STM32Cube BSP FW for STM32F769I-Discovery
Dependents: mbed-os-example-blinky-5 DISCO-F769NI_TOUCHSCREEN_demo_custom_1 Datarecorder2 DISCO-F769NI_TOUCHSCREEN_demo ... more
stm32f769i_discovery_sd.c
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f769i_discovery_sd.c 00004 * @author MCD Application Team 00005 * @brief This file includes the uSD card driver mounted on STM32F769I-Discovery 00006 * board. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* File Info : ----------------------------------------------------------------- 00038 User NOTES 00039 1. How To use this driver: 00040 -------------------------- 00041 - This driver is used to drive the micro SD external card mounted on STM32F769I-Discovery 00042 board. 00043 - This driver does not need a specific component driver for the micro SD device 00044 to be included with. 00045 00046 2. Driver description: 00047 --------------------- 00048 + Initialization steps: 00049 o Initialize the micro SD card using the BSP_SD_Init() function. This 00050 function includes the MSP layer hardware resources initialization and the 00051 SDIO interface configuration to interface with the external micro SD. It 00052 also includes the micro SD initialization sequence. 00053 o To check the SD card presence you can use the function BSP_SD_IsDetected() which 00054 returns the detection status 00055 o If SD presence detection interrupt mode is desired, you must configure the 00056 SD detection interrupt mode by calling the function BSP_SD_ITConfig(). The interrupt 00057 is generated as an external interrupt whenever the micro SD card is 00058 plugged/unplugged in/from the discovery board. 00059 o The function BSP_SD_GetCardInfo() is used to get the micro SD card information 00060 which is stored in the structure "HAL_SD_CardInfoTypedef". 00061 00062 + Micro SD card operations 00063 o The micro SD card can be accessed with read/write block(s) operations once 00064 it is reay for access. The access cand be performed whether using the polling 00065 mode by calling the functions BSP_SD_ReadBlocks()/BSP_SD_WriteBlocks(), or by DMA 00066 transfer using the functions BSP_SD_ReadBlocks_DMA()/BSP_SD_WriteBlocks_DMA() 00067 o The DMA transfer complete is used with interrupt mode. Once the SD transfer 00068 is complete, the SD interrupt is handeled using the function BSP_SD_IRQHandler(), 00069 the DMA Tx/Rx transfer complete are handeled using the functions 00070 BSP_SD_DMA_Tx_IRQHandler()/BSP_SD_DMA_Rx_IRQHandler(). The corresponding user callbacks 00071 are implemented by the user at application level. 00072 o The SD erase block(s) is performed using the function BSP_SD_Erase() with specifying 00073 the number of blocks to erase. 00074 o The SD runtime status is returned when calling the function BSP_SD_GetCardState(). 