
This application provides a set of demos with X-NUCLEO-NFC01A1 expansion board.
Dependencies: NDefLib X_NUCLEO_NFC01A1 mbed
Fork of X-MBED-NFC1 by
SampleSync_writeAndChangeAll.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file SampleSynch_writeAndChangeAll.cpp 00004 * @author ST / Central Labs 00005 * @date 03 Dic 2015 00006 * @brief This demo write an ndef message different records, when the user press the buttun 00007 * read the tag, change some data and write it again 00008 ****************************************************************************** 00009 * 00010 * COPYRIGHT(c) 2015 STMicroelectronics 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 #include "mbed.h" 00038 00039 #include "NDefLib/NDefNfcTag.h" 00040 00041 #include "NDefLib/RecordType/RecordAAR.h" 00042 #include "NDefLib/RecordType/RecordSMS.h" 00043 #include "NDefLib/RecordType/RecordGeo.h" 00044 #include "NDefLib/RecordType/RecordURI.h" 00045 #include "NDefLib/RecordType/RecordMail.h" 00046 #include "NDefLib/RecordType/RecordText.h" 00047 #include "NDefLib/RecordType/RecordMimeType.h" 00048 #include "NDefLib/RecordType/RecordVCard.h" 00049 #include "NDefLib/RecordType/RecordWifiConf.h" 00050 00051 #include "XNucleoNFC01A1.h" 00052 00053 /** 00054 * Shift the led status between the 3 leds. 00055 */ 00056 static void shift_led(DigitalOut &led1,DigitalOut &led2,DigitalOut &led3){ 00057 const uint8_t prevLed1=led1; 00058 const uint8_t prevLed2=led2; 00059 const uint8_t prevLed3=led3; 00060 led1=prevLed3; 00061 led2=prevLed1; 00062 led3=prevLed2; 00063 } 00064 00065 /** 00066 * Create a message that contains all the possible records, and write it in the tag. 00067 * @param tag Nfc tag where write the message. 00068 */ 00069 static void write_nfc_tag(NDefLib::NDefNfcTag &tag){ 00070 00071 bool writeStatus=false; 00072 bool closeStatus=false; 00073 if(tag.open_session()){ 00074 printf("Open session\r\n"); 00075 NDefLib::Message msg; 00076 00077 NDefLib::RecordAAR rAAR("com.st.BlueMS"); 00078 msg.add_record(&rAAR); 00079 00080 NDefLib::RecordSMS rSMS("123456789","st.com.BlueMS"); 00081 msg.add_record(&rSMS); 00082 00083 NDefLib::RecordGeo rGeo(123.123,-456.789); 00084 msg.add_record(&rGeo); 00085 00086 NDefLib::RecordURI rUri(NDefLib::RecordURI::HTTP_WWW,"http://www.st.com"); 00087 msg.add_record(&rUri); 00088 00089 NDefLib::RecordMail rMail("mail@st.com","ciao","da nfc tag"); 00090 msg.add_record(&rMail); 00091 00092 NDefLib::RecordMimeType rText1("text/plain",(const uint8_t*)"ciao",4); 00093 msg.add_record(&rText1); 00094 00095 NDefLib::RecordText rText3(NDefLib::RecordText::UTF8,"it","ciao"); 00096 msg.add_record(&rText3); 00097 00098 NDefLib::RecordWifiConf rWifi("OpenNetworkd"); 00099 msg.add_record(&rWifi); 00100 00101 NDefLib::RecordVCard::VCardInfo_t cardInfo; 00102 cardInfo[NDefLib::RecordVCard::FORMATTED_NAME]="prova prova1"; 00103 cardInfo[NDefLib::RecordVCard::ADDRESS_HOME]=";;1 Main St.;Springfield;IL;12345;USA"; 00104 cardInfo[NDefLib::RecordVCard::ADDRESS_WORK]=";;2 Main St.;Springfield;IL;12345;USA"; 00105 cardInfo[NDefLib::RecordVCard::EMAIL_WORK]="workmail@st.com"; 00106 