
Publisher for IBM Quickstart and Watson IoT cloud.
Dependencies: MQTT NDefLib X_NUCLEO_IKS01A2 X_NUCLEO_NFC01A1
Fork of Cloud_IBM_MbedOS by
main.cpp
00001 /* SpwfInterface NetworkSocketAPI Example Program 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include <string.h> 00019 #include "easy-connect.h" 00020 #include "MQTTClient.h" 00021 #include "XNucleoIKS01A2.h" 00022 #include "XNucleoNFC01A1.h" 00023 #include "NDefNfcTag.h" 00024 #include "NDefLib/RecordType/RecordURI.h" 00025 #include "RecordWifiConf.h" 00026 #include "MQTTmbed.h" 00027 #include "MQTTNetwork.h" 00028 00029 /**** System configuration define ****/ 00030 #define ORG_QUICKSTART // comment to connect to play.internetofthings.ibmcloud.com 00031 //#define SUBSCRIBE // uncomment to subscribe to broker msgs (not to be used with IBM broker) 00032 #define X_NUCLEO_NFC01A1_PRESENT // uncomment to add NFC support 00033 #ifndef ORG_QUICKSTART 00034 //#define TLS_EN // uncomment to add TLS to NON quickstart connections 00035 #endif 00036 00037 #ifdef TLS_EN // Digicert Root Certificate in PEM format (from IBM website) 00038 const char SSL_CA_PEM[] ="-----BEGIN CERTIFICATE-----\n" 00039 "MIIDrzCCApegAwIBAgIQCDvgVpBCRrGhdWrJWZHHSjANBgkqhkiG9w0BAQUFADBh\n" 00040 "MQswCQYDVQQGEwJVUzEVMBMGA1UEChMMRGlnaUNlcnQgSW5jMRkwFwYDVQQLExB3\n" 00041 "d3cuZGlnaWNlcnQuY29tMSAwHgYDVQQDExdEaWdpQ2VydCBHbG9iYWwgUm9vdCBD\n" 00042 "QTAeFw0wNjExMTAwMDAwMDBaFw0zMTExMTAwMDAwMDBaMGExCzAJBgNVBAYTAlVT\n" 00043 "MRUwEwYDVQQKEwxEaWdpQ2VydCBJbmMxGTAXBgNVBAsTEHd3dy5kaWdpY2VydC5j\n" 00044 "b20xIDAeBgNVBAMTF0RpZ2lDZXJ0IEdsb2JhbCBSb290IENBMIIBIjANBgkqhkiG\n" 00045 "9w0BAQEFAAOCAQ8AMIIBCgKCAQEA4jvhEXLeqKTTo1eqUKKPC3eQyaKl7hLOllsB\n" 00046 "CSDMAZOnTjC3U/dDxGkAV53ijSLdhwZAAIEJzs4bg7/fzTtxRuLWZscFs3YnFo97\n" 00047 "nh6Vfe63SKMI2tavegw5BmV/Sl0fvBf4q77uKNd0f3p4mVmFaG5cIzJLv07A6Fpt\n" 00048 "43C/dxC//AH2hdmoRBBYMql1GNXRor5H4idq9Joz+EkIYIvUX7Q6hL+hqkpMfT7P\n" 00049 "T19sdl6gSzeRntwi5m3OFBqOasv+zbMUZBfHWymeMr/y7vrTC0LUq7dBMtoM1O/4\n" 00050 "gdW7jVg/tRvoSSiicNoxBN33shbyTApOB6jtSj1etX+jkMOvJwIDAQABo2MwYTAO\n" 00051 "BgNVHQ8BAf8EBAMCAYYwDwYDVR0TAQH/BAUwAwEB/zAdBgNVHQ4EFgQUA95QNVbR\n" 00052 "TLtm8KPiGxvDl7I90VUwHwYDVR0jBBgwFoAUA95QNVbRTLtm8KPiGxvDl7I90VUw\n" 00053 "DQYJKoZIhvcNAQEFBQADggEBAMucN6pIExIK+t1EnE9SsPTfrgT1eXkIoyQY/Esr\n" 00054 "hMAtudXH/vTBH1jLuG2cenTnmCmrEbXjcKChzUyImZOMkXDiqw8cvpOp/2PV5Adg\n" 00055 "06O/nVsJ8dWO41P0jmP6P6fbtGbfYmbW0W5BjfIttep3Sp+dWOIrWcBAI+0tKIJF\n" 00056 "PnlUkiaY4IBIqDfv8NZ5YBberOgOzW6sRBc4L0na4UU+Krk2U886UAb3LujEV0ls\n" 00057 "YSEY1QSteDwsOoBrp+uvFRTp2InBuThs4pFsiv9kuXclVzDAGySj4dzp30d8tbQk\n" 00058 "CAUw7C29C79Fv1C5qfPrmAESrciIxpg0X40KPMbp1ZWVbd4=\n" 00059 "-----END CERTIFICATE-----\n"; 00060 #endif 00061 00062 //------------------------------------ 00063 // Hyperterminal configuration 00064 // 9600 bauds, 8-bit data, no parity 00065 //------------------------------------ 00066 static Serial pc(SERIAL_TX, SERIAL_RX); 00067 static DigitalOut myled(LED1); 00068 static bool quickstartMode = true; // set to false to connect with authentication tocken 00069 static bool BlueButtonToggle = false; 00070 00071 #define MQTT_MAX_PACKET_SIZE 400 00072 #define MQTT_MAX_PAYLOAD_SIZE 300 00073 00074 // Configuration