Low voltage digital temperature sensor
Dependencies: X_NUCLEO_COMMON ST_INTERFACES
Dependents: X_NUCLEO_IKS01A3 X_NUCLEO_IKS01A3 X_NUCLEO_IKS01A3
stts751_reg.h
00001 /* 00002 ****************************************************************************** 00003 * @file stts751_reg.h 00004 * @author Sensors Software Solution Team 00005 * @brief This file contains all the functions prototypes for the 00006 * stts751_reg.c driver. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2018 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without 00013 * modification, are permitted provided that the following conditions 00014 * are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright 00018 * notice, this list of conditions and the following disclaimer in the 00019 * documentation and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its 00021 * contributors may be used to endorse or promote products derived from 00022 * this software without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE 00027 * ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE 00028 * LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR 00029 * CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF 00030 * SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00031 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00032 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 00033 * ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00034 * POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef STTS751_REGS_H 00040 #define STTS751_REGS_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include <stdint.h> 00048 #include <math.h> 00049 00050 /** @addtogroup STTS751 00051 * @{ 00052 * 00053 */ 00054 00055 /** @defgroup STTS751_sensors_common_types 00056 * @{ 00057 * 00058 */ 00059 00060 #ifndef MEMS_SHARED_TYPES 00061 #define MEMS_SHARED_TYPES 00062 00063 /** 00064 * @defgroup axisXbitXX_t 00065 * @brief These unions are useful to represent different sensors data type. 00066 * These unions are not need by the driver. 00067 * 00068 * REMOVING the unions you are compliant with: 00069 * MISRA-C 2012 [Rule 19.2] -> " Union are not allowed " 00070 * 00071 * @{ 00072 * 00073 */ 00074 00075 typedef union { 00076 int16_t i16bit[3]; 00077 uint8_t u8bit[6]; 00078 } axis3bit16_t; 00079 00080 typedef union { 00081 int16_t i16bit; 00082 uint8_t u8bit[2]; 00083 } axis1bit16_t; 00084 00085 typedef union { 00086 int32_t i32bit[3]; 00087 uint8_t u8bit[12]; 00088 } axis3bit32_t; 00089 00090 typedef union { 00091 int32_t i32bit; 00092 uint8_t u8bit[4]; 00093 } axis1bit32_t; 00094 00095 /** 00096 * @} 00097 * 00098 */ 00099 00100 typedef struct { 00101 uint8_t bit0 : 1; 00102 uint8_t bit1 : 1; 00103 uint8_t bit2 : 1; 00104 uint8_t bit3 : 1; 00105 uint8_t bit4 : 1; 00106 uint8_t bit5 : 1; 00107 uint8_t bit6 : 1; 00108 uint8_t bit7 : 1; 00109 } bitwise_t; 00110 00111 #define PROPERTY_DISABLE (0U) 00112 #define PROPERTY_ENABLE (1U) 00113 00114 #endif /* MEMS_SHARED_TYPES */ 00115 00116 /** 00117 * @} 00118 * 00119 */ 00120 00121 /** @addtogroup STTS751_Interfaces_Functions 00122 * @brief This section provide a set of functions used to read and 00123 * write a generic register of the device. 