
Motion and Environmental sensor reader application connected via BLE to ST BlueMS iOS/Android application.
Dependencies: HTS221 LIS3MDL LPS22HB LSM303AGR LSM6DSL
Fork of MOTENV_Mbed by
CustomSensorsService.h
00001 /** 00002 ****************************************************************************** 00003 * @file CustomSensorService.h 00004 * @author Central Labs / AST 00005 * @version V0.9.0 00006 * @date 23-Dec-2015 00007 * @brief BLE MEMS and environmental sensors service 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 #ifndef __CUSTOM_BLE_SENSORS_SERVICE_H__ 00039 #define __CUSTOM_BLE_SENSORS_SERVICE_H__ 00040 00041 #include "BLE.h" 00042 #include "ble_utils.h" 00043 #include "ble_debug.h" 00044 #include "UUID.h" 00045 00046 //#define DISAGGREGATED_ENV_CHAR // uncomment to enable the disaggregated environmental temp,hum,pres characteristics, attention because of BLE mem limitations // the maximum number of characteristics is limited check BLUENRG_STACK_MODE 00047 //#define DISAGGREGATED_MOT_CHAR // " motion acc, gyro, mag " 00048 00049 const UUID::LongUUIDBytes_t SENS_SERVICE_UUID_128 = { 0x00,0x00,0x00,0x00,0x00,0x01,0x11,0xe1,0x9a,0xb4,0x00,0x02,0xa5,0xd5,0xc5,0x1b }; // temp, pressure, humidity, 00050 #ifdef DISAGGREGATED_ENV_CHAR 00051 const UUID::LongUUIDBytes_t SENS_TEMP_CHAR_UUID_128 = { 0x00,0x04,0x00,0x00,0x00,0x01,0x11,0xe1,0xac,0x36,0x00,0x02,0xa5,0xd5,0xc5,0x1b }; 00052 const UUID::LongUUIDBytes_t SENS_HUMI_CHAR_UUID_128 = { 0x00,0x08,0x00,0x00,0x00,0x01,0x11,0xe1,0xac,0x36,0x00,0x02,0xa5,0xd5,0xc5,0x1b }; 00053 const UUID::LongUUIDBytes_t SENS_PRES_CHAR_UUID_128 = { 0x00,0x10,0x00,0x00,0x00,0x01,0x11,0xe1,0xac,0x36,0x00,0x02,0xa5,0xd5,0xc5,0x1b }; 00054 #endif 00055 #ifdef DISAGGREGATED_MOT_CHAR 00056 const UUID::LongUUIDBytes_t SENS_MAGN_CHAR_UUID_128 = { 0x00,0x20,0x00,0x00,0x00,0x01,0x11,0xe1,0xac,0x36,0x00,0x02,0xa5,0xd5,0xc5,0x1b }; 00057 const UUID::LongUUIDBytes_t SENS_GYRO_CHAR_UUID_128 = { 0x00,0x40,0x00,0x00,0x00,0x01,0x11,0xe1,0xac,0x36,0x00,0x02,0xa5,0xd5,0xc5,0x1b }; 00058 const UUID::LongUUIDBytes_t SENS_ACCE_CHAR_UUID_128 = { 0x00,0x80,0x00,0x00,0x00,0x01,0x11,0xe1,0xac,0x36,0x00,0x02,0xa5,0xd5,0xc5,0x1b }; 00059 #endif 00060 const UUID::LongUUIDBytes_t SENS_ACC_GYRO_MAG_CHAR_UUID_128 = { 0x00,0xE0,0x00,0x00,0x00,0x01,0x11,0xe1,0xac,0x36,0x00,0x02,0xa5,0xd5,0xc5,0x1b }; 00061 const UUID::LongUUIDBytes_t SENS_PRES_HUM_TEMP_CHAR_UUID_128= { 0x00,0x1C,0x00,0x00,0x00,0x01,0x11,0xe1,0xac,0x36,0x00,0x02,0xa5,0xd5,0xc5,0x1b }; 00062 00063 00064 #define TEMP_DATA_LEN 2+2 00065 #define HUM_DATA_LEN 2+2 00066 #define PRES_DATA_LEN 2+4 00067 #define ACC_DATA_LEN 6+2 00068 #define MAG_DATA_LEN 6+2 00069 #define GYRO_DATA_LEN 6+2 00070 #define ACCGYROMAG_DATA_LEN 2+3*3*2 00071 #define PRESHUMTEMP_DATA_LEN 2+4+2+2 00072 00073 /* Custom Sensors Service */ 00074 class CustomSensorService 00075 { 00076 public: 00077 