
X-NUCLEO-IKS01A1 Environmental/Motion sensors data transmitted via X-NUCLEO-IDB04A1 BLE board. Compatible with iOS/Android ST BlueMS V2.1 application.
Dependencies: BLE_API X_NUCLEO_IDB0XA1 X_NUCLEO_IKS01A1 mbed
Fork of Bluemicrosystem1 by
CustomConsoleService.h
00001 /** 00002 ****************************************************************************** 00003 * @file CustomConsoleService.h 00004 * @author Central Labs / AST 00005 * @version V0.9.0 00006 * @date 23-Dec-2015 00007 * @brief BLE console service 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 #ifndef __CUSTOM_BLE_CONSOLE_SERVICE_H__ 00039 #define __CUSTOM_BLE_CONSOLE_SERVICE_H__ 00040 00041 #include "BLE.h" 00042 00043 const LongUUIDBytes_t CONS_SERVICE_UUID_128 = { 0x00,0x00,0x00,0x00,0x00,0x0E,0x11,0xe1,0x9a,0xb4,0x00,0x02,0xa5,0xd5,0xc5,0x1b }; 00044 const LongUUIDBytes_t CONS_TERM_CHAR_UUID_128 = { 0x00,0x00,0x00,0x01,0x00,0x0E,0x11,0xe1,0xac,0x36,0x00,0x02,0xa5,0xd5,0xc5,0x1b }; 00045 const LongUUIDBytes_t CONS_STDERR_CHAR_UUID_128 = { 0x00,0x00,0x00,0x02,0x00,0x0E,0x11,0xe1,0xac,0x36,0x00,0x02,0xa5,0xd5,0xc5,0x1b }; 00046 00047 #define W2ST_CONSOLE_MAX_CHAR_LEN 20 00048 00049 /* Custom Sensors Service */ 00050 class CustomConsoleService { 00051 public: 00052 CustomConsoleService(BLE &_ble) : 00053 ble(_ble), 00054 consTermCharacteristic(CONS_TERM_CHAR_UUID_128,LastTermBuffer, W2ST_CONSOLE_MAX_CHAR_LEN, W2ST_CONSOLE_MAX_CHAR_LEN, GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE | 00055 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY), 00056 consStderrCharacteristic(CONS_STDERR_CHAR_UUID_128, LastStderrBuffer, W2ST_CONSOLE_MAX_CHAR_LEN, W2ST_CONSOLE_MAX_CHAR_LEN, 00057 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY) 00058 { 00059 00060 static bool serviceAdded = false; /* We should only ever need to add the env service once. */ 00061 if (serviceAdded) { 00062 return; 00063 } 00064 00065 GattCharacteristic *charTable[] = {&consTermCharacteristic, &consStderrCharacteristic}; 00066 00067 GattService consService(CONS_SERVICE_UUID_128, charTable, sizeof(charTable) / sizeof(GattCharacteristic *)); 00068 00069 ble.gattServer().addService(consService); 00070 00071 memset (LastTermBuffer, 0, W2ST_CONSOLE_MAX_CHAR_LEN); 00072 memset (LastStderrBuffer, 0, W2ST_CONSOLE_MAX_CHAR_LEN); 00073 00074 isBTLEConnected = DISCONNECTED; 00075 isEnabledTermNotify = false; 00076 isEnabledStderrNotify = false; 00077 serviceAdded = true; 00078 } 00079 00080 tBleStatus updateTerm (uint8_t *data,uint8_t length) { 00081 tBleStatus ret; 00082 uint8_t Offset; 00083 uint8_t DataToSend; 00084 // uint8_t BufferToWrite[W2ST_CONSOLE_MAX_CHAR_LEN]; 00085 // uint8_t BytesToWrite; 00086 00087 if (isEnabledTermNotify && isBTLEConnected==CONNECTED) { 00088 /* Split the code in packages */ 00089 for(Offset =0; Offset<length; Offset +=W2ST_CONSOLE_MAX_CHAR_LEN){ 00090 DataToSend = (length-Offset); 00091 DataToSend = (DataToSend>W2ST_CONSOLE_MAX_CHAR_LEN) ? W2ST_CONSOLE_MAX_CHAR_LEN : DataToSend; 00092 00093 /* keep a copy */ 00094 memcpy(LastTermBuffer,data+Offset,DataToSend); 00095 LastTermLen = DataToSend; 00096 