Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
window_surfaces.c
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2014 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /** 00024 * @file window_surfaces.c 00025 * @brief $Module: CLibCommon $ $PublicVersion: 1.00 $ (=CLIB_VERSION) 00026 * $Rev: 51 $ 00027 * $Date:: 2014-03-14 18:42:33 +0900#$ 00028 */ 00029 00030 00031 /****************************************************************************** 00032 Includes <System Includes> , "Project Includes" 00033 *******************************************************************************/ 00034 #include "r_typedefs.h" 00035 #include "lcd_panel.h" 00036 #include "r_ospl.h" 00037 #include "clib_registers.h " 00038 #include "clib_drivers.h " 00039 #include "clib_samples.h" 00040 #include "window_surfaces.h" 00041 #include "vsync.h " 00042 #include "window_surfaces_private.h" 00043 #include "RGA.h" 00044 #include "RGA_Port.h" 00045 #if USE_LCD 00046 #include "lcd_if.h" 00047 #endif 00048 #include <stdio.h> /* in R_WINDOW_SURFACES_DoMessageLoop */ 00049 00050 00051 /****************************************************************************** 00052 Typedef definitions 00053 ******************************************************************************/ 00054 00055 /** 00056 * @typedef gs_frame_width_height_t 00057 * @brief gs_frame_width_height_t 00058 */ 00059 #ifdef IS_WINDOW_SURFACES_EX 00060 typedef struct st_gs_frame_width_height_t gs_frame_width_height_t; 00061 struct st_gs_frame_width_height_t { 00062 int_fast32_t Width; 00063 int_fast32_t Height; 00064 }; 00065 #endif 00066 00067 00068 /** 00069 * @typedef gs_rectangle_t 00070 * @brief gs_rectangle_t 00071 */ 00072 #ifdef IS_WINDOW_SURFACES_EX 00073 typedef struct st_gs_rectangle_t gs_rectangle_t; 00074 struct st_gs_rectangle_t { 00075 int_fast32_t Left; 00076 int_fast32_t Top; 00077 int_fast32_t Right; /* include right bound pixel */ 00078 int_fast32_t Bottom; /* include bottom bound pixel */ /* Top < Bottom */ 00079 }; 00080 #endif 00081 00082 00083 /****************************************************************************** 00084 Macro definitions 00085 ******************************************************************************/ 00086 00087 /* GS_ : global static */ 00088 /* VDC5_CHANNEL_0 = LCD_VDC5_CH0_PANEL = ... */ 00089 /* VDC5_CHANNEL_1 = LCD_VDC5_CH1_PANEL = ... */ 00090 #if WINDOW_SURFACES_DEFAULT_CHANNEL 00091 #define GS_CHANNEL_n VDC5_CHANNEL_1 00092 #define GS_LCD_CHn_SIG_FV LCD_CH1_SIG_FV 00093 #define GS_LCD_CHn_SIG_FH LCD_CH1_SIG_FH 00094 #define GS_LCD_CHn_DISP_VS LCD_CH1_DISP_VS 00095 #define GS_LCD_CHn_DISP_VW LCD_CH1_DISP_VW 00096 #define GS_LCD_CHn_DISP_HS LCD_CH1_DISP_HS 00097 #define GS_LCD_CHn_DISP_HW LCD_CH1_DISP_HW 00098 #define GS_LCD_CHn_TCON_HALF LCD_CH1_TCON_HALF 00099 #define GS_LCD_CHn_TCON_OFFSET LCD_CH1_TCON_OFFSET 00100 #define GS_LCD_CHn_OUT_EDGE LCD_CH1_OUT_EDGE 00101 #define GS_LCD_CHn_OUT_FORMAT LCD_CH1_OUT_FORMAT 00102 #define GS_LCD_CHn_PANEL_CLK LCD_CH1_PANEL_CLK 00103 #define GS_LCD_CHn_PANEL_CLK_DIV LCD_CH1_PANEL_CLK_DIV 00104 #define GS_DISPLAY_CH 1 /* 1 or 0 */ 00105 #else 00106 #define GS_CHANNEL_n VDC5_CHANNEL_0 00107 #define GS_LCD_CHn_SIG_FV LCD_CH0_SIG_FV 00108 #define GS_LCD_CHn_SIG_FH LCD_CH0_SIG_FH 00109 #define GS_LCD_CHn_DISP_VS LCD_CH0_DISP_VS 00110 #define GS_LCD_CHn_DISP_VW LCD_CH0_DISP_VW 00111 #define GS_LCD_CHn_DISP_HS LCD_CH0_DISP_HS 00112 #define GS_LCD_CHn_DISP_HW LCD_CH0_DISP_HW 00113 #define GS_LCD_CHn_TCON_HALF LCD_CH0_TCON_HALF 00114 #define GS_LCD_CHn_TCON_OFFSET LCD_CH0_TCON_OFFSET 00115 #define GS_LCD_CHn_OUT_EDGE LCD_CH0_OUT_EDGE 00116 #define GS_LCD_CHn_OUT_FORMAT LCD_CH0_OUT_FORMAT 00117 #define GS_LCD_CHn_PANEL_CLK LCD_CH0_PANEL_CLK 00118 #define GS_LCD_CHn_PANEL_CLK_DIV LCD_CH0_PANEL_CLK_DIV 00119 #define GS_DISPLAY_CH 0 /* 1 or 0 */ 00120 #endif 00121 00122 #if 1 00123 #define GS_BUFFER_WIDTH 800 00124 #define GS_BUFFER_HEIGHT 480 00125 #else 00126 #define GS_BUFFER_WIDTH GS_LCD_CHn_DISP_HW 00127 #define GS_BUFFER_HEIGHT GS_LCD_CHn_DISP_VW 00128 #endif 00129 00130 #define GS_MEASURE_GPU_LOAD 0 00131 00132 #define GS_DEFAULT_CLEAR_COLOR R_RGA_DEFAULT_CLEAR_COLOR 00133 00134 #ifdef R_REE_INIT 00135 #define GRAPHICS_GetLvdsParam Graphics_GetLvdsParam 00136 #define GRAPHICS_SetLcdTconSettings Graphics_SetLcdTconSettings 00137 #define GRAPHICS_SetLcdPanel Graphics_SetLcdPanel 00138 #endif 00139 #ifdef RZ_A1L 00140 #define GRAPHICS_GetLvdsParam( ch ) NULL 00141 #define GRAPHICS_SetLcdTconSettings( ch, setting ) GRAPHICS_L_SetLcdTconSettings( setting ) 00142 #define GRAPHICS_SetLcdPanel( ch ) GRAPHICS_L_SetLcdPanel() 00143 #endif 00144 00145 /** 00146 * @def GS_OFFSET_BYTE_NOT_SHOW 00147 * @brief GS_OFFSET_BYTE_NOT_SHOW 00148 */ 00149 enum { GS_OFFSET_BYTE_NOT_SHOW = -1 }; 00150 00151 00152 /****************************************************************************** 00153 Imported global variables and functions (from other files) 00154 ******************************************************************************/ 00155 00156 /****************************************************************************** 00157 Exported global variables and functions (to be accessed by other files) 00158 ******************************************************************************/ 00159 00160 /****************************************************************************** 00161 Private global variables and functions 00162 ******************************************************************************/ 00163 00164 static errnum_t R_WINDOW_SURFACES_SwapBuffers_Sub( window_surfaces_t *const self, 00165 int_fast32_t const layer_num ); 00166 00167 #ifdef IS_WINDOW_SURFACES_EX 00168 errnum_t GS_ToInFrame( 00169 gs_frame_width_height_t *SourceFrameBuffer, 00170 gs_frame_width_height_t *DestinationFrameBuffer, 00171 gs_rectangle_t *SourceRect, 00172 gs_rectangle_t *DestinationRect ); 00173 #endif 00174 00175 00176 /** 00177 * @brief alloc_VRAM_stack_sub 00178 * 00179 * @param in_out_StackPointer in_out_StackPointer 00180 * @param OverOfVRAM OverOfVRAM 00181 * @param in_out_FrameBuffer in_out_FrameBuffer 00182 * @return Error code, 0=No error 00183 * 00184 * @par Description 00185 * - (input) - >stride, ->height, ->buffer_count 00186 * - (output) - >buffer_address[(all)] 00187 */ 00188 static errnum_t alloc_VRAM_stack_sub( uint8_t **const in_out_StackPointer, 00189 const uint8_t *const OverOfVRAM, 00190 frame_buffer_t *const in_out_FrameBuffer ); /* QAC-3450 */ 00191 static errnum_t alloc_VRAM_stack_sub( uint8_t **const in_out_StackPointer, 00192 const uint8_t *const OverOfVRAM, 00193 frame_buffer_t *const in_out_FrameBuffer ) 00194 { 00195 errnum_t e; 00196 int_fast32_t size_1; 00197 int_fast32_t size_all; 00198 int_fast32_t buffer_num; 00199 uint8_t *stack_pointer; 00200 00201 00202 IF_DQ( in_out_StackPointer == NULL ) { 00203 e=E_OTHERS; 00204 goto fin; 00205 } 00206 IF_DQ( in_out_FrameBuffer == NULL ) { 00207 e=E_OTHERS; 00208 goto fin; 00209 } 00210 00211 00212 stack_pointer = *in_out_StackPointer; 00213 00214 00215 /* Set "size_1" */ 00216 size_1 = in_out_FrameBuffer->stride * in_out_FrameBuffer->height; 00217 size_1 = ( R_Ceil_64s( size_1 ) ); 00218 00219 00220 /* Set "size_all" */ 00221 size_all = in_out_FrameBuffer->buffer_count * size_1; 00222 00223 00224 /* Check */ 00225 /* ->MISRA 17.4 */ /* ->SEC R1.3.1 (1) */ 00226 IF ( (stack_pointer + size_all) > OverOfVRAM ) { 00227 in_out_FrameBuffer->buffer_count = 0; 00228 e=E_FEW_ARRAY; 00229 goto fin; 00230 } 00231 /* <-MISRA 17.4 */ /* <-SEC R1.3.1 (1) */ 00232 00233 IF ( in_out_FrameBuffer->buffer_count > 00234 (int_fast32_t) R_COUNT_OF( in_out_FrameBuffer->buffer_address ) ) { 00235 e=E_OTHERS; 00236 goto fin; 00237 } 00238 00239 00240 /* Set "in_out_FrameBuffer->buffer_address" */ 00241 for ( buffer_num = 0; 00242 buffer_num < in_out_FrameBuffer->buffer_count; 00243 buffer_num += 1 ) { 00244 in_out_FrameBuffer->buffer_address[ buffer_num ] = stack_pointer; 00245 00246 /* ->MISRA 17.4 */ /* ->SEC R1.3.1 (1) */ 00247 stack_pointer += size_1; /* MISRA 17.4: Bound check is done by "OverOfVRAM" */ 00248 /* <-MISRA 17.4 */ /* <-SEC R1.3.1 (1) */ 00249 } 00250 for ( /* buffer_num */; 00251 buffer_num < (int_fast32_t) R_COUNT_OF( in_out_FrameBuffer->buffer_address ); 00252 buffer_num += 1 ) { 00253 in_out_FrameBuffer->buffer_address[ buffer_num ] = NULL; 00254 } 00255 00256 *in_out_StackPointer = stack_pointer; 00257 00258 e=0; 00259 fin: 00260 return e; 00261 } 00262 00263 00264 /** 00265 * @brief free_VRAM_stack_sub 00266 * 00267 * @param in_out_StackPointer in_out_StackPointer 00268 * @param frame_buffer frame_buffer 00269 * @param StartOfVRAM StartOfVRAM 00270 * @return Error code, 0=No error 00271 * 00272 * @par Description 00273 * - If frame_buffer - >buffer_count == 0, do nothing. 