00075 00076 ------------------------------------------------------------------------------*/ 00077 00078 /* Dependencies 00079 - stm32f7xx_hal_sd.c 00080 - stm32f7xx_ll_sdmmc.c 00081 - stm32f7xx_hal_dma.c 00082 - stm32f7xx_hal_gpio.c 00083 - stm32f7xx_hal_cortex.c 00084 - stm32f7xx_hal_rcc_ex.h 00085 EndDependencies */ 00086 00087 /* Includes ------------------------------------------------------------------*/ 00088 #include "stm32f769i_discovery_sd.h" 00089 00090 /** @addtogroup BSP 00091 * @{ 00092 */ 00093 00094 /** @addtogroup STM32F769I_DISCOVERY 00095 * @{ 00096 */ 00097 00098 /** @defgroup STM32F769I_DISCOVERY_SD STM32F769I_DISCOVERY SD 00099 * @{ 00100 */ 00101 00102 00103 /** @defgroup STM32F769I_DISCOVERY_SD_Private_TypesDefinitions STM32F769I Discovery Sd Private TypesDef 00104 * @{ 00105 */ 00106 /** 00107 * @} 00108 */ 00109 00110 /** @defgroup STM32F769I_DISCOVERY_SD_Private_Defines STM32F769I Discovery Sd Private Defines 00111 * @{ 00112 */ 00113 /** 00114 * @} 00115 */ 00116 00117 /** @defgroup STM32F769I_DISCOVERY_SD_Private_Macros STM32F769I Discovery Sd Private Macro 00118 * @{ 00119 */ 00120 /** 00121 * @} 00122 */ 00123 00124 /** @defgroup STM32F769I_DISCOVERY_SD_Private_Variables STM32F769I Discovery Sd Private Variables 00125 * @{ 00126 */ 00127 SD_HandleTypeDef uSdHandle; 00128 00129 /** 00130 * @} 00131 */ 00132 00133 /** @defgroup STM32F769I_DISCOVERY_SD_Private_FunctionPrototypes STM32F769I Discovery Sd Private Prototypes 00134 * @{ 00135 */ 00136 /** 00137 * @} 00138 */ 00139 00140 /** @defgroup STM32F769I_DISCOVERY_SD_Private_Functions STM32F769I Discovery Sd Private Functions 00141 * @{ 00142 */ 00143 00144 /** 00145 * @brief Initializes the SD card device. 00146 * @retval SD status 00147 */ 00148 uint8_t BSP_SD_Init(void) 00149 { 00150 uint8_t sd_state = MSD_OK; 00151 00152 /* PLLSAI is dedicated to LCD periph. Do not use it to get 48MHz*/ 00153 00154 /* uSD device interface configuration */ 00155 uSdHandle.Instance = SDMMC2; 00156 uSdHandle.Init.ClockEdge = SDMMC_CLOCK_EDGE_RISING; 00157 uSdHandle.Init.ClockBypass = SDMMC_CLOCK_BYPASS_DISABLE; 00158 uSdHandle.Init.ClockPowerSave = SDMMC_CLOCK_POWER_SAVE_DISABLE; 00159 uSdHandle.Init.BusWide = SDMMC_BUS_WIDE_1B; 00160 uSdHandle.Init.HardwareFlowControl = SDMMC_HARDWARE_FLOW_CONTROL_DISABLE; 00161 uSdHandle.Init.ClockDiv = SDMMC_TRANSFER_CLK_DIV; 00162 00163 /* Msp SD Detect pin initialization */ 00164 BSP_SD_Detect_MspInit(&uSdHandle, NULL); 00165 if(BSP_SD_IsDetected() != SD_PRESENT) /* Check if SD card is present */ 00166 { 00167 return MSD_ERROR_SD_NOT_PRESENT; 00168 } 00169 00170 /* Msp SD initialization */ 00171 BSP_SD_MspInit(&uSdHandle, NULL); 00172 00173 /* HAL SD initialization */ 00174 if(HAL_SD_Init(&uSdHandle) != HAL_OK) 00175 { 00176 sd_state = MSD_ERROR; 00177 } 00178 00179 /* Configure SD Bus width */ 00180 if(sd_state == MSD_OK) 00181 { 00182 /* Enable wide operation */ 00183 if(HAL_SD_ConfigWideBusOperation(&uSdHandle, SDMMC_BUS_WIDE_4B) != HAL_OK) 00184 { 00185 sd_state = MSD_ERROR; 00186 } 00187 else 00188 { 00189 sd_state = MSD_OK; 00190 } 00191 } 00192 return sd_state; 00193 } 00194 00195 /** 00196 * @brief DeInitializes the SD card device. 