CustomSensorService(BLE &_ble) : 00078 ble(_ble), 00079 #ifdef DISAGGREGATED_ENV_CHAR 00080 envTemperatureCharacteristic(SENS_TEMP_CHAR_UUID_128,envTemperature, TEMP_DATA_LEN, TEMP_DATA_LEN, 00081 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY), 00082 envHumidityCharacteristic(SENS_HUMI_CHAR_UUID_128, envHumidity, HUM_DATA_LEN, HUM_DATA_LEN, 00083 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY), 00084 envPressureCharacteristic(SENS_PRES_CHAR_UUID_128, envPressure, PRES_DATA_LEN, PRES_DATA_LEN, 00085 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY), 00086 #endif 00087 #ifdef DISAGGREGATED_MOT_CHAR 00088 envMagnetometerCharacteristic(SENS_MAGN_CHAR_UUID_128,envMagn, MAG_DATA_LEN, MAG_DATA_LEN, 00089 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY), 00090 envAccelerometerCharacteristic(SENS_ACCE_CHAR_UUID_128,envAcce, ACC_DATA_LEN, ACC_DATA_LEN, 00091 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY), 00092 envGyroCharacteristic(SENS_GYRO_CHAR_UUID_128,envGyro, GYRO_DATA_LEN, GYRO_DATA_LEN, 00093 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY), 00094 #endif 00095 envAccGyroMagCharacteristic(SENS_ACC_GYRO_MAG_CHAR_UUID_128,envAccGyroMag, ACCGYROMAG_DATA_LEN, ACCGYROMAG_DATA_LEN, 00096 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY), 00097 envPresHumTempCharacteristic(SENS_PRES_HUM_TEMP_CHAR_UUID_128,envPresHumTemp, PRESHUMTEMP_DATA_LEN, PRESHUMTEMP_DATA_LEN, 00098 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY) 00099 { 00100 00101 static bool serviceAdded = false; /* We should only ever need to add the env service once. */ 00102 if (serviceAdded) { 00103 return; 00104 } 00105 00106 GattCharacteristic *charTable[] = { 00107 #ifdef DISAGGREGATED_ENV_CHAR 00108 &envTemperatureCharacteristic, &envHumidityCharacteristic, &envPressureCharacteristic, 00109 #endif 00110 &envPresHumTempCharacteristic, 00111 #ifdef DISAGGREGATED_MOT_CHAR 00112 &envMagnetometerCharacteristic, &envAccelerometerCharacteristic, &envGyroCharacteristic, 00113 #endif 00114 &envAccGyroMagCharacteristic 00115 }; 00116 00117 GattService envService(SENS_SERVICE_UUID_128, charTable, sizeof(charTable) / sizeof(GattCharacteristic *)); 00118 00119 ble.gattServer().addService(envService); 00120 00121 #ifdef DISAGGREGATED_ENV_CHAR 00122 isEnabledTempNotify = false; 00123 isEnabledHumNotify = false; 00124 isEnabledPresNotify = false; 00125 #endif 00126 #ifdef DISAGGREGATED_ENV_CHAR 00127 isEnabledGyroNotify = false; 00128 isEnabledAccNotify = false; 00129 isEnabledMagNotify = false; 00130 #endif 00131 isEnabledAccGyroMagNotify = false; 00132 isEnabledPresHumTempNotify = false; 00133 00134 isTempCalibrated = false; 00135 isHumCalibrated = false; 00136 isPresCalibrated = false; 00137 isMagCalibrated = false; 00138 isAccCalibrated = false; 00139 isAGyroCalibrated = false; 00140 00141 memset (pastenvTemperature, 0, TEMP_DATA_LEN); 00142 memset (pastenvHumidity, 0, HUM_DATA_LEN); 00143 memset (pastenvPressure, 0, PRES_DATA_LEN); 00144 00145 isBTLEConnected = DISCONNECTED; 00146 serviceAdded = true; 00147 } 00148 #ifdef DISAGGREGATED_ENV_CHAR 00149 uint32_t sendEnvTemperature (int16_t Temp, uint16_t TimeStamp) { 00150 STORE_LE_16(envTemperature,TimeStamp); 00151 STORE_LE_16(envTemperature+2,Temp); 00152 memcpy (pastenvTemperature, envTemperature, TEMP_DATA_LEN); 00153 return ble.gattServer().write(envTemperatureCharacteristic.getValueAttribute().getHandle(), envTemperature, TEMP_DATA_LEN, 0); 00154 } 00155 00156 /** 00157 * Update the temperature with a new value. Valid values range from 00158 * 0..100. Anything outside this range will be ignored. 00159 * @param newLevel New level. */ 00160 uint32_t updateEnvTemperature (int16_t Temp, uint16_t TimeStamp) { 00161 if (memcmp (&pastenvTemperature[2], &Temp, 2) != 0) { 00162 return sendEnvTemperature (Temp, TimeStamp); 00163 } 00164 return 0; 00165 } 00166 00167 uint32_t sendEnvHumidity(uint16_t Hum, uint16_t TimeStamp) { 00168 STORE_LE_16(envHumidity,TimeStamp); 00169 STORE_LE_16(envHumidity+2,Hum); 00170 memcpy (pastenvHumidity, envHumidity, HUM_DATA_LEN); 00171 return ble.gattServer().write(envHumidityCharacteristic.getValueAttribute().getHandle(), envHumidity, HUM_DATA_LEN, 0); 00172 } 00173 00174 uint32_t updateEnvHumidity(uint16_t Hum, uint16_t TimeStamp) { 00175 if (memcmp (&pastenvHumidity[2], &Hum, 2) != 0) { 00176 return sendEnvHumidity(Hum, TimeStamp); 00177 } 00178 return 0; 00179 } 00180 00181 uint32_t sendEnvPressure(uint32_t Press, uint16_t TimeStamp) { 00182 STORE_LE_16(envPressure,TimeStamp); 00183 STORE_LE_32(envPressure+2,Press); 00184 memcpy (pastenvPressure, envPressure, PRES_DATA_LEN); 00185 return ble.gattServer().write(envPressureCharacteristic.getValueAttribute().getHandle(), envPressure, PRES_DATA_LEN, 0); 00186 } 00187 00188 uint32_t updateEnvPressure(uint32_t Press, uint16_t TimeStamp) { 00189 if (memcmp (&pastenvPressure[2], &Press, 2) != 0) { 00190 return sendEnvPressure(Press, TimeStamp); 00191 } 00192 return 0; 00193 } 00194 #endif 00195 00196 uint32_t sendEnvPresHumTemp(uint32_t Press, uint16_t Hum, int16_t Temp, uint16_t TimeStamp) { 00197 STORE_LE_16(envPressure,TimeStamp); 00198 STORE_LE_32(envPressure+2,Press); 00199 memcpy (pastenvPressure, envPressure, PRES_DATA_LEN); 00200 STORE_LE_16(envHumidity,TimeStamp); 00201 STORE_LE_16(envHumidity+2,Hum); 00202 memcpy (pastenvHumidity, envHumidity, HUM_DATA_LEN); 00203 STORE_LE_16(envTemperature,TimeStamp); 00204 STORE_LE_16(envTemperature+2,Temp); 00205 memcpy (pastenvTemperature, envTemperature, TEMP_DATA_LEN); 00206 STORE_LE_16(envPresHumTemp, TimeStamp); 00207 memcpy (envPresHumTemp+2, envPressure+2, 4); 00208 memcpy (envPresHumTemp+2+4, envHumidity+2, 2); 00209 memcpy (envPresHumTemp+2+4+2, envTemperature+2, 2); 00210 return