00274 */ 00275 static errnum_t free_VRAM_stack_sub( uint8_t **const in_out_StackPointer, 00276 const frame_buffer_t *const frame_buffer, 00277 const uint8_t *const StartOfVRAM ); /* QAC-3450 */ 00278 static errnum_t free_VRAM_stack_sub( uint8_t **const in_out_StackPointer, 00279 const frame_buffer_t *const frame_buffer, 00280 const uint8_t *const StartOfVRAM ) 00281 { 00282 errnum_t e; 00283 int_fast32_t size_1; 00284 int_fast32_t size_all; 00285 int_fast32_t buffer_num; 00286 uint8_t *next_stack_pointer; 00287 00288 00289 IF_DQ( frame_buffer == NULL ) { 00290 e=E_OTHERS; 00291 goto fin; 00292 } 00293 IF_DQ( in_out_StackPointer == NULL ) { 00294 e=E_OTHERS; 00295 goto fin; 00296 } 00297 00298 00299 /* Set "size_1" */ 00300 size_1 = frame_buffer->stride * frame_buffer->height; 00301 size_1 = ( R_Ceil_64s( size_1 ) ); 00302 R_STATIC_ASSERT( RGA_STACK_ADDRESS_ALIGNMENT == 64, "" ); /* check of ceil_xx */ 00303 00304 00305 /* Set "size_all" */ 00306 size_all = frame_buffer->buffer_count * size_1; 00307 00308 00309 /* Set "next_stack_pointer" */ 00310 /* ->MISRA 17.4 */ /* ->SEC R1.3.1 (1) */ 00311 next_stack_pointer = *in_out_StackPointer - size_all; 00312 /* MISRA 17.4: Bound check is done by "StartOfVRAM" */ 00313 /* <-MISRA 17.4 */ /* <-SEC R1.3.1 (1) */ 00314 00315 00316 /* Check */ 00317 IF ( next_stack_pointer < StartOfVRAM ) { 00318 e=E_OTHERS; 00319 goto fin; 00320 } 00321 00322 IF ( frame_buffer->buffer_count > (int_fast32_t) R_COUNT_OF( frame_buffer->buffer_address ) ) { 00323 e=E_OTHERS; 00324 goto fin; 00325 } 00326 00327 for ( buffer_num = frame_buffer->buffer_count - 1; buffer_num >= 0; buffer_num -= 1 ) { 00328 /* ->MISRA 17.4 */ /* ->SEC R1.3.1 (1) */ 00329 IF ( frame_buffer->buffer_address[ buffer_num ] != 00330 (next_stack_pointer + (size_1 * buffer_num)) ) { 00331 e=E_ACCESS_DENIED; 00332 goto fin; 00333 } 00334 /* MISRA 17.4: Bound check is done by "size_all" */ 00335 /* <-MISRA 17.4 */ /* <-SEC R1.3.1 (1) */ 00336 } 00337 00338 00339 /* Set "*in_out_StackPointer" */ 00340 *in_out_StackPointer = next_stack_pointer; 00341 00342 e=0; 00343 fin: 00344 return e; 00345 } 00346 00347 00348 /*-------------------------------------------------------------------------*/ 00349 /* <<<< ### (window_surfaces_vdc5_layer_t) Class implement >>>> */ 00350 /*-------------------------------------------------------------------------*/ 00351 00352 /** 00353 * @brief Initialize <window_surfaces_vdc5_layer_t> 00354 * 00355 * @param self window_surfaces_vdc5_layer_t 00356 * @param layer_num layer_num 00357 * @param graphics_layer_ID graphics_layer_ID 00358 * @return Error code, 0=No error 00359 */ 00360 static errnum_t window_surfaces_vdc5_layer_t__initialize( 00361 window_surfaces_vdc5_layer_t *const self, 00362 int_fast32_t const layer_num, 00363 vdc5_graphics_type_t const graphics_layer_ID ); /* QAC-3450 */ 00364 00365 static errnum_t window_surfaces_vdc5_layer_t__initialize( 00366 window_surfaces_vdc5_layer_t *const self, 00367 int_fast32_t const layer_num, 00368 vdc5_graphics_type_t const graphics_layer_ID ) 00369 { 00370 errnum_t e; 00371 00372 IF_DQ( self == NULL ) { 00373 e=E_OTHERS; 00374 goto fin; 00375 } 00376 00377 e= R_int_t_to_int8_t( layer_num, &self->layer_num ); 00378 IF(e!=0) { 00379 goto fin; 00380 } 00381 00382 self->graphics_layer_ID = graphics_layer_ID; 00383 00384 self->is_data_control = false; 00385 00386 self->layer_color = GS_DEFAULT_CLEAR_COLOR; 00387 00388 switch ( graphics_layer_ID ) { 00389 case VDC5_GR_TYPE_GR0: 00390 self->data_control_ID = VDC5_LAYER_ID_0_RD; 00391 break; 00392 #ifndef RZ_A1L 00393 case VDC5_GR_TYPE_GR1: 00394 self->data_control_ID = VDC5_LAYER_ID_1_RD; 00395 break; 00396 #endif 00397 case VDC5_GR_TYPE_GR2: 00398 self->data_control_ID = VDC5_LAYER_ID_2_RD; 00399 break; 00400 case VDC5_GR_TYPE_GR3: 00401 self->data_control_ID = VDC5_LAYER_ID_3_RD; 00402 break; 00403 default: 00404 e=E_OTHERS; 00405 goto fin; /* Bad "graphics_layer_ID" */ 00406 } 00407 00408 e=0; 00409 fin: 00410 return e; 00411 } 00412 00413 00414 00415 /*-------------------------------------------------------------------------*/ 00416 /* <<<< ### (window_surfaces_t) Class implement >>>> */ 00417 /*-------------------------------------------------------------------------*/ 00418 00419 /** 00420 * @brief Get <window_surfaces_vdc5_layer_t> 00421 * 00422 * @param self window_surfaces_t 00423 * @param in_LayerNum in_LayerNum 00424 * @param out_Layer out_Layer 00425 * @return Error code, 0=No error 00426 */ 00427 STATIC_INLINE errnum_t window_surfaces_t__get_layer( window_surfaces_t *const self, 00428 int_fast32_t const in_LayerNum, 00429 window_surfaces_vdc5_layer_t **const out_Layer ); /* QAC-3450 */ 00430 /* ->MISRA 16.7 : "self" can not "const", because "out_Layer" is not "const" */ 00431 /* ->SEC M1.11.1 */ 00432 STATIC_INLINE errnum_t window_surfaces_t__get_layer( window_surfaces_t *const self, 00433 int_fast32_t const in_LayerNum, 00434 window_surfaces_vdc5_layer_t **const out_Layer ) 00435 /* <-MISRA 16.7 */ /* <-SEC M1.11.1 */ 00436 { 00437 errnum_t e; 00438 uint_fast32_t index; 00439 00440 IF_DQ( self == NULL ) { 00441 e=E_OTHERS; 00442 goto fin; 00443 } 00444 IF_DQ( out_Layer == NULL ) { 00445 e=E_OTHERS; 00446 goto fin; 00447 } 00448 00449 index = in_LayerNum - self->layer_num_min; 00450 00451 IF ( index >= R_COUNT_OF( self->layers ) ) { 00452 e = E_OTHERS; 00453 goto fin; 00454 } 00455 00456 *out_Layer = &self->layers[ index ]; 00457 00458 e=0; 00459 fin: 00460 return e; 00461 } 00462 00463 00464 /** 00465 * @brief Get <window_surfaces_attribute_t> 00466 * 00467 * @param self window_surfaces_t 00468 * @param in_LayerNum in_LayerNum 00469 * @param out_Attribute out_Attribute 00470 * @return Error code, 0=No error 00471 */ 00472 #ifdef IS_WINDOW_SURFACES_EX 00473 STATIC_INLINE errnum_t window_surfaces_t__get_attribute( window_surfaces_t *const self, 00474 int_fast32_t const in_LayerNum, 00475 window_surfaces_attribute_t **const out_Attribute ); /* QAC-3450 */ 00476 /* ->MISRA 16.7 : "self" can not "const", because "out_Attribute" is not "const" */ 00477 /* ->SEC M1.11.1 */ 00478 STATIC_INLINE errnum_t window_surfaces_t__get_attribute( window_surfaces_t *const self, 00479 int_fast32_t const in_LayerNum, 00480 window_surfaces_attribute_t **const out_Attribute ) 00481 /* <-MISRA 16.7 */ /* <-SEC M1.11.1 */ 00482 { 00483 errnum_t e; 00484 uint_fast32_t index; 00485 00486 IF_DQ( self == NULL ) { 00487 e=E_OTHERS; 00488 goto fin; 00489 } 00490 IF_DQ( out_Attribute == NULL ) { 00491 e=E_OTHERS; 00492 goto fin; 00493 } 00494 00495 index = in_LayerNum - self->layer_num_min; 00496 00497 IF ( index >= R_COUNT_OF( self->layers ) ) { 00498 e = E_OTHERS; 00499 goto fin; 00500 } 00501 00502 *out_Attribute = &self->attributes[ index ]; 00503 00504 e=0; 00505 fin: 00506 return e; 00507 } 00508 #endif 00509 00510 00511 /*********************************************************************** 00512 * Class: window_surfaces_t 00513 ************************************************************************/ 00514 00515 /*********************************************************************** 00516 * Implement: R_WINDOW_SURFACES_InitConst 00517 ************************************************************************/ 00518 00519 static bool_t gs_window_surfaces_t_is_init = false; 00520 00521 void R_WINDOW_SURFACES_InitConst( window_surfaces_t *const self ) 00522 { 00523 int_fast32_t i; 00524 00525 IF_DQ( self == NULL ) { 00526 goto fin; 00527 } 00528 00529 for ( i = 0; i < (ssize_t) R_COUNT_OF( self->frame_buffers ); i += 1 ) { 00530 self->frame_buffers[i].buffer_address[0] = NULL; 00531 } 00532 self->is_initialized = gs_window_surfaces_t_is_init; 00533 00534 fin: 00535 return; 00536 } 00537 00538 00539 /*********************************************************************** 00540 * Implement: R_WINDOW_SURFACES_Initialize 00541 ************************************************************************/ 00542 00543 static void vdc5_init_func( uint32_t user_num ); 00544 00545 errnum_t R_WINDOW_SURFACES_Initialize( window_surfaces_t *const self, 00546 window_surfaces_config_t *in_out_config ) 00547 { 00548 errnum_t e; 00549 errnum_t ee; 00550 vdc5_error_t error_vdc; 00551 window_surfaces_config_t default_config; 00552 int_fast32_t layer_num; 00553 window_surfaces_vdc5_layer_t *main_layer[ R_COUNT_OF( self->frame_buffers ) ]; 00554 window_surfaces_vdc5_layer_t *back_layer; 00555 00556 enum { num_2 = 2, num_4 = 4 }; 00557 00558 00559 IF_DQ( self == NULL ) { 00560 e=E_OTHERS; 00561 goto fin; 00562 } 00563 00564 self->screen_channel = GS_CHANNEL_n; 00565 00566 00567 /* Set default configuration */ 00568 if ( in_out_config == NULL ) { 00569 in_out_config = &default_config; 00570 in_out_config->flags = 0; 00571 } 00572 00573 if ( IS_BIT_NOT_SET( in_out_config->flags, F_WINDOW_SURFACES_PIXEL_FORMAT ) ) { 00574 in_out_config->pixel_format = PIXEL_FORMAT_ARGB8888; 00575 