Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
vsync_pl.c
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2014 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /** 00024 * @file vsync_pl.c 00025 * @brief $Module: CLibCommon $ $PublicVersion: 1.00 $ (=CLIB_VERSION) 00026 * $Rev: 44 $ 00027 * $Date:: 2013-12-20 11:20:00 +0900#$ 00028 */ 00029 00030 00031 /****************************************************************************** 00032 Includes <System Includes> , "Project Includes" 00033 *******************************************************************************/ 00034 #include "r_ospl.h" 00035 #include "r_vdc5.h" 00036 #include "vsync.h " 00037 #include "vsync_pl.h " 00038 00039 #if ! R_OSPL_IS_PREEMPTION 00040 #include "dev_drv.h" 00041 #endif 00042 00043 /****************************************************************************** 00044 Typedef definitions 00045 ******************************************************************************/ 00046 00047 typedef void (* gs_rtx_interrupt_t )(void); /* RTX */ 00048 typedef void (* gs_cint_interrupt_t )( uint32_t int_sense ); /* OS less INTC */ 00049 typedef void (* gs_vdc_interrupt_t )( vdc5_int_type_t int_type ); 00050 00051 typedef struct st_r_v_sync_pl_channel_t r_v_sync_pl_channel_t; 00052 struct st_r_v_sync_pl_channel_t { 00053 const r_ospl_caller_t *InterruptCallbackCaller; 00054 bool_t IsVDC5_Callback; 00055 }; 00056 00057 static r_v_sync_pl_channel_t gs_v_sync_pl_channel[ R_V_SYNC_CHANNEL_COUNT ]; 00058 00059 00060 /****************************************************************************** 00061 Macro definitions 00062 ******************************************************************************/ 00063 00064 /** 00065 * @def GS_VSYNC_INTERRUPT_PRIORITY 00066 * @brief GS_VSYNC_INTERRUPT_PRIORITY 00067 * @par Parameters 00068 * None 00069 * @return None. 00070 */ 00071 enum { GS_VSYNC_INTERRUPT_PRIORITY = 7 }; 00072 00073 00074 /** 00075 * @def GS_INTERRUPT_FUNCTION_TYPE 00076 * @brief GS_INTERRUPT_FUNCTION_TYPE 00077 * @par Parameters 00078 * None 00079 * @return None. 00080 * 00081 * - 0 : RTX 00082 * - 1 : INTC 00083 */ 00084 #if R_OSPL_IS_PREEMPTION 00085 #define GS_INTERRUPT_FUNCTION_TYPE 0 00086 #else 00087 #define GS_INTERRUPT_FUNCTION_TYPE 1 00088 #endif 00089 00090 00091 /** 00092 * @typedef gs_interrupt_t 00093 * @brief Interrupt callback function type 00094 */ 00095 #if GS_INTERRUPT_FUNCTION_TYPE == 0 00096 #define gs_interrupt_t gs_rtx_interrupt_t 00097 #else 00098 #define gs_interrupt_t gs_cint_interrupt_t 00099 #endif 00100 00101 00102 /****************************************************************************** 00103 Imported global variables and functions (from other files) 00104 ******************************************************************************/ 00105 00106 /****************************************************************************** 00107 Exported global variables and functions (to be accessed by other files) 00108 ******************************************************************************/ 00109 00110 /****************************************************************************** 00111 Private global variables and functions 00112 ******************************************************************************/ 00113 