ble.gattServer().write(envPresHumTempCharacteristic.getValueAttribute().getHandle(), envPresHumTemp, PRESHUMTEMP_DATA_LEN, 0); 00211 } 00212 00213 uint32_t updateEnvPresHumTemp(uint32_t Press, uint16_t Hum, int16_t Temp, uint16_t TimeStamp) { 00214 if ((memcmp (&pastenvPressure[2], &Press, 2) != 0) || 00215 (memcmp (&pastenvHumidity[2], &Hum, 2) != 0) || 00216 (memcmp (&pastenvTemperature[2], &Temp, 2) != 0)) { 00217 return sendEnvPresHumTemp (Press, Hum, Temp, TimeStamp); 00218 } 00219 return 0; 00220 } 00221 #ifdef DISAGGREGATED_MOT_CHAR 00222 uint32_t sendEnvMagnetometer(AxesRaw_TypeDef *Magn, uint16_t TimeStamp, osxMFX_calibFactor *magOffset) { 00223 STORE_LE_16(envMagn,TimeStamp); 00224 STORE_LE_16(envMagn+2,(Magn->AXIS_X - magOffset->magOffX)); 00225 STORE_LE_16(envMagn+4,(Magn->AXIS_Y - magOffset->magOffY)); 00226 STORE_LE_16(envMagn+6,(Magn->AXIS_Z - magOffset->magOffZ)); 00227 return ble.gattServer().write(envMagnetometerCharacteristic.getValueAttribute().getHandle(), envMagn, MAG_DATA_LEN, 0); 00228 } 00229 00230 uint32_t updateEnvMagnetometer(AxesRaw_TypeDef *Magn, uint16_t TimeStamp, osxMFX_calibFactor *magOffset) { 00231 if (isMagNotificationEn()) return sendEnvMagnetometer(Magn, TimeStamp, magOffset); 00232 return 0; 00233 } 00234 00235 uint32_t sendEnvAccelerometer (AxesRaw_TypeDef *Acc, uint16_t TimeStamp) { 00236 STORE_LE_16(envAcce,TimeStamp); 00237 STORE_LE_16(envAcce+2,Acc->AXIS_X); 00238 STORE_LE_16(envAcce+4,Acc->AXIS_Y); 00239 STORE_LE_16(envAcce+6,Acc->AXIS_Z); 00240 return ble.gattServer().write(envAccelerometerCharacteristic.getValueAttribute().getHandle(), envAcce, ACC_DATA_LEN, 0); 00241 } 00242 00243 uint32_t updateEnvAccelerometer (AxesRaw_TypeDef *Acc, uint16_t TimeStamp) { 00244 if (isAccNotificationEn()) return sendEnvAccelerometer (Acc, TimeStamp); 00245 return 0; 00246 } 00247 00248 uint32_t sendEnvGyroscope (AxesRaw_TypeDef *Gyro, uint16_t TimeStamp) { 00249 STORE_LE_16(envGyro,TimeStamp); 00250 STORE_LE_16(envGyro+2,Gyro->AXIS_X); 00251 STORE_LE_16(envGyro+4,Gyro->AXIS_Y); 00252 STORE_LE_16(envGyro+6,Gyro->AXIS_Z); 00253 return ble.gattServer().write(envGyroCharacteristic.getValueAttribute().getHandle(), envGyro, GYRO_DATA_LEN, 0); 00254 } 00255 00256 uint32_t updateEnvGyroscope (AxesRaw_TypeDef *Gyro, uint16_t TimeStamp) { 00257 if (isGyroNotificationEn()) return sendEnvGyroscope (Gyro, TimeStamp); 00258 return 0; 00259 } 00260 #endif 00261 uint32_t sendEnvAccGyroMag (AxesRaw_TypeDef *Acc, AxesRaw_TypeDef *Gyro, AxesRaw_TypeDef *Magn, uint16_t TimeStamp, osxMFX_calibFactor *magOffset) { 00262 STORE_LE_16(envAccGyroMag,TimeStamp); 00263 STORE_LE_16(envAccGyroMag+2,Acc->AXIS_X); 00264 STORE_LE_16(envAccGyroMag+4,Acc->AXIS_Y); 00265 STORE_LE_16(envAccGyroMag+6,Acc->AXIS_Z); 00266 00267 STORE_LE_16(envAccGyroMag+8,Gyro->AXIS_X); 00268 STORE_LE_16(envAccGyroMag+10,Gyro->AXIS_Y); 00269 STORE_LE_16(envAccGyroMag+12,Gyro->AXIS_Z); 00270 00271 