in_out_config->flags |= F_WINDOW_SURFACES_PIXEL_FORMAT; 00576 } 00577 00578 00579 if ( IS_BIT_NOT_SET( in_out_config->flags, F_WINDOW_SURFACES_LAYER_COUNT ) ) { 00580 in_out_config->layer_count = 1; 00581 in_out_config->flags |= F_WINDOW_SURFACES_LAYER_COUNT; 00582 } 00583 ASSERT_D( in_out_config->layer_count >= 1 && in_out_config->layer_count <= 2, 00584 e=E_OTHERS; goto fin ); 00585 00586 00587 if ( IS_BIT_NOT_SET( in_out_config->flags, F_WINDOW_SURFACES_BUFFER_HEIGHT ) ) { 00588 in_out_config->buffer_height = R_ToSigned( GS_BUFFER_HEIGHT ); 00589 in_out_config->flags |= F_WINDOW_SURFACES_BUFFER_HEIGHT; 00590 } 00591 00592 00593 if ( IS_BIT_NOT_SET( in_out_config->flags, F_WINDOW_SURFACES_BACKGROUND_COLOR ) ) { 00594 in_out_config->background_color = GS_DEFAULT_CLEAR_COLOR; 00595 in_out_config->flags |= F_WINDOW_SURFACES_BACKGROUND_COLOR; 00596 } 00597 00598 00599 if ( IS_BIT_NOT_SET( in_out_config->flags, F_WINDOW_SURFACES_BACKGROUND_FORMAT ) ) { 00600 in_out_config->background_format = BACKGROUND_FORMAT_SOLID_COLOR; 00601 in_out_config->flags |= F_WINDOW_SURFACES_BACKGROUND_FORMAT; 00602 } 00603 00604 00605 /* Set layers */ 00606 #ifndef RZ_A1L /* RZ/A1H */ 00607 self->layer_num_min = -1; 00608 self->layer_num_max = in_out_config->layer_count - 1; 00609 ASSERT_D( (ssize_t) R_COUNT_OF( self->layers ) >= self->layer_num_max - self->layer_num_min + 1, 00610 e=E_OTHERS; goto fin ); 00611 e= window_surfaces_vdc5_layer_t__initialize( &self->layers[0], -1, VDC5_GR_TYPE_GR0 ); 00612 IF(e!=0) { 00613 goto fin; 00614 } 00615 e= window_surfaces_vdc5_layer_t__initialize( &self->layers[1], 0, VDC5_GR_TYPE_GR1 ); 00616 IF(e!=0) { 00617 goto fin; 00618 } 00619 e= window_surfaces_vdc5_layer_t__initialize( &self->layers[2], 1, VDC5_GR_TYPE_GR2 ); 00620 IF(e!=0) { 00621 goto fin; 00622 } 00623 #else 00624 if ( in_out_config->pixel_format != PIXEL_FORMAT_YUV422 ) { 00625 self->layer_num_min = -1; 00626 self->layer_num_max = in_out_config->layer_count - 1; 00627 ASSERT_D( R_COUNT_OF( self->layers ) >= self->layer_num_max - self->layer_num_min + 1, 00628 e=E_OTHERS; goto fin ); 00629 e= window_surfaces_vdc5_layer_t__initialize( &self->layers[0], -1, VDC5_GR_TYPE_GR0 ); 00630 IF(e!=0) { 00631 goto fin; 00632 } 00633 e= window_surfaces_vdc5_layer_t__initialize( &self->layers[1], 0, VDC5_GR_TYPE_GR2 ); 00634 IF(e!=0) { 00635 goto fin; 00636 } 00637 e= window_surfaces_vdc5_layer_t__initialize( &self->layers[2], 1, VDC5_GR_TYPE_GR3 ); 00638 IF(e!=0) { 00639 goto fin; 00640 } 00641 } else { /* PIXEL_FORMAT_YUV422 */ 00642 self->layer_num_min = 0; 00643 self->layer_num_max = in_out_config->layer_count - 1; 00644 ASSERT_D( R_COUNT_OF( self->layers ) >= self->layer_num_max - self->layer_num_min + 1, 00645 e=E_OTHERS; goto fin ); 00646 e= window_surfaces_vdc5_layer_t__initialize( &self->layers[0], 0, VDC5_GR_TYPE_GR0 ); 00647 IF(e!=0) { 00648 goto fin; 00649 } 00650 e= window_surfaces_vdc5_layer_t__initialize( &self->layers[1], 1, VDC5_GR_TYPE_GR2 ); 00651 IF(e!=0) { 00652 goto fin; 00653 } 00654 } 00655 #endif 00656 00657 00658 /* Set "main_layer", "back_layer" */ 00659 for ( layer_num = 0; layer_num <= self->layer_num_max; layer_num += 1 ) { 00660 e= window_surfaces_t__get_layer( self, layer_num, &main_layer[ layer_num ] ); 00661 IF(e!=0) { 00662 goto fin; 00663 } 00664 IF_DQ( main_layer[ layer_num ] == NULL ) { 00665 e=E_OTHERS; 00666 goto fin; 00667 } 00668 } 00669 00670 self->background_format = in_out_config->background_format; 00671 if ( self->layer_num_min >= 0 ) { 00672 back_layer = NULL; 00673 self->background_frame_count = 0; 00674 } else { 00675 back_layer = NULL; 00676 /* NULL is for avoiding warning C417W of mbed cloud compiler */ 00677 e= window_surfaces_t__get_layer( self, -1, &back_layer ); 00678 IF(e!=0) { 00679 goto fin; 00680 } 00681 IF_DQ( back_layer == NULL ) { 00682 e=E_OTHERS; 00683 goto fin; 00684 } 00685 00686 if ( in_out_config->background_format != BACKGROUND_FORMAT_SOLID_COLOR ) { 00687 self->background_frame_count = 1; 00688 } else { 00689 self->background_frame_count = 0; 00690 } 00691 } 00692 00693 00694 { 00695 uint8_t *memory_address; 00696 size_t memory_size; 00697 00698 00699 /* Set "physical_address", ... */ 00700 e= R_Sample_GetBigMemory( &memory_address, &memory_size ); 00701 IF ( e != 0 ) { 00702 goto fin; 00703 } 00704 /* 64byte alignment */ 00705 ASSERT_R( R_Mod_64u( (uintptr_t) memory_address ) == 0, e=E_OTHERS; goto fin ); 00706 00707 00708 /* Set "self->start_of_VRAM", ... */ 00709 self->start_of_VRAM = memory_address; 00710 /* ->MISRA 17.4 */ /* ->SEC R1.3.1 (1) */ 00711 self->over_of_VRAM = self->start_of_VRAM + memory_size; /* MISRA 17.4: This is a bound */ 00712 /* <-MISRA 17.4 */ /* <-SEC R1.3.1 (1) */ 00713 self->stack_pointer_of_VRAM = self->start_of_VRAM; 00714 } 00715 00716 00717 for ( layer_num = -self->background_frame_count; layer_num <= self->layer_num_max; layer_num += 1 ) { 00718 frame_buffer_t *frame; 00719 window_surfaces_vdc5_layer_t *layer = main_layer[ layer_num ]; 00720 pixel_format_t pixel_format; 00721 00722 e= R_WINDOW_SURFACES_GetLayerFrameBuffer( self, layer_num, &frame ); 00723 IF(e) { 00724 goto fin; 00725 } 00726 00727 if ( layer_num <= -1 && in_out_config->background_format != BACKGROUND_FORMAT_SOLID_COLOR ) { 00728 pixel_format = PIXEL_FORMAT_YUV422; 00729 } else if ( layer_num >= 1 && in_out_config->pixel_format == PIXEL_FORMAT_YUV422 ) { 00730 pixel_format = PIXEL_FORMAT_ARGB4444; 00731 } else { 00732 pixel_format = in_out_config->pixel_format; 00733 } 00734 00735 00736 /* Set "self->frame_buffers" */ 00737 frame->buffer_count = num_2; 00738 frame->show_buffer_index = 0; 00739 frame->draw_buffer_index = 0; 00740 frame->width = R_ToSigned( GS_BUFFER_WIDTH ); 00741 frame->height = in_out_config->buffer_height; 00742 frame->pixel_format = pixel_format; 00743 frame->delegate = NULL; 00744 00745 switch ( frame->pixel_format ) { 00746 case PIXEL_FORMAT_ARGB8888: 00747 frame->buffer_count = 1; 00748 frame->draw_buffer_index = 0; 00749 frame->byte_per_pixel = num_4; 00750 layer->vdc5_format = VDC5_GR_FORMAT_ARGB8888; 00751 break; 00752 00753 case PIXEL_FORMAT_XRGB8888: 00754 frame->buffer_count = 1; 00755 frame->draw_buffer_index = 0; 00756 frame->byte_per_pixel = num_4; 00757 layer->vdc5_format = VDC5_GR_FORMAT_RGB888; 00758 break; 00759 00760 case PIXEL_FORMAT_RGB565: 00761 frame->buffer_count = num_2; 00762 frame->draw_buffer_index = 1; 00763 frame->byte_per_pixel = num_2; 00764 layer->vdc5_format = VDC5_GR_FORMAT_RGB565; 00765 break; 00766 00767 case PIXEL_FORMAT_ARGB1555: 00768 frame->buffer_count = num_2; 00769 frame->draw_buffer_index = 1; 00770 frame->byte_per_pixel = num_2; 00771 layer->vdc5_format = VDC5_GR_FORMAT_ARGB1555; 00772 break; 00773 00774 case PIXEL_FORMAT_ARGB4444: 00775 frame->buffer_count = num_2; 00776 frame->draw_buffer_index = 1; 00777 frame->byte_per_pixel = num_2; 00778 layer->vdc5_format = VDC5_GR_FORMAT_ARGB4444; 00779 break; 00780 00781 case PIXEL_FORMAT_YUV422: 00782 frame->buffer_count = num_2; 00783 frame->draw_buffer_index = 1; 00784 frame->byte_per_pixel = num_2; 00785 layer->vdc5_format = VDC5_GR_FORMAT_YCBCR422; 00786 break; 00787 00788 case PIXEL_FORMAT_CLUT8: 00789 frame->buffer_count = 2; 00790 frame->draw_buffer_index = 1; 00791 frame->byte_per_pixel = R_RGA_BitPerPixelType_To_BytePerPixelType( 8 ); 00792 layer->vdc5_format = VDC5_GR_FORMAT_CLUT8; 00793 break; 00794 00795 case PIXEL_FORMAT_CLUT4: 00796 frame->buffer_count = 2; 00797 frame->draw_buffer_index = 1; 00798 frame->byte_per_pixel = R_RGA_BitPerPixelType_To_BytePerPixelType( 4 ); 00799 layer->vdc5_format = VDC5_GR_FORMAT_CLUT4; 00800 break; 00801 00802 case PIXEL_FORMAT_CLUT1: 00803 frame->buffer_count = 2; 00804 frame->draw_buffer_index = 1; 00805 frame->byte_per_pixel = R_RGA_BitPerPixelType_To_BytePerPixelType( 1 ); 00806 layer->vdc5_format = VDC5_GR_FORMAT_CLUT1; 00807 break; 00808 00809 default: 00810 layer->vdc5_format = VDC5_GR_FORMAT_NUM; /* dummy data */ 00811 break; 00812 } 00813 if( R_BYTE_PER_PIXEL_IsInteger( frame->byte_per_pixel ) ) { 00814 frame->stride = frame->width * frame->byte_per_pixel; 00815 } else { 00816 frame->stride = R_Ceil_8s( frame->width * 00817 R_RGA_BytePerPixelType_To_BitPerPixelType( frame->byte_per_pixel ) ) / 8; 00818 frame->stride = R_Ceil_32s( frame->stride ); 00819 } 00820 e= alloc_VRAM_stack_sub( &self->stack_pointer_of_VRAM, 00821 self->over_of_VRAM, frame ); 00822 IF(e!