Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
window_surfaces_cpp.cpp
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2014 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /** 00024 * @file window_surfaces_cpp.cpp 00025 * @brief $Module: CLibCommon $ $PublicVersion: 1.20 $ (=CLIB_VERSION) 00026 * $Rev: 47 $ 00027 * $Date:: 2014-01-06 18:18:48 +0900#$ 00028 * - Description: Window Surfaces for mbed style C++ API 00029 */ 00030 00031 00032 /****************************************************************************** 00033 Includes <System Includes> , "Project Includes" 00034 *******************************************************************************/ 00035 #include "window_surfaces.hpp " 00036 #include "window_surfaces.h" 00037 00038 00039 /****************************************************************************** 00040 Typedef definitions 00041 ******************************************************************************/ 00042 00043 /****************************************************************************** 00044 Macro definitions 00045 ******************************************************************************/ 00046 #define GS_DEFAULT_INT_VALUE 0x7FFFFFFF 00047 #define GS_DEFAULT_HEIGHT -1 00048 #define GS_DEFAULT_CLEAR_COLOR 0x00000100 00049 00050 00051 /****************************************************************************** 00052 Imported global variables and functions (from other files) 00053 ******************************************************************************/ 00054 00055 /****************************************************************************** 00056 Exported global variables and functions (to be accessed by other files) 00057 ******************************************************************************/ 00058 00059 /****************************************************************************** 00060 Private global variables and functions 00061 ******************************************************************************/ 00062 00063 00064 /*********************************************************************** 00065 * ClassImplement: WindowSurfacesClass 00066 ************************************************************************/ 00067 00068 /*********************************************************************** 00069 * Implement: WindowSurfacesClass 00070 ************************************************************************/ 00071 WindowSurfacesClass::WindowSurfacesClass() 00072 { 00073 this->_self = NULL; 00074 } 00075 00076 00077 /*********************************************************************** 00078 * Implement: ~WindowSurfacesClass 00079 ************************************************************************/ 00080 WindowSurfacesClass::~WindowSurfacesClass() 00081 { 00082 this->destroy(); 00083 } 00084 00085 00086 /*********************************************************************** 00087 * Implement: initialize 00088 ************************************************************************/ 00089 errnum_t WindowSurfacesClass::initialize( WindowSurfacesConfigClass &in_out_config ) 00090 { 00091 this->_self = new window_surfaces_t; 00092 ASSERT_R( this->_self != NULL, return E_FEW_MEMORY ); 00093 00094 in_out_config.flags = 00095 F_WINDOW_SURFACES_PIXEL_FORMAT | 00096 F_WINDOW_SURFACES_LAYER_COUNT | 00097 F_WINDOW_SURFACES_BACKGROUND_COLOR | 00098 F_WINDOW_SURFACES_BUFFER_HEIGHT; 00099 00100 if ( in_out_config.buffer_height == GS_DEFAULT_HEIGHT ) { 00101 in_out_config.flags &= ~F_WINDOW_SURFACES_BUFFER_HEIGHT; 00102 } 00103 if ( in_out_config.background_color.Value == GS_DEFAULT_CLEAR_COLOR ) { 00104 in_out_config.flags &= ~F_WINDOW_SURFACES_BACKGROUND_COLOR; 00105 } 00106 00107 R_WINDOW_SURFACES_InitConst( this->_self ); 00108 00109 return