT_IMPLEMENTED Not implemented 02665 */ 02666 VL53L0X_Error VL53L0X_get_upper_limit_milli_meter(VL53L0X_DEV dev, 02667 uint16_t *p_upper_limit_milli_meter); 02668 02669 /** 02670 * @brief Get the tuning settings pointer and the internal external switch 02671 * value. 02672 * 02673 * This function is used to get the Tuning settings buffer pointer and the 02674 * value. 02675 * of the switch to select either external or internal tuning settings. 02676 * 02677 * @note This function Access to the device 02678 * 02679 * @param dev Device Handle 02680 * @param pp_tuning_setting_buffer Pointer to tuning settings buffer. 02681 * @param p_use_internal_tuning_settings Pointer to store Use internal tuning 02682 * settings value. 02683 * @return VL53L0X_ERROR_NONE Success 02684 * @return "Other error code" See ::VL53L0X_Error 02685 */ 02686 VL53L0X_Error VL53L0X_get_tuning_setting_buffer(VL53L0X_DEV dev, 02687 uint8_t **pp_tuning_setting_buffer, uint8_t *p_use_internal_tuning_settings); 02688 02689 /** 02690 * @brief Set the tuning settings pointer 02691 * 02692 * This function is used to specify the Tuning settings buffer to be used 02693 * for a given device. The buffer contains all the necessary data to permit 02694 * the API to write tuning settings. 02695 * This function permit to force the usage of either external or internal 02696 * tuning settings. 02697 * 02698 * @note This function Access to the device 02699 * 02700 * @param dev Device Handle 02701 * @param p_tuning_setting_buffer Pointer to tuning settings buffer. 02702 * @param use_internal_tuning_settings Use internal tuning settings value. 02703 * @return VL53L0X_ERROR_NONE Success 02704 * @return "Other error code" See ::VL53L0X_Error 02705 */ 02706 VL53L0X_Error VL53L0X_set_tuning_setting_buffer(VL53L0X_DEV dev, 02707 uint8_t *p_tuning_setting_buffer, uint8_t use_internal_tuning_settings); 02708 02709 /** 02710 * @defgroup VL53L0X_registerAccess_group PAL Register Access Functions 02711 * @brief PAL Register Access Functions 02712 * @{ 02713 */ 02714 02715 /** 02716 * Lock comms interface to serialize all commands to a shared I2C interface for a specific device 02717 * @param dev Device Handle 02718 * @return VL53L0X_ERROR_NONE Success 02719 * @return "Other error code" See ::VL53L0X_Error 02720 */ 02721 VL53L0X_Error VL53L0X_lock_sequence_access(VL53L0X_DEV dev); 02722 02723 /** 02724 * Unlock comms interface to serialize all commands to a shared I2C interface for a specific device 02725 * @param dev Device Handle 02726 * @return VL53L0X_ERROR_NONE Success 02727 * @return "Other error code" See ::VL53L0X_Error 02728 */ 02729 VL53L0X_Error VL53L0X_unlock_sequence_access(VL53L0X_DEV dev); 02730 02731 /** 02732 * @brief Prepare device for operation 02733 * @par Function Description 02734 * Update device with provided parameters 02735 * @li Then start ranging operation. 02736 * 02737 * @note This function Access to the device 02738 * 02739 * @param Dev Device Handle 02740 * @param pDeviceParameters Pointer to store current device parameters. 02741 * @return VL53L0X_ERROR_NONE Success 02742 * @return "Other error code" See ::VL53L0X_Error 02743 */ 02744 VL53L0X_Error vl53L0x_set_device_parameters(VL53L0X_DEV Dev, 02745 const VL53L0X_DeviceParameters_t *pDeviceParameters); 02746 02747 /** 02748 * Set Group parameter Hold state 02749 * 02750 * @par Function Description 02751 * Set or remove device internal group parameter hold 02752 * 02753 * @note This function is not Implemented 02754 * 02755 * @param dev Device Handle 02756 * @param group_param_hold Group parameter Hold state to be set (on/off) 02757 * @return VL53L0X_ERROR_NOT_IMPLEMENTED Not implemented 02758 */ 02759 VL53L0X_Error VL53L0X_set_group_param_hold(VL53L0X_DEV dev, 02760 uint8_t group_param_hold); 02761 02762 02763 /** 02764 * @brief Wait for device ready for a new measurement command. 02765 * Blocking function. 02766 * 02767 * @note This function is not Implemented 02768 * 02769 * @param dev Device Handle 02770 * @param max_loop Max Number of polling loop (timeout). 02771 * @return VL53L0X_ERROR_NOT_IMPLEMENTED Not implemented 02772 */ 02773 VL53L0X_Error VL53L0X_wait_device_ready_for_new_measurement(VL53L0X_DEV dev, 02774 uint32_t max_loop); 02775 02776 VL53L0X_Error VL53L0X_reverse_bytes(uint8_t *data, uint32_t size); 02777 02778 int range_meas_int_continuous_mode(void (*fptr)(void)); 02779 02780 02781 VL53L0X_DeviceInfo_t _device_info; 02782 02783 /* IO Device */ 02784 DevI2C *_dev_i2c; 02785 /* Digital out pin */ 02786 DigitalOut *_gpio0; 02787 /* GPIO expander */ 02788 Stmpe1600DigiOut *_expgpio0; 02789 /* Measure detection IRQ */ 02790 InterruptIn *_gpio1Int; 02791 /* Device data */ 02792 VL53L0X_Dev_t _my_device; 02793 VL53L0X_DEV _device; 02794 }; 02795 02796 02797 #endif /* _VL53L0X_CLASS_H_ */
Generated on Tue Jul 12 2022 19:07:41 by
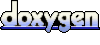