=0) { 00823 goto fin; 00824 } 00825 00826 #ifndef R_OSPL_NDEBUG 00827 printf( "Screen %dx%dx%dx%d vdc5_format=%d stride=%d \n address[0]=0x%08X address[1]=0x%08X\n", 00828 frame->buffer_count, frame->width, frame->height, frame->byte_per_pixel, 00829 layer->vdc5_format, frame->stride, 00830 (uintptr_t) frame->buffer_address[0], (uintptr_t) frame->buffer_address[1] ); 00831 /* Cast of "uintptr_t" is for avoiding "format" warning of GNU_ARM */ 00832 #endif 00833 } 00834 00835 00836 if ( ! self->is_initialized ) { 00837 00838 /* Call "R_VDC5_Initialize" */ 00839 { 00840 vdc5_init_t init; 00841 00842 init.panel_icksel = GS_LCD_CHn_PANEL_CLK; 00843 init.panel_dcdr = GS_LCD_CHn_PANEL_CLK_DIV; 00844 init.lvds = GRAPHICS_GetLvdsParam( self->screen_channel ); 00845 00846 error_vdc = R_VDC5_Initialize( self->screen_channel, &init, 00847 &vdc5_init_func, (uint32_t) self->screen_channel ); 00848 IF ( error_vdc != VDC5_OK ) { 00849 e = E_OTHERS; 00850 goto fin; 00851 } 00852 } 00853 00854 00855 /* Call "R_VDC5_SyncControl" */ 00856 { 00857 vdc5_sync_ctrl_t sync_ctrl; 00858 00859 sync_ctrl.res_vs_sel = VDC5_ON; /* Free-running Vsync ON/OFF */ 00860 sync_ctrl.res_vs_in_sel = VDC5_RES_VS_IN_SEL_SC0; /* SC_RES_VS_IN_SEL */ 00861 sync_ctrl.res_fv = (uint16_t)GS_LCD_CHn_SIG_FV; /* Free-running Vsync period setting */ 00862 sync_ctrl.res_fh = (uint16_t)GS_LCD_CHn_SIG_FH; /* Hsync period setting */ 00863 sync_ctrl.res_vsdly = (uint16_t)0u; /* Vsync signal delay control */ 00864 /* Full-screen enable control */ 00865 sync_ctrl.res_f.vs = (uint16_t)GS_LCD_CHn_DISP_VS; 00866 sync_ctrl.res_f.vw = (uint16_t)GS_LCD_CHn_DISP_VW; 00867 sync_ctrl.res_f.hs = (uint16_t)GS_LCD_CHn_DISP_HS; 00868 sync_ctrl.res_f.hw = (uint16_t)GS_LCD_CHn_DISP_HW; 00869 sync_ctrl.vsync_cpmpe = NULL; /* Vsync signal compensation */ 00870 00871 error_vdc = R_VDC5_SyncControl( self->screen_channel, &sync_ctrl ); 00872 IF ( error_vdc != VDC5_OK ) { 00873 e = E_OTHERS; 00874 goto fin; 00875 } 00876 } 00877 00878 00879 /* Call "R_VDC5_DisplayOutput" */ 00880 { 00881 vdc5_output_t output; 00882 argb8888_t background_color_; /* _ is for MISRA 5.6 */ 00883 00884 /* ->QAC 3198 */ 00885 background_color_.Value = DUMMY_INITIAL_VALUE; /* for avoid ARMCC warning C4017W */ 00886 /* <-QAC 3198 */ 00887 background_color_.u.Alpha = 0; 00888 background_color_.u.Red = in_out_config->background_color.u.Red; 00889 background_color_.u.Green = in_out_config->background_color.u.Green; 00890 background_color_.u.Blue = in_out_config->background_color.u.Blue; 00891 00892 00893 output.tcon_half = (uint16_t)GS_LCD_CHn_TCON_HALF; /* TCON reference timing, 1/2fH timing */ 00894 output.tcon_offset = (uint16_t)GS_LCD_CHn_TCON_OFFSET;/* TCON reference timing, offset Hsync signal timing */ 00895 /* LCD TCON timing setting */ 00896 GRAPHICS_SetLcdTconSettings( self->screen_channel, output.outctrl ); 00897 output.outcnt_lcd_edge = GS_LCD_CHn_OUT_EDGE; /* Output phase control of LCD_DATA23 to LCD_DATA0 pin */ 00898 output.out_endian_on = VDC5_OFF; /* Bit endian change ON/OFF control */ 00899 output.out_swap_on = VDC5_OFF; /* B/R signal swap ON/OFF control */ 00900 output.out_format = GS_LCD_CHn_OUT_FORMAT; /* LCD output format select */ 00901 output.out_frq_sel = VDC5_LCD_PARALLEL_CLKFRQ_1; /* Clock frequency control */ 00902 output.out_dir_sel = VDC5_LCD_SERIAL_SCAN_FORWARD; /* Scan direction select */ 00903 output.out_phase = VDC5_LCD_SERIAL_CLKPHASE_0; /* Clock phase adjustment */ 00904 output.bg_color = background_color_.Value; 00905 00906 error_vdc = R_VDC5_DisplayOutput( self->screen_channel, &output ); 00907 IF ( error_vdc != VDC5_OK ) { 00908 e = E_OTHERS; 00909 goto fin; 00910 } 00911 00912 if ( back_layer != NULL ) { 00913 back_layer->layer_color = in_out_config->background_color; 00914 } 00915 } 00916 00917 self->is_initialized = true; 00918 } 00919 00920 00921 /* Call "R_VDC5_ReadDataControl" */ 00922 { 00923 vdc5_read_t read; 00924 vdc5_width_read_fb_t read_area; 00925 00926 00927 for ( layer_num = 0; layer_num <= self->layer_num_max; layer_num += 1 ) { 00928 int_fast32_t bit_per_pixel; 00929 frame_buffer_t *frame; 00930 window_surfaces_vdc5_layer_t *layer = main_layer[ layer_num ]; 00931 00932 e= R_WINDOW_SURFACES_GetLayerFrameBuffer( self, layer_num, &frame ); 00933 IF(e) { 00934 goto fin; 00935 } 00936 00937 00938 /* Read data parameter */ 00939 read.gr_ln_off_dir = VDC5_GR_LN_OFF_DIR_INC; /* Line offset address direction of the frame buffer */ 00940 read.gr_flm_sel = VDC5_GR_FLM_SEL_FLM_NUM; /* Selects a frame buffer address setting signal */ 00941 read.gr_imr_flm_inv = VDC5_OFF; /* Sets the frame buffer number for distortion correction */ 00942 read.gr_bst_md = VDC5_BST_MD_32BYTE; /* Frame buffer burst transfer mode */ 00943 /* ->QAC 0306 */ 00944 read.gr_base = (void *) frame->buffer_address[ frame->show_buffer_index ]; /* Frame buffer base address */ 00945 /* <-QAC 0306 */ 00946 read.gr_ln_off = (uint32_t) frame->stride; /* Frame buffer line offset address */ 00947 read.width_read_fb = NULL; /* width of the image read from frame buffer */ 00948 read.adj_sel = VDC5_OFF; /* Measures to decrease the influence 00949 by folding pixels/lines (ON/OFF) */ 00950 read.gr_format = layer->vdc5_format; /* Graphics format of the frame buffer read signal */ 00951 read.gr_ycc_swap = VDC5_GR_YCCSWAP_Y1CRY0CB; 00952 /* Controls swapping of data read from buffer in the YCbCr422 format */ 00953 00954 bit_per_pixel = R_RGA_BytePerPixelType_To_BitPerPixelType( frame->byte_per_pixel ); 00955 if ( (bit_per_pixel == 32) || (frame->pixel_format == PIXEL_FORMAT_YCbCr422) ) { 00956 read.gr_rdswa = VDC5_WR_RD_WRSWA_32BIT; 00957 } else if ( bit_per_pixel == 16 ) { 00958 read.gr_rdswa = VDC5_WR_RD_WRSWA_32_16BIT; 00959 } else if ( bit_per_pixel <= 8 ) { 00960 read.gr_rdswa = VDC5_WR_RD_WRSWA_32_16_8BIT; 00961 } 00962 /* Display area */ 00963 read.gr_grc.hs = (uint16_t) GS_LCD_CHn_DISP_HS; 00964 read.gr_grc.hw = (uint16_t) GS_LCD_CHn_DISP_HW; 00965 read.gr_grc.vs = (uint16_t) GS_LCD_CHn_DISP_VS; 00966 if ( GS_BUFFER_HEIGHT < GS_LCD_CHn_DISP_VW ) { 00967 read.gr_grc.vw = (uint16_t) GS_BUFFER_HEIGHT; 00968 } else { 00969 read.gr_grc.vw = (uint16_t) GS_LCD_CHn_DISP_VW; 00970 } 00971 00972 error_vdc = R_VDC5_ReadDataControl( self->screen_channel, layer->data_control_ID, &read ); 00973 IF ( error_vdc != VDC5_OK ) { 00974 e = E_OTHERS; 00975 goto fin; 00976 } 00977 layer->is_data_control = true; 00978 } 00979 00980 if ( back_layer != NULL ) { 00981 if ( self->background_format == BACKGROUND_FORMAT_SOLID_COLOR ) { 00982 00983 /* Set display area for back ground */ 00984 /* "read.gr_base", ... are ignored */ 00985 00986 error_vdc = R_VDC5_ReadDataControl( self->screen_channel, back_layer->data_control_ID, &read ); 00987 IF ( error_vdc != VDC5_OK ) { 00988 e = E_OTHERS; 00989 goto fin; 00990 } 00991 } else { 00992 frame_buffer_t *frame; 00993 window_surfaces_vdc5_layer_t *layer = back_layer; 00994 00995 e= R_WINDOW_SURFACES_GetLayerFrameBuffer( self, -1, &frame ); 00996 IF(e) { 00997 goto fin; 00998 } 00999 01000 01001 /* Read data parameter */ 01002 read.gr_ln_off_dir = VDC5_GR_LN_OFF_DIR_INC; /* Line offset address direction of the frame buffer */ 01003 read.gr_flm_sel = VDC5_GR_FLM_SEL_FLM_NUM; /* Selects a frame buffer address setting signal */ 01004 read.gr_imr_flm_inv = VDC5_OFF; /* Sets the frame buffer number for distortion correction */ 01005 read.gr_bst_md = VDC5_BST_MD_32BYTE; /* Frame buffer burst transfer mode */ 01006 /* ->QAC 0306 */ 01007 read.gr_base = (void *) frame->buffer_address[ frame->show_buffer_index ]; /* Frame buffer base address */ 01008 /* <-QAC 0306 */ 01009 read.gr_ln_off = (uint32_t) frame->stride; /* Frame buffer line offset address */ 01010 read_area.in_hw = frame->width; 01011 read_area.in_vw = frame->height / 2; 01012 read.width_read_fb = &read_area; 01013 read.adj_sel = VDC5_ON; 01014 read.gr_format = layer->vdc5_format; /* Graphics format of the frame buffer read signal */ 01015 read.gr_ycc_swap = VDC5_GR_YCCSWAP_CBY0CRY1; 01016 /* Controls swapping of data read from buffer in the YCbCr422 format */ 01017 read.gr_rdswa = VDC5_WR_RD_WRSWA_16BIT; 01018 01019 /* Display area */ 01020 read.gr_grc.hs = (uint16_t) GS_LCD_CHn_DISP_HS; 01021 read.gr_grc.hw = (uint16_t) GS_LCD_CHn_DISP_HW; 01022 read.gr_grc.vs = (uint16_t) GS_LCD_CHn_DISP_VS; 01023 if ( GS_BUFFER_HEIGHT < GS_LCD_CHn_DISP_VW ) { 01024 read.gr_grc.vw = (uint16_t) GS_BUFFER_HEIGHT; 01025 } else { 01026 read.gr_grc.vw = (uint16_t) GS_LCD_CHn_DISP_VW; 01027 } 01028 01029 error_vdc = R_VDC5_ReadDataControl( self->screen_channel, layer->data_control_ID, &read ); 01030 IF ( error_vdc != VDC5_OK ) { 01031 e = E_OTHERS; 01032 goto fin; 01033 } 01034 } 01035 back_layer->is_data_control = true; 01036 } 01037 } 01038 01039 01040 /* Call "R_VDC5_AlphaBlending" for ARGB1555 */ 01041 for ( layer_num = 0; layer_num <= self->layer_num_max; layer_num += 1 ) { 01042 frame_buffer_t *frame; 01043 01044 e= R_WINDOW_SURFACES_GetLayerFrameBuffer( self, layer_num, &frame ); 01045 IF(e) { 01046 goto fin; 01047 } 01048 01049 if ( frame->pixel_format == PIXEL_FORMAT_ARGB1555 ) { 01050 static vdc5_alpha_argb1555_t alpha_for_argb1555 = { 0x00, U8_255 }; 01051 static vdc5_alpha_blending_t blend = { &alpha_for_argb1555, NULL }; 01052 01053 error_vdc = R_VDC5_AlphaBlending( self->screen_channel, 