00124 * MANDATORY: return 0 -> no Error. 00125 * @{ 00126 * 00127 */ 00128 00129 typedef int32_t (*stts751_write_ptr)(void *, uint8_t, uint8_t *, uint16_t); 00130 typedef int32_t (*stts751_read_ptr)(void *, uint8_t, uint8_t *, uint16_t); 00131 00132 typedef struct { 00133 /** Component mandatory fields **/ 00134 stts751_write_ptr write_reg; 00135 stts751_read_ptr read_reg; 00136 /** Customizable optional pointer **/ 00137 void *handle; 00138 } stts751_ctx_t; 00139 00140 /** 00141 * @} 00142 * 00143 */ 00144 00145 /** @defgroup STTS751_Infos 00146 * @{ 00147 * 00148 */ 00149 00150 /** I2C Device Address 8 bit format **/ 00151 #define STTS751_0xxxx_ADD_7K5 0x91U 00152 #define STTS751_0xxxx_ADD_12K 0x93U 00153 #define STTS751_0xxxx_ADD_20K 0x71U 00154 #define STTS751_0xxxx_ADD_33K 0x73U 00155 00156 #define STTS751_1xxxx_ADD_7K5 0x95U 00157 #define STTS751_1xxxx_ADD_12K 0x97U 00158 #define STTS751_1xxxx_ADD_20K 0x75U 00159 #define STTS751_1xxxx_ADD_33K 0x77U 00160 00161 /** Device Identification **/ 00162 /* Product ID */ 00163 #define STTS751_ID_0xxxx 0x00U 00164 #define STTS751_ID_1xxxx 0x01U 00165 /* Manufacturer ID */ 00166 #define STTS751_ID_MAN 0x53U 00167 /* Revision number */ 00168 #define STTS751_REV 0x01U 00169 00170 /** 00171 * @} 00172 * 00173 */ 00174 00175 #define STTS751_TEMPERATURE_HIGH 0x00U 00176 #define STTS751_STATUS 0x01U 00177 typedef struct { 00178 uint8_t thrm : 1; 00179 uint8_t not_used_01 : 4; 00180 uint8_t t_low : 1; 00181 uint8_t t_high : 1; 00182 uint8_t busy : 1; 00183 } stts751_status_t; 00184 00185 #define STTS751_TEMPERATURE_LOW 0x02U 00186 #define STTS751_CONFIGURATION 0x03U 00187 typedef struct { 00188 uint8_t not_used_01 : 2; 00189 uint8_t tres : 2; 00190 uint8_t not_used_02 : 2; 00191 uint8_t stop : 1; 00192 uint8_t mask1 : 1; 00193 } stts751_configuration_t; 00194 00195 #define STTS751_CONVERSION_RATE 0x04U 00196 typedef struct { 00197 uint8_t conv : 4; 00198 uint8_t not_used_01 : 4; 00199 } stts751_conversion_rate_t; 00200 00201 #define STTS751_TEMPERATURE_HIGH_LIMIT_HIGH 0x05U 00202 #define STTS751_TEMPERATURE_HIGH_LIMIT_LOW 0x06U 00203 #define STTS751_TEMPERATURE_LOW_LIMIT_HIGH 0x07U 00204 #define STTS751_TEMPERATURE_LOW_LIMIT_LOW 0x08U 00205 #define STTS751_ONE_SHOT 0x0FU 00206 #define STTS751_THERM_LIMIT 0x20U 00207 #define STTS751_THERM_HYSTERESIS 0x21U 00208 #define STTS751_SMBUS_TIMEOUT 0x22U 00209 typedef struct { 00210 uint8_t not_used_01 : 7; 00211 uint8_t timeout : 1; 00212 } stts751_smbus_timeout_t; 00213 00214 #define STTS751_PRODUCT_ID 0xFDU 00215 #define STTS751_MANUFACTURER_ID 0xFEU 00216 #define STTS751_REVISION_ID 0xFFU 00217 00218 /** 00219 * @defgroup STTS751_Register_Union 00220 * @brief This union group all the registers that has a bitfield 00221 * description. 00222 * This union is useful but not need by the driver. 