STORE_LE_16(envAccGyroMag+14,(Magn->AXIS_X - magOffset->magOffX)); 00272 STORE_LE_16(envAccGyroMag+16,(Magn->AXIS_Y - magOffset->magOffY)); 00273 STORE_LE_16(envAccGyroMag+18,(Magn->AXIS_Z - magOffset->magOffZ)); 00274 return ble.gattServer().write(envAccGyroMagCharacteristic.getValueAttribute().getHandle(), envAccGyroMag, ACCGYROMAG_DATA_LEN, 0); 00275 } 00276 00277 uint32_t updateEnvAccGyroMag (AxesRaw_TypeDef *Acc, AxesRaw_TypeDef *Gyro, AxesRaw_TypeDef *Magn, uint16_t TimeStamp, osxMFX_calibFactor *magOffset) { 00278 if (isAccGyroMagNotificationEn()) return sendEnvAccGyroMag (Acc, Gyro, Magn, TimeStamp, magOffset); 00279 return 0; 00280 } 00281 void enNotify (Gap::Handle_t a_handle) { 00282 Gap::Handle_t handle = a_handle - BLE_HANDLE_EN_DIS_OFFSET; 00283 #ifdef DISAGGREGATED_ENV_CHAR 00284 if (isTempHandle(handle)) { 00285 isEnabledTempNotify = true; 00286 memset(pastenvTemperature,0,TEMP_DATA_LEN); 00287 printf ("isTempHandle en\n\r"); 00288 return; 00289 } 00290 if (isHumHandle(handle)) { 00291 isEnabledHumNotify = true; 00292 memset(pastenvHumidity,0,HUM_DATA_LEN); 00293 printf ("isHumHandle en\n\r"); 00294 return; 00295 } 00296 if (isPresHandle(handle)) { 00297 isEnabledPresNotify = true; 00298 memset(pastenvPressure,0,PRES_DATA_LEN); 00299 printf ("isPresHandle en\n\r"); 00300 return; 00301 } 00302 #endif 00303 #ifdef DISAGGREGATED_ENV_CHAR 00304 if (isGyroHandle(handle)) { 00305 isEnabledGyroNotify = true; 00306 printf ("isGyroHandle en\n\r"); 00307 return; 00308 } 00309 if (isAccHandle(handle)) { 00310 isEnabledAccNotify = true; 00311 printf ("isAccHandle en\n\r"); 00312 return; 00313 } 00314 if (isMagHandle(handle)) { 00315 isEnabledMagNotify = true; 00316 printf ("isMagHandle en\n\r"); 00317 return; 00318 } 00319 #endif 00320 if (isAccGyroMagHandle(handle)) { 00321 isEnabledAccGyroMagNotify = true; 00322 printf ("isAccGyroMagHandle en\n\r"); 00323 return; 00324 } 00325 if (isPresHumTempHandle(handle)) { 00326 isEnabledPresHumTempNotify = true; 00327 printf ("isPresHumTempHandle en\n\r"); 00328 return; 00329 } 00330 } 00331 00332 void disNotify (Gap::Handle_t a_handle) { 00333 Gap::Handle_t handle = a_handle - BLE_HANDLE_EN_DIS_OFFSET; 00334 #ifdef DISAGGREGATED_ENV_CHAR 00335 if (isTempHandle(handle)) { 00336 isEnabledTempNotify = false; 00337 memset(pastenvTemperature,0,TEMP_DATA_LEN); 00338 printf ("isTempHandle dis\n\r"); 00339 return; 00340 } 00341 if (isHumHandle(handle)) { 00342 isEnabledHumNotify = false; 00343 memset(pastenvHumidity,0,HUM_DATA_LEN); 00344 printf ("isHumHandle dis\n\r"); 00345 return; 00346 } 00347 if (isPresHandle(handle)) { 00348 isEnabledPresNotify = false; 00349 memset(pastenvPressure,0,PRES_DATA_LEN); 00350 printf ("isPresHandle dis\n\r"); 00351 return; 00352 } 00353 #endif 00354 #ifdef DISAGGREGATED_ENV_CHAR 00355 if (isGyroHandle(handle)) { 00356 isEnabledGyroNotify = false; 00357 printf ("isGyroHandle dis\n\r"); 