01054 main_layer[ layer_num ]->data_control_ID, 01055 &blend ); 01056 IF( error_vdc != VDC5_OK ) { 01057 e=E_OTHERS; 01058 goto fin; 01059 } 01060 } 01061 } 01062 01063 01064 /* Call "R_V_SYNC_Initialize" */ 01065 e= R_V_SYNC_Initialize( (int_fast32_t) self->screen_channel ); 01066 IF ( e != 0 ) { 01067 goto fin; 01068 } 01069 01070 01071 /* Wait for avoiding LCD filled white (LCD-KIT-B01) */ 01072 e= R_OSPL_Delay( 80 ); 01073 IF(e) { 01074 goto fin; 01075 } 01076 01077 01078 /* Set graphics display mode */ 01079 { 01080 vdc5_start_t start; 01081 int_fast32_t i; 01082 01083 for ( i = 0; i < (int_fast32_t) R_COUNT_OF( self->vdc5_disp_sel_array ); i += 1 ) { 01084 self->vdc5_disp_sel_array[ i ] = VDC5_DISPSEL_LOWER; /* Reset */ 01085 } 01086 self->vdc5_disp_sel_array[ VDC5_GR_TYPE_GR0 ] = VDC5_DISPSEL_BACK; 01087 01088 start.gr_disp_sel = self->vdc5_disp_sel_array; 01089 error_vdc = R_VDC5_StartProcess( self->screen_channel, VDC5_LAYER_ID_ALL, &start ); 01090 IF ( error_vdc != VDC5_OK ) { 01091 e = E_OTHERS; 01092 goto fin; 01093 } 01094 } 01095 01096 #if USE_LCD 01097 R_OSPL_Delay( 2*17 ); /* Synchronize to LCD */ 01098 LCD_SetBacklight( 100 ); 01099 #endif 01100 01101 01102 /* Set "self->attributes" */ 01103 for ( layer_num = self->layer_num_min; layer_num <= self->layer_num_max; layer_num += 1 ) { 01104 window_surfaces_attribute_t *attribute = NULL; 01105 /* NULL is for avoiding warning C417W of mbed cloud compiler */ 01106 01107 e= window_surfaces_t__get_attribute( self, layer_num, &attribute ); 01108 IF(e) { 01109 goto fin; 01110 } 01111 01112 attribute->X = 0; 01113 attribute->Y = 0; 01114 attribute->Width = R_ToSigned( GS_BUFFER_WIDTH ); 01115 attribute->Height = in_out_config->buffer_height; 01116 attribute->OffsetX = 0; 01117 attribute->OffsetY = 0; 01118 attribute->OffsetByte = 0; 01119 } 01120 01121 01122 e=0; 01123 fin: 01124 if ( e != 0 ) { 01125 if ( self != NULL ) { 01126 ee= R_WINDOW_SURFACES_Finalize( self, e ); 01127 R_UNREFERENCED_VARIABLE( ee ); 01128 } 01129 } 01130 return e; 01131 } 01132 01133 01134 /** 01135 * @brief vdc5_init_func 01136 * 01137 * @param user_num channel 01138 * @return None 01139 */ 01140 static void vdc5_init_func( uint32_t const user_num ) 01141 { 01142 vdc5_channel_t channel; 01143 struct st_cpg *const reg_CPG = R_Get_CPG_Base(); 01144 01145 IF_DQ( reg_CPG == NULL ) { 01146 goto fin; 01147 } 01148 01149 channel = (vdc5_channel_t)user_num; 01150 if (channel == VDC5_CHANNEL_0) { 01151 /* Standby control register 9 (STBCR9) 01152 b1 ------0-; MSTP91 : 0 : Video display controller channel 0 & LVDS enable */ 01153 R_DRV_SET_REGISTER_BIT_FIELD( ®_CPG->STBCR9, STBCR9, MSTP91, false ); 01154 } else { 01155 /* Standby control register 9 (STBCR9) 01156 b1 ------0-; MSTP91 : 0 : Video display controller channel 0 & LVDS enable 01157 b0 -------0; MSTP90 : 0 : Video display controller channel 1 enable */ 01158 R_DRV_SET_REGISTER_BIT_FIELD( ®_CPG->STBCR9, STBCR9, MSTP91, false ); 01159 R_DRV_SET_REGISTER_BIT_FIELD( ®_CPG->STBCR9, STBCR9, MSTP90, false ); 01160 } 01161 GRAPHICS_SetLcdPanel(channel); 01162 01163 fin: 01164 return; 01165 } 01166 01167 01168 /*********************************************************************** 01169 * Implement: R_WINDOW_SURFACES_Finalize 01170 ************************************************************************/ 01171 01172 static void vdc5_quit_func( uint32_t const user_num ); 01173 01174 errnum_t R_WINDOW_SURFACES_Finalize( window_surfaces_t *const self, errnum_t e ) 01175 { 01176 vdc5_error_t error_vdc; 01177 01178 IF_DQ( self == NULL ) { 01179 e=E_OTHERS; 01180 goto fin; 01181 } 01182 01183 e= R_V_SYNC_Finalize( (int_fast32_t) self->screen_channel, e ); 01184 01185 if ( ! self->is_initialized ) { 01186 error_vdc = R_VDC5_Terminate( self->screen_channel, &vdc5_quit_func, 01187 (uint32_t) self->screen_channel ); 01188 IF ( (error_vdc != VDC5_OK) && (e == 0) ) { 01189 e = E_OTHERS; 01190 } 01191 } else { 01192 int_fast32_t i; 01193 01194 for ( i = -self->background_frame_count; i <= self->layer_num_max; i += 1 ) { 01195 frame_buffer_t *frame; 01196 errnum_t ee; 01197 01198 ee= R_WINDOW_SURFACES_GetLayerFrameBuffer( self, i, &frame ); 01199 e= R_OSPL_MergeErrNum( e, ee ); 01200 frame->buffer_address[0] = NULL; 01201 } 01202 01203 for ( i = self->layer_num_min; i <= self->layer_num_max; i += 1 ) { 01204 window_surfaces_vdc5_layer_t *layer = NULL; 01205 /* NULL is for avoiding warning C417W of mbed cloud compiler */ 01206 errnum_t ee = window_surfaces_t__get_layer( self, i, &layer ); 01207 01208 IF_DQ ( layer == NULL ) { 01209 e=E_OTHERS; /* Same check as "ee" */ 01210 goto fin; 01211 } 01212 01213 if ( (ee == 0) && (layer->is_data_control) ) { 01214 error_vdc = R_VDC5_StopProcess( self->screen_channel, 01215 layer->data_control_ID ); 01216 IF ( (error_vdc != VDC5_OK) && (e == 0) ) { 01217 e = E_OTHERS; 01218 } 01219 error_vdc = R_VDC5_ReleaseDataControl( self->screen_channel, 01220 layer->data_control_ID ); 01221 layer->is_data_control = false; 01222 IF ( (error_vdc != VDC5_OK) && (e == 0) ) { 01223 e = E_OTHERS; 01224 } 01225 } 01226 } 01227 01228 #define R_WINDOW_SURFACES_TERMINATE_VDC5 1 /* 0 or 1 */ 01229 #if R_WINDOW_SURFACES_TERMINATE_VDC5 01230 self->is_initialized = false; 01231 01232 error_vdc = R_VDC5_Terminate( self->screen_channel, &vdc5_quit_func, 01233 (uint32_t) self->screen_channel ); 01234 IF ( (error_vdc != VDC5_OK) && (e == 0) ) { 01235 e = E_OTHERS; 01236 } 01237 #endif 01238 } 01239 01240 gs_window_surfaces_t_is_init = self->is_initialized; 01241 01242 fin: 01243 return e; 01244 } 01245 01246 01247 /** 01248 * @brief vdc5_quit_func 01249 * 01250 * @param user_num channel 01251 * @return None 01252 */ 01253 static void vdc5_quit_func( uint32_t const user_num ) 01254 { 01255 vdc5_channel_t const channel = (vdc5_channel_t) user_num; 01256 struct st_cpg *const reg_CPG = R_Get_CPG_Base(); 01257 01258 IF_DQ( reg_CPG == NULL ) { 01259 goto fin; 01260 } 01261 01262 if (channel == VDC5_CHANNEL_0) { 01263 /* If LVDS should remain enabled, the following code should be removed. */ 01264 R_DRV_SET_REGISTER_BIT_FIELD( ®_CPG->STBCR9, STBCR9, MSTP91, true ); 01265 } else { 01266 R_DRV_SET_REGISTER_BIT_FIELD( ®_CPG->STBCR9, STBCR9, MSTP90, true ); 01267 } 01268 01269 fin: 01270 return; 01271 } 01272 01273 01274 /*********************************************************************** 01275 * Implement: R_WINDOW_SURFACES_GetLayerFrameBuffer 01276 ************************************************************************/ 01277 errnum_t R_WINDOW_SURFACES_GetLayerFrameBuffer( const window_surfaces_t *const self, 01278 int_fast32_t const layer_num, frame_buffer_t **const out_frame_buffer ) 01279 { 01280 errnum_t e; 01281 uint_fast32_t index; 01282 01283 IF_DQ( out_frame_buffer == NULL ) { 01284 e=E_OTHERS; 01285 goto fin; 01286 } 01287 IF_DQ( self == NULL ) { 01288 e=E_OTHERS; 01289 goto fin; 01290 } 01291 01292 index = layer_num + self->background_frame_count; 01293 IF ( index >= R_COUNT_OF( self->frame_buffers ) ) { 01294 e = E_OTHERS; 01295 goto fin; 01296 } 01297 01298 *out_frame_buffer = (frame_buffer_t *) &self->frame_buffers[ index ]; 01299 /* Cast is for const */ 01300 01301 e=0; 01302 fin: 01303 return e; 01304 } 01305 01306 01307 /*********************************************************************** 01308 * Implement: R_WINDOW_SURFACES_SwapBuffers 01309 ************************************************************************/ 01310 errnum_t R_WINDOW_SURFACES_SwapBuffers( window_surfaces_t *const self, 01311 int_fast32_t const layer_num, graphics_t *const context ) 01312 { 01313 errnum_t e; 01314 #if GS_MEASURE_GPU_LOAD 01315 enum { interval = 60 }; 01316 uint32_t idle_start_time; 01317 uint32_t idle_end_time; 01318 static uint32_t skip_count; 01319 static uint32_t previous_idle_end_time; 01320 r_ospl_ftimer_spec_t ts; 01321 #endif 01322 01323 01324 e= R_GRAPHICS_Finish( context ); 01325 IF(e!=0) { 01326 goto fin; 01327 } 01328 01329 01330 #if GS_MEASURE_GPU_LOAD 01331 e= R_OSPL_FTIMER_InitializeIfNot( &ts ); 01332 IF(e) { 01333 goto fin; 01334 } 01335 idle_start_time = R_OSPL_FTIMER_Get(); 01336 #endif 01337 01338 01339 e= R_WINDOW_SURFACES_SwapBuffers_Sub( self, layer_num ); 01340 IF(e!=0) { 01341 goto fin; 01342 } 01343 01344 01345 e= R_V_SYNC_Wait( self->screen_channel, 1, true ); 01346 IF(e!