STM32L476G-Discovery board drivers V1.0.0
Dependents: DiscoLogger DISCO_L476VG_GlassLCD DISCO_L476VG_MicrophoneRecorder DISCO_L476VG_UART ... more
stm32l476g_discovery_gyroscope.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l476g_discovery_gyroscope.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of functions needed to manage the L3GD20 00006 * MEMS accelerometer available on STM32L476G-Discovery board. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© Copyright (c) 2016 STMicroelectronics. 00011 * All rights reserved.</center></h2> 00012 * 00013 * This software component is licensed by ST under BSD 3-Clause license, 00014 * the "License"; You may not use this file except in compliance with the 00015 * License. You may obtain a copy of the License at: 00016 * opensource.org/licenses/BSD-3-Clause 00017 * 00018 ****************************************************************************** 00019 */ 00020 00021 /* Includes ------------------------------------------------------------------*/ 00022 #include "stm32l476g_discovery_gyroscope.h" 00023 00024 /** @addtogroup BSP 00025 * @{ 00026 */ 00027 00028 /** @addtogroup STM32L476G_DISCOVERY 00029 * @{ 00030 */ 00031 00032 /** @defgroup STM32L476G_DISCOVERY_GYROSCOPE STM32L476G-DISCOVERY GYROSCOPE 00033 * @{ 00034 */ 00035 00036 /* Private typedef -----------------------------------------------------------*/ 00037 /** @defgroup STM32L476G_DISCOVERY_GYROSCOPE_Private_Types Private Types 00038 * @{ 00039 */ 00040 /** 00041 * @} 00042 */ 00043 00044 /* Private defines ------------------------------------------------------------*/ 00045 /** @defgroup STM32L476G_DISCOVERY_GYROSCOPE_Private_Constants Private Constants 00046 * @{ 00047 */ 00048 /** 00049 * @} 00050 */ 00051 00052 /* Private macros ------------------------------------------------------------*/ 00053 /** @defgroup STM32L476G_DISCOVERY_GYROSCOPE_Private_Macros Private Macros 00054 * @{ 00055 */ 00056 /** 00057 * @} 00058 */ 00059 00060 /* Private variables ---------------------------------------------------------*/ 00061 /** @defgroup STM32L476G_DISCOVERY_GYROSCOPE_Private_Variables Private Variables 00062 * @{ 00063 */ 00064 static GYRO_DrvTypeDef *GyroscopeDrv; 00065 00066 /** 00067 * @} 00068 */ 00069 00070 /* Private function prototypes -----------------------------------------------*/ 00071 /** @defgroup STM32L476G_DISCOVERY_GYROSCOPE_Private_FunctionPrototypes Private Functions 00072 * @{ 00073 */ 00074 /** 00075 * @} 00076 */ 00077 00078 /* Exported functions --------------------------------------------------------*/ 00079 /** @addtogroup STM32L476G_DISCOVERY_GYROSCOPE_Exported_Functions 00080 * @{ 00081 */ 00082 00083 /** 00084 * @brief Initialize Gyroscope. 00085 * @retval GYRO_OK or GYRO_ERROR 00086 */ 00087 uint8_t BSP_GYRO_Init(void) 00088 { 00089 uint8_t ret = GYRO_ERROR; 00090 uint16_t ctrl = 0x0000; 00091 GYRO_InitTypeDef L3GD20_InitStructure; 00092 GYRO_FilterConfigTypeDef L3GD20_FilterStructure = {0, 0}; 00093 00094 if ((L3gd20Drv.ReadID() == I_AM_L3GD20) || (L3gd20Drv.ReadID() == I_AM_L3GD20_TR)) 00095 { 00096 /* Initialize the gyroscope driver structure */ 00097 GyroscopeDrv = &L3gd20Drv; 00098 00099 /* Configure Mems : data rate, power mode, full scale and axes */ 00100 L3GD20_InitStructure.Power_Mode = L3GD20_MODE_ACTIVE; 00101 L3GD20_InitStructure.Output_DataRate = L3GD20_OUTPUT_DATARATE_1; 00102 L3GD20_InitStructure.Axes_Enable = L3GD20_AXES_ENABLE; 00103 L3GD20_InitStructure.Band_Width = L3GD20_BANDWIDTH_4; 00104 L3GD20_InitStructure.BlockData_Update = L3GD20_BlockDataUpdate_Continous; 00105 L3GD20_InitStructure.Endianness = L3GD20_BLE_LSB; 00106 L3GD20_InitStructure.Full_Scale = L3GD20_FULLSCALE_500; 00107 00108 /* Configure MEMS: data rate, power mode, full scale and axes */ 00109 ctrl = (uint16_t)(L3GD20_InitStructure.Power_Mode | L3GD20_InitStructure.Output_DataRate | \ 00110 L3GD20_InitStructure.Axes_Enable | L3GD20_InitStructure.Band_Width); 00111 00112 ctrl |= (uint16_t)((L3GD20_InitStructure.BlockData_Update | L3GD20_InitStructure.Endianness | \ 00113 L3GD20_InitStructure.Full_Scale) << 8); 00114 00115 /* Initialize component */ 00116 GyroscopeDrv->Init(ctrl); 00117 00118 L3GD20_FilterStructure.HighPassFilter_Mode_Selection = L3GD20_HPM_NORMAL_MODE_RES; 00119 L3GD20_FilterStructure.HighPassFilter_CutOff_Frequency = L3GD20_HPFCF_0; 00120 00121 ctrl = (uint8_t)((L3GD20_FilterStructure.HighPassFilter_Mode_Selection | \ 00122 L3GD20_FilterStructure.HighPassFilter_CutOff_Frequency)); 00123 00124 /* Configure component filter */ 00125 GyroscopeDrv->FilterConfig(ctrl) ; 00126 00127 /* Enable component filter */ 00128 GyroscopeDrv->FilterCmd(L3GD20_HIGHPASSFILTER_ENABLE); 00129 00130 ret = GYRO_OK; 00131 } 00132 else 00133 { 00134 ret = GYRO_ERROR; 00135 } 00136 00137 return ret; 00138 } 00139 00140 00141 /** 00142 * @brief DeInitialize Gyroscope. 00143 * @retval None 00144 */ 00145 void BSP_GYRO_DeInit(void) 00146 { 00147 GYRO_IO_DeInit(); 00148 } 00149 00150 00151 /** 00152 * @brief Put Gyroscope in low power mode. 00153 * @retval None 00154 */ 00155 void BSP_GYRO_LowPower(void) 00156 { 00157 uint16_t ctrl = 0x0000; 00158 GYRO_InitTypeDef L3GD20_InitStructure; 00159 00160 /* configure only Power_Mode field */ 00161 L3GD20_InitStructure.Power_Mode = L3GD20_MODE_POWERDOWN; 00162 00163 ctrl = (uint16_t)(L3GD20_InitStructure.Power_Mode); 00164 00165 /* Set component in low-power mode */ 00166 GyroscopeDrv->LowPower(ctrl); 00167 00168 00169 } 00170 00171 /** 00172 * @brief Read ID of Gyroscope component. 00173 * @retval ID 00174 */ 00175 uint8_t BSP_GYRO_ReadID(void) 00176 { 00177 uint8_t id = 0x00; 00178 00179 if (GyroscopeDrv->ReadID != NULL) 00180 { 00181 id = GyroscopeDrv->ReadID(); 00182 } 00183 return id; 00184 } 00185 00186 /** 00187 * @brief Reboot memory content of Gyroscope. 00188 * @retval None 00189 */ 00190 void BSP_GYRO_Reset(void) 00191 { 00192 if (GyroscopeDrv->Reset != NULL) 00193 { 00194 GyroscopeDrv->Reset(); 00195 } 00196 } 00197 00198 /** 00199 * @brief Configure Gyroscope interrupts (INT1 or INT2). 00200 * @param pIntConfig: pointer to a GYRO_InterruptConfigTypeDef 00201 * structure that contains the configuration setting for the L3GD20 Interrupt. 00202 * @retval None 00203 */ 00204 void BSP_GYRO_ITConfig(GYRO_InterruptConfigTypeDef *pIntConfig) 00205 { 00206 uint16_t interruptconfig = 0x0000; 00207 00208 if (GyroscopeDrv->ConfigIT != NULL) 00209 { 00210 /* Configure latch Interrupt request and axe interrupts */ 00211 interruptconfig |= ((uint8_t)(pIntConfig->Latch_Request | \ 00212 pIntConfig->Interrupt_Axes) << 8); 00213 00214 interruptconfig |= (uint8_t)(pIntConfig->Interrupt_ActiveEdge); 00215 00216 GyroscopeDrv->ConfigIT(interruptconfig); 00217 } 00218 } 00219 00220 /** 00221 * @brief Enable Gyroscope interrupts (INT1 or INT2). 00222 * @param IntPin: Interrupt pin 00223 * This parameter can be: 00224 * @arg L3GD20_INT1 00225 * @arg L3GD20_INT2 00226 * @retval None 00227 */ 00228 void BSP_GYRO_EnableIT(uint8_t IntPin) 00229 { 00230 if (GyroscopeDrv->EnableIT != NULL) 00231 { 00232 GyroscopeDrv->EnableIT(IntPin); 00233 } 00234 } 00235 00236 /** 00237 * @brief Disable Gyroscope interrupts (INT1 or INT2). 00238 * @param IntPin: Interrupt pin 00239 * This parameter can be: 00240 * @arg L3GD20_INT1 00241 * @arg L3GD20_INT2 00242 * @retval None 00243 */ 00244 void BSP_GYRO_DisableIT(uint8_t IntPin) 00245 { 00246 if (GyroscopeDrv->DisableIT != NULL) 00247 { 00248 GyroscopeDrv->DisableIT(IntPin); 00249 } 00250 } 00251 00252 /** 00253 * @brief Get XYZ angular acceleration from the Gyroscope. 00254 * @param pfData: pointer on floating array 00255 * @retval None 00256 */ 00257 void BSP_GYRO_GetXYZ(float *pfData) 00258 { 00259 if (GyroscopeDrv->GetXYZ != NULL) 00260 { 00261 GyroscopeDrv->GetXYZ(pfData); 00262 } 00263 } 00264 00265 /** 00266 * @} 00267 */ 00268 00269 /** 00270 * @} 00271 */ 00272 00273 /** 00274 * @} 00275 */ 00276 00277 /** 00278 * @} 00279 */ 00280 00281 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 18:37:21 by
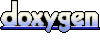