STM32Cube BSP FW for STM32F769I-Discovery
Dependents: mbed-os-example-blinky-5 DISCO-F769NI_TOUCHSCREEN_demo_custom_1 Datarecorder2 DISCO-F769NI_TOUCHSCREEN_demo ... more
mx25l512.h
00001 /** 00002 ****************************************************************************** 00003 * @file mx25l512.h 00004 * @author MCD Application Team 00005 * @brief This file contains all the description of the MX25L51245G QSPI memory. 00006 ****************************************************************************** 00007 * @attention 00008 * 00009 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00010 * 00011 * Redistribution and use in source and binary forms, with or without modification, 00012 * are permitted provided that the following conditions are met: 00013 * 1. Redistributions of source code must retain the above copyright notice, 00014 * this list of conditions and the following disclaimer. 00015 * 2. Redistributions in binary form must reproduce the above copyright notice, 00016 * this list of conditions and the following disclaimer in the documentation 00017 * and/or other materials provided with the distribution. 00018 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00019 * may be used to endorse or promote products derived from this software 00020 * without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00023 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00024 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00025 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00026 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00027 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00028 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00029 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00030 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00031 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00032 * 00033 ****************************************************************************** 00034 */ 00035 00036 /* Define to prevent recursive inclusion -------------------------------------*/ 00037 #ifndef __MX25L512_H 00038 #define __MX25L512_H 00039 00040 #ifdef __cplusplus 00041 extern "C" { 00042 #endif 00043 00044 /* Includes ------------------------------------------------------------------*/ 00045 00046 /** @addtogroup BSP 00047 * @{ 00048 */ 00049 00050 /** @addtogroup Components 00051 * @{ 00052 */ 00053 00054 /** @addtogroup MX25L512 00055 * @{ 00056 */ 00057 00058 /** @defgroup MX25L512_Exported_Types 00059 * @{ 00060 */ 00061 00062 /** 00063 * @} 00064 */ 00065 00066 /** @defgroup MX25L512_Exported_Constants 00067 * @{ 00068 */ 00069 00070 /** 00071 * @brief MX25L512 Configuration 00072 */ 00073 #define MX25L512_FLASH_SIZE 0x4000000 /* 512 MBits => 64MBytes */ 00074 #define MX25L512_SECTOR_SIZE 0x10000 /* 1024 sectors of 64KBytes */ 00075 #define MX25L512_SUBSECTOR_SIZE 0x1000 /* 16384 subsectors of 4kBytes */ 00076 #define MX25L512_PAGE_SIZE 0x100 /* 262144 pages of 256 bytes */ 00077 00078 #define MX25L512_DUMMY_CYCLES_READ_QUAD 3 00079 #define MX25L512_DUMMY_CYCLES_READ 8 00080 #define MX25L512_DUMMY_CYCLES_READ_QUAD_IO 10 00081 #define MX25L512_DUMMY_CYCLES_READ_DTR 6 00082 #define MX25L512_DUMMY_CYCLES_READ_QUAD_DTR 8 00083 00084 #define MX25L512_BULK_ERASE_MAX_TIME 600000 00085 #define MX25L512_SECTOR_ERASE_MAX_TIME 2000 00086 #define MX25L512_SUBSECTOR_ERASE_MAX_TIME 800 00087 00088 /** 00089 * @brief MX25L512 Commands 00090 */ 00091 /* Reset Operations */ 00092 #define RESET_ENABLE_CMD 0x66 00093 #define RESET_MEMORY_CMD 0x99 00094 00095 /* Identification Operations */ 00096 #define READ_ID_CMD 0x9F 00097 #define MULTIPLE_IO_READ_ID_CMD 0xAF 00098 #define READ_SERIAL_FLASH_DISCO_PARAM_CMD 0x5A 00099 00100 /* Read Operations */ 00101 #define READ_CMD 0x03 00102 #define READ_4_BYTE_ADDR_CMD 0x13 00103 00104 #define FAST_READ_CMD 0x0B 00105 #define FAST_READ_DTR_CMD 0x0D 00106 #define FAST_READ_4_BYTE_ADDR_CMD 0x0C 00107 00108 #define DUAL_OUT_FAST_READ_CMD 0x3B 00109 #define DUAL_OUT_FAST_READ_4_BYTE_ADDR_CMD 0x3C 00110 00111 #define DUAL_INOUT_FAST_READ_CMD 0xBB 00112 #define DUAL_INOUT_FAST_READ_DTR_CMD 