=0) { 01347 goto fin; 01348 } 01349 01350 01351 #if GS_MEASURE_GPU_LOAD 01352 idle_end_time = R_OSPL_FTIMER_Get(); 01353 if ( previous_idle_end_time != 0 ) { /* Skip at first */ 01354 skip_count += 1; 01355 if ( skip_count >= interval ) { 01356 printf( "GPU %d%% in %dmsec\n", 01357 ( idle_start_time - previous_idle_end_time ) * 100 / 01358 ( idle_end_time - previous_idle_end_time ), 01359 R_OSPL_FTIMER_CountToTime( &ts, 01360 idle_end_time - previous_idle_end_time ) ); 01361 skip_count = 0; 01362 01363 /* __heapstats( (__heapprt) fprintf, stdout ); */ 01364 01365 /* R_DEBUG_BREAK(); */ /* This is for measuring CPU load */ 01366 } 01367 } 01368 previous_idle_end_time = idle_end_time; 01369 #endif 01370 01371 01372 e=0; 01373 fin: 01374 return e; 01375 } 01376 01377 01378 /*********************************************************************** 01379 * Implement: R_WINDOW_SURFACES_SwapBuffersStart 01380 ************************************************************************/ 01381 errnum_t R_WINDOW_SURFACES_SwapBuffersStart( window_surfaces_t *const self, 01382 int_fast32_t const layer_num, r_ospl_async_t *async ) 01383 { 01384 errnum_t e; 01385 01386 01387 e= R_WINDOW_SURFACES_SwapBuffers_Sub( self, layer_num ); 01388 IF(e!=0) { 01389 goto fin; 01390 } 01391 01392 01393 e= R_V_SYNC_WaitStart( self->screen_channel, 1, true, async ); 01394 IF(e!=0) { 01395 goto fin; 01396 } 01397 01398 e=0; 01399 fin: 01400 return e; 01401 } 01402 01403 01404 /** 01405 * @brief Sub routine of <R_WINDOW_SURFACES_SwapBuffers> 01406 * 01407 * @param self window_surfaces_t 01408 * @param layer_num layer_num 01409 * @return Error code, 0=No error 01410 */ 01411 static errnum_t R_WINDOW_SURFACES_SwapBuffers_Sub( window_surfaces_t *const self, 01412 int_fast32_t const layer_num ) 01413 { 01414 errnum_t e; 01415 vdc5_error_t error_vdc; 01416 frame_buffer_t *frame; 01417 window_surfaces_vdc5_layer_t *layer = NULL; 01418 /* NULL is for avoiding warning C417W of mbed cloud compiler */ 01419 01420 01421 IF_DQ( self == NULL ) { 01422 e=E_OTHERS; 01423 goto fin; 01424 } 01425 01426 e= window_surfaces_t__get_layer( self, layer_num, &layer ); 01427 IF(e!=0) { 01428 goto fin; 01429 } 01430 IF_DQ( layer == NULL ) { 01431 e=E_OTHERS; 01432 goto fin; 01433 } 01434 01435 01436 /* Swap buffer index */ 01437 e= R_WINDOW_SURFACES_GetLayerFrameBuffer( self, layer_num, &frame ); 01438 IF(e) { 01439 goto fin; 01440 } 01441 frame->show_buffer_index = frame->draw_buffer_index; 01442 frame->draw_buffer_index += 1; 01443 if ( frame->draw_buffer_index >= (int_t) frame->buffer_count ) { 01444 frame->draw_buffer_index = 0; 01445 } 01446 01447 01448 /* Show the frame buffer */ 01449 { 01450 vdc5_read_chg_t config; 01451 uintptr_t physical_address; 01452 01453 window_surfaces_attribute_t *attribute = NULL; 01454 /* NULL is for avoiding warning C417W of mbed cloud compiler */ 01455 01456 e= R_OSPL_ToPhysicalAddress( frame->buffer_address[ frame->show_buffer_index ], 01457 &physical_address ); 01458 IF(e!=0) { 01459 goto fin; 01460 } 01461 01462 e= window_surfaces_t__get_attribute( self, layer_num, &attribute ); 01463 IF(e) { 01464 goto fin; 01465 } 01466 01467 if ( attribute->OffsetByte != GS_OFFSET_BYTE_NOT_SHOW ) { 01468 physical_address += attribute->OffsetByte; 01469 01470 config.gr_base = (void *) physical_address; 01471 config.width_read_fb = NULL; 01472 config.gr_grc = NULL; 01473 config.gr_disp_sel = NULL; 01474 error_vdc = R_VDC5_ChangeReadProcess( self->screen_channel, layer->data_control_ID, &config ); 01475 IF ( error_vdc != VDC5_OK ) { 01476 e=E_OTHERS; 01477 goto fin; 01478 } 01479 } 01480 } 01481 01482 01483 /* Show the layer */ 01484 { 01485 vdc5_gr_disp_sel_t new_value; 01486 01487 if ( layer_num == 1 ) { 01488 new_value = VDC5_DISPSEL_BLEND; 01489 } else if ( layer_num == 0 ) { 01490 frame_buffer_t *frame; 01491 01492 e= R_WINDOW_SURFACES_GetLayerFrameBuffer( self, layer_num, &frame ); 01493 IF(e) { 01494 goto fin; 01495 } 01496 01497 if ( frame->pixel_format == PIXEL_FORMAT_YUV422 ) { 01498 new_value = VDC5_DISPSEL_CURRENT; 01499 } else { 01500 new_value = VDC5_DISPSEL_BLEND; 01501 } 01502 } else { 01503 ASSERT_D( layer_num == -1, e=E_OTHERS; goto fin ); 01504 01505 if ( self->background_format == BACKGROUND_FORMAT_SOLID_COLOR ) { 01506 new_value = VDC5_DISPSEL_CURRENT; 01507 } else { 01508 new_value = VDC5_DISPSEL_LOWER; /* "*_LOWER" is "*_CURRENT" for resize */ 01509 } 01510 } 01511 01512 if ( self->vdc5_disp_sel_array[ layer->graphics_layer_ID ] != new_value ) { 01513 vdc5_start_t start; 01514 01515 self->vdc5_disp_sel_array[ layer->graphics_layer_ID ] = new_value; 01516 01517 start.gr_disp_sel = self->vdc5_disp_sel_array; 01518 error_vdc = R_VDC5_StartProcess( self->screen_channel, VDC5_LAYER_ID_ALL, &start ); 01519 IF ( error_vdc != VDC5_OK ) { 01520 e = E_OTHERS; 01521 goto fin; 01522 } 01523 } 01524 } 01525 01526 e=0; 01527 fin: 01528 return e; 01529 } 01530 01531 01532 /*********************************************************************** 01533 * Implement: R_WINDOW_SURFACES_WaitForVSync 01534 ************************************************************************/ 01535 errnum_t R_WINDOW_SURFACES_WaitForVSync( window_surfaces_t *const self, 01536 int_fast32_t const swap_interval, bool_t const is_1_v_sync_at_minimum ) 01537 { 01538 return R_V_SYNC_Wait( self->screen_channel, swap_interval, is_1_v_sync_at_minimum ); 01539 } 01540 01541 01542 /** 01543 * @brief R_WINDOW_SURFACES_AllocOffscreenStack 01544 * 01545 * @par Parameters 01546 * None 01547 * @return None. 01548 */ 01549 errnum_t R_WINDOW_SURFACES_AllocOffscreenStack( window_surfaces_t *const self, 01550 frame_buffer_t *const in_out_frame_buffer ) 01551 { 01552 errnum_t e; 01553 01554 IF_DQ( self == NULL ) { 01555 e=E_OTHERS; 01556 goto fin; 01557 } 01558 01559 e= alloc_VRAM_stack_sub( &self->stack_pointer_of_VRAM, self->over_of_VRAM, 01560 in_out_frame_buffer ); 01561 IF(e!=0) { 01562 goto fin; 01563 } 01564 01565 e=0; 01566 fin: 01567 return e; 01568 } 01569 01570 01571 /** 01572 * @brief R_WINDOW_SURFACES_FreeOffscreenStack 01573 * 01574 * @par Parameters 01575 * None 01576 * @return None. 01577 */ 01578 errnum_t R_WINDOW_SURFACES_FreeOffscreenStack( window_surfaces_t *const self, 01579 const frame_buffer_t *const frame_buffer ) 01580 { 01581 errnum_t e; 01582 01583 IF_DQ( self == NULL ) { 01584 e=E_OTHERS; 01585 goto fin; 01586 } 01587 01588 e= free_VRAM_stack_sub( &self->stack_pointer_of_VRAM, frame_buffer, 01589 self->start_of_VRAM ); 01590 IF(e!=0) { 01591 goto fin; 01592 } 01593 01594 e=0; 01595 fin: 01596 return e; 01597 } 01598 01599 01600 /*********************************************************************** 01601 * Implement: R_WINDOW_SURFACES_DoMessageLoop 01602 ************************************************************************/ 01603 errnum_t R_WINDOW_SURFACES_DoMessageLoop( window_surfaces_t *self ) 01604 { 01605 R_UNREFERENCED_VARIABLE( self ); 01606 01607 R_OSPL_Delay( 2000 ); 01608 01609 return 0; 01610 } 01611 01612 01613 /*********************************************************************** 01614 * Implement: R_WINDOW_SURFACES_AccessLayerAttributes 01615 ************************************************************************/ 01616 errnum_t R_WINDOW_SURFACES_AccessLayerAttributes( window_surfaces_t *self, 01617 layer_attributes_t *in_out_Attributes ) 01618 { 01619 errnum_t e; 01620 vdc5_error_t error_vdc; 01621 window_surfaces_vdc5_layer_t *layer = NULL; 01622 01623 if ( IS_BIT_SET( in_out_Attributes->flags, F_LAYER_ID ) ) { 01624 e= window_surfaces_t__get_layer( self, in_out_Attributes->id, &layer ); 01625 IF(e) { 01626 goto fin; 01627 } 01628 } 01629 01630 ASSERT_R( IS_ALL_BITS_NOT_SET( in_out_Attributes->access, ~ACCESS_ALL_MASK ), e=E_OTHERS; goto fin ); 01631 01632 01633 if ( in_out_Attributes->flags & F_LAYER_LAYER_COLOR ) { 01634 vdc5_output_t output; 01635 vdc5_read_t read; 01636 r8g8b8a8_t previous_layer_color = { {0,0,0,0} }; 01637 /* Avoid Warning: C4017W: previous_layer_color may be used before being set */ 01638 enum { necessary_flags = F_LAYER_ID }; 01639 01640 01641 ASSERT_R( IS_ALL_BITS_SET( in_out_Attributes->flags, necessary_flags ), 01642 e=E_OTHERS; goto fin ); 01643 ASSERT_R( IS_ALL_BITS_NOT_SET( in_out_Attributes->access, ~(ACCESS_READ | ACCESS_WRITE) ), 01644 e=E_OTHERS; goto fin ); 01645 01646 01647 if ( IS_BIT_SET( in_out_Attributes->access, ACCESS_READ ) ) { 01648 previous_layer_color = layer->layer_color; 01649 } 01650 01651 01652 if ( IS_BIT_SET( in_out_Attributes->access, ACCESS_WRITE ) ) { 01653 01654 if ( IS_BIT_SET( in_out_Attributes->flags, F_LAYER_LAYER_COLOR ) ) { 01655 01656 /* Set "output.bg_color" to "R_VDC5_DisplayOutput" */ 01657 output.tcon_half = (uint16_t)GS_LCD_CHn_TCON_HALF; /* TCON reference timing, 1/2fH timing */ 01658 output.tcon_offset = (uint16_t)GS_LCD_CHn_TCON_OFFSET;/* TCON reference timing, offset Hsync signal timing */ 01659 GRAPHICS_SetLcdTconSettings( self->screen_channel, output.outctrl ); 01660 output.outcnt_lcd_edge = GS_LCD_CHn_OUT_EDGE; /* Output phase control of LCD_DATA23 to LCD_DATA0 pin */ 01661 output.out_endian_on = VDC5_OFF; /* Bit endian change ON/OFF control */ 01662 output.out_swap_on = VDC5_OFF; /* B/R signal swap ON/OFF control */ 01663 output.out_format = GS_LCD_CHn_OUT_FORMAT; /* LCD output format select */ 01664 output.out_frq_sel = VDC5_LCD_PARALLEL_CLKFRQ_1; /* Clock frequency control */ 