00358 return; 00359 } 00360 if (isAccHandle(handle)) { 00361 isEnabledAccNotify = false; 00362 printf ("isAccHandle dis\n\r"); 00363 return; 00364 } 00365 if (isMagHandle(handle)) { 00366 isEnabledMagNotify = false; 00367 printf ("isMagHandle dis\n\r"); 00368 return; 00369 } 00370 #endif 00371 if (isAccGyroMagHandle(handle)) { 00372 isEnabledAccGyroMagNotify = false; 00373 printf ("isAccGyroMagHandle dis\n\r"); 00374 return; 00375 } 00376 if (isPresHumTempHandle(handle)) { 00377 isEnabledPresHumTempNotify = false; 00378 printf ("isPresHumTempHandle dis\n\r"); 00379 return; 00380 } 00381 } 00382 #ifdef DISAGGREGATED_ENV_CHAR 00383 bool isTempNotificationEn (void) { 00384 return isEnabledTempNotify; 00385 } 00386 00387 bool isHumNotificationEn (void) { 00388 return isEnabledHumNotify; 00389 } 00390 00391 bool isPresNotificationEn (void) { 00392 return isEnabledPresNotify; 00393 } 00394 #endif 00395 #ifdef DISAGGREGATED_MOT_CHAR 00396 bool isGyroNotificationEn (void) { 00397 return isEnabledGyroNotify; 00398 } 00399 00400 bool isAccNotificationEn (void) { 00401 return isEnabledAccNotify; 00402 } 00403 00404 bool isMagNotificationEn (void) { 00405 return isEnabledMagNotify; 00406 } 00407 #endif 00408 bool isAccGyroMagNotificationEn (void) { 00409 return isEnabledAccGyroMagNotify; 00410 } 00411 00412 bool isPresHumTempNotificationEn (void) { 00413 return isEnabledPresHumTempNotify; 00414 } 00415 #ifdef DISAGGREGATED_ENV_CHAR 00416 bool isTempHandle (Gap::Handle_t handle) { 00417 if (handle == envTemperatureCharacteristic.getValueAttribute().getHandle() - BLE_HANDLE_VALUE_OFFSET) return true; 00418 return false; 00419 } 00420 00421 bool isHumHandle (Gap::Handle_t handle) { 00422 if (handle == envHumidityCharacteristic.getValueAttribute().getHandle() - BLE_HANDLE_VALUE_OFFSET) return true; 00423 return false; 00424 } 00425 00426 bool isPresHandle (Gap::Handle_t handle) { 00427 if (handle == envPressureCharacteristic.getValueAttribute().getHandle() - BLE_HANDLE_VALUE_OFFSET) return true; 00428 return false; 00429 } 00430 #endif 00431 #ifdef DISAGGREGATED_MOT_CHAR 00432 bool isMagHandle (Gap::Handle_t handle) { 00433 if (handle == envMagnetometerCharacteristic.getValueAttribute().getHandle() - BLE_HANDLE_VALUE_OFFSET) return true; 00434 return false; 00435 } 00436 bool isAccHandle (Gap::Handle_t handle) { 00437 if (handle == envAccelerometerCharacteristic.getValueAttribute().getHandle() - BLE_HANDLE_VALUE_OFFSET) return true; 00438 return false; 00439 } 00440 bool isGyroHandle (Gap::Handle_t handle) { 00441 if (handle == envGyroCharacteristic.getValueAttribute().getHandle() - BLE_HANDLE_VALUE_OFFSET) return true; 00442 return false; 00443 } 00444 #endif 00445 bool isAccGyroMagHandle (Gap::Handle_t handle) { 00446 if (handle == envAccGyroMagCharacteristic.getValueAttribute().getHandle() - BLE_HANDLE_VALUE_OFFSET) return true; 00447 return false; 00448 } 00449 