Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
r_ospl_os_less.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /** 00024 * @file r_ospl_os_less.h 00025 * @brief OS Porting Layer API for OS less Compatibility 00026 * 00027 * $Module: OSPL $ $PublicVersion: 0.90 $ (=R_OSPL_VERSION) 00028 * $Rev: 35 $ 00029 * $Date:: 2014-04-15 21:38:18 +0900#$ 00030 */ 00031 00032 #ifndef OSPL_OS_LESS_H 00033 #define OSPL_OS_LESS_H 00034 00035 /****************************************************************************** 00036 Includes <System Includes> , "Project Includes" 00037 ******************************************************************************/ 00038 #include "r_ospl_os_less_typedef.h" 00039 #include "./r_ospl.h" 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif /* __cplusplus */ 00044 00045 00046 /****************************************************************************** 00047 Typedef definitions 00048 ******************************************************************************/ 00049 /* In "ospl_os_less_typedef.h" */ 00050 00051 /****************************************************************************** 00052 Macro definitions 00053 ******************************************************************************/ 00054 /* In "ospl_os_less_typedef.h" */ 00055 00056 /****************************************************************************** 00057 Variable Externs 00058 ******************************************************************************/ 00059 /* In "ospl_os_less_typedef.h" */ 00060 00061 /****************************************************************************** 00062 Functions Prototypes 00063 ******************************************************************************/ 00064 00065 /** 00066 * @brief Set waiting behavior of current thread. 00067 * 00068 * @param OnWait Behavior on waiting 00069 * @return Error code. If there is no error, the return value is 0. 00070 * 00071 * @par Description 00072 * @par - Case of <R_OSPL_IS_PREEMPTION> = 0 00073 * 00074 * Initial value is @ref R_OSPL_WAIT_POLLING. 00075 * If this function was called from the interrupt context, E_STATE error is raised. 00076 * 00077 * @par - Case of <R_OSPL_IS_PREEMPTION> = 1 00078 * 00079 * This function is for compatibility only. 00080 * Arguments are ignored. 00081 * This function returns 0. 00082 * 00083 * Refer to: @ref R_OSPL_THREAD_GetIsWaiting 00084 */ 00085 INLINE errnum_t R_OSPL_THREAD_SetOnWait( r_ospl_wait_t const OnWait ) 00086 { 00087 return 0; 00088 } 00089 00090 00091 /** 00092 * @brief Get waiting behavior of current thread. 00093 * 00094 * @par Parameters 00095 * None 00096 * @return Behavior on waiting 00097 * 00098 * @par Description 00099 * @par - Case of <R_OSPL_IS_PREEMPTION> = 0 00100 * 00101 * Initial value is @ref R_OSPL_WAIT_POLLING. 00102 * If this function was called from the interrupt context, 00103 * this function returns @ref R_OSPL_WAIT_POLLING. 00104 * 00105 * @par - Case of <R_OSPL_IS_PREEMPTION> = 1 00106 * 00107 * This function is for compatibility only. 00108 * This function returns R_OSPL_WAIT_POLLING. 00109 * But it does not polling on waiting 00110 * 00111 * Refer to: @ref R_OSPL_THREAD_SetOnWait 00112 */ 00113 INLINE r_ospl_wait_t R_OSPL_THREAD_GetOnWait(void) 00114 { 00115 return R_OSPL_WAIT_POLLING; 00116 } 00117 00118 00119 /** 00120 * @brief Get whether the current thread is waiting or not. 00121 * 00122 * @par Parameters 00123 * None 00124 * @return Whether the current thread is waiting or not 00125 * 00126 * @par Description 00127 * @par - Case of <R_OSPL_IS_PREEMPTION> = 0 00128 * 00129 * If @ref R_OSPL_WAIT_PM_THREAD was set by @ref R_OSPL_THREAD_SetOnWait function, 00130 * some waiting functions return soon even if the state is waiting and 00131 * @ref R_OSPL_THREAD_GetIsWaiting function returns true. 00132 * 00133 * If time out was set to 0, @ref R_OSPL_THREAD_GetIsWaiting function returns 00134 * true at time out, even if any value was passed to @ref R_OSPL_THREAD_SetOnWait 00135 * function, 00136 * 00137 * If this function was called from the interrupt context, this function 00138 * returns false and @ref ASSERT_D in this function notifies in debug configuration. 00139 * 00140 * @par - Case of <R_OSPL_IS_PREEMPTION> = 1 00141 * 00142 * This function is for compatibility only. 00143 * This function returns false. 00144 * 00145 * @par Example 00146 * @code 00147 * e= R_OSPL_Delay( 100 ); IF(e){goto fin;} 00148 * if ( R_OSPL_THREAD_GetIsWaiting() ) { e=0; goto fin; } 00149 * @endcode 00150 */ 00151 INLINE bool_t R_OSPL_THREAD_GetIsWaiting(void) 00152 { 00153 return false; 00154 } 00155 00156 00157 /** 00158 * @brief Exit waiting state, if current thread was waiting state. 00159 * 00160 * @par Parameters 00161 * None 00162 * @return None 00163 * 00164 * @par Description 00165 * @par - Case of <R_OSPL_IS_PREEMPTION> = 0 00166 * 00167 * The thread returned true from @ref R_OSPL_THREAD_GetIsWaiting function 00168 * after a waiting function must call the waiting function again. 00169 * After exiting waiting state, other operation and other waiting can 00170 * be done. If time out was set to 0, exiting does not have to do. 00171 * 00172 * If it was detected in OSPL API that necessary exiting was not done, 00173 * E_STATE error is raised. However sometimes the state can not be detected. 00174 * In this case, time out will be not correct. 00175 * 00176 * This function does not do anything called from the interrupt context. 00177 * "ASSERT_D" in this function notifies in debug configuration. 00178 * 00179 * @par - Case of <R_OSPL_IS_PREEMPTION> = 1 00180 * 00181 * This function is for compatibility only. 00182 * This function does not do anything. 00183 */ 00184 INLINE void R_OSPL_THREAD_ExitWaiting(void) 00185 { 00186 } 00187 00188 00189 /*********************************************************************** 00190 * End of File: 00191 ************************************************************************/ 00192 #ifdef __cplusplus 00193 } /* extern "C" */ 00194 #endif /* __cplusplus */ 00195 00196 #endif /* OSPL_OS_LESS_H */ 00197
Generated on Tue Jul 12 2022 11:15:03 by
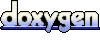