01665 output.out_dir_sel = VDC5_LCD_SERIAL_SCAN_FORWARD; /* Scan direction select */ 01666 output.out_phase = VDC5_LCD_SERIAL_CLKPHASE_0; /* Clock phase adjustment */ 01667 output.bg_color = 01668 ( in_out_Attributes->layer_color.u.Red << 16 ) | 01669 ( in_out_Attributes->layer_color.u.Green << 8 ) | 01670 ( in_out_Attributes->layer_color.u.Blue << 0 ); /* 24-bit RGB color format */ 01671 01672 error_vdc = R_VDC5_DisplayOutput( self->screen_channel, &output ); 01673 IF ( error_vdc != VDC5_OK ) { 01674 e = E_OTHERS; 01675 goto fin; 01676 } 01677 01678 01679 /* Update ReadDataControl */ 01680 01681 /* Release */ 01682 if ( layer->is_data_control ) { 01683 error_vdc = R_VDC5_StopProcess( self->screen_channel, layer->data_control_ID ); 01684 IF ( error_vdc != VDC5_OK ) { 01685 R_NOOP(); 01686 } 01687 error_vdc = R_VDC5_ReleaseDataControl( self->screen_channel, layer->data_control_ID ); 01688 IF ( error_vdc != VDC5_OK ) { 01689 e=E_OTHERS; 01690 goto fin; 01691 } 01692 layer->is_data_control = false; 01693 } 01694 01695 /* Read data parameter : These values are dummy */ 01696 read.gr_ln_off_dir = VDC5_GR_LN_OFF_DIR_INC; /* Line offset address direction of the frame buffer */ 01697 read.gr_flm_sel = VDC5_GR_FLM_SEL_FLM_NUM; /* Selects a frame buffer address setting signal */ 01698 read.gr_imr_flm_inv = VDC5_OFF; /* Sets the frame buffer number for distortion correction */ 01699 read.gr_bst_md = VDC5_BST_MD_32BYTE; /* Frame buffer burst transfer mode */ 01700 read.gr_base = (void *) &read; /* Dummy, Frame buffer base address */ 01701 read.gr_ln_off = 3200; /* Dummy, Frame buffer line offset address */ 01702 read.width_read_fb = NULL; /* width of the image read from frame buffer */ 01703 read.adj_sel = VDC5_OFF; /* Measures to decrease the influence 01704 by folding pixels/lines (ON/OFF) */ 01705 read.gr_format = VDC5_GR_FORMAT_RGB888; /* Graphics format of the frame buffer read signal */ 01706 read.gr_ycc_swap = VDC5_GR_YCCSWAP_Y1CRY0CB; /* Controls swapping of data read from buffer 01707 in the YCbCr422 format */ 01708 read.gr_rdswa = VDC5_WR_RD_WRSWA_32BIT; /* for 32 bit format */ 01709 01710 /* Set bakcground color area */ 01711 read.gr_grc.hs = GS_LCD_CHn_DISP_HS; 01712 read.gr_grc.hw = (uint16_t) GS_LCD_CHn_DISP_HW; 01713 read.gr_grc.vs = GS_LCD_CHn_DISP_VS; 01714 if ( GS_BUFFER_HEIGHT < GS_LCD_CHn_DISP_VW ) { 01715 read.gr_grc.vw = (uint16_t) GS_BUFFER_HEIGHT; 01716 } else { 01717 read.gr_grc.vw = GS_LCD_CHn_DISP_VW; 01718 } 01719 01720 /* Start to read again */ 01721 error_vdc = R_VDC5_ReadDataControl( self->screen_channel, layer->data_control_ID, &read ); 01722 IF ( error_vdc != VDC5_OK ) { 01723 e = E_OTHERS; 01724 goto fin; 01725 } 01726 layer->is_data_control = true; 01727 } 01728 01729 01730 /* Set graphics display mode */ 01731 { 01732 vdc5_start_t start; 01733 01734 start.gr_disp_sel = self->vdc5_disp_sel_array; 01735 error_vdc = R_VDC5_StartProcess( self->screen_channel, VDC5_LAYER_ID_ALL, &start ); 01736 IF ( error_vdc != VDC5_OK ) { 01737 e = E_OTHERS; 01738 goto fin; 01739 } 01740 } 01741 } 01742 01743 if ( IS_BIT_SET( in_out_Attributes->access, ACCESS_READ ) ) { 01744 in_out_Attributes->layer_color = previous_layer_color; 01745 } 01746 } 01747 01748 01749 /* Set window position */ 01750 { 01751 enum { flags_of_window_position = 01752 F_LAYER_X | F_LAYER_Y | F_LAYER_WIDTH | F_LAYER_HEIGHT | 01753 F_LAYER_OFFSET_X | F_LAYER_OFFSET_Y 01754 }; 01755 01756 if ( IS_ANY_BITS_SET( in_out_Attributes->flags, flags_of_window_position ) && 01757 IS_BIT_SET( in_out_Attributes->access, ACCESS_WRITE ) ) { 01758 vdc5_read_chg_t change; 01759 vdc5_width_read_fb_t change_source_rectangle; 01760 vdc5_period_rect_t change_destination_rectangle; 01761 gs_frame_width_height_t source_frame; 01762 gs_frame_width_height_t destination_frame; 01763 gs_rectangle_t source_rectangle; 01764 gs_rectangle_t destination_rectangle; 01765 frame_buffer_t *frame; 01766 bool_t is_interlace; 01767 bool_t is_solid_color; 01768 bool_t is_show = true; 01769 window_surfaces_attribute_t *attribute = NULL; 01770 /* NULL is for avoiding warning C417W of mbed cloud compiler */ 01771 01772 01773 ASSERT_R( IS_ALL_BITS_SET( in_out_Attributes->flags, F_LAYER_ID ), e=E_OTHERS; goto fin ); 01774 01775 e= window_surfaces_t__get_attribute( self, in_out_Attributes->id, &attribute ); 01776 IF(e) { 01777 goto fin; 01778 } 01779 01780 is_interlace = ( self->background_format == BACKGROUND_FORMAT_VIDEO_INTERLACE && 01781 in_out_Attributes->id == -1 ); 01782 01783 is_solid_color = ( self->background_format == BACKGROUND_FORMAT_SOLID_COLOR && 01784 in_out_Attributes->id == -1 ); 01785 01786 change.gr_base = NULL; 01787 change.width_read_fb = NULL; 01788 change.gr_grc = NULL; 01789 change.gr_disp_sel = NULL; 01790 01791 if ( IS_BIT_SET( in_out_Attributes->flags, F_LAYER_X ) ) { 01792 attribute->X = in_out_Attributes->x; 01793 change.gr_grc = &change_destination_rectangle; 01794 } 01795 if ( IS_BIT_SET( in_out_Attributes->flags, F_LAYER_Y ) ) { 01796 attribute->Y = in_out_Attributes->y; 01797 change.gr_grc = &change_destination_rectangle; 01798 } 01799 if ( IS_BIT_SET( in_out_Attributes->flags, F_LAYER_WIDTH ) ) { 01800 attribute->Width = in_out_Attributes->width; 01801 change.gr_grc = &change_destination_rectangle; 01802 } 01803 if ( IS_BIT_SET( in_out_Attributes->flags, F_LAYER_HEIGHT ) ) { 01804 attribute->Height = in_out_Attributes->height; 01805 change.gr_grc = &change_destination_rectangle; 01806 } 01807 if ( IS_BIT_SET( in_out_Attributes->flags, F_LAYER_OFFSET_X ) ) { 01808 attribute->OffsetX = in_out_Attributes->offset_x; 01809 change.gr_grc = &change_destination_rectangle; 01810 } 01811 if ( IS_BIT_SET( in_out_Attributes->flags, F_LAYER_OFFSET_Y ) ) { 01812 attribute->OffsetY = in_out_Attributes->offset_y; 01813 change.gr_grc = &change_destination_rectangle; 01814 } 01815 01816 01817 /* Call "GS_ToInFrame" */ 01818 if ( is_solid_color ) { 01819 e= R_WINDOW_SURFACES_GetLayerFrameBuffer( self, 0, &frame ); 01820 IF(e) { 01821 goto fin; 01822 } 01823 } else { 01824 e= R_WINDOW_SURFACES_GetLayerFrameBuffer( self, in_out_Attributes->id, &frame ); 01825 IF(e) { 01826 goto fin; 01827 } 01828 } 01829 source_frame.Width = frame->width; 01830 source_frame.Height = frame->height; 01831 destination_frame.Width = frame->width; 01832 destination_frame.Height = frame->height; 01833 source_rectangle.Left = attribute->OffsetX; 01834 source_rectangle.Top = attribute->OffsetY; 01835 source_rectangle.Right = attribute->OffsetX + attribute->Width; 01836 source_rectangle.Bottom = attribute->OffsetY + attribute->Height; 01837 destination_rectangle.Left = attribute->X; 01838 destination_rectangle.Top = attribute->Y; 01839 destination_rectangle.Right = attribute->X + attribute->Width; 01840 destination_rectangle.Bottom = attribute->Y + attribute->Height; 01841 01842 e= GS_ToInFrame( &source_frame, &destination_frame, 01843 &source_rectangle, &destination_rectangle ); 01844 IF(e) { 01845 goto fin; 01846 } 01847 if ( source_rectangle.Left > source_rectangle.Right ) { 01848 is_show = false; 01849 } 01850 01851 01852 /* Call "R_VDC5_ChangeReadProcess" */ 01853 if ( is_show ) { 01854 byte_t *source_address; 01855 int_fast32_t offset_byte; 01856 01857 ASSERT_R( ! is_solid_color, e=E_OTHERS; goto fin ); 01858 01859 if ( is_interlace ) { 01860 source_address = frame->buffer_address[0]; 01861 offset_byte = ( source_rectangle.Top / 2 ) * frame->stride; 01862 } else { 01863 source_address = frame->buffer_address[ frame->show_buffer_index ]; 01864 offset_byte = source_rectangle.Top * frame->stride; 01865 } 01866 offset_byte += source_rectangle.Left * frame->byte_per_pixel; 01867 ASSERT_R( R_BYTE_PER_PIXEL_IsInteger( frame->byte_per_pixel ), 01868 e=E_OTHERS; goto fin ); 01869 attribute->OffsetByte = offset_byte; 01870 01871 change.gr_base = source_address + attribute->OffsetByte; 01872 } 01873 if ( change.gr_grc != NULL ) { 01874 change_destination_rectangle.hs = destination_rectangle.Left + GS_LCD_CHn_DISP_HS; 01875 change_destination_rectangle.vs = destination_rectangle.Top + GS_LCD_CHn_DISP_VS; 01876 change_destination_rectangle.hw = destination_rectangle.Right - destination_rectangle.Left + 1; 01877 change_destination_rectangle.vw = destination_rectangle.Bottom - destination_rectangle.Top + 1; 01878 01879 change.width_read_fb = &change_source_rectangle; 01880 change_source_rectangle.in_hw = change_destination_rectangle.hw; 01881 change_source_rectangle.in_vw = change_destination_rectangle.vw; 01882 01883 if ( is_interlace ) { 01884 change_source_rectangle.in_vw /= 2; 01885 } 01886 } 01887 01888 if ( change_source_rectangle.in_hw < 3 || change_source_rectangle.in_vw == 0 ) { 01889 is_show = false; 01890 } 01891 01892 if ( ! is_show ) { 01893 change_destination_rectangle.hs = GS_LCD_CHn_DISP_HS - 2; /* out of visible */ 01894 change_destination_rectangle.vs = GS_LCD_CHn_DISP_VS; 