PRINTF("updateTerm handle: %d string: %s\n\r", consTermCharacteristic.getValueAttribute().getHandle(), LastTermBuffer); 00097 ret = ble.gattServer().write(consTermCharacteristic.getValueAttribute().getHandle(), data+Offset, DataToSend, 0); 00098 if (ret != BLE_ERROR_NONE) { /* FIXME the wrong errcode from BLE requires BLE fix */ 00099 // BytesToWrite = sprintf((char *)BufferToWrite, "Error Updating Stdout Char\r\n"); 00100 // updateStderr(BufferToWrite,BytesToWrite); 00101 } 00102 return ret; 00103 } 00104 } 00105 return BLE_STATUS_SUCCESS; 00106 } 00107 00108 tBleStatus updateStderr (uint8_t *data,uint8_t length) { 00109 00110 uint8_t Offset; 00111 uint8_t DataToSend; 00112 00113 if (isEnabledStderrNotify && isBTLEConnected==CONNECTED) { 00114 /* Split the code in packages */ 00115 for(Offset =0; Offset<length; Offset +=W2ST_CONSOLE_MAX_CHAR_LEN){ 00116 DataToSend = (length-Offset); 00117 DataToSend = (DataToSend>W2ST_CONSOLE_MAX_CHAR_LEN) ? W2ST_CONSOLE_MAX_CHAR_LEN : DataToSend; 00118 00119 /* keep a copy */ 00120 memcpy(LastStderrBuffer,data+Offset,DataToSend); 00121 LastStderrLen = DataToSend; 00122 PRINTF("updateStderr handle: %d string: %s\n\r", consStderrCharacteristic.getValueAttribute().getHandle(), LastStderrBuffer); 00123 return (ble.gattServer().write(consStderrCharacteristic.getValueAttribute().getHandle(), data+Offset, DataToSend, 0)); 00124 } 00125 } 00126 return BLE_STATUS_SUCCESS; 00127 } 00128 00129 void updateConnectionStatus (ConnectionStatus_t status){ 00130 isBTLEConnected = status; 00131 isEnabledTermNotify = false; 00132 isEnabledStderrNotify = false; 00133 } 00134 00135 bool isTermHandle (Gap::Handle_t handle) { 00136 if (handle == consTermCharacteristic.getValueAttribute().getHandle() - BLE_HANDLE_VALUE_OFFSET) return true; 00137 return false; 00138 } 00139 00140 bool isStderrHandle (Gap::Handle_t handle) { 00141 if (handle == consStderrCharacteristic.getValueAttribute().getHandle() - BLE_HANDLE_VALUE_OFFSET) return true; 00142 return false; 00143 } 00144 00145 void enNotify (Gap::Handle_t handle) { 00146 if (isTermHandle(handle - BLE_HANDLE_EN_DIS_OFFSET)) { isEnabledTermNotify = true; return; } 00147 if (isStderrHandle(handle - BLE_HANDLE_EN_DIS_OFFSET)) { isEnabledStderrNotify = true; return; } 00148 } 00149 00150 void disNotify (Gap::Handle_t handle) { 00151 if (isTermHandle(handle - BLE_HANDLE_EN_DIS_OFFSET)) { isEnabledTermNotify = false; return; } 00152 if (isStderrHandle(handle - BLE_HANDLE_EN_DIS_OFFSET)) { isEnabledStderrNotify = false; return; } 00153 } 00154 00155 00156 private: 00157 BLE &ble; 00158 uint8_t LastTermBuffer [W2ST_CONSOLE_MAX_CHAR_LEN]; 00159 uint8_t LastTermLen; 00160 uint8_t LastStderrBuffer [W2ST_CONSOLE_MAX_CHAR_LEN]; 00161 uint8_t LastStderrLen; 00162 00163 GattCharacteristic consTermCharacteristic; 00164 GattCharacteristic consStderrCharacteristic; 00165 ConnectionStatus_t isBTLEConnected; 00166 bool isEnabledTermNotify; 00167 bool isEnabledStderrNotify; 00168 }; 00169 00170 #endif /* #ifndef __CUSTOM_BLE_CONSOLE_SERVICE_H__*/
Generated on Sun Jul 17 2022 23:51:22 by
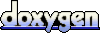