values needed to connect to IBM IoT Cloud 00075 #ifdef ORG_QUICKSTART 00076 #define ORG "quickstart" // connect to quickstart.internetofthings.ibmcloud.com/ For a registered connection, replace with your org 00077 #define ID "" 00078 #define AUTH_TOKEN "" 00079 //#define DEFAULT_TYPE_NAME "iotsample-mbed-Nucleo" 00080 #define DEFAULT_TYPE_NAME "sensor" 00081 #define DEFAULT_PORT MQTT_PORT 00082 #else // not def ORG_QUICKSTART 00083 #define ORG MQTT_ORG_ID // connect to ORG.internetofthings.ibmcloud.com/ For a registered connection, replace with your org 00084 #define ID MQTT_DEVICE_ID // For a registered connection is your device id 00085 #define AUTH_TOKEN MQTT_DEVICE_PASSWORD // For a registered connection is a device auth-token 00086 #define DEFAULT_TYPE_NAME MQTT_DEVICE_TYPE // For a registered connection is device type 00087 #ifdef TLS_EN 00088 #define DEFAULT_PORT MQTT_TLS_PORT 00089 #else 00090 #define DEFAULT_PORT MQTT_PORT 00091 #endif 00092 #endif 00093 00094 #define TYPE DEFAULT_TYPE_NAME // For a registered connection, replace with your type 00095 #define IBM_IOT_PORT DEFAULT_PORT 00096 00097 #define MAXLEN_MBED_CONF_APP_WIFI_SSID 32 // same as WIFI_SSID_MAX_LEN in easy_connect 00098 #define MAXLEN_MBED_CONF_APP_WIFI_PASSWORD 64 // same as WIFI_PASSWORD_MAX_LEN 00099 00100 static char id[30] = ID; // mac without colons 00101 static char org[12] = ORG; 00102 static int connack_rc = 0; // MQTT connack return code 00103 static char type[30] = TYPE; 00104 static char auth_token[30] = AUTH_TOKEN; // Auth_token is only used in non-quickstart mode 00105 static bool netConnecting = false; 00106 static int connectTimeout = 1000; 00107 static bool mqttConnecting = false; 00108 static bool netConnected = false; 00109 static bool connected = false; 00110 static int retryAttempt = 0; 00111 static char subscription_url[MQTT_MAX_PAYLOAD_SIZE]; 00112 static char ssid[MAXLEN_MBED_CONF_APP_WIFI_SSID]; // Network must be visible otherwise it can't connect 00113 static char seckey[MAXLEN_MBED_CONF_APP_WIFI_PASSWORD]; 00114 00115 static LPS22HBSensor *pressure_sensor; 00116 static HTS221Sensor *humidity_sensor; 00117 static HTS221Sensor *temp_sensor1; 00118 00119 #ifdef X_NUCLEO_NFC01A1_PRESENT 00120 // Read from NFC the Wifi record 00121 void NFCReadRecordWIFI (XNucleoNFC01A1 *nfcNucleo) { 00122 00123 NDefLib::NDefNfcTag& tag = nfcNucleo->get_M24SR().get_NDef_tag(); 00124 00125 printf ("Write to NFC tag the WiFi record ...\n\r"); 00126 for (int ReadSSIDPassw=0; ReadSSIDPassw!=1; wait_ms(1000)) { 00127 //open the i2c session with the nfc chip 00128 NDefLib::Message readMsg; 00129 if(tag.open_session(1)){ 00130 tag.read(&readMsg); 00131 // printf ("---- N record %d\n\r", readMsg.get_N_records()); 00132 if(readMsg.get_N_records()==0){ 00133 printf("Error Read\r\n"); 00134 }else { 00135 for(uint32_t i=0;i<readMsg.get_N_records();i++){ 00136 NDefLib::Record *r = readMsg[i]; 00137 // printf ("N record %d\n\r", readMsg.get_N_records()); 00138 if (r != NULL) { 00139 // printf ("Record RecordType_t: %d\n\r", r->get_type()); 00140 if (r->get_type() == NDefLib::Record::TYPE_WIFI_CONF) { 00141 NDefLib::RecordWifiConf * temp = (NDefLib::RecordWifiConf*)r; 00142 sprintf (ssid, "%s", temp->get_network_ssid().c_str()); 00143 sprintf (seckey, "%s", temp->get_network_key().c_str()); 00144 printf ("Read SSID: %s Passw: %s\n\r", ssid, /*seckey*/"*****"); 00145 ReadSSIDPassw =1; 00146 } 00147 else if (r->get_type() == NDefLib::Record::TYPE_UNKNOWN) { printf ("NFC RECORD TYPE_UNKNOWN\n\r"); } 00148 } 00149 if (r != NULL) delete r; 00150 }//for all the NFC records 00151 }//nfc n records 00152 //close the i2c session 00153 if(!tag.close_session()){ 00154 printf("Error Closing the session\r\n"); 00155 } 00156 }else printf("Error open Session\r\n"); 00157 } 00158 } 00159 00160 // add to NFC the HTTP_BROKER_URL URL swapping the two NFC records in order to set the HTTP_BROKER_URL as a first 00161 // record allowing to read URL from any device. 00162 void NFCWriteRecordURI (XNucleoNFC01A1 *nfcNucleo) { 00163 00164 NDefLib::NDefNfcTag& tag = nfcNucleo->get_M24SR().get_NDef_tag(); 00165 //open the i2c session with the nfc chip 00166 if(tag.open_session()){ 00167 //create the NDef message and record 00168 NDefLib::Message msg; 00169 tag.read(&msg); 00170 // printf ("---- N record present: %d\n\r", msg.get_N_records()); 00171 NDefLib::Record *r = msg[0]; 00172 if ((r != NULL) && (r->get_type() == NDefLib::Record::TYPE_WIFI_CONF)) { // first time record 0 must be wifi 00173 NDefLib::RecordWifiConf * const pWIFI = (NDefLib::RecordWifiConf*)r; 00174 NDefLib::Message msg2; 00175 NDefLib::RecordURI rURL(NDefLib::RecordURI::HTTPS, subscription_url); 00176 msg2.add_record(&rURL); // add a new HTTP record to msg2 00177 msg2.add_record(pWIFI); // copy a Wifi record to msg2 00178 //write the tag 00179 if(tag.write(msg2)){ 00180 printf("Tag writed \r\n"); 00181 } 00182 NDefLib::Message::remove_and_delete_all_record(msg); 00183 } 00184 //close the i2c session 00185 if(!tag.close_session()){ 00186 printf("Error Closing the session\r\n"); 00187 } 00188 }else printf("Error open Session\r\n"); 00189 } 00190 00191 #endif 00192 00193 #ifndef TARGET_SENSOR_TILE 00194 static void BlueButtonPressed () 00195 { 00196 BlueButtonToggle = true; 00197 } 00198 #endif 00199 00200 #ifdef SUBSCRIBE 00201 void subscribe_cb(MQTT::MessageData & msgMQTT) { 00202 char msg[MQTT_MAX_PAYLOAD_SIZE]; 00203 msg[0]='\0'; 00204 strncat (msg, (char*)msgMQTT.message.payload, msgMQTT.message.payloadlen); 00205 printf ("--->>> subscribe_cb msg: %s\n\r", msg); 00206 } 00207 00208 int subscribe(char *pubTopic, MQTT::Client<MQTTNetwork, Countdown, MQTT_MAX_PACKET_SIZE>* client) 00209 { 00210 return client->subscribe(pubTopic, MQTT::QOS1, subscribe_cb); 00211 } 00212 #endif 00213 00214 int connect(MQTT::Client<MQTTNetwork, Countdown, MQTT_MAX_PACKET_SIZE>* client, MQTTNetwork *mqttNetwork, NetworkInterface* network) 00215 { 00216 const char* iot_ibm = MQTT_BROKER_URL; 00217 char hostname[strlen(org) + strlen(iot_ibm) + 1]; 00218 00219 sprintf(hostname, "%s%s", org, iot_ibm); 00220 // Construct clientId - d:org:type:id 00221 char clientId[strlen(org) + strlen(type) + strlen(id) + 5]; 00222 sprintf(clientId, "d:%s:%s:%s", org, type, id); 00223 sprintf(subscription_url, "%s.