01895 change_destination_rectangle.hw = 3; 01896 change_destination_rectangle.vw = 1; 01897 change_source_rectangle.in_hw = 3; 01898 change_source_rectangle.in_vw = 1; 01899 attribute->OffsetByte = GS_OFFSET_BYTE_NOT_SHOW; 01900 } 01901 01902 error_vdc = R_VDC5_ChangeReadProcess( 01903 self->screen_channel, layer->data_control_ID, &change ); 01904 IF ( error_vdc != VDC5_OK ) { 01905 e = E_OTHERS; 01906 goto fin; 01907 } 01908 } 01909 if ( IS_ANY_BITS_SET( in_out_Attributes->flags, flags_of_window_position ) && 01910 IS_BIT_SET( in_out_Attributes->access, ACCESS_READ ) ) { 01911 window_surfaces_attribute_t *attribute = NULL; 01912 /* NULL is for avoiding warning C417W of mbed cloud compiler */ 01913 01914 ASSERT_R( IS_ALL_BITS_SET( in_out_Attributes->flags, F_LAYER_ID ), e=E_OTHERS; goto fin ); 01915 01916 e= window_surfaces_t__get_attribute( self, in_out_Attributes->id, &attribute ); 01917 IF(e) { 01918 goto fin; 01919 } 01920 01921 if ( IS_BIT_SET( in_out_Attributes->flags, F_LAYER_X ) ) { 01922 in_out_Attributes->x = attribute->X; 01923 } 01924 if ( IS_BIT_SET( in_out_Attributes->flags, F_LAYER_Y ) ) { 01925 in_out_Attributes->y = attribute->Y; 01926 } 01927 if ( IS_BIT_SET( in_out_Attributes->flags, F_LAYER_WIDTH ) ) { 01928 in_out_Attributes->width = attribute->Width; 01929 } 01930 if ( IS_BIT_SET( in_out_Attributes->flags, F_LAYER_HEIGHT ) ) { 01931 in_out_Attributes->height = attribute->Height; 01932 } 01933 if ( IS_BIT_SET( in_out_Attributes->flags, F_LAYER_OFFSET_X ) ) { 01934 in_out_Attributes->offset_x = attribute->OffsetX; 01935 } 01936 if ( IS_BIT_SET( in_out_Attributes->flags, F_LAYER_OFFSET_Y ) ) { 01937 in_out_Attributes->offset_y = attribute->OffsetY; 01938 } 01939 } 01940 } 01941 01942 01943 /* Call "R_VDC5_CLUT" */ 01944 { 01945 enum { flags_of_CLUT = F_LAYER_CLUT | F_LAYER_CLUT_COUNT }; 01946 01947 if ( IS_ANY_BITS_SET( in_out_Attributes->flags, flags_of_CLUT ) ) { 01948 vdc5_clut_t clut_data; 01949 enum { necessary_flags = flags_of_CLUT | F_LAYER_ID }; 01950 01951 ASSERT_R( IS_ALL_BITS_SET( in_out_Attributes->flags, necessary_flags ), 01952 e=E_OTHERS; goto fin ); 01953 ASSERT_R( IS_ANY_BITS_NOT_SET( 01954 in_out_Attributes->access, ~ (bit_flags_fast32_t) ACCESS_WRITE ), 01955 e=E_OTHERS; goto fin ); 01956 01957 clut_data.color_num = in_out_Attributes->CLUT_count; 01958 clut_data.clut = (uint32_t *)( in_out_Attributes->CLUT ); 01959 error_vdc = R_VDC5_CLUT( self->screen_channel, layer->data_control_ID, &clut_data ); 01960 IF( error_vdc != VDC5_OK ) { 01961 e=E_OTHERS; 01962 goto fin; 01963 } 01964 } 01965 } 01966 01967 IF( IS_ANY_BITS_SET( in_out_Attributes->flags, 01968 ~( F_LAYER_ID | F_LAYER_LAYER_COLOR | F_LAYER_CLUT_COUNT | F_LAYER_CLUT | 01969 F_LAYER_X | F_LAYER_Y | F_LAYER_WIDTH | F_LAYER_HEIGHT | 01970 F_LAYER_OFFSET_X | F_LAYER_OFFSET_Y ) ) ) { 01971 e=E_LIMITATION; 01972 goto fin; 01973 } 01974 01975 e=0; 01976 fin: 01977 return e; 01978 } 01979 01980 01981 /* Section: Global */ 01982 /** 01983 * @brief Put "SourceRect" and "DestinationRect" into the frame. 01984 * 01985 * @param SourceFrameBuffer gs_frame_width_height_t 01986 * @param DestinationFrameBuffer gs_frame_width_height_t 01987 * @param SourceRect gs_rectangle_t 01988 * @param DestinationRect gs_rectangle_t 01989 * @return Error Code. 0=No Error. 01990 * 01991 * @par Description 01992 * - If out of frame then "SourceRect - >Left > SourceRect->Right". 01993 */ 01994 errnum_t GS_ToInFrame( 01995 gs_frame_width_height_t *SourceFrameBuffer, 01996 gs_frame_width_height_t *DestinationFrameBuffer, 01997 gs_rectangle_t *SourceRect, 01998 gs_rectangle_t *DestinationRect ) 01999 { 02000 errnum_t e; 02001 int src_top, src_bottom, dst_top, dst_bottom; /* top down coord */ 02002 int diff; 02003 02004 02005 /* fast culling by out of frame buffer horizontal */ 02006 if ( SourceRect->Left >= SourceFrameBuffer->Width || 02007 DestinationRect->Left >= DestinationFrameBuffer->Width || 02008 SourceRect->Right < 0 || 02009 DestinationRect->Right < 0 ) { 02010 SourceRect->Right = SourceRect->Left - 1; /* out of frame */ 02011 e = 0; 02012 goto fin; 02013 } 02014 02015 02016 /* set "src_top", "src_bottom", "dst_top", "dst_bottom" */ 02017 src_top = SourceRect->Top; 02018 src_bottom = SourceRect->Bottom; 02019 dst_top = DestinationRect->Top; 02020 dst_bottom = DestinationRect->Bottom; 02021 02022 02023 /* fast culling by out of frame buffer vertical */ 02024 if ( src_top >= SourceFrameBuffer->Height || 02025 dst_top >= DestinationFrameBuffer->Height || 02026 src_bottom < 0 || 02027 dst_bottom < 0 ) { 02028 SourceRect->Right = SourceRect->Left - 1; /* out of frame */ 02029 e = 0; 02030 goto fin; 02031 } 02032 02033 02034 /* set top >= 0 */ 02035 if ( src_top < 0 ) { 02036 dst_top += - src_top; 02037 src_top = 0; 02038 } 02039 02040 if ( dst_top < 0 ) { 02041 src_top += - dst_top; 02042 dst_top = 0; 02043 } 02044 02045 02046 /* set bottom < frame height */ 02047 if ( src_bottom >= SourceFrameBuffer->Height ) { 02048 diff = src_bottom - SourceFrameBuffer->Height + 1; 02049 src_bottom -= diff; 02050 dst_bottom -= diff; 02051 } 02052 02053 if ( dst_bottom >= DestinationFrameBuffer->Height ) { 02054 diff = dst_bottom - DestinationFrameBuffer->Height + 1; 02055 src_bottom -= diff; 02056 dst_bottom -= diff; 02057 } 02058 02059 02060 /* culling by out of frame buffer vertical for modified top and bottom */ 02061 if ( src_top < 0 || 02062 dst_top < 0 || 02063 src_bottom >= SourceFrameBuffer->Height || 02064 dst_bottom >= DestinationFrameBuffer->Height ) { 02065 SourceRect->Right = SourceRect->Left - 1; /* out of frame */ 02066 e = 0; 02067 goto fin; 02068 } 02069 02070 02071 /* return from "src_top", "src_bottom", "dst_top", "dst_bottom" */ 02072 SourceRect->Top = src_top; 02073 SourceRect->Bottom = src_bottom; 02074 DestinationRect->Top = dst_top; 02075 DestinationRect->Bottom = dst_bottom; 02076 02077 02078 /* set left >= 0 */ 02079 if ( SourceRect->Left < 0 ) { 02080 DestinationRect->Left += - SourceRect->Left; 02081 SourceRect->Left = 0; 02082 } 02083 02084 if ( DestinationRect->Left < 0 ) { 02085 SourceRect->Left += - DestinationRect->Left; 02086 DestinationRect->Left = 0; 02087 } 02088 02089 02090 /* set right < frame width */ 02091 if ( SourceRect->Right >= SourceFrameBuffer->Width ) { 02092 diff = SourceRect->Right - SourceFrameBuffer->Width + 1; 02093 SourceRect->Right -= diff; 02094 DestinationRect->Right -= diff; 02095 } 02096 02097 if ( DestinationRect->Right >= DestinationFrameBuffer->Width ) { 02098 diff = DestinationRect->Right - DestinationFrameBuffer->Width + 1; 02099 SourceRect->Right -= diff; 02100 DestinationRect->Right -= diff; 02101 } 02102 02103 02104 /* culling by out of frame buffer horizontal for modified top and bottom */ 02105 if ( SourceRect->Left >= SourceFrameBuffer->Width || 02106 DestinationRect->Left >= DestinationFrameBuffer->Width || 02107 SourceRect->Right < 0 || 02108 DestinationRect->Right < 0 ) { 02109 SourceRect->Right = SourceRect->Left - 1; /* out of frame */ 02110 e = 0; 02111 goto fin; 02112 } 02113 02114 e=0; 02115 fin: 02116 return e; 02117 } 02118 02119 02120 /*********************************************************************** 02121 * Class: vram_ex_stack_t 02122 ************************************************************************/ 02123 02124 /*********************************************************************** 02125 * Implement: R_VRAM_EX_STACK_Initialize 02126 ************************************************************************/ 02127 errnum_t R_VRAM_EX_STACK_Initialize( vram_ex_stack_t *self, void *NullConfig ) 02128 { 02129 errnum_t e; 02130 uint8_t *address; 02131 size_t size; 02132 02133 R_UNREFERENCED_VARIABLE( NullConfig ); 02134 02135 e= R_EXRAM_GetBigMemory( &address, &size ); 02136 IF(e) { 02137 goto fin; 02138 } 02139 02140 self->Start = address; 02141 self->Over = address + size; 02142 self->StackPointer = self->Start; 02143 02144 e=0; 02145 fin: 02146 return e; 02147 } 02148 02149 02150 /*********************************************************************** 02151 * Implement: R_VRAM_EX_STACK_Alloc 02152 ************************************************************************/ 02153 errnum_t R_VRAM_EX_STACK_Alloc( vram_ex_stack_t *self, frame_buffer_t *in_out_FrameBuffer ) 02154 { 02155 return alloc_VRAM_stack_sub( &self->StackPointer, self->Over, in_out_FrameBuffer ); 02156 } 02157 02158 02159 /*********************************************************************** 02160 * Implement: R_VRAM_EX_STACK_Free 02161 ************************************************************************/ 02162 errnum_t R_VRAM_EX_STACK_Free( vram_ex_stack_t *self, frame_buffer_t *frame_buffer ) 02163 { 02164 return free_VRAM_stack_sub( &self->StackPointer, frame_buffer, self->Start ); 02165 } 02166 02167
Generated on Tue Jul 12 2022 11:15:05 by
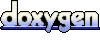