Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
r_ospl_RTX.c
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2014 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /** 00024 * @file r_ospl_RTX.c 00025 * @brief OS Porting Layer API for RTX 00026 * 00027 * $Module: OSPL $ $PublicVersion: 0.90 $ (=R_OSPL_VERSION) 00028 * $Rev: 35 $ 00029 * $Date:: 2014-04-15 21:38:18 +0900#$ 00030 */ 00031 00032 00033 /****************************************************************************** 00034 Includes <System Includes> , "Project Includes" 00035 ******************************************************************************/ 00036 #include "r_ospl.h" 00037 #include "r_ospl_os_less_private.h" 00038 #include "r_ospl_private.h" 00039 #include "pl310.h" /* 2nd cache */ 00040 #if R_OSPL_IS_PREEMPTION 00041 #include "cmsis_os.h" 00042 #include "gic.h" 00043 #include "r_ospl_RTX_private.h" 00044 #endif 00045 00046 00047 /****************************************************************************** 00048 Typedef definitions 00049 ******************************************************************************/ 00050 00051 /****************************************************************************** 00052 Macro definitions 00053 ******************************************************************************/ 00054 00055 /** 00056 * @def OS_ERROR_SIGNAL 00057 * @brief CMSIS-RTOS defined immediate value 00058 * @par Parameters 00059 * None 00060 * @return None. 00061 */ 00062 #define OS_ERROR_SIGNAL 0x80000000 00063 00064 00065 /** 00066 * @def R_OSPL_EVENT_WATCH 00067 * @brief Debug tool 00068 * @par Parameters 00069 * None 00070 * @return None. 00071 */ 00072 #define R_OSPL_EVENT_WATCH 0 /* [R_OSPL_EVENT_WATCH] 0 or 1 */ 00073 00074 00075 /****************************************************************************** 00076 Imported global variables and functions (from other files) 00077 ******************************************************************************/ 00078 00079 /****************************************************************************** 00080 Exported global variables and functions (to be accessed by other files) 00081 ******************************************************************************/ 00082 00083 /****************************************************************************** 00084 Private global variables and functions 00085 ******************************************************************************/ 00086 00087 00088 /*********************************************************************** 00089 * Implement: R_OSPL_Initialize 00090 ************************************************************************/ 00091 errnum_t R_OSPL_Initialize( const void *const in_NullConfig ) 00092 { 00093 R_UNREFERENCED_VARIABLE( in_NullConfig ); 00094 return 0; 00095 } 00096 00097 00098 /*********************************************************************** 00099 * Implement: R_OSPL_THREAD_GetCurrentId 00100 ************************************************************************/ 00101 r_ospl_thread_id_t R_OSPL_THREAD_GetCurrentId(void) 00102 { 00103 return osThreadGetId(); 00104 } 00105 00106 00107 /*********************************************************************** 00108 * Implement: R_OSPL_EVENT_Set 00109 ************************************************************************/ 00110 void R_OSPL_EVENT_Set( r_ospl_thread_id_t const ThreadId, bit_flags32_t const SetFlags ) 00111 { 00112 int32_t ret; 00113 00114 if ( ThreadId != NULL ) { 00115 #if R_OSPL_EVENT_WATCH 00116 R_D_AddToIntLog( 0x70100000 + SetFlags ); 00117 R_D_AddToIntLog( (uintptr_t) R_OSPL_THREAD_GetCurrentId() ); 00118 R_D_AddToIntLog( (uintptr_t) ThreadId ); 00119 #endif 00120 00121 ret = osSignalSet( (osThreadId) ThreadId, (int32_t) SetFlags ); 00122 ASSERT_D( (ret & OS_ERROR_SIGNAL) == 0, R_NOOP() ); 00123 R_UNREFERENCED_VARIABLE( ret ); /* for Release configuration */ 00124 } 00125 } 00126 00127 