Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
vsync.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2014 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /** 00024 * @file vsync.h 00025 * @brief $Module: CLibCommon $ $PublicVersion: 1.00 $ (=CLIB_VERSION) 00026 * $Rev: 38 $ 00027 * $Date:: 2014-03-18 16:14:45 +0900#$ 00028 */ 00029 00030 #ifndef VSYNC_H 00031 #define VSYNC_H 00032 00033 /****************************************************************************** 00034 Includes <System Includes> , "Project Includes" 00035 ******************************************************************************/ 00036 #include "r_typedefs.h" 00037 #include "vsync_typedef.h " 00038 00039 #ifdef __cplusplus 00040 extern "C" { 00041 #endif /* __cplusplus */ 00042 00043 00044 /****************************************************************************** 00045 Typedef definitions 00046 ******************************************************************************/ 00047 /* In "vsync_typedef.h" */ 00048 00049 /****************************************************************************** 00050 Macro definitions 00051 ******************************************************************************/ 00052 /* In "vsync_typedef.h" */ 00053 00054 /****************************************************************************** 00055 Variable Externs 00056 ******************************************************************************/ 00057 /* In "vsync_typedef.h" */ 00058 00059 /****************************************************************************** 00060 Functions Prototypes 00061 ******************************************************************************/ 00062 00063 /** 00064 * @brief Initialize V-Sync object of specified channel number 00065 * 00066 * @param ChannelNum ChannelNum 00067 * @return Error Code. 0=No Error. 00068 */ 00069 errnum_t R_V_SYNC_Initialize( int_fast32_t const ChannelNum ); 00070 00071 00072 /** 00073 * @brief R_V_SYNC_Finalize 00074 * 00075 * @param ChannelNum ChannelNum 00076 * @param e Errors that have occurred. No error = 0 00077 * @return Error code or e, 0 = successful and input e=0 00078 */ 00079 errnum_t R_V_SYNC_Finalize( int_fast32_t const ChannelNum, errnum_t e ); 00080 00081 00082 /** 00083 * @brief Wait for V-Sync 00084 * 00085 * @param ChannelNum ChannelNum 00086 * @param SwapInterval If 1=60fps Then 2=30fps, 3=20fps 00087 * @param Is1VSyncAtMinimum false = It is possible to return soon 00088 * @return Error Code. 0=No Error. 00089 */ 00090 errnum_t R_V_SYNC_Wait( int_fast32_t const ChannelNum, 00091 int_fast32_t const SwapInterval, bool_t const Is1VSyncAtMinimum ); 00092 00093 00094 /** 00095 * @brief Asynchronous function of <R_V_SYNC_Wait> 00096 * 00097 * @param ChannelNum ChannelNum 00098 * @param SwapInterval If 1=60fps Then 2=30fps, 3=20fps 00099 * @param Is1VSyncAtMinimum false = It is possible to set event soon 00100 * @param Async r_ospl_async_t 00101 * @return Error Code. 0=No Error. 00102 */ 00103 errnum_t R_V_SYNC_WaitStart( int_fast32_t const ChannelNum, 00104 int_fast32_t const SwapInterval, bool_t const Is1VSyncAtMinimum, 00105 r_ospl_async_t *const Async ); 00106 00107 00108 /** 00109 * @brief Receive interrupt information. 00110 * 00111 * @param InterruptSource r_ospl_interrupt_t 00112 * @return Error Code. 0=No Error. 00113 */ 00114 errnum_t R_V_SYNC_OnInterrupting( const r_ospl_interrupt_t *const InterruptSource ); 00115 00116 00117 /** 00118 * @brief Get <r_v_sync_async_status_t> 00119 * 00120 * @param ChannelNum ChannelNum 00121 * @param out_Status AsyncStatus 00122 * @return Error Code. 0=No Error. 00123 */ 00124 errnum_t R_V_SYNC_GetAsyncStatus( int_fast32_t const ChannelNum, 00125 const r_v_sync_async_status_t **const out_Status ); 00126 00127 00128 /* Following functions can not be called from Application. */ 00129 void R_V_SYNC_EnableInterrupt( int_fast32_t const ChannelNum ); 00130 bool_t R_V_SYNC_DisableInterrupt( int_fast32_t const ChannelNum ); 00131 /* It is not necessary: R_V_SYNC_FinalizeAsync(), R_V_SYNC_OnInterrupted() */ 00132 00133 00134 #ifdef __cplusplus 00135 } /* extern "C" */ 00136 #endif /* __cplusplus */ 00137 00138 #endif
Generated on Tue Jul 12 2022 11:15:05 by
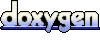