STM32Cube BSP FW for STM32F769I-Discovery
Dependents: mbed-os-example-blinky-5 DISCO-F769NI_TOUCHSCREEN_demo_custom_1 Datarecorder2 DISCO-F769NI_TOUCHSCREEN_demo ... more
adv7533.h
00001 00002 /** 00003 ****************************************************************************** 00004 * @file adv7533.h 00005 * @author MCD Application Team 00006 * @brief This file contains all the constants parameters for the ADV7533 00007 * which is the HDMI bridge between DSI and HDMI 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __ADV7533_H 00040 #define __ADV7533_H 00041 00042 /* Includes ------------------------------------------------------------------*/ 00043 #include "../Common/audio.h" 00044 00045 /** @addtogroup BSP 00046 * @{ 00047 */ 00048 00049 /** @addtogroup Components 00050 * @{ 00051 */ 00052 00053 /** @addtogroup adv7533 00054 * @{ 00055 */ 00056 00057 /** @addtogroup ADV7533_Exported_Types 00058 * @{ 00059 */ 00060 00061 typedef struct { 00062 uint8_t DSI_LANES; 00063 uint16_t HACT; 00064 uint16_t HSYNC; 00065 uint16_t HBP; 00066 uint16_t HFP; 00067 uint16_t VACT; 00068 uint16_t VSYNC; 00069 uint16_t VBP; 00070 uint16_t VFP; 00071 uint8_t ASPECT_RATIO; 00072 } adv7533ConfigTypeDef; 00073 00074 /** @defgroup ADV7533_Exported_Constants 00075 * @{ 00076 */ 00077 00078 /** 00079 * @brief HDMI audio output DEVICE 00080 */ 00081 #define OUTPUT_DEVICE_ADV7533_HDMI ((uint16_t)0x1000) 00082 00083 /** 00084 * @brief ADV7533 I2C Addresses 0x7A / 0x78 00085 */ 00086 #define ADV7533_MAIN_I2C_ADDR ((uint8_t)0x7A) 00087 #define ADV7533_CEC_DSI_I2C_ADDR ((uint8_t)0x78) 00088 00089 /** 00090 * @brief ADV7533 Aspect ratio 00091 */ 00092 #define ADV7533_ASPECT_RATIO_16_9 ((uint8_t)0x00) 00093 #define ADV7533_ASPECT_RATIO_4_3 ((uint8_t)0x01) 00094 00095 /** 00096 * @brief ADV7533 Aspect ratio 00097 */ 00098 #define ADV7533_MODE_HDMI 0x0 00099 #define ADV7533_MODE_DVI 0x1 00100 00101 /** 00102 * @brief ADV7533 Main Registers 00103 */ 00104 #define ADV7533_MAIN_SYNC_REG ((uint8_t)0x17) 00105 #define ADV7533_MAIN_POWER_DOWN_REG ((uint8_t)0x41) 00106 #define ADV7533_MAIN_HPG_REG ((uint8_t)0x42) 00107 00108 /** 00109 * @brief ADV7533 Main Features Parameters 00110 */ 00111 00112 /** 00113 * @brief ADV7533 CEC DSI Registers 00114 */ 00115 #define ADV7533_CEC_DSI_INTERNAL_TIMING_REG ((uint8_t)0x27) 00116 #define ADV7533_CEC_DSI_TOTAL_WIDTH_H_REG ((uint8_t)0x28) 00117 #define ADV7533_CEC_DSI_TOTAL_WIDTH_L_REG ((uint8_t)0x29) 00118 #define ADV7533_CEC_DSI_HSYNC_H_REG ((uint8_t)0x2A) 00119 #define ADV7533_CEC_DSI_HSYNC_L_REG ((uint8_t)0x2B) 00120 #define ADV7533_CEC_DSI_HFP_H_REG ((uint8_t)0x2C) 00121 #define ADV7533_CEC_DSI_HFP_L_REG ((uint8_t)0x2D) 00122 #define ADV7533_CEC_DSI_HBP_H_REG ((uint8_t)0x2E) 00123 #define ADV7533_CEC_DSI_HBP_L_REG ((uint8_t)0x2F) 00124 00125 #define ADV7533_CEC_DSI_TOTAL_HEIGHT_H_REG ((uint8_t)0x30) 00126 #define ADV7533_CEC_DSI_TOTAL_HEIGHT_L_REG ((uint8_t)0x31) 00127 #define ADV7533_CEC_DSI_VSYNC_H_REG ((uint8_t)0x32) 00128 #define ADV7533_CEC_DSI_VSYNC_L_REG ((uint8_t)0x33) 00129 #define ADV7533_CEC_DSI_VFP_H_REG ((uint8_t)0x34) 00130 #define ADV7533_CEC_DSI_VFP_L_REG ((uint8_t)0x35) 00131 #define ADV7533_CEC_DSI_VBP_H_REG ((uint8_t)0x36) 00132 #define ADV7533_CEC_DSI_VBP_L_REG ((uint8_t)0x37) 00133 00134 /** @Brief adv7533 ID 00135 */ 00136 #define ADV7533_ID 0x7533 00137 00138 /** @Brief device ID register 00139 */ 00140 #define ADV7533_CHIPID_ADDR0 0x00 00141 #define ADV7533_CHIPID_ADDR1 0x01 00142 00143 /* MUTE commands */ 00144 #define AUDIO_MUTE_ON 1 00145 #define AUDIO_MUTE_OFF 0 00146 00147 /* AUDIO FREQUENCY */ 00148 #define AUDIO_FREQUENCY_192K ((uint32_t)192000) 00149 #define AUDIO_FREQUENCY_176K ((uint32_t)176400) 00150 #define AUDIO_FREQUENCY_96K ((uint32_t)96000) 00151 #define AUDIO_FREQUENCY_88K ((uint32_t)88200) 00152 #define AUDIO_FREQUENCY_48K ((uint32_t)48000) 00153 #define AUDIO_FREQUENCY_44K ((uint32_t)44100) 00154 #define AUDIO_FREQUENCY_32K ((uint32_t)32000) 00155 00156 /** 00157 * @} 00158 */ 00159 00160 /* Exported macro ------------------------------------------------------------*/ 00161 00162 /** @defgroup ADV7533_Exported_Macros ADV7533 Exported Macros 00163 * @{ 00164 */ 00165 00166 /** 00167 * @} 00168 */ 00169 00170 /* Exported functions --------------------------------------------------------*/ 00171 00172 /** @addtogroup ADV7533_Exported_Functions 00173 * @{ 00174 */ 00175 00176 /*------------------------------------------------------------------------------ 00177 HDMI video functions 00178 ------------------------------------------------------------------------------*/ 00179 uint8_t ADV7533_Init(void); 00180 void ADV7533_PowerOn(void); 00181 void ADV7533_PowerDown(void); 00182 void ADV7533_Configure(adv7533ConfigTypeDef * config); 00183 void ADV7533_PatternEnable(void); 00184 void ADV7533_PatternDisable(void); 00185 00186 /*------------------------------------------------------------------------------ 00187 HDMI Audio functions 00188 ------------------------------------------------------------------------------*/ 00189 uint32_t adv7533_AudioInit(uint16_t DeviceAddr, uint16_t OutputDevice, uint8_t Volume,uint32_t AudioFreq); 00190 void adv7533_DeInit(void); 00191 uint32_t adv7533_ReadID(uint16_t DeviceAddr); 00192 uint32_t adv7533_Play(uint16_t DeviceAddr, uint16_t* pBuffer, uint16_t Size); 00193 uint32_t adv7533_Stop(uint16_t DeviceAddr,uint32_t Cmd); 00194 uint32_t adv7533_Pause(uint16_t DeviceAddr); 00195 uint32_t adv7533_Resume(uint16_t DeviceAddr); 00196 uint32_t adv7533_SetMute(uint16_t DeviceAddr, uint32_t Cmd); 00197 uint32_t adv7533_SetVolume(uint16_t, uint8_t); 00198 uint32_t adv7533_Reset(uint16_t DeviceAddr); 00199 uint32_t adv7533_SetOutputMode(uint16_t DeviceAddr, uint8_t Output); 00200 uint32_t adv7533_SetFrequency(uint16_t DeviceAddr, uint32_t AudioFreq); 00201 00202 00203 /* HDMI IO functions */ 00204 void HDMI_IO_Init(void); 00205 void HDMI_IO_Write(uint8_t addr, uint8_t reg, uint8_t value); 00206 uint8_t HDMI_IO_Read(uint8_t addr, uint8_t reg); 00207 void HDMI_IO_Delay(uint32_t delay); 00208 void AUDIO_IO_DeInit(void); 00209 00210 /** 00211 * @} 00212 */ 00213 00214 /* HDMI Audio driver structure */ 00215 extern AUDIO_DrvTypeDef adv7533_drv; 00216 00217 #endif /* __ADV7533_H */ 00218 /** 00219 * @} 00220 */ 00221 00222 /** 00223 * @} 00224 */ 00225 00226 /** 00227 * @} 00228 */ 00229 00230 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 17:55:03 by
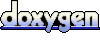