Updates to follow mbed SDK coding style guidelines.
Dependencies: ST_INTERFACES X_NUCLEO_COMMON
Dependents: 53L0A1_Satellites_with_Interrupts_OS5 Display_53L0A1_OS5
Fork of X_NUCLEO_53L0A1 by
Display_class.h
00001 /** 00002 ****************************************************************************** 00003 * @file Display.h 00004 * @author AST / EST 00005 * @version V0.0.1 00006 * @date 14-April-2015 00007 * @brief Header file for display 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 #ifndef __DISPLAY_H 00039 #define __DISPLAY_H 00040 00041 /* Includes ------------------------------------------------------------------*/ 00042 #include "mbed.h" 00043 #include "stmpe1600_class.h" 00044 #include "DevI2C.h" 00045 00046 #ifdef __cplusplus 00047 extern "C" { 00048 #endif 00049 00050 /** 00051 * GPIO monitor pin state register 00052 * 16 bit register LSB at lowest offset (little endian) 00053 */ 00054 #define GPMR 0x10 00055 /** 00056 * STMPE1600 GPIO set pin state register 00057 * 16 bit register LSB at lowest offset (little endian) 00058 */ 00059 #define GPSR 0x12 00060 /** 00061 * STMPE1600 GPIO set pin direction register 00062 * 16 bit register LSB at lowest offset 00063 */ 00064 #define GPDR 0x14 00065 00066 00067 /** 00068 * cache the full set of expanded GPIO values to avoid i2c reading 00069 */ 00070 static union CurIOVal_u { 00071 uint8_t bytes [4]; /*!< 4 bytes array i/o view */ 00072 uint32_t u32 ; /*!< single dword i/o view */ 00073 } 00074 /** cache the extended IO values */ 00075 cur_io_val; 00076 00077 /** 00078 * lookup table for digit to bit position 00079 */ 00080 static int display_bit_posn[4] = {0, 7, 16, 16 + 7}; 00081 00082 /** 00083 * @defgroup XNUCLEO53L0A1_7Segment 7 segment display 00084 * 00085 * macro use for human readable segment building 00086 * @code 00087 * --s0-- 00088 * s s 00089 * 5 1 00090 * --s6-- 00091 * s s 00092 * 4 2 00093 * --s3-- . s7 (dp) 00094 * @endcode 00095 * 00096 * @{ 00097 */ 00098 /** decimal point bit mapping* */ 00099 #define DP (1 << 7) 00100 00101 /** sgement s0 bit mapping*/ 00102 #define S0 (1 << 3) 00103 /** sgement s1 bit mapping*/ 00104 #define S1 (1 << 5) 00105 /** sgement s2 bit mapping*/ 00106 #define S2 (1 << 6) 00107 /** sgement s3 bit mapping*/ 00108 #define S3 (1 << 4) 00109 /** sgement s4 bit mapping*/ 00110 #define S4 (1 << 0) 00111 /** sgement s5 bit mapping*/ 00112 #define S5 (1 << 1) 00113 /** sgement s6 bit mapping*/ 00114 #define S6 (1 << 2) 00115 00116 /** 00117 * build a character by defining the non lighted segment (not one and no DP) 00118 * 00119 * @param ... literal sum and or combine of any macro to define any segment #S0 .. #S6 00120 * 00121 * example '9' is all segment on but S4 00122 * @code 00123 * ['9']= NOT_7_NO_DP(S4), 00124 * @endcode 00125 */ 00126 #define NOT_7_NO_DP( ... ) (uint8_t) ~( __VA_ARGS__ + DP ) 00127 00128 /** 00129 * Ascii to 7 segment lookup table 00130 * 00131 * Most common character are supported and follow http://www.twyman.org.uk/Fonts/ 00132 * few extra special \@ ^~ ... etc are present for specific demo purpose 00133 */ 00134 #ifndef __cpluplus 00135 /* refer to http://www.twyman.org.uk/Fonts/ */ 00136 static const uint8_t ascii_to_display_lut[256] = { 00137 0, 0, 0, 0, 0, 0, 0, 0, 00138 0, 0, 0, 0, 0, 0, 0, 0, 00139 0, 0, 0, 0, 0, 0, 0, 0, 00140 0, 0, 0, 0, 0, 0, 0, 0, 00141 [32] = 0, 00142 0, 0, 0, 0, 0, 0, 0, 0, 00143 0, 00144 [42] = NOT_7_NO_DP(), 00145 0, 0, 00146 [45] = S6, 00147 0, 0, 00148 [48] = NOT_7_NO_DP(S6), 00149 [49] = S1 + S2, 00150 [50] = S0 + S1 + S6 + S4 + S3, 00151 [51] = NOT_7_NO_DP(S4 + S5), 00152 [52] = S5 + S1 + S6 + S2, 00153 [53] = NOT_7_NO_DP(S1 + S4), 00154 [54] = NOT_7_NO_DP(S1), 00155 [55] = S0 + S1 + S2, 00156 [56] = NOT_7_NO_DP(0), 00157 [57] = NOT_7_NO_DP(S4), 00158 0, 0, 0, 00159 [61] = S3 + S6, 00160 0, 00161 [63] = NOT_7_NO_DP(S5 + S3 + S2), 00162 [64] = S0 + S3, 00163 [65] = NOT_7_NO_DP(S3), 00164 [66] = NOT_7_NO_DP(S0 + S1), /* as b */ 00165 [67] = S0 + S3 + S4 + S5, // same as [ 00166 [68] = S0 + S3 + S4 + S5, // same as [ DUMMY 00167 [69] = NOT_7_NO_DP(S1 + S2), 00168 [70] = S6 + S5 + S4 + S0, 00169 [71] = NOT_7_NO_DP(S4), /* same as 9 */ 00170 [72] = NOT_7_NO_DP(S0 + S3), 00171 [73] = S1 + S2, 00172 [74] = S1 + S2 + S3 + S4, 00173 [75] = NOT_7_NO_DP(S0 + S3), /* same as H */ 00174 [76] = S3 + S4 + S5, 00175 [77] = S0 + S4 + S2, /* same as m*/ 00176 [78] = S2 + S4 + S6, /* same as n*/ 00177 [79] = NOT_7_NO_DP(S6), 00178 [80] = S0 + S1 + S2 + S5 + S6, // sames as 'q' 00179 [81] = NOT_7_NO_DP(S3 + S2), 00180 [82] = S4 + S6, 00181 [83] = NOT_7_NO_DP(S1 + S4), /* sasme as 5 */ 00182 [84] = NOT_7_NO_DP(S0 + S1 + S2), /* sasme as t */ 00183 [85] = NOT_7_NO_DP(S6 + S0), 00184 [86] = S4 + S3 + S2, // is u but u use U 00185 [87] = S1 + S3 + S5, 00186 [88] = NOT_7_NO_DP(S0 + S3), // similar to H 00187 [89] = NOT_7_NO_DP(S0 + S4), 00188 [90] = S0 + S1 + S6 + S4 + S3, // same as 2 00189 [91] = S0 + S3 + S4 + S5, 00190 0, 00191 [93] = S0 + S3 + S2 + S1, 00192 [94] = S0, /* use as top bar */ 00193 [95] = S3, 00194 0, 00195 [97] = S2 + S3 + S4 + S6, 00196 [98] = NOT_7_NO_DP(S0 + S1), 00197 [99] = S6 + S4 + S3, 00198 [100] = NOT_7_NO_DP(S0 + S5), 00199 [101] = NOT_7_NO_DP(S2), 00200 [102] = S6 + S5 + S4 + S0, /* same as F */ 00201 [103] = NOT_7_NO_DP(S4), /* same as 9 */ 00202 [104] = S6 + S5 + S4 + S2, 00203 [105] = S4, 00204 [106] = S1 + S2 + S3 + S4, 00205 [107] = S6 + S5 + S4 + S2, /* a h */ 00206 [108] = S3 + S4, 00207 [109] = S0 + S4 + S2, /* same as */ 00208 [110] = S2 + S4 + S6, 00209 [111] = S6 + S4 + S3 + S2, 00210 [112] = NOT_7_NO_DP(S3 + S2), // same as P 00211 [113] = S0 + S1 + S2 + S5 + S6, 00212 [114] = S4 + S6, 00213 [115] = NOT_7_NO_DP(S1 + S4), 00214 [116] = NOT_7_NO_DP(S0 + S1 + S2), 00215 [117] = S4 + S3 + S2 + S5 + S1, // U 00216 [118] = S4 + S3 + S2, // is u but u use U 00217 [119] = S1 + S3 + S5, 00218 [120] = NOT_7_NO_DP(S0 + S3), // similar to H 00219 [121] = NOT_7_NO_DP(S0 + S4), 00220 [122] = S0 + S1 + S6 + S4 + S3, // same as 2 00221 0, 0, 0, 00222 [126] = S0 + S3 + S6 /* 3 h bar */ 00223 }; 00224 #else 00225 /* refer to http://www.twyman.org.uk/Fonts/ */ 00226 static const uint8_t ascii_to_display_lut[256] = { 