Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
window_surfaces.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2014 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /****************************************************************************** 00024 * $FileName: window_surfaces.h $ 00025 * $Module: CLibCommon $ $PublicVersion: 1.00 $ (=CLIB_VERSION) 00026 * $Rev: 47 $ 00027 * $Date:: 2014-01-06 18:18:48 +0900#$ 00028 * Description: Sample API 00029 *******************************************************************************/ 00030 00031 #ifndef WINDOW_SURFACES_H 00032 #define WINDOW_SURFACES_H 00033 00034 /****************************************************************************** 00035 Includes <System Includes> , "Project Includes" 00036 ******************************************************************************/ 00037 #include "clib_drivers_typedef.h " 00038 #include "window_surfaces_typedef.h " 00039 #include "r_multi_compiler_typedef.h" 00040 #include "window_surfaces_inline.h " 00041 #ifdef IS_WINDOW_SURFACES_EX 00042 #include "window_surfaces_ex.h " 00043 #include "RGA_API_typedef.h " 00044 #endif 00045 00046 #ifdef __cplusplus 00047 extern "C" { 00048 #endif /* __cplusplus */ 00049 00050 00051 /****************************************************************************** 00052 Typedef definitions 00053 ******************************************************************************/ 00054 /* In "frame_buffer_typedef.h" */ 00055 00056 /****************************************************************************** 00057 Macro definitions 00058 ******************************************************************************/ 00059 /* In "frame_buffer_typedef.h" */ 00060 00061 /****************************************************************************** 00062 Variable Externs 00063 ******************************************************************************/ 00064 /* In "frame_buffer_typedef.h" */ 00065 00066 /****************************************************************************** 00067 Functions Prototypes 00068 ******************************************************************************/ 00069 00070 /** 00071 * @brief R_WINDOW_SURFACES_InitConst 00072 * 00073 * @param self window_surfaces_t 00074 * @return None 00075 */ 00076 void R_WINDOW_SURFACES_InitConst( window_surfaces_t *const self ); 00077 00078 00079 /** 00080 * @brief R_WINDOW_SURFACES_Initialize 00081 * 00082 * @param self window_surfaces_t 00083 * @param in_out_config window_surfaces_config_t 00084 * @return Error code, 0=No error 00085 */ 00086 errnum_t R_WINDOW_SURFACES_Initialize( window_surfaces_t *const self, 00087 window_surfaces_config_t *in_out_config ); 00088 00089 00090 /** 00091 * @brief R_WINDOW_SURFACES_Finalize 00092 * 00093 * @param self window_surfaces_t 00094 * @param e Errors that have occurred. No error = 0 00095 * @return Error code or e, 0 = successful and input e=0 00096 */ 00097 errnum_t R_WINDOW_SURFACES_Finalize( window_surfaces_t *const self, errnum_t e ); 00098 00099 00100 /** 00101 * @brief Get <frame_buffer_t>* of specified layer number 00102 * 00103 * @param self window_surfaces_t 00104 * @param layer_num layer_num 00105 * @param out_frame_buffer out_frame_buffer 00106 * @return Error code, 0=No error 00107 */ 00108 errnum_t R_WINDOW_SURFACES_GetLayerFrameBuffer( const window_surfaces_t *const self, 00109 int_fast32_t const layer_num, frame_buffer_t **const out_frame_buffer ); 00110 00111 00112 /** 00113 * @brief R_WINDOW_SURFACES_SwapBuffers 00114 * 00115 * @param self window_surfaces_t 00116 * @param layer_num layer_num 00117 * @param context Graphics context or NULL 00118 * @return Error code, 0=No error 00119 */ 00120 #ifdef IS_WINDOW_SURFACES_EX 00121 errnum_t R_WINDOW_SURFACES_SwapBuffers( window_surfaces_t *const self, 00122 int_fast32_t const layer_num, graphics_t *const context ); 00123 #else 00124 errnum_t R_WINDOW_SURFACES_SwapBuffers( window_surfaces_t *const self, 00125 int_fast32_t const layer_num, const void *const null_context ); 00126 #endif 00127 00128 00129 /** 00130 * @brief Asynchronous function of <R_WINDOW_SURFACES_SwapBuffers> 00131 * 00132 * @param self window_surfaces_t 00133 * @param layer_num layer_num 00134 * @param async r_ospl_async_t 00135 * @return Error code, 0=No error 00136 */ 00137 errnum_t R_WINDOW_SURFACES_SwapBuffersStart( window_surfaces_t *const self, 00138 int_fast32_t const layer_num, r_ospl_async_t *async ); 00139 00140 00141 /** 00142 * @brief Wait for V-Sync 00143 * 00144 * @param swap_interval If 1=60fps Then 2=30fps, 3=20fps 00145 * @param is_1_v_sync_at_minimum false = It is possible to return soon 00146 * @return Error Code. 0=No Error. 00147 */ 00148 errnum_t R_WINDOW_SURFACES_WaitForVSync( window_surfaces_t *const self, 00149 int_fast32_t const swap_interval, bool_t const is_1_v_sync_at_minimum ); 00150 00151 00152 /** 00153 * @brief Allocate offscreen from stack 00154 * 00155 * @param self window_surfaces_t 00156 * @param in_out_frame_buffer frame_buffer_t 00157 * @return Error code, 0=No error 00158 * 00159 * @par Description 00160 * - (input) - >stride, ->height, ->buffer_count 00161 * - (output) - >buffer_address[(all)] 00162 */ 00163 errnum_t R_WINDOW_SURFACES_AllocOffscreenStack( window_surfaces_t *const self, 00164 frame_buffer_t *const in_out_frame_buffer ); 00165 00166 00167 /** 00168 * @brief Free offscreen to stack 00169 * 00170 * @param self window_surfaces_t 00171 * @param frame_buffer frame_buffer_t 00172 * @return Error code, 0=No error 00173 * 00174 * @par Description 00175 * - If frame_buffer - >buffer_count == 0, do nothing. 00176 */ 00177 errnum_t R_WINDOW_SURFACES_FreeOffscreenStack( window_surfaces_t *const self, 00178 const frame_buffer_t *const frame_buffer ); 00179 00180 00181 #ifdef __cplusplus 00182 } /* extern "C" */ 00183 #endif /* __cplusplus */ 00184 00185 #endif
Generated on Tue Jul 12 2022 11:15:05 by
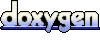