Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
ncg_vg_isr.c
00001 /****************************************************************************** 00002 * Copyright(c) 2010-2015 Renesas Electronics Corporation. All rights reserved. 00003 * 00004 * brief : R-GPVG control functions 00005 * 00006 * author : Renesas Electronics Corporation 00007 * 00008 * history: 2010.10.08 00009 * - Created the initial code. 00010 * 2011.03.07 00011 * - Fixed bug : NCGVG_Detach_ISR / [R-GPVG_common_P_033] 00012 * 2012.08.22 00013 * - Moved definition to "ncg_vg_isr.h". 00014 * - RGPVG_INT_LEVEL 00015 * - Moved definition to "ncg_register.h". 00016 * - RTIP_VG_BASE 00017 * - VG_REG_VG_ISR 00018 * 2012.08.22 00019 * - Added error message in the debug mode. 00020 * - Update all comments 00021 * 2013.02.21 00022 * - Modified the argument of the NCGVG_RGPVG_ISR. 00023 * - Modified the call to R_INTC_RegistIntFunc function 00024 * by NCGVG_Attach_ISR. 00025 * 2014.12.22 00026 * - Applied to OSPL and RGA. 00027 * 00028 *******************************************************************************/ 00029 00030 00031 /*============================================================================= 00032 * Includes 00033 */ 00034 00035 #include "Project_Config.h" 00036 #include "ncg_defs.h" 00037 #include "ncg_debug.h" 00038 #include "ncg_vg_isr.h" 00039 #include "RGA.h" 00040 00041 /* Depending on the build environment */ 00042 #include "r_typedefs.h" 00043 #include "r_ospl.h" 00044 00045 #ifdef RGAH_VERSION 00046 00047 /*============================================================================= 00048 * Internal definitions 00049 */ 00050 00051 00052 /*============================================================================= 00053 * Prototyping of internal functions 00054 */ 00055 static void NCGVG_RGPVG_ISR(void) ; 00056 static void NCGVG_RGPVG_ISR_0(void) ; 00057 static void NCGVG_RGPVG_ISR_1(void) ; 00058 static void NCGVG_RGPVG_ISR_2(void) ; 00059 static void NCGVG_RGPVG_ISR_3(void) ; 00060 00061 00062 /*============================================================================= 00063 * Private global variables and functions 00064 */ 00065 static NCGboolean NCGVG_ISR_Initialized = NCG_FALSE ; 00066 static NCGVGISRfp NCGVG_pRGPVG_Interrupt = NCG_NULL ; 00067 00068 00069 /*============================================================================= 00070 * Global Function 00071 */ 00072 00073 /*---------------------------------------------------------------------------- 00074 NAME : NCGVG_Attach_ISR 00075 FUNCTION : Attach the function pointer of OpenVG ISR. 00076 PARAMETERS : pfnInterrupt : [IN ] The pointer to the function of OpenVG ISR. 00077 RETURN : Error code of the NCG. 00078 ------------------------------------------------------------------------------*/ 00079 NCGint32 00080 NCGVG_Attach_ISR( 00081 NCGVGISRfp pfnInterrupt ) 00082 { 00083 NCGint32 rc_val = NCG_no_err; 00084 bsp_int_err_t eb; 00085 errnum_t e; 00086 00087 NCG_DEBUG_PRINT_STRING( "[LOG] NCGVG_Attach_ISR" ); 00088 00089 NCG_ASSERT(pfnInterrupt != NCG_NULL); 00090 00091 if( NCGVG_ISR_Initialized != NCG_FALSE ) { 00092 NCG_DEBUG_MAKE_MSG_START( NCG_G_MASSAGE_BUFF, "[NCG][ERROR]NCGVG_Attach_ISR(1) Initialized.