STM32F429ZI Discovery board drivers
Dependents: 2a 2b 2c 2d1 ... more
stm32f429i_discovery_lcd.h
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f429i_discovery_lcd.h 00004 * @author MCD Application Team 00005 * @brief This file contains all the functions prototypes for the 00006 * stm32f429i_discovery_lcd.c driver. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Define to prevent recursive inclusion -------------------------------------*/ 00038 #ifndef __STM32F429I_DISCOVERY_LCD_H 00039 #define __STM32F429I_DISCOVERY_LCD_H 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 /* Includes ------------------------------------------------------------------*/ 00046 #include "stm32f429i_discovery.h" 00047 /* Include SDRAM Driver */ 00048 #include "stm32f429i_discovery_sdram.h" 00049 #include "../Fonts/fonts.h" 00050 /* Include LCD component driver */ 00051 #include "../Components/ili9341/ili9341.h" 00052 00053 /** @addtogroup BSP 00054 * @{ 00055 */ 00056 00057 /** @addtogroup STM32F429I_DISCOVERY 00058 * @{ 00059 */ 00060 00061 /** @addtogroup STM32F429I_DISCOVERY_LCD 00062 * @{ 00063 */ 00064 00065 /** @defgroup STM32F429I_DISCOVERY_LCD_Exported_Types STM32F429I DISCOVERY LCD Exported Types 00066 * @{ 00067 */ 00068 typedef enum 00069 { 00070 LCD_OK = 0, 00071 LCD_ERROR = 1, 00072 LCD_TIMEOUT = 2 00073 }LCD_StatusTypeDef; 00074 00075 typedef struct 00076 { 00077 uint32_t TextColor; 00078 uint32_t BackColor; 00079 sFONT *pFont; 00080 }LCD_DrawPropTypeDef; 00081 00082 typedef struct 00083 { 00084 int16_t X; 00085 int16_t Y; 00086 } Point, * pPoint; 00087 00088 /** 00089 * @brief Line mode structures definition 00090 */ 00091 typedef enum 00092 { 00093 CENTER_MODE = 0x01, /* center mode */ 00094 RIGHT_MODE = 0x02, /* right mode */ 00095 LEFT_MODE = 0x03, /* left mode */ 00096 }Text_AlignModeTypdef; 00097 /** 00098 * @} 00099 */ 00100 00101 /** @defgroup STM32F429I_DISCOVERY_LCD_Exported_Constants STM32F429I DISCOVERY LCD Exported Constants 00102 * @{ 00103 */ 00104 #define LCD_LayerCfgTypeDef LTDC_LayerCfgTypeDef 00105 00106 /** 00107 * @brief LCD status structure definition 00108 */ 00109 #define MAX_LAYER_NUMBER 2 00110 #define LCD_FRAME_BUFFER ((uint32_t)0xD0000000) 00111 #define BUFFER_OFFSET ((uint32_t)0x50000) 00112 00113 /** 00114 * @brief LCD color 00115 */ 00116 #define LCD_COLOR_BLUE 0xFF0000FF 00117 #define LCD_COLOR_GREEN 0xFF00FF00 00118 #define LCD_COLOR_RED 0xFFFF0000 00119 #define LCD_COLOR_CYAN 0xFF00FFFF 00120 #define LCD_COLOR_MAGENTA 0xFFFF00FF 00121 #define LCD_COLOR_YELLOW 0xFFFFFF00 00122 #define LCD_COLOR_LIGHTBLUE 0xFF8080FF 00123 #define LCD_COLOR_LIGHTGREEN 0xFF80FF80 00124 #define LCD_COLOR_LIGHTRED 0xFFFF8080 00125 #define LCD_COLOR_LIGHTCYAN 0xFF80FFFF 00126 #define LCD_COLOR_LIGHTMAGENTA 0xFFFF80FF 00127 #define LCD_COLOR_LIGHTYELLOW 0xFFFFFF80 00128 #define LCD_COLOR_DARKBLUE 0xFF000080 00129 #define LCD_COLOR_DARKGREEN 0xFF008000 00130 #define LCD_COLOR_DARKRED 0xFF800000 00131 #define LCD_COLOR_DARKCYAN 0xFF008080 00132 #define LCD_COLOR_DARKMAGENTA 0xFF800080 00133 #define LCD_COLOR_DARKYELLOW 0xFF808000 00134 #define LCD_COLOR_WHITE 0xFFFFFFFF 00135 #define LCD_COLOR_LIGHTGRAY 0xFFD3D3D3 00136 #define LCD_COLOR_GRAY 0xFF808080 00137 #define LCD_COLOR_DARKGRAY 0xFF404040 00138 #define LCD_COLOR_BLACK 0xFF000000 00139 #define LCD_COLOR_BROWN 0xFFA52A2A 00140 #define LCD_COLOR_ORANGE 0xFFFFA500 00141 #define LCD_COLOR_TRANSPARENT 0xFF000000 00142 /** 00143 * @brief LCD default font 00144 */ 00145 #define LCD_DEFAULT_FONT Font24 00146 00147 /** 00148 * @brief LCD Reload Types 00149 */ 00150 #define LCD_RELOAD_IMMEDIATE ((uint32_t)LTDC_SRCR_IMR) 00151 #define LCD_RELOAD_VERTICAL_BLANKING ((uint32_t)LTDC_SRCR_VBR) 00152 00153 /** 00154 * @brief LCD Layer 00155 */ 00156 #define LCD_BACKGROUND_LAYER 0x0000 00157 #define LCD_FOREGROUND_LAYER 0x0001 00158 00159 /** 00160 * @} 00161 */ 00162 00163 /** @defgroup STM32F429I_DISCOVERY_LCD_Exported_Macros STM32F429I DISCOVERY LCD Exported Macros 00164 * @{ 00165 */ 00166 /** 00167 * @brief LCD Pixel format 00168 */ 00169 #define LCD_PIXEL_FORMAT_ARGB8888 LTDC_PIXEL_FORMAT_ARGB8888 00170 #define