R_WINDOW_SURFACES_Initialize( this->_self, 00110 (window_surfaces_config_t *) &in_out_config ); 00111 } 00112 00113 00114 /*********************************************************************** 00115 * Implement: destroy 00116 ************************************************************************/ 00117 void WindowSurfacesClass::destroy() 00118 { 00119 if ( this->_self != NULL ) { 00120 errnum_t e = R_WINDOW_SURFACES_Finalize( this->_self, 0 ); 00121 ASSERT_R( e == 0, R_NOOP() ); 00122 } 00123 } 00124 00125 00126 /*********************************************************************** 00127 * Implement: get_layer_frame_buffer 00128 ************************************************************************/ 00129 errnum_t WindowSurfacesClass::get_layer_frame_buffer( int_fast32_t const layer_num, frame_buffer_t **const out_frame_buffer ) 00130 { 00131 return R_WINDOW_SURFACES_GetLayerFrameBuffer( this->_self, layer_num, out_frame_buffer ); 00132 } 00133 00134 00135 /*********************************************************************** 00136 * Implement: swap_buffers 00137 ************************************************************************/ 00138 #ifdef IS_WINDOW_SURFACES_EX 00139 errnum_t WindowSurfacesClass::swap_buffers( int_fast32_t const layer_num, Canvas2D_ContextClass &context ) 00140 { 00141 return R_WINDOW_SURFACES_SwapBuffers( this->_self, layer_num, context.c_LanguageContext ); 00142 } 00143 #else 00144 errnum_t WindowSurfacesClass::swap_buffers( int_fast32_t const layer_num, const void *const null_context ) 00145 { 00146 return R_WINDOW_SURFACES_SwapBuffers( this->_self, layer_num, null_context ); 00147 } 00148 #endif 00149 00150 00151 /*********************************************************************** 00152 * Implement: swap_buffers_start 00153 ************************************************************************/ 00154 errnum_t WindowSurfacesClass::swap_buffers_start( int_fast32_t const layer_num, r_ospl_async_t *async ) 00155 { 00156 return R_WINDOW_SURFACES_SwapBuffersStart( this->_self, layer_num, async ); 00157 } 00158 00159 00160 /*********************************************************************** 00161 * Implement: wait_for_v_sync 00162 ************************************************************************/ 00163 errnum_t WindowSurfacesClass::wait_for_v_sync( int_fast32_t const swap_interval, bool_t const is_1_v_sync_at_minimum ) 00164 { 00165 return R_WINDOW_SURFACES_WaitForVSync( this->_self, swap_interval, is_1_v_sync_at_minimum ); 00166 } 00167 00168 00169 /*********************************************************************** 00170 * Implement: alloc_offscreen_stack 00171 ************************************************************************/ 00172 errnum_t WindowSurfacesClass::alloc_offscreen_stack( frame_buffer_t *const in_out_frame_buffer ) 00173 { 00174 return R_WINDOW_SURFACES_AllocOffscreenStack( this->_self, in_out_frame_buffer ); 00175 } 00176 00177 00178 /*********************************************************************** 00179 * Implement: free_offscreen_stack 00180 ************************************************************************/ 00181 errnum_t WindowSurfacesClass::free_offscreen_stack( const frame_buffer_t *const frame_buffer ) 00182 { 00183 return R_WINDOW_SURFACES_FreeOffscreenStack( this->_self, frame_buffer ); 00184 } 00185 00186 00187 /*********************************************************************** 00188 * Implement: do_message_loop 00189 ************************************************************************/ 00190 #ifdef IS_WINDOW_SURFACES_EX 00191 errnum_t WindowSurfacesClass::do_message_loop() 00192 { 00193 return R_WINDOW_SURFACES_DoMessageLoop( this->_self ); 00194 } 00195 #endif 00196 00197 00198 /*********************************************************************** 