%s" ) 00093 NCG_DEBUG_MAKE_MSG_PARAMETER( NCG_CRLF ) 00094 NCG_DEBUG_MAKE_MSG_END(); 00095 NCG_DEBUG_PRINT_MSG( NCG_G_MASSAGE_BUFF ); 00096 rc_val = NCG_err_isr_management_failed ; 00097 } else { 00098 NCGVG_ISR_Initialized = NCG_TRUE ; 00099 NCGVG_pRGPVG_Interrupt = pfnInterrupt ; 00100 00101 /* Regist function */ 00102 eb= R_BSP_InterruptWrite( BSP_INT_SRC_INT0, NCGVG_RGPVG_ISR_0 ); 00103 IF ( eb != BSP_INT_SUCCESS ) { 00104 rc_val = NCG_err_isr_management_failed; 00105 goto fin; 00106 } 00107 eb= R_BSP_InterruptWrite( BSP_INT_SRC_INT1, NCGVG_RGPVG_ISR_1 ); 00108 IF ( eb != BSP_INT_SUCCESS ) { 00109 rc_val = NCG_err_isr_management_failed; 00110 goto fin; 00111 } 00112 eb= R_BSP_InterruptWrite( BSP_INT_SRC_INT2, NCGVG_RGPVG_ISR_2 ); 00113 IF ( eb != BSP_INT_SUCCESS ) { 00114 rc_val = NCG_err_isr_management_failed; 00115 goto fin; 00116 } 00117 eb= R_BSP_InterruptWrite( BSP_INT_SRC_INT3, NCGVG_RGPVG_ISR_3 ); 00118 IF ( eb != BSP_INT_SUCCESS ) { 00119 rc_val = NCG_err_isr_management_failed; 00120 goto fin; 00121 } 00122 00123 /* set priority */ 00124 e= R_OSPL_SetInterruptPriority( BSP_INT_SRC_INT0, NCGVG_INT_LEVEL ); 00125 IF(e) { 00126 rc_val = NCG_err_isr_management_failed; 00127 goto fin; 00128 } 00129 e= R_OSPL_SetInterruptPriority( BSP_INT_SRC_INT1, NCGVG_INT_LEVEL ); 00130 IF(e) { 00131 rc_val = NCG_err_isr_management_failed; 00132 goto fin; 00133 } 00134 e= R_OSPL_SetInterruptPriority( BSP_INT_SRC_INT2, NCGVG_INT_LEVEL ); 00135 IF(e) { 00136 rc_val = NCG_err_isr_management_failed; 00137 goto fin; 00138 } 00139 e= R_OSPL_SetInterruptPriority( BSP_INT_SRC_INT3, NCGVG_INT_LEVEL ); 00140 IF(e) { 00141 rc_val = NCG_err_isr_management_failed; 00142 goto fin; 00143 } 00144 00145 /* Enable interrupt from Renesas OpenVG library */ 00146 eb= R_BSP_InterruptControl( BSP_INT_SRC_INT0, BSP_INT_CMD_INTERRUPT_ENABLE, FIT_NO_PTR ); 00147 IF ( eb != BSP_INT_SUCCESS ) { 00148 rc_val = NCG_err_isr_management_failed; 00149 goto fin; 00150 } 00151 eb= R_BSP_InterruptControl( BSP_INT_SRC_INT1, BSP_INT_CMD_INTERRUPT_ENABLE, FIT_NO_PTR ); 00152 IF ( eb != BSP_INT_SUCCESS ) { 00153 rc_val = NCG_err_isr_management_failed; 00154 goto fin; 00155 } 00156 eb= R_BSP_InterruptControl( BSP_INT_SRC_INT2, BSP_INT_CMD_INTERRUPT_ENABLE, FIT_NO_PTR ); 00157 IF ( eb != BSP_INT_SUCCESS ) { 00158 rc_val = NCG_err_isr_management_failed; 00159 goto fin; 00160 } 00161 eb= R_BSP_InterruptControl( BSP_INT_SRC_INT3, BSP_INT_CMD_INTERRUPT_ENABLE, FIT_NO_PTR ); 00162 IF ( eb != BSP_INT_SUCCESS ) { 00163 rc_val = NCG_err_isr_management_failed; 00164 goto fin; 00165 } 00166 } 00167 00168 fin: 00169 return rc_val; 00170 } 00171 00172 00173 /*---------------------------------------------------------------------------- 00174 NAME : NCGVG_Detach_ISR 00175 FUNCTION : Detach the