KX022 Accelerometer library
Dependents: LazuriteGraph_Hello KX022_Hello GR-PEACH_IoT_Platform_HTTP_sample
KX022.h
00001 /* Copyright (c) 2015 ARM Ltd., MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 * 00018 * 00019 * KX022 Accelerometer library 00020 * 00021 * @author Toyomasa Watarai 00022 * @version 1.0 00023 * @date 30-December-2015 00024 * 00025 * Library for "KX022 Accelerometer library" from Kionix a Rohm group 00026 * http://www.kionix.com/product/KX022-1020 00027 * 00028 */ 00029 00030 #ifndef KX022_H 00031 #define KX022_H 00032 00033 #include "mbed.h" 00034 00035 #define KX022_DEFAULT_SLAVE_ADDRESS (0x1E << 1) 00036 #define KX022_WAI_VAL (0x14) 00037 00038 #define KX022_XOUT_L (0x06) 00039 #define KX022_XOUT_H (0x07) 00040 #define KX022_YOUT_L (0x08) 00041 #define KX022_YOUT_H (0x09) 00042 #define KX022_ZOUT_L (0x0A) 00043 #define KX022_ZOUT_H (0x0B) 00044 #define KX022_WHO_AM_I (0x0F) 00045 #define KX022_CNTL1 (0x18) 00046 #define KX022_CNTL3 (0x1A) 00047 #define KX022_ODCNTL (0x1B) 00048 #define KX022_TILT_TIMER (0x22) 00049 00050 #define _DEBUG 00051 #ifdef _DEBUG 00052 extern Serial pc; 00053 #define DEBUG_PRINT(...) pc.printf(__VA_ARGS__) 00054 #else 00055 #define DEBUG_PRINT(...) 00056 #endif 00057 00058 /** 00059 * KX022 accelerometer example 00060 * 00061 * @code 00062 * #include "mbed.h" 00063 * #include "KX022.h" 00064 * 00065 * int main(void) { 00066 * 00067 * KX022 acc(I2C_SDA, I2C_SCL); 00068 * PwmOut rled(LED_RED); 00069 * PwmOut gled(LED_GREEN); 00070 * PwmOut bled(LED_BLUE); 00071 * 00072 * while (true) { 00073 * rled = 1.0 - abs(acc.getAccX()); 00074 * gled = 1.0 - abs(acc.getAccY()); 00075 * bled = 1.0 - abs(acc.getAccZ()); 00076 * wait(0.1); 00077 * } 00078 * } 00079 * @endcode 00080 */ 00081 class KX022 00082 { 00083 public: 00084 /** 00085 * KX022 constructor 00086 * 00087 * @param sda SDA pin 00088 * @param sdl SCL pin 00089 * @param addr slave address of the I2C peripheral (default: 0x3C) 00090 */ 00091 KX022(PinName sda, PinName scl, int addr = KX022_DEFAULT_SLAVE_ADDRESS); 00092 00093 /** 00094 * Create a KX022 instance which is connected to specified I2C pins 00095 * with specified address 00096 * 00097 * @param i2c_obj I2C object (instance) 00098 * @param addr slave address of the I2C-bus peripheral (default: 0x3C) 00099 */ 00100 KX022(I2C &i2c_obj, int addr = KX022_DEFAULT_SLAVE_ADDRESS); 00101 00102 /** 00103 * KX022 destructor 00104 */ 00105 ~KX022(); 00106 00107 /** Initializa KX022 sensor 00108 * 00109 * Configure sensor setting 00110 * 00111 */ 00112 void initialize(void); 00113 00114 /** 00115 * Get X axis acceleration 00116 * 00117 * @returns X axis acceleration 00118 */ 00119 float getAccX(); 00120 00121 /** 00122 * Get Y axis acceleration 00123 * 00124 * @returns Y axis acceleration 00125 */ 00126 float getAccY(); 00127 00128 /** 00129 * Get Z axis acceleration 00130 * 00131 * @returns Z axis acceleration 00132 */ 00133 float getAccZ(); 00134 00135 /** 00136 * Get XYZ axis acceleration 00137 * 00138 * @param res array where acceleration data will be stored 00139 */ 00140 void getAccAllAxis(float * res); 00141 00142 private: 00143 I2C m_i2c; 00144 int m_addr; 00145 void readRegs(int addr, uint8_t * data, int len); 00146 void writeRegs(uint8_t * data, int len); 00147 int16_t getAccAxis(uint8_t addr); 00148 00149 }; 00150 00151 #endif
Generated on Fri Jul 15 2022 08:19:45 by
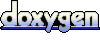