
SD demo
Dependencies: BSP_DISCO_F413ZH mbed
main.cpp
00001 #include "mbed.h" 00002 #include "stm32f413h_discovery.h" 00003 #include "stm32f413h_discovery_lcd.h" 00004 #include "stm32f413h_discovery_sd.h" 00005 00006 #define BLOCK_START_ADDR 0 /* Block start address */ 00007 #define NUM_OF_BLOCKS 5 /* Total number of blocks */ 00008 #define BUFFER_WORDS_SIZE ((512 * NUM_OF_BLOCKS) >> 2) /* Total data size in bytes */ 00009 00010 uint32_t aTxBuffer[BUFFER_WORDS_SIZE]; 00011 uint32_t aRxBuffer[BUFFER_WORDS_SIZE]; 00012 00013 static void Fill_Buffer(uint32_t *pBuffer, uint32_t uwBufferLenght, uint32_t uwOffset); 00014 static uint8_t Buffercmp(uint32_t* pBuffer1, uint32_t* pBuffer2, uint16_t BufferLength); 00015 static void print_error(uint8_t *msg); 00016 00017 int main() 00018 { 00019 /* Init LCD and display example information */ 00020 BSP_LCD_Init(); 00021 BSP_LCD_Clear(LCD_COLOR_WHITE); 00022 BSP_LCD_SetFont(&Font16); 00023 00024 /* SD init */ 00025 if (BSP_SD_Init() == MSD_OK) { 00026 BSP_LCD_SetTextColor(LCD_COLOR_GREEN); 00027 BSP_LCD_FillRect(0, 0, BSP_LCD_GetXSize(), 40); 00028 BSP_LCD_SetTextColor(LCD_COLOR_BLACK); 00029 BSP_LCD_SetBackColor(LCD_COLOR_GREEN); 00030 BSP_LCD_DisplayStringAt(0, 15, (uint8_t *)"SD detected", CENTER_MODE); 00031 BSP_LCD_SetBackColor(LCD_COLOR_WHITE); 00032 } else { 00033 BSP_LCD_SetTextColor(LCD_COLOR_RED); 00034 BSP_LCD_FillRect(0, 0, BSP_LCD_GetXSize(), 40); 00035 BSP_LCD_SetTextColor(LCD_COLOR_BLACK); 00036 BSP_LCD_SetBackColor(LCD_COLOR_RED); 00037 BSP_LCD_DisplayStringAt(0, 15, (uint8_t *)"SD not detected", CENTER_MODE); 00038 print_error((uint8_t *)"Demo aborted"); 00039 } 00040 00041 wait(1); 00042 // Check if SD card is detected 00043 if (BSP_SD_IsDetected() == SD_PRESENT) { 00044 BSP_LCD_DisplayStringAt(0, 50, (uint8_t *)"SD detected OK", CENTER_MODE); 00045 } else { 00046 print_error((uint8_t *)"SD not detected"); 00047 } 00048 00049 wait(1); 00050 // Erase SD card 00051 if(BSP_SD_Erase(BLOCK_START_ADDR, (512 * NUM_OF_BLOCKS)) == MSD_OK) { 00052 BSP_LCD_DisplayStringAt(0, 70, (uint8_t *)"SD erased OK", CENTER_MODE); 00053 } else { 00054 print_error((uint8_t *)"Erased failed"); 00055 } 00056 00057 wait(1); 00058 // Write data in SD card 00059 Fill_Buffer(aTxBuffer, BUFFER_WORDS_SIZE, 0x22FF); 00060 if (BSP_SD_WriteBlocks(aTxBuffer, BLOCK_START_ADDR, NUM_OF_BLOCKS, SD_DATATIMEOUT) == MSD_OK) { 00061 BSP_LCD_DisplayStringAt(0, 90, (uint8_t *)"SD write OK", CENTER_MODE); 00062 } else { 00063 print_error((uint8_t *)"Write failed"); 00064 } 00065 00066 wait(1); 00067 // Read data from SD card and verify them 00068 if (BSP_SD_ReadBlocks(aRxBuffer, BLOCK_START_ADDR, NUM_OF_BLOCKS, SD_DATATIMEOUT) == MSD_OK) { 00069 BSP_LCD_DisplayStringAt(0, 110, (uint8_t *)"SD read OK", CENTER_MODE); 00070 if (Buffercmp(aTxBuffer, aRxBuffer, BUFFER_WORDS_SIZE) == 0) { 00071 BSP_LCD_DisplayStringAt(0, 130, (uint8_t *)"Data OK", CENTER_MODE); 00072 } else { 00073 print_error((uint8_t *)"Data wrong"); 00074 } 00075 } else { 00076 print_error((uint8_t *)"Read failed"); 00077 } 00078 00079 wait(1); 00080 BSP_LCD_DisplayStringAt(0, 200, (uint8_t *)"Demo finished OK", CENTER_MODE); 00081 while(1); 00082 } 00083 00084 /** 00085 * @brief Fills buffer with user predefined data. 00086 * @param pBuffer: pointer on the buffer to fill 00087 * @param uwBufferLenght: size of the buffer to fill 00088 * @param uwOffset: first value to fill on the buffer 00089 * @retval None 00090 */ 00091 static void Fill_Buffer(uint32_t *pBuffer, uint32_t uwBufferLength, uint32_t uwOffset) 00092 { 00093 uint32_t tmpIndex = 0; 00094 00095 /* Put in global buffer different values */ 00096 for (tmpIndex = 0; tmpIndex < uwBufferLength; tmpIndex++ ) { 00097 pBuffer[tmpIndex] = tmpIndex + uwOffset; 00098 } 00099 } 00100 00101 /** 00102 * @brief Compares two buffers. 00103 * @param pBuffer1, pBuffer2: buffers to be compared. 00104 * @param BufferLength: buffer's length 00105 * @retval 1: pBuffer identical to pBuffer1 00106 * 0: pBuffer differs from pBuffer1 00107 */ 00108 static uint8_t Buffercmp(uint32_t* pBuffer1, uint32_t* pBuffer2, uint16_t BufferLength) 00109 { 00110 while (BufferLength--) { 00111 if (*pBuffer1 != *pBuffer2) { 00112 return 1; 00113 } 00114 00115 pBuffer1++; 00116 pBuffer2++; 00117 } 00118 00119 return 0; 00120 } 00121 00122 /** 00123 * @brief Print an error message on LCD and stop 00124 * @param Message string 00125 */ 00126 static void print_error(uint8_t *msg) 00127 { 00128 BSP_LCD_SetBackColor(LCD_COLOR_WHITE); 00129 BSP_LCD_SetTextColor(LCD_COLOR_RED); 00130 BSP_LCD_DisplayStringAt(0, 200, msg, CENTER_MODE); 00131 while(1); 00132 }
Generated on Sat Jul 16 2022 11:21:13 by
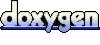