%s/#/device/%s/%s/", org, "internetofthings.ibmcloud.com", id, TYPE); 00224 00225 // Network debug statements 00226 LOG("=====================================\n\r"); 00227 LOG("Nucleo IP ADDRESS: %s\n\r", network->get_ip_address()); 00228 LOG("Nucleo MAC ADDRESS: %s\n\r", network->get_mac_address()); 00229 LOG("Server Hostname: %s port: %d\n\r", hostname, IBM_IOT_PORT); 00230 // for(int i = 0; clientId[i]; i++){ // set lowercase mac 00231 // clientId[i] = tolower(clientId[i]); 00232 // } 00233 LOG("Client ID: %s\n\r", clientId); 00234 LOG("Topic: %s\n\r",MQTT_TOPIC); 00235 LOG("Subscription URL: %s\n\r", subscription_url); 00236 LOG("=====================================\n\r"); 00237 netConnecting = true; 00238 00239 #ifdef ORG_QUICKSTART 00240 int tls = TLS_OFF; 00241 const char * cert = NULL; 00242 unsigned int sizeof_cert = 0; 00243 #else // if !QUICKSTART possible to connect with TLS or not 00244 #ifdef TLS_EN 00245 int tls = TLS_ON; 00246 const char * cert = SSL_CA_PEM; 00247 unsigned int sizeof_cert = sizeof(SSL_CA_PEM); 00248 #else 00249 int tls = TLS_OFF; 00250 const char * cert = 0; 00251 unsigned int sizeof_cert = 0; 00252 #endif 00253 #endif 00254 00255 int rc = mqttNetwork->connect(hostname, IBM_IOT_PORT, tls, cert, sizeof_cert); 00256 if (rc != 0) 00257 { 00258 printf("rc from TCP connect is %d\r\n", rc); 00259 return rc; 00260 } 00261 netConnected = true; 00262 netConnecting = false; 00263 00264 // MQTT Connect 00265 mqttConnecting = true; 00266 MQTTPacket_connectData data = MQTTPacket_connectData_initializer; 00267 data.MQTTVersion = 4; 00268 data.struct_version=0; 00269 data.clientID.cstring = clientId; 00270 data.keepAliveInterval = 0; //MQTT_KEEPALIVE; // in Sec 00271 if (!quickstartMode) 00272 { 00273 data.username.cstring = "use-token-auth"; 00274 data.password.cstring = auth_token; 00275 printf ("AutToken: %s\n\r", auth_token); 00276 } 00277 if ((rc = client->connect(data)) != MQTT::SUCCESS) { 00278 printf("rc from MQTT connect is %d\r\n", rc); 00279 connack_rc = rc; 00280 return rc; 00281 } 00282 connected = true; 00283 printf ("--->MQTT Connected\n\r"); 00284 #ifdef SUBSCRIBE 00285 int rc=0; 00286 if ((rc=subscribe(MQTT_TOPIC, client)) == 0) LOG ("--->>>MQTT subscribed to: %s\n\r",MQTT_TOPIC); 00287 else LOG ("--->>>ERROR MQTT subscribe : %s\n\r",MQTT_TOPIC); 00288 #endif 00289 mqttConnecting = false; 00290 connack_rc = rc; 00291 return rc; 00292 } 00293 00294 00295 int getConnTimeout(int attemptNumber) 00296 { // First 10 attempts try within 3 seconds, next 10 attempts retry after every 1 minute 00297 // after 20 attempts, retry every 10 minutes 00298 return (attemptNumber < 10) ? 3 : (attemptNumber < 20) ? 60 : 600; 00299 } 00300 00301 00302 void attemptConnect(MQTT::Client<MQTTNetwork, Countdown, MQTT_MAX_PACKET_SIZE>* client, MQTTNetwork *mqttNetwork, NetworkInterface* network) 00303 { 00304 connected = false; 00305 00306 while (connect(client, mqttNetwork, network) != MQTT_CONNECTION_ACCEPTED) 00307 { 00308 if (connack_rc == MQTT_NOT_AUTHORIZED || connack_rc == MQTT_BAD_USERNAME_OR_PASSWORD) { 00309 printf ("Error MQTT_BAD_USERNAME_OR_PASSWORDFile: %s, Line: %d Error: %d \n\r",__FILE__,__LINE__, connack_rc); 00310 return; // don't reattempt to connect if credentials are wrong 00311 } 00312 int timeout = getConnTimeout(++retryAttempt); 00313 WARN("Retry attempt number %d waiting %d\n", retryAttempt, timeout); 00314 00315 // if ipstack and client were on the heap we could deconstruct and goto a label where they are constructed 00316 // or maybe just add the proper members to do this disconnect and call attemptConnect(...) 