00227 [' '] = 0, 00228 ['-'] = S6, 00229 ['_'] = S3, 00230 ['='] = S3 + S6, 00231 ['~'] = S0 + S3 + S6, /* 3 h bar */ 00232 ['^'] = S0, /* use as top bar */ 00233 00234 ['?'] = NOT_7_NO_DP(S5 + S3 + S2), 00235 ['*'] = NOT_7_NO_DP(), 00236 ['['] = S0 + S3 + S4 + S5, 00237 [']'] = S0 + S3 + S2 + S1, 00238 ['@'] = S0 + S3, 00239 00240 ['0'] = NOT_7_NO_DP(S6), 00241 ['1'] = S1 + S2, 00242 ['2'] = S0 + S1 + S6 + S4 + S3, 00243 ['3'] = NOT_7_NO_DP(S4 + S5), 00244 ['4'] = S5 + S1 + S6 + S2, 00245 ['5'] = NOT_7_NO_DP(S1 + S4), 00246 ['6'] = NOT_7_NO_DP(S1), 00247 ['7'] = S0 + S1 + S2, 00248 ['8'] = NOT_7_NO_DP(0), 00249 ['9'] = NOT_7_NO_DP(S4), 00250 00251 ['a'] = S2 + S3 + S4 + S6, 00252 ['b'] = NOT_7_NO_DP(S0 + S1), 00253 ['c'] = S6 + S4 + S3, 00254 ['d'] = NOT_7_NO_DP(S0 + S5), 00255 ['e'] = NOT_7_NO_DP(S2), 00256 ['f'] = S6 + S5 + S4 + S0, /* same as F */ 00257 ['g'] = NOT_7_NO_DP(S4), /* same as 9 */ 00258 ['h'] = S6 + S5 + S4 + S2, 00259 ['i'] = S4, 00260 ['j'] = S1 + S2 + S3 + S4, 00261 ['k'] = S6 + S5 + S4 + S2, /* a h */ 00262 ['l'] = S3 + S4, 00263 ['m'] = S0 + S4 + S2, /* same as */ 00264 ['n'] = S2 + S4 + S6, 00265 ['o'] = S6 + S4 + S3 + S2, 00266 ['p'] = NOT_7_NO_DP(S3 + S2), // same as P 00267 ['q'] = S0 + S1 + S2 + S5 + S6, 00268 ['r'] = S4 + S6, 00269 ['s'] = NOT_7_NO_DP(S1 + S4), 00270 ['t'] = NOT_7_NO_DP(S0 + S1 + S2), 00271 ['u'] = S4 + S3 + S2 + S5 + S1, // U 00272 ['v'] = S4 + S3 + S2, // is u but u use U 00273 ['w'] = S1 + S3 + S5, 00274 ['x'] = NOT_7_NO_DP(S0 + S3), // similar to H 00275 ['y'] = NOT_7_NO_DP(S0 + S4), 00276 ['z'] = S0 + S1 + S6 + S4 + S3, // same as 2 00277 00278 ['A'] = NOT_7_NO_DP(S3), 00279 ['B'] = NOT_7_NO_DP(S0 + S1), /* as b */ 00280 ['C'] = S0 + S3 + S4 + S5, // same as [ 00281 ['E'] = NOT_7_NO_DP(S1 + S2), 00282 ['F'] = S6 + S5 + S4 + S0, 00283 ['G'] = NOT_7_NO_DP(S4), /* same as 9 */ 00284 ['H'] = NOT_7_NO_DP(S0 + S3), 00285 ['I'] = S1 + S2, 00286 ['J'] = S1 + S2 + S3 + S4, 00287 ['K'] = NOT_7_NO_DP(S0 + S3), /* same as H */ 00288 ['L'] = S3 + S4 + S5, 00289 ['M'] = S0 + S4 + S2, /* same as m*/ 00290 ['N'] = S2 + S4 + S6, /* same as n*/ 00291 ['O'] = NOT_7_NO_DP(S6), 00292 ['P'] = S0 + S1 + S2 + S5 + S6, // sames as 'q' 00293 ['Q'] = NOT_7_NO_DP(S3 + S2), 00294 ['R'] = S4 + S6, 00295 ['S'] = NOT_7_NO_DP(S1 + S4), /* sasme as 5 */ 00296 ['T'] = NOT_7_NO_DP(S0 + S1 + S2), /* sasme as t */ 00297 ['U'] = NOT_7_NO_DP(S6 + S0), 00298 ['V'] = S4 + S3 + S2, // is u but u use U 00299 ['W'] = S1 + S3 + S5, 00300 ['X'] = NOT_7_NO_DP(S0 + S3), // similar to H 00301 ['Y'] = NOT_7_NO_DP(S0 + S4), 00302 ['Z'] = S0 + S1 + S6 + S4 + S3 // same as 2 00303 }; 00304 #endif 00305 00306 00307 #undef S0 00308 #undef S1 00309 #undef S2 00310 #undef S3 00311 #undef S4 00312 #undef S5 00313 #undef S6 00314 #undef DP 00315 00316 /** @} */ 00317 00318 //#define DISPLAY_DELAY 1 // in mSec 00319 00320 /* Classes -------------------------------------------------------------------*/ 00321 /** Class representing Display 00322 */ 00323 00324 class Display 00325 { 00326 private: 00327 Stmpe1600 &stmpe1600_exp0; 00328 Stmpe1600 &stmpe1600_exp1; 00329 public: 00330 /** Constructor 00331 * @param[in] &stmpe_1600 device handler to be used for display control 00332 */ 00333 Display(Stmpe1600 &stmpe_1600_exp0, Stmpe1600 &stmpe_1600_exp1) : stmpe1600_exp0(stmpe_1600_exp0), 00334 stmpe1600_exp1(stmpe_1600_exp1) 00335 { 00336 uint16_t expander_data; 00337 00338 // detect the extenders 00339 stmpe1600_exp0.read_16bit_reg(0x00, &expander_data); 00340 // if (ExpanderData != 0x1600) {/* log - failed to find expander exp0 */ } 00341 stmpe1600_exp1.read_16bit_reg(0x00, &expander_data); 00342 // if (ExpanderData != 0x1600) {/* log - failed to find expander exp1 */ } 00343 00344 // configure all necessary GPIO pins as outputs 00345 expander_data = 0xFFFF; 00346 stmpe1600_exp0.write_16bit_reg(GPDR, &expander_data); 00347 expander_data = 0xBFFF; // leave bit 14 as an input, for the pushbutton, PB1. 00348 stmpe1600_exp1.write_16bit_reg(GPDR, &expander_data); 00349 00350 // shut down all segment and all device 00351 expander_data = 0x7F + (0x7F << 7); 00352 stmpe1600_exp0.write_16bit_reg(GPSR, &expander_data); 00353 stmpe1600_exp1.write_16bit_reg(GPSR, &expander_data); 00354 } 00355 00356 /*** Interface Methods ***/ 00357 /** 00358 * @brief Print the string on display 00359 * @param[in] String to be printed 00360 * @param[in] String lenght [min 1, max 4] 00361 * @return void 00362 */ 00363 void display_string(const char *str) 00364 { 00365 uint16_t expander_data; 00366 uint32_t segments; 00367 int bit_posn; 00368 int i; 00369 00370 for (i = 0; (i < 4 && str[i] != 0); i++) { 00371 segments = (uint32_t) ascii_to_display_lut[(uint8_t) str[i]]; 00372 segments = (~segments) & 0x7F; 00373 bit_posn = display_bit_posn[i]; 00374 cur_io_val.u32 &= ~(0x7F << bit_posn); 00375 cur_io_val.u32 |= segments << bit_posn; 00376 } 00377 /* clear unused digit */ 00378 for (; i < 4; i++) { 00379 bit_posn = display_bit_posn[i]; 00380 cur_io_val.u32 |= 0x7F << bit_posn; 00381 } 00382 00383 // stmpe1600_exp0.write16bitReg(GPSR, (uint16_t *)&CurIOVal.bytes[0]); 00384 // stmpe1600_exp1.write16bitReg(GPSR, (uint16_t *)&CurIOVal.bytes[2]); 00385 00386 // ordered low-byte/high-byte! 00387 cur_io_val.bytes [1] |= 0xC0; // ensure highest bits are high, as these are xshutdown pins for left & right sensors! 00388 expander_data = (cur_io_val.bytes [1] << 8) + cur_io_val.bytes [0]; 00389 stmpe1600_exp0.write_16bit_reg(GPSR, &expander_data); 00390 cur_io_val.bytes [3] |= 0x80; // ensure highest bit is high, as this is xshutdown pin on central sensor! 00391 expander_data = (cur_io_val.bytes [3] << 8) + cur_io_val.bytes [2]; 00392 stmpe1600_exp1.write_16bit_reg(GPSR, &expander_data); 00393 00394 } 00395 00396 void clear_display(void) 00397 { 00398 uint16_t expander_data; 00399 00400 expander_data = 0x7F + (0x7F << 7); 00401 stmpe1600_exp0.write_16bit_reg(GPSR, &expander_data); 00402 stmpe1600_exp1.write_16bit_reg(GPSR, &expander_data); 00403 } 00404 }; 00405 00406 #ifdef __cplusplus 00407 } 00408 #endif 00409 #endif // __DISPLAY_H 00410
Generated on Wed Jul 13 2022 19:35:41 by
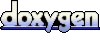