cardInfo[NDefLib::RecordVCard::EMAIL_HOME]="homemail@st.com"; 00107 cardInfo[NDefLib::RecordVCard::GEO]="39.95;-75.1667"; 00108 cardInfo[NDefLib::RecordVCard::IMPP]="aim:johndoe@aol.com"; 00109 cardInfo[NDefLib::RecordVCard::NAME]="prova2;prova3"; 00110 cardInfo[NDefLib::RecordVCard::NICKNAME]="test"; 00111 cardInfo[NDefLib::RecordVCard::NOTE]="A good test"; 00112 cardInfo[NDefLib::RecordVCard::ORGANIZATION]="STM"; 00113 cardInfo[NDefLib::RecordVCard::TEL_HOME]="123"; 00114 cardInfo[NDefLib::RecordVCard::TEL_MOBILE]="456"; 00115 cardInfo[NDefLib::RecordVCard::TEL_WORK]="789"; 00116 cardInfo[NDefLib::RecordVCard::TITLE]="King"; 00117 cardInfo[NDefLib::RecordVCard::URL]="www.st.com"; 00118 cardInfo[NDefLib::RecordVCard::PHOTO_URI]="http://www.st.com/st-web-ui/static/active/en/fragment/multimedia/image/picture/customer_focus.jpg"; 00119 NDefLib::RecordVCard rVCard(cardInfo); 00120 msg.add_record(&rVCard); 00121 00122 writeStatus = tag.write(msg); 00123 00124 closeStatus = tag.close_session(); 00125 00126 } else { 00127 printf("Error open Session\n\r"); 00128 } 00129 00130 if (writeStatus) { 00131 printf("Write Done\n\r"); 00132 } else { 00133 printf("Write Fail\n\r"); 00134 } 00135 00136 if (closeStatus) { 00137 printf("Close Done\n\r"); 00138 } else { 00139 printf("Close Fail\n\r"); 00140 } 00141 } 00142 00143 /** 00144 * Print the record content. 00145 * @param r Record to print. 00146 */ 00147 static void print_record(NDefLib::Record *const r){ 00148 using namespace NDefLib; 00149 switch(r->get_type()){ 00150 case Record::TYPE_TEXT: { 00151 const RecordText *const temp = ( RecordText* )r; 00152 printf("Read Text: %s\r\n",temp->get_text().c_str()); 00153 break; } 00154 case Record::TYPE_AAR:{ 00155 const RecordAAR *const temp = ( RecordAAR* )r; 00156 printf("Read ARR: %s\r\n",temp->get_package().c_str()); 00157 break; } 00158 case Record::TYPE_MIME:{ 00159 const RecordMimeType *const temp = ( RecordMimeType* )r; 00160 printf("Read mimeType: %s\r\n",temp->get_mime_type().c_str()); 00161 printf("Read mimeData: %s\r\n", 00162 std::string((const char*)temp->get_mime_data(), 00163 temp->get_mime_data_lenght()).c_str()); 00164 break;} 00165 case Record::TYPE_URI:{ 00166 RecordURI *const temp = (RecordURI*)r; 00167 printf("Read uriId: %d\r\n",temp->get_uri_id()); 00168 printf("Read uriType: %s\r\n",temp->get_uri_type().c_str()); 00169 printf("Read uriContent: %s\r\n",temp->get_content().c_str()); 00170 break;} 00171 case Record::TYPE_URI_MAIL:{ 00172 const RecordMail*const temp = (RecordMail*)r; 00173 printf("Read Dest: %s\r\n",temp->get_to_address().c_str()); 00174 printf("Read Subject: %s\r\n",temp->get_subject().c_str()); 00175 printf("Read Body: %s\r\n",temp->get_body().c_str()); 00176 break;} 00177 case Record::TYPE_URI_SMS:{ 00178 const RecordSMS*const temp = (RecordSMS*)r; 00179 printf("Read number: %s\r\n",temp->get_number().c_str()); 00180 printf("Read message: %s\r\n",temp->get_messagge().c_str()); 00181 break;} 00182 case Record::TYPE_URI_GEOLOCATION:{ 00183 const RecordGeo*const temp = (RecordGeo*)r; 00184 printf("Read lat: %f\r\n",temp->get_latitude()); 00185 printf("Read long: %f\r\n",temp->get_longitude()); 00186 break;} 00187 case Record::TYPE_MIME_VCARD:{ 00188 const RecordVCard *const temp = (RecordVCard*)r; 00189 printf("Read Name: %s\r\n",(*temp)[RecordVCard::NAME].c_str()); 00190 printf("Read Mail: %s\r\n",(*temp)[RecordVCard::EMAIL_WORK].c_str()); 00191 printf("Read ORG: %s\r\n",(*temp)[RecordVCard::ORGANIZATION].c_str()); 00192 break;} 00193 case Record::TYPE_WIFI_CONF:{ 00194 const RecordWifiConf *const temp = (RecordWifiConf*)r; 00195 printf("Nework Name: %s\r\n",temp->get_network_ssid().c_str()); 00196 printf("Nework Key: %s\r\n",temp->get_network_key().c_str()); 00197 printf("Nework Auth: %X\r\n",temp->get_auth_type()); 00198 printf("Nework Enc: %X\r\n",temp->get_encryption()); 00199 break;} 00200 case Record::TYPE_UNKNOWN:{ 00201 printf("Unknown record\r\n"); 00202 break;} 00203 }//switch 00204 } 00205 00206 /** 00207 * Change the record content. 00208 * @param r Record to change. 00209 */ 00210 static void change_record(NDefLib::Record const* r){ 00211 using namespace NDefLib; 00212 switch(r->get_type()){ 00213 case Record::TYPE_TEXT: { 00214 RecordText *temp = (RecordText*)r; 00215 temp->set_text("Hello"); 00216 break; } 00217 case Record::TYPE_AAR:{ 00218 RecordAAR *temp = (RecordAAR*)r; 00219 temp->set_package("set Package Ok"); 00220 break; } 00221 case Record::TYPE_MIME:{ 00222 RecordMimeType *temp = (RecordMimeType*)r; 00223 temp->copy_mime_data((const uint8_t *)"String2",sizeof("String2")); 00224 break;} 00225 case Record::TYPE_URI:{ 00226 RecordURI *temp = (RecordURI*)r; 00227 temp->set_content("mbed.com"); 00228 break;} 00229 case Record::TYPE_URI_MAIL:{ 00230 RecordMail *temp = (RecordMail*)r; 00231 temp->set_to_address("newMail@st.com"); 00232 temp->set_subject("tag change"); 00233 temp->set_body("read/change Works!"); 00234 break;} 00235 case Record::TYPE_URI_SMS:{ 00236 RecordSMS *temp = (RecordSMS*)r; 00237 temp->set_message("Message Change"); 00238 temp->set_number("0987654321"); 00239 break;} 00240 case Record::TYPE_URI_GEOLOCATION:{ 00241 RecordGeo *temp = (RecordGeo*)r; 00242 temp->set_latitude(-temp->get_latitude()); 00243 temp->set_longitude(-temp->get_longitude()); 00244 break;} 00245 case Record::TYPE_MIME_VCARD:{ 00246 RecordVCard *temp = (RecordVCard*)r; 00247 (*temp)[RecordVCard::NAME]="name change"; 00248 (*temp)[RecordVCard::NICKNAME]="nic change"; 00249 break;} 00250 case Record::TYPE_WIFI_CONF:{ 00251 RecordWifiConf * temp = (RecordWifiConf*)r; 00252 temp->set_network_ssid("hackMe"); 00253 temp->set_network_key("qwerty"); 00254 temp->set_auth_type(RecordWifiConf::AUTH_WPA2_PSK); 00255 temp->set_encryption_type(RecordWifiConf::ENC_TYPE_AES_TKIP); 00256 break;} 00257 case Record::TYPE_UNKNOWN:{ 00258 printf("Unknown record\r\n"); 00259 break;} 00260 }//switch 00261 } 00262 00263 /** 00264 * Read the nfc message and print the content on the serial console 00265 * @param tag Nfc tag where read the content 00266 */ 00267 static void read_and_print_nfc_tag(NDefLib::NDefNfcTag &tag){ 00268 using namespace NDefLib; 00269 00270 if(tag.open_session()){ 00271 printf("Open Session\r\n"); 00272 NDefLib::Message