Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
r_ospl_debug.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /** 00024 * @file r_ospl_debug.h 00025 * @brief Debug tools provided by OSPL. 00026 * 00027 * $Module: OSPL $ $PublicVersion: 0.90 $ (=R_OSPL_VERSION) 00028 * $Rev: 35 $ 00029 * $Date:: 2014-04-15 21:38:18 +0900#$ 00030 */ 00031 00032 #ifndef OSPL_DEBUG_H 00033 #define OSPL_DEBUG_H 00034 00035 00036 /****************************************************************************** 00037 Includes <System Includes> , "Project Includes" 00038 ******************************************************************************/ 00039 #include "r_typedefs.h" 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif /* __cplusplus */ 00044 00045 00046 /****************************************************************************** 00047 Typedef definitions 00048 ******************************************************************************/ 00049 00050 /****************************************************************************** 00051 Macro definitions 00052 ******************************************************************************/ 00053 00054 /** 00055 * @def R_OSPL_DEBUG_TOOL 00056 * @brief Whether debug tools function is defined 00057 * @par Parameters 00058 * None 00059 * @return None. 00060 * 00061 * @par Description 00062 * The value can be changed. 00063 * The value is 1 or 0. 00064 */ 00065 #ifndef R_OSPL_DEBUG_TOOL 00066 #ifndef R_OSPL_NDEBUG 00067 #define R_OSPL_DEBUG_TOOL 1 00068 #else 00069 #define R_OSPL_DEBUG_TOOL 0 00070 #endif 00071 #endif 00072 00073 00074 /****************************************************************************** 00075 Variable Externs 00076 ******************************************************************************/ 00077 00078 /****************************************************************************** 00079 Functions Prototypes 00080 ******************************************************************************/ 00081 00082 /* Group: Watch */ 00083 00084 /** 00085 * @brief Registers watching integer variable or pointer variable 00086 * 00087 * @param IndexNum Watch Number, 0 or more 00088 * @param Address Address of watching integer variable or pointer variable 00089 * @param BreakValue Breaking value of variable when "R_D_Watch" was called 00090 * @param IsPrintf Whether "printf" is called, when "R_D_Watch" was called 00091 * @return None 00092 * 00093 * @par Description 00094 * @ref R_D_Add and @ref R_D_Watch are APIs related to watch function. 00095 * This debug tool is available, if @ref R_OSPL_DEBUG_TOOL was set to 1. 00096 * 00097 * There is not this function, if @ref R_OSPL_DEBUG_TOOL macro was defined 00098 * to be 0. This function is available, if @ref R_OSPL_DEBUG_TOOL macro was 00099 * defined to be 1. 00100 * 00101 * @par Example 00102 * @code 00103 * R_D_Add( 0, &var, 0xB0, true ); // 0xB0 may be not hit 00104 * @endcode 00105 */ 00106 #if R_OSPL_DEBUG_TOOL 00107 void R_D_Add( int_fast32_t IndexNum, volatile const void *Address, uint32_t BreakValue, bool_t IsPrintf ); 00108 #endif 00109 00110 00111 /** 00112 * @brief Show and Check watching variable's value 00113 * 00114 * @param IndexNum Watch Number, 0 or more 00115 * @return None 00116 * 00117 * @par Description 00118 * @ref R_D_Add and @ref R_D_Watch are APIs related to watch function. 00119 * This debug tool is available, if "R_OSPL_DEBUG_TOOL" was set to 1. 00120 * This function calls "printf" with the value of variable registered 00121 * by "R_D_Add" and breaks when watching variable becomes registered value. 00122 * This function can be called from out of scope of registered variable. 00123 * Then this function can be written at many places without care of the scope. 00124 * 00125 * There is not this function, if @ref R_OSPL_DEBUG_TOOL macro was defined 00126 * to be 0. This function is available, if @ref R_OSPL_DEBUG_TOOL macro was 00127 * defined to be 1. 