function pointer of OpenVG ISR. 00176 PARAMETERS : pfnInterrupt : [IN ] The pointer to the function of OpenVG ISR. 00177 RETURN : Error code of the NCG. 00178 ------------------------------------------------------------------------------*/ 00179 NCGint32 00180 NCGVG_Detach_ISR( 00181 NCGVGISRfp pfnInterrupt ) 00182 { 00183 NCGint32 rc_val = NCG_no_err; 00184 bsp_int_err_t eb; 00185 00186 NCG_DEBUG_PRINT_STRING( "[LOG] NCGVG_Detach_ISR" ); 00187 00188 NCG_ASSERT(pfnInterrupt != NCG_NULL); 00189 00190 if ( NCGVG_ISR_Initialized == NCG_FALSE ) { 00191 NCG_DEBUG_MAKE_MSG_START( NCG_G_MASSAGE_BUFF, "[NCG][ERROR]NCGVG_Detach_ISR(1) Not initialized.%s" ) 00192 NCG_DEBUG_MAKE_MSG_PARAMETER( NCG_CRLF ) 00193 NCG_DEBUG_MAKE_MSG_END(); 00194 NCG_DEBUG_PRINT_MSG( NCG_G_MASSAGE_BUFF ); 00195 rc_val = NCG_err_isr_management_failed; 00196 } else { 00197 NCGVG_ISR_Initialized = NCG_FALSE ; 00198 00199 if( pfnInterrupt == NCGVG_pRGPVG_Interrupt ) { 00200 /* Disable interrupt from Renesas OpenVG library */ 00201 eb= R_BSP_InterruptControl( BSP_INT_SRC_INT3, BSP_INT_CMD_INTERRUPT_DISABLE, FIT_NO_PTR ); 00202 IF ( eb != BSP_INT_SUCCESS ) { 00203 rc_val = NCG_err_isr_management_failed; 00204 goto fin; 00205 } 00206 eb= R_BSP_InterruptControl( BSP_INT_SRC_INT2, BSP_INT_CMD_INTERRUPT_DISABLE, FIT_NO_PTR ); 00207 IF ( eb != BSP_INT_SUCCESS ) { 00208 rc_val = NCG_err_isr_management_failed; 00209 goto fin; 00210 } 00211 eb= R_BSP_InterruptControl( BSP_INT_SRC_INT1, BSP_INT_CMD_INTERRUPT_DISABLE, FIT_NO_PTR ); 00212 IF ( eb != BSP_INT_SUCCESS ) { 00213 rc_val = NCG_err_isr_management_failed; 00214 goto fin; 00215 } 00216 eb= R_BSP_InterruptControl( BSP_INT_SRC_INT0, BSP_INT_CMD_INTERRUPT_DISABLE, FIT_NO_PTR ); 00217 IF ( eb != BSP_INT_SUCCESS ) { 00218 rc_val = NCG_err_isr_management_failed; 00219 goto fin; 00220 } 00221 00222 /* Unregist function */ 00223 eb= R_BSP_InterruptWrite( BSP_INT_SRC_INT3, FIT_NO_FUNC ); 00224 IF ( eb != BSP_INT_SUCCESS ) { 00225 rc_val = NCG_err_isr_management_failed; 00226 goto fin; 00227 } 00228 eb= R_BSP_InterruptWrite( BSP_INT_SRC_INT2, FIT_NO_FUNC ); 00229 IF ( eb != BSP_INT_SUCCESS ) { 00230 rc_val = NCG_err_isr_management_failed; 00231 goto fin; 00232 } 00233 eb= R_BSP_InterruptWrite( BSP_INT_SRC_INT1, FIT_NO_FUNC ); 00234 IF ( eb != BSP_INT_SUCCESS ) { 00235 rc_val = NCG_err_isr_management_failed; 00236 goto fin; 00237 } 00238 eb= R_BSP_InterruptWrite( BSP_INT_SRC_INT0, FIT_NO_FUNC ); 00239 IF ( eb != BSP_INT_SUCCESS ) { 00240 rc_val = NCG_err_isr_management_failed; 00241 goto fin; 00242 } 00243 00244 NCGVG_pRGPVG_Interrupt = NCG_NULL ; 00245 } else { 00246 NCG_DEBUG_MAKE_MSG_START( NCG_G_MASSAGE_BUFF, "[NCG][ERROR]NCGVG_Detach_ISR(2) Miss match function pointer.%s" ) 00247 NCG_DEBUG_MAKE_MSG_PARAMETER( NCG_CRLF ) 00248 NCG_DEBUG_MAKE_MSG_END(); 00249 NCG_DEBUG_PRINT_MSG( NCG_G_MASSAGE_BUFF ); 00250 rc_val = NCG_err_isr_management_failed; 00251 } 00252 } 00253 00254 fin: 00255 return rc_val; 00256 } 00257 00258 00259 /*============================================================================= 00260 * Internal functions 00261 */ 00262 00263 /*----------------------------------------------------------------------------- 00264 NAME : NCGVG_RGPVG_ISR 00265 FUNCTION : The Interrrupt from OpenVG. 