00197 * @retval SD status 00198 */ 00199 uint8_t BSP_SD_DeInit(void) 00200 { 00201 uint8_t sd_state = MSD_OK; 00202 00203 uSdHandle.Instance = SDMMC2; 00204 00205 /* HAL SD deinitialization */ 00206 if(HAL_SD_DeInit(&uSdHandle) != HAL_OK) 00207 { 00208 sd_state = MSD_ERROR; 00209 } 00210 00211 /* Msp SD deinitialization */ 00212 uSdHandle.Instance = SDMMC2; 00213 BSP_SD_MspDeInit(&uSdHandle, NULL); 00214 00215 return sd_state; 00216 } 00217 00218 /** 00219 * @brief Configures Interrupt mode for SD detection pin. 00220 * @retval Returns 0 00221 */ 00222 uint8_t BSP_SD_ITConfig(void) 00223 { 00224 GPIO_InitTypeDef gpio_init_structure; 00225 00226 /* Configure Interrupt mode for SD detection pin */ 00227 gpio_init_structure.Pin = SD_DETECT_PIN; 00228 gpio_init_structure.Pull = GPIO_PULLUP; 00229 gpio_init_structure.Speed = GPIO_SPEED_FAST; 00230 gpio_init_structure.Mode = GPIO_MODE_IT_RISING_FALLING; 00231 HAL_GPIO_Init(SD_DETECT_GPIO_PORT, &gpio_init_structure); 00232 00233 /* Enable and set SD detect EXTI Interrupt to the lowest priority */ 00234 HAL_NVIC_SetPriority((IRQn_Type)(SD_DETECT_EXTI_IRQn), 0x0F, 0x00); 00235 HAL_NVIC_EnableIRQ((IRQn_Type)(SD_DETECT_EXTI_IRQn)); 00236 00237 return MSD_OK; 00238 } 00239 00240 /** 00241 * @brief Detects if SD card is correctly plugged in the memory slot or not. 00242 * @retval Returns if SD is detected or not 00243 */ 00244 uint8_t BSP_SD_IsDetected(void) 00245 { 00246 __IO uint8_t status = SD_PRESENT; 00247 00248 /* Check SD card detect pin */ 00249 if (HAL_GPIO_ReadPin(SD_DETECT_GPIO_PORT, SD_DETECT_PIN) == GPIO_PIN_SET) 00250 { 00251 status = SD_NOT_PRESENT; 00252 } 00253 00254 return status; 00255 } 00256 00257 /** 00258 * @brief Reads block(s) from a specified address in an SD card, in polling mode. 00259 * @param pData: Pointer to the buffer that will contain the data to transmit 00260 * @param ReadAddr: Address from where data is to be read 00261 * @param NumOfBlocks: Number of SD blocks to read 00262 * @param Timeout: Timeout for read operation 00263 * @retval SD status 00264 */ 00265 uint8_t BSP_SD_ReadBlocks(uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks, uint32_t Timeout) 00266 { 00267 if(HAL_SD_ReadBlocks(&uSdHandle, (uint8_t *)pData, ReadAddr, NumOfBlocks, Timeout) != HAL_OK) 00268 { 00269 return MSD_ERROR; 00270 } 00271 else 00272 { 00273 return MSD_OK; 00274 } 00275 } 00276 00277 /** 00278 * @brief Writes block(s) to a specified address in an SD card, in polling mode. 00279 * @param pData: Pointer to the buffer that will contain the data to transmit 00280 * @param WriteAddr: Address from where data is to be written 00281 * @param NumOfBlocks: Number of SD blocks to write 00282 * @param Timeout: Timeout for write operation 00283 * @retval SD status 00284 */ 00285 uint8_t BSP_SD_WriteBlocks(uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks, uint32_t Timeout) 00286 { 00287 if(HAL_SD_WriteBlocks(&uSdHandle, (uint8_t *)pData, WriteAddr, NumOfBlocks, Timeout) != HAL_OK) 00288 { 00289 return MSD_ERROR; 00290 } 00291 else 00292 { 00293 return MSD_OK; 00294 } 00295 } 00296 00297 /** 00298 * @brief Reads block(s) from a specified address in an SD card, in DMA mode. 00299 * @param pData: Pointer to the buffer that will contain the data to transmit 00300 * @param ReadAddr: Address from where data is to be read 00301 * @param NumOfBlocks: Number of SD blocks to read 00302 * @retval SD status 00303 */ 00304 uint8_t BSP_SD_ReadBlocks_DMA(uint32_t *pData, uint32_t ReadAddr, uint32_t NumOfBlocks) 00305 { 00306 /* Read block(s) in DMA