00128 /*********************************************************************** 00129 * Implement: R_OSPL_EVENT_Clear 00130 ************************************************************************/ 00131 void R_OSPL_EVENT_Clear( r_ospl_thread_id_t const ThreadId, bit_flags32_t const ClearFlags1 ) 00132 { 00133 int32_t ret; 00134 00135 if ( ThreadId != NULL ) { 00136 IF_D( ( ClearFlags1 & ~0xFFFF ) != 0 ) { 00137 R_NOOP(); 00138 } 00139 00140 #if R_OSPL_EVENT_WATCH 00141 R_D_AddToIntLog( 0x70C00000 + ClearFlags1 ); 00142 R_D_AddToIntLog( (uintptr_t) R_OSPL_THREAD_GetCurrentId() ); 00143 R_D_AddToIntLog( (uintptr_t) ThreadId ); 00144 #endif 00145 00146 ret = osSignalClear( (osThreadId) ThreadId, (int32_t) ClearFlags1 ); 00147 /* "& 0xFFFF" is for avoiding error in osSignalClear */ 00148 ASSERT_D( (ret & OS_ERROR_SIGNAL) == 0, R_NOOP() ); 00149 R_UNREFERENCED_VARIABLE( ret ); /* for Release configuration */ 00150 } 00151 } 00152 00153 00154 /*********************************************************************** 00155 * Implement: R_OSPL_EVENT_Get 00156 ************************************************************************/ 00157 #if ( ! defined( osCMSIS ) || osCMSIS <= 0x10001 ) && R_OSPL_VERSION < 85 00158 bit_flags32_t R_OSPL_EVENT_Get( r_ospl_thread_id_t const ThreadId ) 00159 { 00160 int32_t ret; 00161 00162 if ( ThreadId == NULL ) { 00163 ret = 0; 00164 } else { 00165 ret = osSignalGet( (osThreadId) ThreadId ); 00166 ASSERT_D( (ret & OS_ERROR_SIGNAL) == 0, R_NOOP() ); 00167 } 00168 00169 return (bit_flags32_t) ret; 00170 } 00171 #endif 00172 00173 00174 /*********************************************************************** 00175 * Implement: R_OSPL_EVENT_Wait 00176 ************************************************************************/ 00177 errnum_t R_OSPL_EVENT_Wait( bit_flags32_t const WaigingFlags, bit_flags32_t *const out_GotFlags, 00178 uint32_t const Timeout_msec ) 00179 { 00180 errnum_t e; 00181 osEvent event; 00182 00183 R_STATIC_ASSERT( R_OSPL_INFINITE == osWaitForever, "" ); 00184 00185 #if R_OSPL_EVENT_WATCH 00186 R_D_AddToIntLog( 0x70BE0000 + WaigingFlags ); 00187 R_D_AddToIntLog( (uintptr_t) R_OSPL_THREAD_GetCurrentId() ); 00188 00189 { 00190 static int tc; 00191 if ( R_D_Counter( &tc, 0, NULL ) ) { 00192 R_DEBUG_BREAK(); 00193 } 00194 } 00195 #endif 00196 00197 00198 event = osSignalWait( (int32_t) WaigingFlags, Timeout_msec ); 00199 00200 00201 #if R_OSPL_EVENT_WATCH 00202 R_D_AddToIntLog( 0x70AF0000 ); 00203 R_D_AddToIntLog( (uintptr_t) R_OSPL_THREAD_GetCurrentId() ); 00204 #endif 00205 00206 if ( (event.status == osOK) || (event.status == osEventTimeout) ) { 00207 if ( out_GotFlags != NULL ) { 00208 *out_GotFlags = R_OSPL_TIMEOUT; 00209 } 00210 IF ( event.status == osEventTimeout ) { 00211 e=E_TIME_OUT; 00212 goto fin; 00213 } 00214 } else { 00215 ASSERT_R( event.status == osEventSignal, e=E_OTHERS; goto fin ); 00216 00217 if ( out_GotFlags != NULL ) { 00218 *out_GotFlags = (bit_flags32_t) event.value.signals; 00219 } 00220 } 00221 00222 e=0; 00223 fin: 00224 return e; 00225 } 00226 00227 00228 /*********************************************************************** 00229 * Implement: R_OSPL_SetInterruptPriority 00230 ************************************************************************/ 00231 errnum_t R_OSPL_SetInterruptPriority( bsp_int_src_t const IRQ_Num, int_fast32_t const Priority ) 00232 { 00233 GIC_SetPriority( IRQ_Num, (uint32_t) Priority ); 00234 return 0; 00235 } 00236 00237 00238 /*********************************************************************** 00239 * Implement: R_OSPL_MEMORY_Flush 00240 ************************************************************************/ 00241 void R_OSPL_MEMORY_Flush( r_ospl_flush_t const FlushType ) 00242 { 00243 if ( FlushType == R_OSPL_FLUSH_WRITEBACK_INVALIDATE ) { 00244 #if 0 00245 printf( "L1Flush\n" ); 00246 #endif 00247 00248 #if IS_RTX_USED 00249 __v7_clean_inv_dcache_all(); 00250 #else 00251 #error 