RZ/A1H CMSIS-RTOS RTX BSP for GR-PEACH.
Dependents: GR-PEACH_Azure_Speech ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample ... more
Fork of R_BSP by
R_BSP_Ssif.cpp
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer* 00021 * Copyright (C) 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 00024 #include "r_bsp_cmn.h" 00025 #include "R_BSP_Ssif.h" 00026 #include "ssif_if.h" 00027 #include "ssif_api.h" 00028 00029 R_BSP_Ssif::R_BSP_Ssif(PinName sck, PinName ws, PinName tx, PinName rx, uint8_t int_level, int32_t max_write_num, int32_t max_read_num) { 00030 int32_t wk_channel; 00031 00032 wk_channel = ssif_init(sck, ws, tx, rx); 00033 if (wk_channel != NC) { 00034 ssif_ch = wk_channel; 00035 00036 ssif_cfg.enabled = true; 00037 ssif_cfg.int_level = 0x78; 00038 ssif_cfg.slave_mode = true; 00039 ssif_cfg.sample_freq = 44100u; 00040 ssif_cfg.clk_select = SSIF_CFG_CKS_AUDIO_X1; 00041 ssif_cfg.multi_ch = SSIF_CFG_MULTI_CH_1; 00042 ssif_cfg.data_word = SSIF_CFG_DATA_WORD_16; 00043 ssif_cfg.system_word = SSIF_CFG_SYSTEM_WORD_32; 00044 ssif_cfg.bclk_pol = SSIF_CFG_FALLING; 00045 ssif_cfg.ws_pol = SSIF_CFG_WS_LOW; 00046 ssif_cfg.padding_pol = SSIF_CFG_PADDING_LOW; 00047 ssif_cfg.serial_alignment = SSIF_CFG_DATA_FIRST; 00048 ssif_cfg.parallel_alignment = SSIF_CFG_LEFT; 00049 ssif_cfg.ws_delay = SSIF_CFG_DELAY; 00050 ssif_cfg.noise_cancel = SSIF_CFG_ENABLE_NOISE_CANCEL; 00051 ssif_cfg.tdm_mode = SSIF_CFG_DISABLE_TDM; 00052 ssif_cfg.romdec_direct.mode = SSIF_CFG_DISABLE_ROMDEC_DIRECT; 00053 ssif_cfg.romdec_direct.p_cbfunc = NULL; 00054 00055 init_channel(R_SSIF_MakeCbTbl_mbed(), ssif_ch, &ssif_cfg, max_write_num, max_read_num); 00056 } 00057 } 00058 00059 R_BSP_Ssif::~R_BSP_Ssif() { 00060 // do nothing 00061 } 00062 00063 bool R_BSP_Ssif::ConfigChannel(const ssif_channel_cfg_t* const p_ch_cfg) { 00064 return ioctl(SSIF_CONFIG_CHANNEL, (void *)p_ch_cfg); 00065 } 00066 00067 bool R_BSP_Ssif::GetStatus(uint32_t* const p_status) { 00068 return ioctl(SSIF_CONFIG_CHANNEL, (void *)p_status); 00069 }
Generated on Tue Jul 12 2022 20:43:58 by
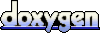