00317 // this works - reset the system when the retry count gets to a threshold 00318 if (retryAttempt == 5) 00319 NVIC_SystemReset(); 00320 else 00321 wait(timeout); 00322 } 00323 } 00324 00325 int publish(MQTT::Client<MQTTNetwork, Countdown, MQTT_MAX_PACKET_SIZE>* client) 00326 { 00327 MQTT::Message message; 00328 const char* pubTopic = MQTT_TOPIC; 00329 00330 char buf[MQTT_MAX_PAYLOAD_SIZE]; 00331 float temp, press, hum; 00332 00333 if (!client->isConnected()) { printf ("publish failed: MQTT disconnected\n\r"); return MQTT::FAILURE; } 00334 temp_sensor1->get_temperature(&temp); 00335 pressure_sensor->get_pressure(&press); 00336 humidity_sensor->get_humidity(&hum); 00337 sprintf(buf, 00338 "{\"d\":{\"ST\":\"Nucleo-IoT-mbed\",\"Temp\":%0.4f,\"Pressure\":%0.4f,\"Humidity\":%0.4f}}", 00339 temp, press, hum); 00340 message.qos = MQTT::QOS0; 00341 message.retained = false; 00342 message.dup = false; 00343 message.payload = (void*)buf; 00344 message.payloadlen = strlen(buf); 00345 00346 if( (message.payloadlen + strlen(pubTopic)+1) >= MQTT_MAX_PACKET_SIZE ) 00347 printf("message too long!\r\n"); 00348 00349 LOG("Publishing %s\n\r", buf); 00350 return client->publish(pubTopic, message); 00351 } 00352 00353 00354 int main() 00355 { 00356 myled=0; 00357 DevI2C *i2c = new DevI2C(I2C_SDA, I2C_SCL); 00358 i2c->frequency(400000); 00359 00360 XNucleoIKS01A2 *mems_expansion_board = XNucleoIKS01A2::instance(i2c); 00361 pressure_sensor = mems_expansion_board->pt_sensor; 00362 temp_sensor1 = mems_expansion_board->ht_sensor; 00363 humidity_sensor = mems_expansion_board->ht_sensor; 00364 pressure_sensor->enable(); 00365 temp_sensor1->enable(); 00366 humidity_sensor->enable(); 00367 00368 #if !defined (TARGET_SENSOR_TILE) 00369 InterruptIn BlueButton(USER_BUTTON); 00370 BlueButton.fall(&BlueButtonPressed); 00371 BlueButtonToggle = false; 00372 #endif 00373 00374 pc.printf("\r\nCloud_IBM_MbedOS Application\r\n"); 00375 #if defined(MBED_MAJOR_VERSION) 00376 printf("Using Mbed OS %d.%d.%d\n", MBED_MAJOR_VERSION, MBED_MINOR_VERSION, MBED_PATCH_VERSION); 00377 #else 00378 printf("Using Mbed OS from master.\n"); 00379 #endif 00380 pc.printf("\r\nconnecting to AP\r\n"); 00381 00382 quickstartMode=false; 00383 if (strcmp(org, "quickstart") == 0){quickstartMode = true;} 00384 00385 #ifdef X_NUCLEO_NFC01A1_PRESENT 00386 // program NFC with broker URL 00387 XNucleoNFC01A1 *nfcNucleo = XNucleoNFC01A1::instance(*i2c, NULL, XNucleoNFC01A1::DEFAULT_GPO_PIN, XNucleoNFC01A1::DEFAULT_RF_DISABLE_PIN, NC,NC,NC); 00388 NDefLib::NDefNfcTag& tag = nfcNucleo->get_M24SR().get_NDef_tag(); 00389 printf("NFC Init done: !