00252 #endif 00253 } else if ( FlushType == R_OSPL_FLUSH_WRITEBACK_INVALIDATE_2ND ) { 00254 #if 0 00255 printf( "PL310Flush\n" ); 00256 #endif 00257 00258 PL310_CleanInvAllByWay(); 00259 } else { 00260 ASSERT_D( false, R_NOOP() ); 00261 } 00262 } 00263 00264 00265 /** 00266 * @brief R_OSPL_Is1bitOnly_Fast32_Sub 00267 * 00268 * @par Parameters 00269 * None 00270 * @return None. 00271 */ 00272 #ifndef R_OSPL_NDEBUG 00273 static bool_t R_OSPL_Is1bitOnly_Fast32_Sub( uint_fast32_t Value ) 00274 { 00275 if ( (Value & 0x0000FFFFu) == 0 ) { 00276 Value >>= 16; 00277 } 00278 if ( (Value & 0x000000FFu) == 0 ) { 00279 Value >>= 8; 00280 } 00281 if ( (Value & 0x0000000Fu) == 0 ) { 00282 Value >>= 4; 00283 } 00284 if ( (Value & 0x00000003u) == 0 ) { 00285 Value >>= 2; 00286 } 00287 if ( (Value & 0x00000001u) == 0 ) { 00288 Value >>= 1; 00289 } 00290 return ( Value == 1 ); 00291 } 00292 #endif 00293 00294 00295 /*********************************************************************** 00296 * Implement: R_OSPL_MEMORY_RangeFlush 00297 ************************************************************************/ 00298 errnum_t R_OSPL_MEMORY_RangeFlush( r_ospl_flush_t const FlushType, 00299 const void *const StartAddress, size_t const Length ) 00300 { 00301 errnum_t e; 00302 size_t cache_line_size; 00303 size_t cache_line_mask; 00304 uintptr_t start; 00305 uintptr_t over; 00306 00307 ASSERT_R( FlushType == R_OSPL_FLUSH_INVALIDATE, e=E_BAD_COMMAND_ID; goto fin ); 00308 00309 cache_line_size = R_OSPL_MEMORY_GetCacheLineSize(); 00310 cache_line_mask = cache_line_size - 1u; 00311 ASSERT_D( R_OSPL_Is1bitOnly_Fast32_Sub( cache_line_size ), e=E_OTHERS; goto fin ); 00312 00313 /* ->MISRA 11.3 */ /* ->SEC R2.7.1 */ 00314 ASSERT_R( ( (uintptr_t) StartAddress & cache_line_mask ) == 0u, e=E_OTHERS; goto fin ); 00315 ASSERT_R( ( Length & cache_line_mask ) == 0u, e=E_OTHERS; goto fin ); 00316 00317 start = (uintptr_t) StartAddress; 00318 over = ((uintptr_t) StartAddress + Length) - 1u; 00319 /* <-MISRA 11.3 */ /* <-SEC R2.7.1 */ 00320 00321 R_OSPL_MEMORY_RangeFlush_Sub( start, over, cache_line_size ); 00322 00323 e=0; 00324 fin: 00325 return e; 00326 } 00327 00328 00329 /*********************************************************************** 00330 * Implement: R_OSPL_MEMORY_GetSpecification 00331 ************************************************************************/ 00332 void R_OSPL_MEMORY_GetSpecification( r_ospl_memory_spec_t *const out_MemorySpec ) 00333 { 00334 IF_DQ( out_MemorySpec == NULL ) { 00335 goto fin; 00336 } 00337 00338 out_MemorySpec->CacheLineSize = R_OSPL_MEMORY_GetCacheLineSize(); 00339 00340 fin: 00341 return; 00342 } 00343 00344 00345 /*********************************************************************** 00346 * Implement: R_OSPL_Delay 00347 ************************************************************************/ 00348 errnum_t R_OSPL_Delay( uint32_t const DelayTime_msec ) 00349 { 00350 errnum_t e; 00351 osStatus rs; 00352 bool_t const is_overflow = ( DelayTime_msec > R_OSPL_MAX_TIME_OUT ); 00353 uint32_t const delay_parameter = DelayTime_msec + 1u; 00354 00355 ASSERT_D( ! is_overflow, R_NOOP() ); 00356 /* RTX 5.16: If delay_parameter = 100000, "osDelay" waits 65534 */ 00357 00358 rs= osDelay( delay_parameter ); 00359 IF ( rs == osErrorISR ) { 00360 e=E_NOT_THREAD; 00361 R_OSPL_RaiseUnrecoverable( e ); 00362 goto fin; 00363 } 00364 IF ( IS( is_overflow ) ) { 00365 e=E_TIME_OUT; 00366 goto fin; 00367 } 00368 00369 IF ( 00370 (rs != osOK) && /* for delay_parameter == 0 */ 00371 (rs != osEventTimeout) ) { /* for delay_parameter != 0 */ 00372 e=E_OTHERS; 00373 goto fin; 00374 } 00375 00376 e=0; 00377 fin: 00378 return e; 00379 } 00380 00381 00382 /*********************************************************************** 00383 * Implement: R_OSPL_QUEUE_Create 00384 ************************************************************************/ 00385 errnum_t R_OSPL_QUEUE_Create( r_ospl_queue_t **out_self, r_ospl_queue_def_t *QueueDefine ) 00386 { 00387 errnum_t e; 00388 r_ospl_queue_t *self = QueueDefine; 00389 00390 self->MailQId = osMailCreate( (osMailQDef_t *) QueueDefine->MailQDef, NULL ); 00391 IF ( self->MailQId == NULL ) { 00392 e=E_OTHERS; 00393 goto fin; 00394 } 00395 self->PublicStatus.UsedCount = 0; 00396 00397 *out_self = self; 00398 00399 e=0; 00400 fin: 00401 return e; 00402 } 00403 00404 00405 /*********************************************************************** 00406 * Implement: R_OSPL_QUEUE_GetStatus 00407 ************************************************************************/ 00408 errnum_t R_OSPL_QUEUE_GetStatus( r_ospl_queue_t *self, const r_ospl_queue_status_t **out_Status ) 00409 { 00410 *out_Status = &self->PublicStatus; 00411 return 0; 00412 } 00413 00414 00415 /*********************************************************************** 00416 * Implement: R_OSPL_QUEUE_Allocate 00417 ************************************************************************/ 00418 errnum_t R_OSPL_QUEUE_Allocate( r_ospl_queue_t *self, void *out_Address, uint32_t Timeout_msec ) 00419 { 00420 errnum_t e; 00421 void *address; 00422 bool_t was_all_enabled = false; 00423 00424 address = osMailAlloc( self->MailQId, Timeout_msec ); 00425 *(void **) out_Address = address; 00426 IF ( address == NULL && Timeout_msec > 0 ) { 00427 if ( R_OSPL_THREAD_GetCurrentId() == NULL ) { 00428 e=E_NOT_THREAD; 00429 } else { 00430 e=E_TIME_OUT; 00431 } 00432 goto fin; 00433 } 00434 00435 was_all_enabled = R_OSPL_DisableAllInterrupt(); 00436 self->PublicStatus.UsedCount += 1; 00437 00438 e=0; 00439 fin: 00440 if ( was_all_enabled ) { 00441 R_OSPL_EnableAllInterrupt(); 00442 } 00443 return e; 00444 } 00445 00446 00447 /*********************************************************************** 00448 * Implement: R_OSPL_QUEUE_Put 00449 ************************************************************************/ 00450 errnum_t R_OSPL_QUEUE_Put( r_ospl_queue_t *self, void *Address ) 00451 { 00452 errnum_t e; 00453 osStatus status; 00454 00455 status = osMailPut( self->MailQId, Address ); 00456 IF ( status != osOK ) { 00457 e=E_OTHERS; 00458 goto fin; 00459 } 00460 00461 e=0; 00462 fin: 00463 return e; 00464 } 00465 00466 00467 /*********************************************************************** 00468 * Implement: R_OSPL_QUEUE_Get 00469 ************************************************************************/ 00470 errnum_t R_OSPL_QUEUE_Get( r_ospl_queue_t *self, void *out_Address, uint32_t Timeout_msec ) 00471 { 00472 errnum_t e; 00473 osEvent event; 00474 00475 event = osMailGet( self->MailQId, Timeout_msec ); 00476 00477 if ( event.status != osOK ) { 00478 IF ( event.status != osEventMail ) { 00479 if ( event.status == osEventTimeout ) { 00480 e = E_TIME_OUT; 00481 } else if ( event.status == osErrorParameter ) { 00482 if ( R_OSPL_THREAD_GetCurrentId() == NULL ) { 00483 e = E_NOT_THREAD; 00484 } else { 00485 e = E_OTHERS; 00486 } 00487 } else { 00488 e = E_OTHERS; 00489 } 00490 goto fin; 00491 } 00492 } 00493 00494 *(void **) out_Address = event.value.p; 00495 00496 e=0; 00497 fin: 00498 return e; 00499 } 00500 00501 00502 /*********************************************************************** 00503 * Implement: R_OSPL_QUEUE_Free 00504 ************************************************************************/ 00505 errnum_t R_OSPL_QUEUE_Free( r_ospl_queue_t *self, void *Address ) 00506 { 00507 errnum_t e; 00508 osStatus status; 00509 bool_t was_all_enabled = false; 00510 00511 status = osMailFree( self->MailQId, Address ); 00512 IF ( status != osOK ) { 00513 e=E_OTHERS; 00514 goto fin; 00515 } 00516 00517 was_all_enabled = R_OSPL_DisableAllInterrupt(); 00518 self->PublicStatus.UsedCount -= 1; 00519 00520 e=0; 00521 fin: 00522 if ( was_all_enabled ) { 00523 R_OSPL_EnableAllInterrupt(); 00524 } 00525 return e; 00526 } 00527 00528
Generated on Tue Jul 12 2022 11:15:03 by
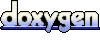