LCD_PIXEL_FORMAT_RGB888 LTDC_PIXEL_FORMAT_RGB888 00171 #define LCD_PIXEL_FORMAT_RGB565 LTDC_PIXEL_FORMAT_RGB565 00172 #define LCD_PIXEL_FORMAT_ARGB1555 LTDC_PIXEL_FORMAT_ARGB1555 00173 #define LCD_PIXEL_FORMAT_ARGB4444 LTDC_PIXEL_FORMAT_ARGB4444 00174 #define LCD_PIXEL_FORMAT_L8 LTDC_PIXEL_FORMAT_L8 00175 #define LCD_PIXEL_FORMAT_AL44 LTDC_PIXEL_FORMAT_AL44 00176 #define LCD_PIXEL_FORMAT_AL88 LTDC_PIXEL_FORMAT_AL88 00177 /** 00178 * @} 00179 */ 00180 00181 /** @defgroup STM32F429I_DISCOVERY_LCD_Exported_Functions STM32F429I DISCOVERY LCD Exported Functions 00182 * @{ 00183 */ 00184 uint8_t BSP_LCD_Init(void); 00185 uint32_t BSP_LCD_GetXSize(void); 00186 uint32_t BSP_LCD_GetYSize(void); 00187 00188 /* functions using the LTDC controller */ 00189 void BSP_LCD_LayerDefaultInit(uint16_t LayerIndex, uint32_t FrameBuffer); 00190 void BSP_LCD_SetTransparency(uint32_t LayerIndex, uint8_t Transparency); 00191 void BSP_LCD_SetTransparency_NoReload(uint32_t LayerIndex, uint8_t Transparency); 00192 void BSP_LCD_SetLayerAddress(uint32_t LayerIndex, uint32_t Address); 00193 void BSP_LCD_SetLayerAddress_NoReload(uint32_t LayerIndex, uint32_t Address); 00194 void BSP_LCD_SetColorKeying(uint32_t LayerIndex, uint32_t RGBValue); 00195 void BSP_LCD_SetColorKeying_NoReload(uint32_t LayerIndex, uint32_t RGBValue); 00196 void BSP_LCD_ResetColorKeying(uint32_t LayerIndex); 00197 void BSP_LCD_ResetColorKeying_NoReload(uint32_t LayerIndex); 00198 void BSP_LCD_SetLayerWindow(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00199 void BSP_LCD_SetLayerWindow_NoReload(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00200 void BSP_LCD_SelectLayer(uint32_t LayerIndex); 00201 void BSP_LCD_SetLayerVisible(uint32_t LayerIndex, FunctionalState state); 00202 void BSP_LCD_SetLayerVisible_NoReload(uint32_t LayerIndex, FunctionalState State); 00203 void BSP_LCD_Relaod(uint32_t ReloadType); 00204 00205 void BSP_LCD_SetTextColor(uint32_t Color); 00206 void BSP_LCD_SetBackColor(uint32_t Color); 00207 uint32_t BSP_LCD_GetTextColor(void); 00208 uint32_t BSP_LCD_GetBackColor(void); 00209 void BSP_LCD_SetFont(sFONT *pFonts); 00210 sFONT *BSP_LCD_GetFont(void); 00211 00212 uint32_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos); 00213 void BSP_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint32_t pixel); 00214 void BSP_LCD_Clear(uint32_t Color); 00215 void BSP_LCD_ClearStringLine(uint32_t Line); 00216 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr); 00217 void BSP_LCD_DisplayStringAt(uint16_t X, uint16_t Y, uint8_t *pText, Text_AlignModeTypdef mode); 00218 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii); 00219 00220 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00221 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00222 void BSP_LCD_DrawLine(uint16_t X1, uint16_t Y1, uint16_t X2, uint16_t Y2); 00223 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00224 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00225 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount); 00226 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00227 void BSP_LCD_DrawBitmap(uint32_t X, uint32_t Y, uint8_t *pBmp); 00228 00229 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00230 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00231 void BSP_LCD_FillTriangle(uint16_t X1, uint16_t X2, uint16_t X3, uint16_t Y1, uint16_t Y2, uint16_t Y3); 00232 void BSP_LCD_FillPolygon(pPoint Points, uint16_t PointCount); 00233 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00234 00235 void BSP_LCD_DisplayOff(void); 00236 void BSP_LCD_DisplayOn(void); 00237 00238 /* This function can be modified in case the current settings need to be changed 00239 for specific application needs */ 00240 void BSP_LCD_MspInit(void); 00241 00242 /** 00243 * @} 00244 */ 00245 00246 /** 00247 * @} 00248 */ 00249 00250 /** 00251 * @} 00252 */ 00253 00254 /** 00255 * @} 00256 */ 00257 00258 #ifdef __cplusplus 00259 } 00260 #endif 00261 00262 #endif /* __STM32F429I_DISCOVERY_LCD_H */ 00263 00264 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 16:29:23 by
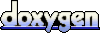