\r\n"); 00390 //open the i2c session with the nfc chip 00391 NFCReadRecordWIFI (nfcNucleo); 00392 NetworkInterface* network = easy_connect(true, ssid, seckey); // Wifi SSID and passw from NFC tag 00393 #else 00394 NetworkInterface* network = easy_connect(true); // // Wifi SSID and passw from mbed_app.json 00395 #endif 00396 if (!network) { 00397 printf ("Error easy_connect\n\r"); 00398 return -1; 00399 } 00400 //================= TODO Set System Time ideally from NTP srv or from shell 00401 #if 0 00402 time_t ctTime; 00403 ctTime = time(NULL); 00404 printf ("Start Secure Socket connection with one way server autentication test\n\r"); 00405 printf("Initial System Time is: %s\r\n", ctime(&ctTime)); 00406 printf("Need to adjust time? if yes enter time in seconds elapsed since Epoch (cmd: date +'%%s'), otherwise enter 0 "); 00407 int t=0; 00408 scanf("%d",&t); 00409 printf ("entered time is: %d \n\r", t); 00410 if (t != 0) { time_t txTm = t; set_time(txTm); } // set Nucleo system time 00411 ctTime = time(NULL); 00412 printf ("The current system time is: %s", ctime (&ctTime)); // set WiFi module systm time 00413 WiFiInterface* wifi = easy_get_wifi(0); 00414 if (!((SpwfSAInterface*)wifi)->set_time(ctTime)) printf ("ERROR set_time\n\r"); 00415 #endif 00416 //================= 00417 MQTTNetwork mqttNetwork(network); 00418 MQTT::Client<MQTTNetwork, Countdown, MQTT_MAX_PACKET_SIZE> client(mqttNetwork); 00419 00420 if (quickstartMode){ 00421 char mac[50]; // remove all : from mac 00422 char *digit=NULL; 00423 sprintf (id,"%s", ""); 00424 sprintf (mac,"%s",network->get_mac_address()); 00425 digit = strtok (mac,":"); 00426 while (digit != NULL) 00427 { 00428 strcat (id, digit); 00429 digit = strtok (NULL, ":"); 00430 } 00431 } 00432 printf ("ATTEMPT CONNECT\n\r"); 00433 attemptConnect(&client, &mqttNetwork, network); 00434 if (connack_rc == MQTT_NOT_AUTHORIZED || connack_rc == MQTT_BAD_USERNAME_OR_PASSWORD) 00435 { 00436 printf ("---ERROR line : %d\n\r", __LINE__); 00437 while (true) 00438 wait(1.0); // Permanent failures - don't retry 00439 } 00440 00441 #ifdef X_NUCLEO_NFC01A1_PRESENT 00442 NFCWriteRecordURI (nfcNucleo); 00443 #endif 00444 myled=1; 00445 int count = 0; 00446 while (true) 00447 { 00448 if (BlueButtonToggle == false && connected == true) { 00449 if (++count == 6) 00450 { 00451 // Publish a message every 3 second 00452 if (publish(&client) != MQTT::SUCCESS) { 00453 myled=0; 00454 count=0; 00455 client.disconnect(); 00456 mqttNetwork.disconnect(); 00457 attemptConnect(&client, &mqttNetwork, network); // if we have lost the connection 00458 } else { 00459 myled=1; 00460 count=0; 00461 } 00462 } 00463 if (client.isConnected()) client.yield(500); // allow the MQTT client to receive subscribe messages and manage keep alive 00464 } else if (BlueButtonToggle == true && connected == true){ // disconnect MQTT 00465 printf ("--->> MQTT Disconnect\n\r"); 00466 connected = false; 00467 myled=0; 00468 count = 0; 00469 BlueButtonToggle = false; 00470 #ifdef SUBSCRIBE 00471 // unsubscribe(const char* topicFilter); // unsubscribe if subscribed 00472 #endif 00473 client.disconnect(); 00474 printf ("--->> TCP Disconnect\n\r"); 00475 mqttNetwork.disconnect(); 00476 } else if (BlueButtonToggle == true && connected == false) { 00477 attemptConnect(&client, &mqttNetwork, network); 00478 connected = true; 00479 BlueButtonToggle = false; 00480 } else wait (0.5); 00481 } 00482 } 00483
Generated on Wed Jul 13 2022 18:42:12 by
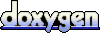