0xBD 00113 #define DUAL_INOUT_FAST_READ_4_BYTE_ADDR_CMD 0xBC 00114 00115 #define QUAD_OUT_FAST_READ_CMD 0x6B 00116 #define QUAD_OUT_FAST_READ_4_BYTE_ADDR_CMD 0x6C 00117 00118 #define QUAD_INOUT_FAST_READ_CMD 0xEB 00119 #define QUAD_INOUT_FAST_READ_DTR_CMD 0xED 00120 #define QSPI_READ_4_BYTE_ADDR_CMD 0xEC 00121 00122 /* Write Operations */ 00123 #define WRITE_ENABLE_CMD 0x06 00124 #define WRITE_DISABLE_CMD 0x04 00125 00126 /* Register Operations */ 00127 #define READ_STATUS_REG_CMD 0x05 00128 #define READ_CFG_REG_CMD 0x15 00129 #define WRITE_STATUS_CFG_REG_CMD 0x01 00130 00131 #define READ_LOCK_REG_CMD 0x2D 00132 #define WRITE_LOCK_REG_CMD 0x2C 00133 00134 #define READ_EXT_ADDR_REG_CMD 0xC8 00135 #define WRITE_EXT_ADDR_REG_CMD 0xC5 00136 00137 /* Program Operations */ 00138 #define PAGE_PROG_CMD 0x02 00139 #define QSPI_PAGE_PROG_4_BYTE_ADDR_CMD 0x12 00140 00141 #define QUAD_IN_FAST_PROG_CMD 0x38 00142 #define EXT_QUAD_IN_FAST_PROG_CMD 0x38 00143 #define QUAD_IN_FAST_PROG_4_BYTE_ADDR_CMD 0x3E 00144 00145 /* Erase Operations */ 00146 #define SUBSECTOR_ERASE_CMD 0x20 00147 #define SUBSECTOR_ERASE_4_BYTE_ADDR_CMD 0x21 00148 00149 #define SECTOR_ERASE_CMD 0xD8 00150 #define SECTOR_ERASE_4_BYTE_ADDR_CMD 0xDC 00151 00152 #define BULK_ERASE_CMD 0xC7 00153 00154 #define PROG_ERASE_RESUME_CMD 0x30 00155 #define PROG_ERASE_SUSPEND_CMD 0xB0 00156 00157 /* 4-byte Address Mode Operations */ 00158 #define ENTER_4_BYTE_ADDR_MODE_CMD 0xB7 00159 #define EXIT_4_BYTE_ADDR_MODE_CMD 0xE9 00160 00161 /* Quad Operations */ 00162 #define ENTER_QUAD_CMD 0x35 00163 #define EXIT_QUAD_CMD 0xF5 00164 00165 /* Added for compatibility */ 00166 #define QPI_READ_4_BYTE_ADDR_CMD QSPI_READ_4_BYTE_ADDR_CMD 00167 #define QPI_PAGE_PROG_4_BYTE_ADDR_CMD QSPI_PAGE_PROG_4_BYTE_ADDR_CMD 00168 00169 /** 00170 * @brief MX25L512 Registers 00171 */ 00172 /* Status Register */ 00173 #define MX25L512_SR_WIP ((uint8_t)0x01) /*!< Write in progress */ 00174 #define MX25L512_SR_WREN ((uint8_t)0x02) /*!< Write enable latch */ 00175 #define MX25L512_SR_BLOCKPR ((uint8_t)0x5C) /*!< Block protected against program and erase operations */ 00176 #define MX25L512_SR_PRBOTTOM ((uint8_t)0x20) /*!< Protected memory area defined by BLOCKPR starts from top or bottom */ 00177 #define MX25L512_SR_QUADEN ((uint8_t)0x40) /*!< Quad IO mode enabled if =1 */ 00178 #define MX25L512_SR_SRWREN ((uint8_t)0x80) /*!< Status register write enable/disable */ 00179 00180 /* Configuration Register */ 00181 #define MX25L512_CR_ODS ((uint8_t)0x07) /*!< Output driver strength */ 00182 #define MX25L512_CR_ODS_30 ((uint8_t)0x07) /*!< Output driver strength 30 ohms (default)*/ 00183 #define MX25L512_CR_ODS_15 ((uint8_t)0x06) /*!< Output driver strength 15 ohms */ 00184 #define MX25L512_CR_ODS_20 ((uint8_t)0x05) /*!< Output driver strength 20 ohms */ 00185 #define MX25L512_CR_ODS_45 ((uint8_t)0x03) /*!< Output driver strength 45 ohms */ 00186 #define MX25L512_CR_ODS_60 ((uint8_t)0x02) /*!< Output driver strength 60 ohms */ 00187 #define MX25L512_CR_ODS_90 ((uint8_t)0x01) /*!< Output driver strength 90 ohms */ 00188 #define MX25L512_CR_TB ((uint8_t)0x08) /*!< Top/Bottom bit used to configure the block protect area */ 00189 #define MX25L512_CR_PBE ((uint8_t)0x10) /*!< Preamble Bit Enable */ 00190 #define MX25L512_CR_4BYTE ((uint8_t)0x20) /*!< 3-bytes or 4-bytes addressing */ 00191 #define MX25L512_CR_NB_DUMMY ((uint8_t)0xC0) /*!< Number of dummy clock cycles */ 00192 00193 #define MX25L512_MANUFACTURER_ID ((uint8_t)0xC2) 00194 #define MX25L512_DEVICE_ID_MEM_TYPE ((uint8_t)0x20) 00195 #define MX25L512_DEVICE_ID_MEM_CAPACITY ((uint8_t)0x1A) 00196 #define MX25L512_UNIQUE_ID_DATA_LENGTH ((uint8_t)0x10) 00197 00198 /** 00199 * @} 00200 */ 00201 00202 /** @defgroup MX25L512_Exported_Functions 00203 * @{ 00204 */ 00205 /** 00206 * @} 00207 */ 00208 00209 #ifdef __cplusplus 00210 } 00211 #endif 00212 00213 #endif /* __MX25L512_H */ 00214 00215 /** 00216 * @} 00217 */ 00218 00219 /** 00220 * @} 00221 */ 00222 00223 /** 00224 * @} 00225 */ 00226 00227 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 17:55:04 by
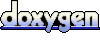