transfer mode */ 00307 if(HAL_SD_ReadBlocks_DMA(&uSdHandle, (uint8_t *)pData, ReadAddr, NumOfBlocks) != HAL_OK) 00308 { 00309 return MSD_ERROR; 00310 } 00311 else 00312 { 00313 return MSD_OK; 00314 } 00315 } 00316 00317 /** 00318 * @brief Writes block(s) to a specified address in an SD card, in DMA mode. 00319 * @param pData: Pointer to the buffer that will contain the data to transmit 00320 * @param WriteAddr: Address from where data is to be written 00321 * @param NumOfBlocks: Number of SD blocks to write 00322 * @retval SD status 00323 */ 00324 uint8_t BSP_SD_WriteBlocks_DMA(uint32_t *pData, uint32_t WriteAddr, uint32_t NumOfBlocks) 00325 { 00326 /* Write block(s) in DMA transfer mode */ 00327 if(HAL_SD_WriteBlocks_DMA(&uSdHandle, (uint8_t *)pData, WriteAddr, NumOfBlocks) != HAL_OK) 00328 { 00329 return MSD_ERROR; 00330 } 00331 else 00332 { 00333 return MSD_OK; 00334 } 00335 } 00336 00337 /** 00338 * @brief Erases the specified memory area of the given SD card. 00339 * @param StartAddr: Start byte address 00340 * @param EndAddr: End byte address 00341 * @retval SD status 00342 */ 00343 uint8_t BSP_SD_Erase(uint32_t StartAddr, uint32_t EndAddr) 00344 { 00345 if(HAL_SD_Erase(&uSdHandle, StartAddr, EndAddr) != HAL_OK) 00346 { 00347 return MSD_ERROR; 00348 } 00349 else 00350 { 00351 return MSD_OK; 00352 } 00353 } 00354 00355 /** 00356 * @brief Initializes the SD MSP. 00357 * @param hsd: SD handle 00358 * @param Params : pointer on additional configuration parameters, can be NULL. 00359 */ 00360 __weak void BSP_SD_MspInit(SD_HandleTypeDef *hsd, void *Params) 00361 { 00362 static DMA_HandleTypeDef dma_rx_handle; 00363 static DMA_HandleTypeDef dma_tx_handle; 00364 GPIO_InitTypeDef gpio_init_structure; 00365 00366 /* Enable SDMMC2 clock */ 00367 __HAL_RCC_SDMMC2_CLK_ENABLE(); 00368 00369 /* Enable DMA2 clocks */ 00370 __DMAx_TxRx_CLK_ENABLE(); 00371 00372 /* Enable GPIOs clock */ 00373 __HAL_RCC_GPIOB_CLK_ENABLE(); 00374 __HAL_RCC_GPIOD_CLK_ENABLE(); 00375 __HAL_RCC_GPIOG_CLK_ENABLE(); 00376 00377 /* Common GPIO configuration */ 00378 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00379 gpio_init_structure.Pull = GPIO_PULLUP; 00380 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00381 00382 /* GPIOB configuration */ 00383 gpio_init_structure.Alternate = GPIO_AF10_SDMMC2; 00384 gpio_init_structure.Pin = GPIO_PIN_3 | GPIO_PIN_4; 00385 HAL_GPIO_Init(GPIOB, &gpio_init_structure); 00386 00387 /* GPIOD configuration */ 00388 gpio_init_structure.Alternate = GPIO_AF11_SDMMC2; 00389 gpio_init_structure.Pin = GPIO_PIN_6 | GPIO_PIN_7; 00390 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00391 00392 /* GPIOG configuration */ 00393 gpio_init_structure.Pin = GPIO_PIN_9 | GPIO_PIN_10; 00394 HAL_GPIO_Init(GPIOG, &gpio_init_structure); 00395 00396 /* NVIC configuration for SDMMC2 interrupts */ 00397 HAL_NVIC_SetPriority(SDMMC2_IRQn, 0x0E, 0); 00398 HAL_NVIC_EnableIRQ(SDMMC2_IRQn); 00399 00400 /* Configure DMA Rx parameters */ 00401 dma_rx_handle.Init.Channel = SD_DMAx_Rx_CHANNEL; 00402 dma_rx_handle.Init.Direction = DMA_PERIPH_TO_MEMORY; 00403 dma_rx_handle.Init.PeriphInc = DMA_PINC_DISABLE; 00404 dma_rx_handle.Init.MemInc = DMA_MINC_ENABLE; 00405 dma_rx_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00406 dma_rx_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00407 dma_rx_handle.Init.Mode = DMA_PFCTRL; 00408 dma_rx_handle.