00199 * Implement: access_layer_attributes 00200 ************************************************************************/ 00201 #ifdef IS_WINDOW_SURFACES_EX 00202 errnum_t WindowSurfacesClass::access_layer_attributes( LayerAttributes &in_out_Attributes ) 00203 { 00204 in_out_Attributes.flags = 00205 F_LAYER_ID | 00206 F_LAYER_PRIORITY | 00207 F_LAYER_X | 00208 F_LAYER_Y | 00209 F_LAYER_WIDTH | 00210 F_LAYER_HEIGHT | 00211 F_LAYER_OFFSET_X | 00212 F_LAYER_OFFSET_Y | 00213 F_LAYER_CLUT | 00214 F_LAYER_CLUT_COUNT; 00215 00216 if ( in_out_Attributes.id == GS_DEFAULT_INT_VALUE ) { 00217 in_out_Attributes.flags &= ~F_LAYER_ID; 00218 } 00219 if ( in_out_Attributes.priority == GS_DEFAULT_INT_VALUE ) { 00220 in_out_Attributes.flags &= ~F_LAYER_PRIORITY; 00221 } 00222 if ( in_out_Attributes.x == GS_DEFAULT_INT_VALUE ) { 00223 in_out_Attributes.flags &= ~F_LAYER_X; 00224 } 00225 if ( in_out_Attributes.y == GS_DEFAULT_INT_VALUE ) { 00226 in_out_Attributes.flags &= ~F_LAYER_Y; 00227 } 00228 if ( in_out_Attributes.width == GS_DEFAULT_INT_VALUE ) { 00229 in_out_Attributes.flags &= ~F_LAYER_WIDTH; 00230 } 00231 if ( in_out_Attributes.height == GS_DEFAULT_INT_VALUE ) { 00232 in_out_Attributes.flags &= ~F_LAYER_HEIGHT; 00233 } 00234 if ( in_out_Attributes.offset_x == GS_DEFAULT_INT_VALUE ) { 00235 in_out_Attributes.flags &= ~F_LAYER_OFFSET_X; 00236 } 00237 if ( in_out_Attributes.offset_y == GS_DEFAULT_INT_VALUE ) { 00238 in_out_Attributes.flags &= ~F_LAYER_OFFSET_Y; 00239 } 00240 if ( in_out_Attributes.CLUT == NULL ) { 00241 in_out_Attributes.flags &= ~F_LAYER_CLUT; 00242 } 00243 if ( in_out_Attributes.CLUT_count == 0 ) { 00244 in_out_Attributes.flags &= ~F_LAYER_CLUT_COUNT; 00245 } 00246 00247 return R_WINDOW_SURFACES_AccessLayerAttributes( this->_self, 00248 (layer_attributes_t *) &in_out_Attributes ); 00249 } 00250 #endif 00251 00252 00253 /*********************************************************************** 00254 * ClassImplement: WindowSurfacesConfigClass 00255 ************************************************************************/ 00256 00257 /*********************************************************************** 00258 * Implement: WindowSurfacesConfigClass 00259 ************************************************************************/ 00260 WindowSurfacesConfigClass::WindowSurfacesConfigClass() 00261 { 00262 this->flags = 0; 00263 this->pixel_format = PIXEL_FORMAT_ARGB8888; 00264 this->layer_count = 1; 00265 this->buffer_height = GS_DEFAULT_HEIGHT; 00266 this->background_color.Value = GS_DEFAULT_CLEAR_COLOR; 00267 } 00268 00269 00270 /*********************************************************************** 00271 * ClassImplement: LayerAttributes 00272 ************************************************************************/ 00273 00274 /*********************************************************************** 00275 * Implement: LayerAttributes 00276 ************************************************************************/ 00277 #ifdef IS_WINDOW_SURFACES_EX 00278 LayerAttributes::LayerAttributes() 00279 { 00280 this->access = ACCESS_READ; 00281 this->flags = 0; 00282 this->id = GS_DEFAULT_INT_VALUE; 00283 this->priority = GS_DEFAULT_INT_VALUE; 00284 this->is_show = false; 00285 this->is_color_key = false; 00286 this->color_key.Value = GS_DEFAULT_CLEAR_COLOR; 00287 this->layer_color.Value = 0; 00288 this->x = GS_DEFAULT_INT_VALUE; 00289 this->y = GS_DEFAULT_INT_VALUE; 00290 this->width = GS_DEFAULT_INT_VALUE; 00291 this->height = GS_DEFAULT_INT_VALUE; 00292 this->offset_x = GS_DEFAULT_INT_VALUE; 00293 this->offset_y = GS_DEFAULT_INT_VALUE; 00294 this->CLUT = NULL; 00295 this->CLUT_count = 0; 00296 } 00297 #endif 00298 00299
Generated on Tue Jul 12 2022 11:15:05 by
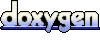