readMsg; 00273 00274 tag.read(&readMsg); 00275 printf("Message Read\r\n"); 00276 00277 if(readMsg.get_N_records()==0){ 00278 printf("Error Read\r\n"); 00279 }else{ 00280 for(uint32_t i=0;i<readMsg.get_N_records();i++){ 00281 Record *r = readMsg[i]; 00282 print_record(r); 00283 delete r; 00284 }//for 00285 }//if-else 00286 00287 tag.close_session(); 00288 printf("Close session\r\n"); 00289 }else{ 00290 printf("Error open read Session\n\r"); 00291 } 00292 } 00293 00294 /** 00295 * Read a nfc message, change the content of each record and write the new message. 00296 * @param tag Tag where read and write the nfc message. 00297 */ 00298 static void read_and_change_nfc_tag(NDefLib::NDefNfcTag &tag){ 00299 using NDefLib::Record; 00300 using NDefLib::Message; 00301 00302 if(tag.open_session()){ 00303 printf("Open Session\r\n"); 00304 Message readMsg; 00305 00306 tag.read(&readMsg); 00307 printf("Read Message\r\n"); 00308 00309 if(readMsg.get_N_records()==0){ 00310 printf("Error Read\r\n"); 00311 }else{ 00312 for(uint32_t i=0;i<readMsg.get_N_records();i++){ 00313 Record *r = readMsg[i]; 00314 change_record(r); 00315 }//for 00316 tag.write(readMsg); 00317 printf("Message Wrote\r\n"); 00318 }//if-else 00319 00320 tag.close_session(); 00321 printf("Close Session\r\n"); 00322 }else{ 00323 printf("Error open SessionChange\n\r"); 00324 } 00325 } 00326 00327 static volatile bool buttonPress=false; /// true when the user press the message 00328 00329 /** 00330 * Call back called when the user press the button 00331 */ 00332 static void set_button_press(){ 00333 buttonPress=true; 00334 }//if buttonPress 00335 00336 /** 00337 * Write a message and when the user press the button it read the message, change it and update it. 00338 */ 00339 void sampleSync_writeAndChangeAll() { 00340 00341 //instance the board with the default paramiters 00342 I2C i2cChannel(XNucleoNFC01A1::DEFAULT_SDA_PIN,XNucleoNFC01A1::DEFAULT_SDL_PIN); 00343 XNucleoNFC01A1 *nfcNucleo = XNucleoNFC01A1::instance(i2cChannel); 00344 //retrieve the NdefLib interface 00345 NDefLib::NDefNfcTag& tag = nfcNucleo->get_M24SR().get_NDef_tag(); 00346 00347 //switch on the first led 00348 nfcNucleo->get_led1()=1; 00349 nfcNucleo->get_led2()=0; 00350 nfcNucleo->get_led3()=0; 00351 00352 printf("Init Done\r\n"); 00353 00354 //write the message 00355 write_nfc_tag(tag); 00356 //read the message and write it on console 00357 read_and_print_nfc_tag(tag); 00358 00359 //enable the button 00360 #if defined(TARGET_STM) 00361 InterruptIn userButton(USER_BUTTON); 00362 #else 00363 InterruptIn userButton(SW2); 00364 #endif 00365 userButton.fall(set_button_press); 00366 00367 //each second change the led status and see if the user press the button 00368 while(true) { 00369 if(buttonPress){ 00370 //update the message content 00371 read_and_change_nfc_tag(tag); 00372 //write the new message on console 00373 read_and_print_nfc_tag(tag); 00374 shift_led(nfcNucleo->get_led1(),nfcNucleo->get_led2(),nfcNucleo->get_led3()); 00375 buttonPress=false; 00376 }//if 00377 }//while 00378 }
Generated on Wed Jul 13 2022 03:11:25 by
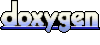