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00409 dma_rx_handle.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00410 dma_rx_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00411 dma_rx_handle.Init.MemBurst = DMA_MBURST_INC4; 00412 dma_rx_handle.Init.PeriphBurst = DMA_PBURST_INC4; 00413 00414 dma_rx_handle.Instance = SD_DMAx_Rx_STREAM; 00415 00416 /* Associate the DMA handle */ 00417 __HAL_LINKDMA(hsd, hdmarx, dma_rx_handle); 00418 00419 /* Deinitialize the stream for new transfer */ 00420 HAL_DMA_DeInit(&dma_rx_handle); 00421 00422 /* Configure the DMA stream */ 00423 HAL_DMA_Init(&dma_rx_handle); 00424 00425 /* Configure DMA Tx parameters */ 00426 dma_tx_handle.Init.Channel = SD_DMAx_Tx_CHANNEL; 00427 dma_tx_handle.Init.Direction = DMA_MEMORY_TO_PERIPH; 00428 dma_tx_handle.Init.PeriphInc = DMA_PINC_DISABLE; 00429 dma_tx_handle.Init.MemInc = DMA_MINC_ENABLE; 00430 dma_tx_handle.Init.PeriphDataAlignment = DMA_PDATAALIGN_WORD; 00431 dma_tx_handle.Init.MemDataAlignment = DMA_MDATAALIGN_WORD; 00432 dma_tx_handle.Init.Mode = DMA_PFCTRL; 00433 dma_tx_handle.Init.Priority = DMA_PRIORITY_VERY_HIGH; 00434 dma_tx_handle.Init.FIFOMode = DMA_FIFOMODE_ENABLE; 00435 dma_tx_handle.Init.FIFOThreshold = DMA_FIFO_THRESHOLD_FULL; 00436 dma_tx_handle.Init.MemBurst = DMA_MBURST_INC4; 00437 dma_tx_handle.Init.PeriphBurst = DMA_PBURST_INC4; 00438 00439 dma_tx_handle.Instance = SD_DMAx_Tx_STREAM; 00440 00441 /* Associate the DMA handle */ 00442 __HAL_LINKDMA(hsd, hdmatx, dma_tx_handle); 00443 00444 /* Deinitialize the stream for new transfer */ 00445 HAL_DMA_DeInit(&dma_tx_handle); 00446 00447 /* Configure the DMA stream */ 00448 HAL_DMA_Init(&dma_tx_handle); 00449 00450 /* NVIC configuration for DMA transfer complete interrupt */ 00451 HAL_NVIC_SetPriority(SD_DMAx_Rx_IRQn, 0x0F, 0); 00452 HAL_NVIC_EnableIRQ(SD_DMAx_Rx_IRQn); 00453 00454 /* NVIC configuration for DMA transfer complete interrupt */ 00455 HAL_NVIC_SetPriority(SD_DMAx_Tx_IRQn, 0x0F, 0); 00456 HAL_NVIC_EnableIRQ(SD_DMAx_Tx_IRQn); 00457 } 00458 00459 /** 00460 * @brief Initializes the SD Detect pin MSP. 00461 * @param hsd: SD handle 00462 * @param Params : pointer on additional configuration parameters, can be NULL. 00463 * @retval None 00464 */ 00465 __weak void BSP_SD_Detect_MspInit(SD_HandleTypeDef *hsd, void *Params) 00466 { 00467 GPIO_InitTypeDef gpio_init_structure; 00468 00469 SD_DETECT_GPIO_CLK_ENABLE(); 00470 00471 /* GPIO configuration in input for uSD_Detect signal */ 00472 gpio_init_structure.Pin = SD_DETECT_PIN; 00473 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00474 gpio_init_structure.Pull = GPIO_PULLUP; 00475 gpio_init_structure.Speed = GPIO_SPEED_HIGH; 00476 HAL_GPIO_Init(SD_DETECT_GPIO_PORT, &gpio_init_structure); 00477 } 00478 00479 /** 00480 * @brief DeInitializes the SD MSP. 00481 * @param hsd: SD handle 00482 * @param Params : pointer on additional configuration parameters, can be NULL. 00483 */ 00484 __weak void BSP_SD_MspDeInit(SD_HandleTypeDef *hsd, void *Params) 00485 { 00486 static DMA_HandleTypeDef dma_rx_handle; 00487 static DMA_HandleTypeDef dma_tx_handle; 00488 00489 /* Disable NVIC for DMA transfer complete interrupts */ 00490 HAL_NVIC_DisableIRQ(SD_DMAx_Rx_IRQn); 00491 HAL_NVIC_DisableIRQ(SD_DMAx_Tx_IRQn); 00492 00493 /* Deinitialize the stream for new transfer */ 00494 dma_rx_handle.Instance = SD_DMAx_Rx_STREAM; 00495 HAL_DMA_DeInit(&dma_rx_handle); 00496 00497 /* Deinitialize the stream for new transfer */ 00498 dma_tx_handle.Instance = SD_DMAx_Tx_STREAM; 00499 HAL_DMA_DeInit(&dma_tx_handle); 00500 00501 /* Disable NVIC for SDIO interrupts */ 00502 HAL_NVIC_DisableIRQ(SDIO_IRQn); 00503 00504 /* DeInit GPIO pins can be done in the application 00505 (by surcharging this __weak function) */ 00506 00507 /* Disable SDIO clock */ 00508 __HAL_RCC_SDIO_CLK_DISABLE(); 00509 00510 /* GPOI pins clock and DMA cloks can be shut down in the applic 00511 by surcgarging this __weak function */ 00512 } 00513 00514 /** 00515 * @brief Gets the current SD card data status. 00516 * @retval Data transfer state. 00517 * This value can be one of the following values: 00518 * @arg SD_TRANSFER_OK: No data transfer is acting 00519 * @arg SD_TRANSFER_BUSY: Data transfer is acting 00520 */ 00521 uint8_t BSP_SD_GetCardState(void) 00522 { 00523 return((HAL_SD_GetCardState(&uSdHandle) == HAL_SD_CARD_TRANSFER ) ? SD_TRANSFER_OK : SD_TRANSFER_BUSY); 00524 } 00525 00526 00527 /** 00528 * @brief Get SD information about specific SD card. 00529 * @param CardInfo: Pointer to HAL_SD_CardInfoTypedef structure 00530 * @retval None 00531 */ 00532 void BSP_SD_GetCardInfo(HAL_SD_CardInfoTypeDef *CardInfo) 00533 { 00534 /* Get SD card Information */ 00535 HAL_SD_GetCardInfo(&uSdHandle, CardInfo); 00536 } 00537 00538 /** 00539 * @brief SD Abort callbacks 00540 * @param hsd: SD handle 00541 * @retval None 00542 */ 00543 void HAL_SD_AbortCallback(SD_HandleTypeDef *hsd) 00544 { 00545 BSP_SD_AbortCallback(); 00546 } 00547 00548 /** 00549 * @brief Tx Transfer completed callbacks 00550 * @param hsd: SD handle 00551 * @retval None 00552 */ 00553 void HAL_SD_TxCpltCallback(SD_HandleTypeDef *hsd) 00554 { 00555 BSP_SD_WriteCpltCallback(); 00556 } 00557 00558 /** 00559 * @brief Rx Transfer completed callbacks 00560 * @param hsd: SD handle 00561 * @retval None 00562 */ 00563 void HAL_SD_RxCpltCallback(SD_HandleTypeDef *hsd) 00564 { 00565 BSP_SD_ReadCpltCallback(); 00566 } 00567 00568 /** 00569 * @brief BSP SD Abort callbacks 00570 * @retval None 00571 */ 00572 __weak void BSP_SD_AbortCallback(void) 00573 { 00574 00575 } 00576 00577 /** 00578 * @brief BSP Tx Transfer completed callbacks 00579 * @retval None 00580 */ 00581 __weak void BSP_SD_WriteCpltCallback(void) 00582 { 00583 00584 } 00585 00586 /** 00587 * @brief BSP Rx Transfer completed callbacks 00588 * @retval None 00589 */ 00590 __weak void BSP_SD_ReadCpltCallback(void) 00591 { 00592 00593 } 00594 00595 /** 00596 * @} 00597 */ 00598 00599 /** 00600 * @} 00601 */ 00602 00603 /** 00604 * @} 00605 */ 00606 00607 /** 00608 * @} 00609 */ 00610 00611 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 17:55:04 by
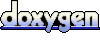