00223 * 00224 * REMOVING this union you are compliant with: 00225 * MISRA-C 2012 [Rule 19.2] -> " Union are not allowed " 00226 * 00227 * @{ 00228 * 00229 */ 00230 typedef union { 00231 stts751_status_t status; 00232 stts751_configuration_t configuration; 00233 stts751_conversion_rate_t conversion_rate; 00234 stts751_smbus_timeout_t smbus_timeout; 00235 bitwise_t bitwise; 00236 uint8_t byte; 00237 } stts751_reg_t; 00238 00239 /** 00240 * @} 00241 * 00242 */ 00243 00244 int32_t stts751_read_reg(stts751_ctx_t *ctx, uint8_t reg, uint8_t *data, 00245 uint16_t len); 00246 int32_t stts751_write_reg(stts751_ctx_t *ctx, uint8_t reg, uint8_t *data, 00247 uint16_t len); 00248 00249 extern float stts751_from_lsb_to_celsius(int16_t lsb); 00250 extern int16_t stts751_from_celsius_to_lsb(float celsius); 00251 00252 typedef enum { 00253 STTS751_TEMP_ODR_OFF = 0x80, 00254 STTS751_TEMP_ODR_ONE_SHOT = 0x90, 00255 STTS751_TEMP_ODR_62mHz5 = 0x00, 00256 STTS751_TEMP_ODR_125mHz = 0x01, 00257 STTS751_TEMP_ODR_250mHz = 0x02, 00258 STTS751_TEMP_ODR_500mHz = 0x03, 00259 STTS751_TEMP_ODR_1Hz = 0x04, 00260 STTS751_TEMP_ODR_2Hz = 0x05, 00261 STTS751_TEMP_ODR_4Hz = 0x06, 00262 STTS751_TEMP_ODR_8Hz = 0x07, 00263 STTS751_TEMP_ODR_16Hz = 0x08, /* 9, 10, or 11-bit resolutions only */ 00264 STTS751_TEMP_ODR_32Hz = 0x09, /* 9 or 10-bit resolutions only */ 00265 } stts751_odr_t; 00266 int32_t stts751_temp_data_rate_set(stts751_ctx_t *ctx, stts751_odr_t val); 00267 int32_t stts751_temp_data_rate_get(stts751_ctx_t *ctx, stts751_odr_t *val); 00268 00269 typedef enum { 00270 STTS751_9bit = 2, 00271 STTS751_10bit = 0, 00272 STTS751_11bit = 1, 00273 STTS751_12bit = 3, 00274 } stts751_tres_t; 00275 int32_t stts751_resolution_set(stts751_ctx_t *ctx, stts751_tres_t val); 00276 int32_t stts751_resolution_get(stts751_ctx_t *ctx, stts751_tres_t *val); 00277 00278 int32_t stts751_status_reg_get(stts751_ctx_t *ctx, stts751_status_t *val); 00279 00280 int32_t stts751_flag_busy_get(stts751_ctx_t *ctx, uint8_t *val); 00281 00282 int32_t stts751_temperature_raw_get(stts751_ctx_t *ctx, int16_t *buff); 00283 00284 int32_t stts751_pin_event_route_set(stts751_ctx_t *ctx, uint8_t val); 00285 int32_t stts751_pin_event_route_get(stts751_ctx_t *ctx, uint8_t *val); 00286 00287 00288 int32_t stts751_high_temperature_threshold_set(stts751_ctx_t *ctx, 00289 int16_t buff); 00290 int32_t stts751_high_temperature_threshold_get(stts751_ctx_t *ctx, 00291 int16_t *buff); 00292 00293 int32_t stts751_low_temperature_threshold_set(stts751_ctx_t *ctx, 00294 int16_t buff); 00295 int32_t stts751_low_temperature_threshold_get(stts751_ctx_t *ctx, 00296 int16_t *buff); 00297 00298 int32_t stts751_ota_thermal_limit_set(stts751_ctx_t *ctx, int8_t val); 00299 int32_t stts751_ota_thermal_limit_get(stts751_ctx_t *ctx, int8_t *val); 00300 00301 int32_t stts751_ota_thermal_hyst_set(stts751_ctx_t *ctx, int8_t val); 00302 int32_t stts751_ota_thermal_hyst_get(stts751_ctx_t *ctx, int8_t *val); 00303 00304 int32_t stts751_smbus_timeout_set(stts751_ctx_t *ctx, uint8_t val); 00305 int32_t stts751_smbus_timeout_get(stts751_ctx_t *ctx, uint8_t *val); 00306 00307 typedef struct { 00308 uint8_t product_id; 00309 uint8_t manufacturer_id; 00310 uint8_t revision_id; 00311 } stts751_id_t; 00312 int32_t stts751_device_id_get(stts751_ctx_t *ctx, stts751_id_t *buff); 00313 00314 /** 00315 * @} 00316 * 00317 */ 00318 00319 #ifdef __cplusplus 00320 } 00321 #endif 00322 00323 #endif /*STTS751_REGS_H */ 00324 00325 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Jul 15 2022 18:33:31 by
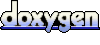