static void R_V_SYNC_IRQ_HandlerN( int_fast32_t const ChannelNum ); 00114 static void R_V_SYNC_IRQ_Handler0( vdc5_int_type_t const int_type ); 00115 static void R_V_SYNC_IRQ_Handler1( vdc5_int_type_t const int_type ); 00116 #if GS_INTERRUPT_FUNCTION_TYPE != 1 00117 static gs_cint_interrupt_t gs_cint_vdc5_interrupt_handler[2]; 00118 static void R_V_SYNC_IRQ_HandlerRoot0(void); 00119 static void R_V_SYNC_IRQ_HandlerRoot1(void); 00120 #endif 00121 00122 00123 /** Table of interrupt line to channel number */ 00124 static const r_ospl_interrupt_t gs_array_of_i_context[ R_V_SYNC_CHANNEL_COUNT ] = { 00125 { BSP_INT_SRC_GR3_VLINE0, 0 }, 00126 { BSP_INT_SRC_GR3_VLINE1, 1 } 00127 }; 00128 00129 00130 /*********************************************************************** 00131 * Implement: R_V_SYNC_SetDefaultAsync 00132 ************************************************************************/ 00133 void R_V_SYNC_SetDefaultAsync( r_ospl_async_t *const Async, r_ospl_async_type_t AsyncType ) 00134 { 00135 R_UNREFERENCED_VARIABLE( AsyncType ); 00136 00137 IF_DQ( Async == NULL ) { 00138 goto fin; 00139 } 00140 00141 if ( IS_BIT_NOT_SET( Async->Flags, R_F_OSPL_A_Thread ) ) { 00142 Async->A_Thread = NULL; 00143 } 00144 00145 if ( IS_BIT_NOT_SET( Async->Flags, R_F_OSPL_A_EventValue ) ) { 00146 Async->A_EventValue = R_OSPL_A_FLAG; 00147 } 00148 00149 if ( IS_BIT_NOT_SET( Async->Flags, R_F_OSPL_I_Thread ) ) { 00150 Async->I_Thread = NULL; 00151 } 00152 00153 if ( IS_BIT_NOT_SET( Async->Flags, R_F_OSPL_I_EventValue ) ) { 00154 Async->I_EventValue = R_OSPL_I_FLAG; 00155 } 00156 00157 if ( IS_BIT_NOT_SET( Async->Flags, R_F_OSPL_InterruptCallback ) ) { 00158 Async->InterruptCallback = (r_ospl_callback_t)&( R_V_SYNC_OnInterruptDefault ); 00159 } 00160 /* MISRA 16.9 */ 00161 00162 Async->Flags = R_F_OSPL_A_Thread | R_F_OSPL_A_EventValue | 00163 R_F_OSPL_I_Thread | R_F_OSPL_I_EventValue | 00164 R_F_OSPL_InterruptCallback | R_F_OSPL_Delegate; 00165 00166 fin: 00167 return; 00168 } 00169 00170 00171 /*********************************************************************** 00172 * Implement: R_V_SYNC_OnInitialize 00173 ************************************************************************/ 00174 errnum_t R_V_SYNC_OnInitialize( int_fast32_t const ChannelNum ) 00175 { 00176 #if GS_INTERRUPT_FUNCTION_TYPE == 1 00177 bsp_int_cb_t handler; 00178 #endif 00179 errnum_t e; 00180 vdc5_error_t er; 00181 bsp_int_err_t eb; 00182 bsp_int_src_t int_id; 00183 vdc5_int_t interrupt; 00184 r_v_sync_pl_channel_t *const self = &gs_v_sync_pl_channel[ ChannelNum ]; 00185 const r_ospl_interrupt_t *const i_context = &gs_array_of_i_context[ ChannelNum ]; 00186 00187 /*[gs_IRQHandlers]*/ 00188 static const gs_vdc_interrupt_t gs_IRQHandlers[ R_V_SYNC_CHANNEL_COUNT ] = { 00189 &( R_V_SYNC_IRQ_Handler0 ), /* MISRA 16.9 */ 00190 &( R_V_SYNC_IRQ_Handler1 ) 00191 }; 00192 00193 #if GS_INTERRUPT_FUNCTION_TYPE != 1 00194 /*[gs_IRQHandlerRoots]*/ 00195 static const gs_interrupt_t gs_IRQHandlerRoots[ R_V_SYNC_CHANNEL_COUNT ] = { 00196 &( R_V_SYNC_IRQ_HandlerRoot0 ), /* MISRA 16.9 */ 00197 &( R_V_SYNC_IRQ_HandlerRoot1 ) 00198 }; 00199 #endif 00200 00201 00202 IF ( (ChannelNum < 0) || (ChannelNum >= R_V_SYNC_CHANNEL_COUNT) ) { 00203 e=E_OTHERS; 00204 goto fin; 00205 } 00206 00207 