
A simple application providing an example of asynchronous access to the X-NUCLEO_NFC01A1 Dynamic NFC Tag board.
Dependencies: NDefLib X_NUCLEO_NFC01A1 mbed
Fork of HelloWord_Async_NFC01A1 by
main.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file main.cpp 00004 * @date 22/01/2016 00005 * @brief Test the async comunication api 00006 ****************************************************************************** 00007 * 00008 * COPYRIGHT(c) 2015 STMicroelectronics 00009 * 00010 * Redistribution and use in source and binary forms, with or without modification, 00011 * are permitted provided that the following conditions are met: 00012 * 1. Redistributions of source code must retain the above copyright notice, 00013 * this list of conditions and the following disclaimer. 00014 * 2. Redistributions in binary form must reproduce the above copyright notice, 00015 * this list of conditions and the following disclaimer in the documentation 00016 * and/or other materials provided with the distribution. 00017 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00018 * may be used to endorse or promote products derived from this software 00019 * without specific prior written permission. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00022 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00023 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00024 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00025 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00026 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00027 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00028 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00029 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00030 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00031 * 00032 ****************************************************************************** 00033 */ 00034 00035 #include "mbed.h" 00036 #include "XNucleoNFC01A1.h" 00037 #include "ReadUriCallbacks.h" 00038 #include "WriteUriCallbacks.h" 00039 #include "NDefNfcTagM24SR.h" 00040 #include "NDefLib/RecordType/RecordURI.h" 00041 00042 00043 /** variable set to true when we receive an interrupt from the nfc component*/ 00044 static volatile bool nfcInterruptFlag=false; 00045 00046 /** variable set to true when the user press the board user button*/ 00047 static volatile bool buttonPress=false; 00048 00049 /** Nfc ISR called when the nfc component has a message ready*/ 00050 static void nfc_interrupt_callback() { 00051 nfcInterruptFlag=true; 00052 }//nfcInterruptCallback 00053 00054 static void set_button_press() { 00055 buttonPress=true; 00056 }//if buttonPress 00057 00058 int main(int argc,char *args[]) { 00059 (void)argc; (void)args; 00060 00061 #if defined(TARGET_STM) 00062 InterruptIn userButton(USER_BUTTON); 00063 userButton.fall(set_button_press); 00064 #endif 00065 00066 //create the nfc component 00067 I2C i2cChannel(XNucleoNFC01A1::DEFAULT_SDA_PIN,XNucleoNFC01A1::DEFAULT_SDL_PIN); 00068 00069 XNucleoNFC01A1 *nfcNucleo = XNucleoNFC01A1::instance(i2cChannel,&nfc_interrupt_callback, 00070 XNucleoNFC01A1::DEFAULT_GPO_PIN,XNucleoNFC01A1::DEFAULT_RF_DISABLE_PIN, 00071 XNucleoNFC01A1::DEFAULT_LED1_PIN,XNucleoNFC01A1::DEFAULT_LED2_PIN, 00072 XNucleoNFC01A1::DEFAULT_LED3_PIN); 00073 00074 //No call back needed since default behavior is sync 00075 nfcNucleo->get_M24SR().get_session(); 00076 nfcNucleo->get_M24SR().manage_I2C_GPO(M24SR::I2C_ANSWER_READY); //switch to async mode 00077 00078 NDefLib::NDefNfcTag &tag = nfcNucleo->get_M24SR().get_NDef_tag(); 00079 printf("System Init done!\n\r"); 00080 00081 //crate the callback to use for write a tag 00082 WriteUriCallbacks NDefWriteCallback(nfcNucleo->get_led1(),nfcNucleo->get_led2(),nfcNucleo->get_led3()); 00083 ReadUriCallbacks NDefReadCallback(nfcNucleo->get_led1(),nfcNucleo->get_led2(),nfcNucleo->get_led3()); 00084 tag.set_callback(&NDefWriteCallback); //set the callback 00085 tag.open_session(); //start the callback chain 00086 00087 printf("Start Main Loop\n\r"); 00088 while(true) { 00089 if (nfcInterruptFlag) { 00090 nfcInterruptFlag=false; 00091 //manage an async event from the nfc component 00092 nfcNucleo->get_M24SR().manage_event(); 00093 }//if 00094 #if defined(TARGET_STM) 00095 if (buttonPress) { 00096 buttonPress=false; 00097 printf("Pressed"); 00098 tag.set_callback(&NDefReadCallback); 00099 tag.open_session(); 00100 } 00101 #endif 00102 __WFE(); 00103 }//while 00104 00105 //return 0; 00106 }
Generated on Tue Jul 12 2022 22:53:54 by
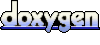