00128 * 00129 * If internal data of the debug tool was broken, the global variable in 00130 * "r_ospl_debug.o" file should be move to safe address (memory map). 00131 * 00132 * @par Example 00133 * @code 00134 * printf( 41 ); // Show the value indicated this place 00135 * R_D_Watch( 0 ); 00136 * @endcode 00137 */ 00138 #if R_OSPL_DEBUG_TOOL 00139 void R_D_Watch( int_fast32_t IndexNum ); 00140 #endif 00141 00142 00143 /* Group: Int Log */ 00144 00145 /** 00146 * @brief Records to the log fast 00147 * 00148 * @param Value Recording value 00149 * @return None 00150 * 00151 * @par Description 00152 * @ref R_D_AddToIntLog, @ref g_IntLog and @ref g_IntLogLength are APIs related 00153 * to Int Log function. 00154 * This debug tool is available, if @ref R_OSPL_DEBUG_TOOL was set to 1. 00155 * This function overwrites from the first of the log, if max element 00156 * value of "g_IntLog" was over. 00157 * It is recommended to record not only the showing value of variable, 00158 * but also the value of the identifier of the place and the value of 00159 * current time. 00160 * 00161 * There is not this function, if @ref R_OSPL_DEBUG_TOOL macro was defined 00162 * to be 0. This function is available, if @ref R_OSPL_DEBUG_TOOL macro was 00163 * defined to be 1. 00164 * 00165 * If internal data of the debug tool was broken, the global variable in 00166 * "r_ospl_debug.o" file should be move to safe address (memory map). 00167 */ 00168 #if R_OSPL_DEBUG_TOOL 00169 void R_D_AddToIntLog( int_fast32_t Value ); 00170 #endif 00171 00172 00173 /** 00174 * @def g_IntLogCount 00175 * @brief Max count of int log 00176 */ 00177 #if R_OSPL_DEBUG_TOOL 00178 enum { g_IntLogCount = 100 }; 00179 #endif 00180 00181 00182 /** Memory area of int log */ 00183 #if R_OSPL_DEBUG_TOOL 00184 extern volatile int_fast32_t g_IntLog[ g_IntLogCount ]; 00185 #endif 00186 00187 00188 /** Length of recorded in <g_IntLog> */ 00189 #if R_OSPL_DEBUG_TOOL 00190 extern volatile int_fast32_t g_IntLogLength; 00191 #endif 00192 00193 00194 /* Group: Debug Variable */ 00195 00196 /** 00197 * @def g_DebugVarCount 00198 * @brief Count of debug variable. 00199 */ 00200 #if R_OSPL_DEBUG_TOOL 00201 enum { g_DebugVarCount = 10 }; 00202 #endif 00203 00204 00205 /** Debug variables. */ 00206 #if R_OSPL_DEBUG_TOOL 00207 extern volatile uint_fast32_t g_DebugVar[ g_DebugVarCount ]; 00208 #endif 00209 00210 00211 /* Group: Through Counter */ 00212 00213 /** 00214 * @brief Count the through count 00215 * 00216 * @param in_out_Counter Input/Output: The through counter 00217 * @param TargetCount The value comparing with the through counter 00218 * @param Label The label for "printf", NULL=printf : no output 00219 * @return None 00220 * 00221 * @par Description 00222 * This debug tool is available, if @ref R_OSPL_DEBUG_TOOL was set to 1. 00223 * If this function was called with "TargetCount = 0", the count of 00224 * through is output by "printf" for each calling. If "TargetCount" 00225 * argument was set to the through count and restart the program, 00226 * when the counter was counted up to "TargetCount", this function 00227 * returns "true". If there were many "printf" output, set "Label = NULL". 00228 * At first calling, the address of the counter is output by "printf". 00229 * The counter can be look by the debugger. 00230 * 00231 * @par Example 00232 * @code 00233 * { static int tc; if ( R_D_Counter( &tc, 0, "A" ) ) { 00234 * R_DEBUG_BREAK(); }} 00235 * @endcode 00236 */ 00237 #if R_OSPL_DEBUG_TOOL 00238 bool_t R_D_Counter( int_fast32_t *in_out_Counter, int_fast32_t TargetCount, char_t *Label ); 00239 #endif 00240 00241 00242 /*********************************************************************** 00243 * End of File: 00244 ************************************************************************/ 00245 #ifdef __cplusplus 00246 } /* extern "C" */ 00247 #endif /* __cplusplus */ 00248 00249 #endif /* _OSPL_DEBUG_H */ 00250
Generated on Tue Jul 12 2022 11:15:03 by
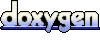