self->InterruptCallbackCaller = NULL; 00208 00209 int_id = i_context->IRQ_Num; 00210 #if GS_INTERRUPT_FUNCTION_TYPE == 1 00211 handler = R_VDC5_GetISR( (vdc5_channel_t) ChannelNum, VDC5_INT_TYPE_VLINE ); 00212 eb= R_BSP_InterruptWrite( int_id, handler ); 00213 #else 00214 gs_cint_vdc5_interrupt_handler[ChannelNum] = R_VDC5_GetISR( 00215 (vdc5_channel_t) ChannelNum, VDC5_INT_TYPE_VLINE ); 00216 eb= R_BSP_InterruptWrite( int_id, gs_IRQHandlerRoots[ChannelNum] ); 00217 #endif 00218 IF ( eb != BSP_INT_SUCCESS ) { 00219 e=E_OTHERS; 00220 goto fin; 00221 } 00222 00223 e= R_OSPL_SetInterruptPriority( int_id, GS_VSYNC_INTERRUPT_PRIORITY ); 00224 IF ( e != 0 ) { 00225 goto fin; 00226 } 00227 00228 interrupt.type = VDC5_INT_TYPE_VLINE; 00229 interrupt.callback = gs_IRQHandlers[ ChannelNum ]; 00230 interrupt.line_num = (uint16_t) 0; 00231 00232 er = R_VDC5_CallbackISR( (vdc5_channel_t) ChannelNum, &interrupt ); 00233 IF ( er != VDC5_OK ) { 00234 e=E_OTHERS; 00235 goto fin; 00236 } 00237 00238 self->IsVDC5_Callback = true; 00239 00240 e=0; 00241 fin: 00242 return e; 00243 } 00244 00245 00246 /*********************************************************************** 00247 * Implement: R_V_SYNC_OnFinalize 00248 ************************************************************************/ 00249 errnum_t R_V_SYNC_OnFinalize( int_fast32_t const ChannelNum, errnum_t e ) 00250 { 00251 r_v_sync_pl_channel_t *const self = &gs_v_sync_pl_channel[ ChannelNum ]; 00252 00253 if ( (ChannelNum >= 0) && (ChannelNum < R_V_SYNC_CHANNEL_COUNT) ) { 00254 if ( IS( self->IsVDC5_Callback ) ) { 00255 vdc5_error_t er; 00256 vdc5_int_t interrupt; 00257 00258 interrupt.type = VDC5_INT_TYPE_VLINE; 00259 interrupt.callback = NULL; 00260 interrupt.line_num = (uint16_t) 0; 00261 00262 er = R_VDC5_CallbackISR( (vdc5_channel_t) ChannelNum, &interrupt ); 00263 IF ( (er != VDC5_OK) && (e == 0) ) { 00264 e=E_OTHERS; 00265 } 00266 00267 self->IsVDC5_Callback = false; 00268 } 00269 } 00270 00271 return e; 00272 } 00273 00274 00275 /*********************************************************************** 00276 * Implement: R_V_SYNC_SetInterruptCallbackCaller 00277 ************************************************************************/ 00278 errnum_t R_V_SYNC_SetInterruptCallbackCaller( int_fast32_t const ChannelNum, 00279 const r_ospl_caller_t *const Caller ) 00280 { 00281 r_v_sync_pl_channel_t *const self = &gs_v_sync_pl_channel[ ChannelNum ]; 00282 00283 self->InterruptCallbackCaller = Caller; 00284 00285 return 0; 00286 } 00287 00288 00289 /*********************************************************************** 00290 * Implement: R_V_SYNC_OnEnableInterrupt 00291 ************************************************************************/ 00292 void R_V_SYNC_OnEnableInterrupt( int_fast32_t const ChannelNum, 00293 r_v_sync_interrupt_lines_t const Enables ) 00294 { 00295 bsp_int_err_t eb; 00296 00297 if ( IS_BIT_SET( Enables, R_V_SYNC_INTERRUPT_LINE_V_LINE ) ) { 00298 bsp_int_src_t const num_of_IRQ = gs_array_of_i_context[ ChannelNum ].IRQ_Num; 00299 00300 eb= R_BSP_InterruptControl( num_of_IRQ, BSP_INT_CMD_INTERRUPT_ENABLE, FIT_NO_PTR ); 00301 ASSERT_D( eb == 0, R_NOOP() ); 00302 R_UNREFERENCED_VARIABLE( eb ); /* for Release configuration */ 00303 } 00304 } 00305 00306 00307 /*********************************************************************** 00308 * Implement: R_V_SYNC_OnDisableInterrupt 00309 ************************************************************************/ 00310 void R_V_SYNC_OnDisableInterrupt( int_fast32_t const ChannelNum, 00311 r_v_sync_interrupt_lines_t const Disables1 ) 00312 { 00313 bsp_int_err_t eb; 00314 00315 if ( IS_BIT_SET( Disables1, R_V_SYNC_INTERRUPT_LINE_V_LINE ) ) { 00316 bsp_int_src_t const num_of_IRQ = gs_array_of_i_context[ ChannelNum ].IRQ_Num; 00317 00318 eb= R_BSP_InterruptControl( num_of_IRQ, BSP_INT_CMD_INTERRUPT_DISABLE, FIT_NO_PTR ); 00319 ASSERT_D( eb == 0, R_NOOP() ); 00320 R_UNREFERENCED_VARIABLE( eb ); /* for Release configuration */ 00321 } 00322 } 00323 00324 00325 /*********************************************************************** 00326 * Implement: R_V_SYNC_OnInterruptDefault 00327 ************************************************************************/ 00328 errnum_t R_V_SYNC_OnInterruptDefault( const r_ospl_interrupt_t *const InterruptSource, 00329 const r_ospl_caller_t *const Caller ) 00330 { 00331 R_UNREFERENCED_VARIABLE( Caller ); 00332 00333 return R_V_SYNC_OnInterrupting( InterruptSource ); 00334 } 00335 00336 00337 /** 00338 * @brief Interrupt service routine for all channels 00339 * 00340 * @param ChannelNum ChannelNum 00341 * @return None 00342 */ 00343 static void R_V_SYNC_IRQ_HandlerN( int_fast32_t const ChannelNum ) 00344 { 00345 r_v_sync_pl_channel_t *const self = &gs_v_sync_pl_channel[ ChannelNum ]; 00346 const r_ospl_interrupt_t *const i_context = &gs_array_of_i_context[ ChannelNum ]; 00347 00348 /* V-Sync interrupt always be enabled. Because clear interrupt status */ 00349 00350 if ( self->InterruptCallbackCaller != NULL ) { 00351 R_OSPL_CallInterruptCallback( self->InterruptCallbackCaller, i_context ); 00352 } 00353 } 00354 00355 00356 /** 00357 * @brief Interrupt service routine for INTC channel 0 00358 * 00359 * @param int_type ignored 00360 * @return None 00361 */ 00362 static void R_V_SYNC_IRQ_Handler0( vdc5_int_type_t const int_type ) 00363 { 00364 R_UNREFERENCED_VARIABLE( int_type ); 00365 R_V_SYNC_IRQ_HandlerN( 0 ); 00366 } 00367 00368 00369 /** 00370 * @brief Interrupt service routine for INTC channel 1 00371 * 00372 * @param int_type ignored 00373 * @return None 00374 */ 00375 static void R_V_SYNC_IRQ_Handler1( vdc5_int_type_t const int_type ) 00376 { 00377 R_UNREFERENCED_VARIABLE( int_type ); 00378 R_V_SYNC_IRQ_HandlerN( 1 ); 00379 } 00380 00381 00382 /** 00383 * @brief Interrupt service routine for RTX channel 0 00384 * 00385 * @par Parameters 00386 * None 00387 * @return None 00388 */ 00389 #if GS_INTERRUPT_FUNCTION_TYPE != 1 00390 static void R_V_SYNC_IRQ_HandlerRoot0(void) 00391 { 00392 gs_cint_vdc5_interrupt_handler[0]( 0 ); 00393 GIC_EndInterrupt( BSP_INT_SRC_GR3_VLINE0 ); 00394 } 00395 #endif 00396 00397 00398 /** 00399 * @brief Interrupt service routine for RTX channel 1 00400 * 00401 * @par Parameters 00402 * None 00403 * @return None 00404 */ 00405 #if GS_INTERRUPT_FUNCTION_TYPE != 1 00406 static void R_V_SYNC_IRQ_HandlerRoot1(void) 00407 { 00408 gs_cint_vdc5_interrupt_handler[1]( 0 ); 00409 GIC_EndInterrupt( BSP_INT_SRC_GR3_VLINE0 ); 00410 } 00411 #endif 00412
Generated on Tue Jul 12 2022 11:15:05 by
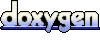