bool isPresHumTempHandle (Gap::Handle_t handle) { 00450 if (handle == envPresHumTempCharacteristic.getValueAttribute().getHandle() - BLE_HANDLE_VALUE_OFFSET) return true; 00451 return false; 00452 } 00453 00454 00455 void updateConnectionStatus(ConnectionStatus_t status) { 00456 #ifdef DISAGGREGATED_ENV_CHAR 00457 isEnabledTempNotify = false; 00458 isEnabledHumNotify = false; 00459 isEnabledPresNotify = false; 00460 #endif 00461 #ifdef DISAGGREGATED_MOT_CHAR 00462 isEnabledGyroNotify = false; 00463 isEnabledAccNotify = false; 00464 isEnabledMagNotify = false; 00465 #endif 00466 isEnabledPresHumTempNotify = false; 00467 isEnabledAccGyroMagNotify = false; 00468 00469 isTempCalibrated = false; 00470 isHumCalibrated = false; 00471 isPresCalibrated = false; 00472 isMagCalibrated = false; 00473 isAccCalibrated = false; 00474 isAGyroCalibrated = false; 00475 00476 memset (pastenvTemperature, 0, TEMP_DATA_LEN); 00477 memset (pastenvHumidity, 0, HUM_DATA_LEN); 00478 memset (pastenvPressure, 0, PRES_DATA_LEN); 00479 isBTLEConnected = status; 00480 } 00481 00482 00483 private: 00484 BLE &ble; 00485 uint8_t envTemperature [TEMP_DATA_LEN]; /* in C */ 00486 uint8_t pastenvTemperature [TEMP_DATA_LEN]; 00487 uint8_t envHumidity [HUM_DATA_LEN]; /* in % */ 00488 uint8_t pastenvHumidity [HUM_DATA_LEN]; 00489 uint8_t envPressure [PRES_DATA_LEN]; /* in mBar */ 00490 uint8_t pastenvPressure [PRES_DATA_LEN]; 00491 uint8_t envMagn [MAG_DATA_LEN]; 00492 uint8_t envGyro [GYRO_DATA_LEN]; 00493 uint8_t envAcce [ACC_DATA_LEN]; 00494 uint8_t envAccGyroMag [ACCGYROMAG_DATA_LEN]; 00495 uint8_t envPresHumTemp [PRESHUMTEMP_DATA_LEN]; 00496 #ifdef DISAGGREGATED_ENV_CHAR 00497 GattCharacteristic envTemperatureCharacteristic; 00498 GattCharacteristic envHumidityCharacteristic; 00499 GattCharacteristic envPressureCharacteristic; 00500 #endif 00501 #ifdef DISAGGREGATED_MOT_CHAR 00502 GattCharacteristic envMagnetometerCharacteristic; 00503 GattCharacteristic envAccelerometerCharacteristic; 00504 GattCharacteristic envGyroCharacteristic; 00505 #endif 00506 GattCharacteristic envAccGyroMagCharacteristic; 00507 GattCharacteristic envPresHumTempCharacteristic; 00508 00509 ConnectionStatus_t isBTLEConnected; 00510 #ifdef DISAGGREGATED_ENV_CHAR 00511 bool isEnabledTempNotify; 00512 bool isEnabledHumNotify; 00513 bool isEnabledPresNotify; 00514 #endif 00515 #ifdef DISAGGREGATED_MOT_CHAR 00516 bool isEnabledGyroNotify; 00517 bool isEnabledAccNotify; 00518 bool isEnabledMagNotify; 00519 #endif 00520 bool isEnabledAccGyroMagNotify; 00521 bool isEnabledPresHumTempNotify; 00522 00523 bool isTempCalibrated; 00524 bool isHumCalibrated; 00525 bool isPresCalibrated; 00526 bool isMagCalibrated; 00527 bool isAccCalibrated; 00528 bool isAGyroCalibrated; 00529 }; 00530 00531 #endif /* #ifndef __CUSTOM_BLE_SENSORS_SERVICE_H__*/
Generated on Tue Jul 12 2022 21:01:59 by
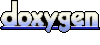