00266 PARAMETERS : 00267 RETURN : None. 00268 -----------------------------------------------------------------------------*/ 00269 static void NCGVG_RGPVG_ISR(void) 00270 { 00271 NCGuint32 ret ; 00272 errnum_t e; 00273 00274 NCG_DEBUG_PRINT_STRING( "[LOG] NCGVG_RGPVG_ISR" ); 00275 00276 if( NCGVG_pRGPVG_Interrupt == NCG_NULL ) { 00277 NCG_DEBUG_MAKE_MSG_START( NCG_G_MASSAGE_BUFF, "[NCG][INFO]NCGVG_RGPVG_ISR Not initialized.%s" ) 00278 NCG_DEBUG_MAKE_MSG_PARAMETER( NCG_CRLF ) 00279 NCG_DEBUG_MAKE_MSG_END(); 00280 NCG_DEBUG_PRINT_MSG( NCG_G_MASSAGE_BUFF ); 00281 } else { 00282 ret = NCGVG_pRGPVG_Interrupt() ; 00283 if ( ret != 0 ) { 00284 NCG_DEBUG_MAKE_MSG_START( NCG_G_MASSAGE_BUFF, "[NCG][ERROR]NCGVG_RGPVG_ISR(0x%08x)%s" ) 00285 NCG_DEBUG_MAKE_MSG_PARAMETER( ret ) 00286 NCG_DEBUG_MAKE_MSG_PARAMETER( NCG_CRLF ) 00287 NCG_DEBUG_MAKE_MSG_END(); 00288 NCG_DEBUG_PRINT_MSG( NCG_G_MASSAGE_BUFF ); 00289 } 00290 00291 e= R_GRAPHICS_OnInterrupting(); 00292 if ( e != 0 ) { 00293 NCG_DEBUG_MAKE_MSG_START( NCG_G_MASSAGE_BUFF, "[NCG][ERROR]NCGVG_RGPVG_ISR(e=0x%08x)%s" ) 00294 NCG_DEBUG_MAKE_MSG_PARAMETER( e ) 00295 NCG_DEBUG_MAKE_MSG_PARAMETER( NCG_CRLF ) 00296 NCG_DEBUG_MAKE_MSG_END(); 00297 NCG_DEBUG_PRINT_MSG( NCG_G_MASSAGE_BUFF ); 00298 } 00299 } 00300 00301 return ; 00302 } 00303 00304 /*----------------------------------------------------------------------------- 00305 NAME : NCGVG_RGPVG_ISR_0 00306 FUNCTION : The Interrrupt from OpenVG. 00307 PARAMETERS : 00308 RETURN : None. 00309 -----------------------------------------------------------------------------*/ 00310 static void NCGVG_RGPVG_ISR_0(void) 00311 { 00312 NCGVG_RGPVG_ISR(); 00313 00314 GIC_EndInterrupt( BSP_INT_SRC_INT0 ); 00315 } 00316 00317 /*----------------------------------------------------------------------------- 00318 NAME : NCGVG_RGPVG_ISR_1 00319 FUNCTION : The Interrrupt from OpenVG. 00320 PARAMETERS : 00321 RETURN : None. 00322 -----------------------------------------------------------------------------*/ 00323 static void NCGVG_RGPVG_ISR_1(void) 00324 { 00325 NCGVG_RGPVG_ISR(); 00326 00327 GIC_EndInterrupt( BSP_INT_SRC_INT1 ); 00328 } 00329 00330 /*----------------------------------------------------------------------------- 00331 NAME : NCGVG_RGPVG_ISR_2 00332 FUNCTION : The Interrrupt from OpenVG. 00333 PARAMETERS : 00334 RETURN : None. 00335 -----------------------------------------------------------------------------*/ 00336 static void NCGVG_RGPVG_ISR_2(void) 00337 { 00338 NCGVG_RGPVG_ISR(); 00339 00340 GIC_EndInterrupt( BSP_INT_SRC_INT2 ); 00341 } 00342 00343 /*----------------------------------------------------------------------------- 00344 NAME : NCGVG_RGPVG_ISR_3 00345 FUNCTION : The Interrrupt from OpenVG. 00346 PARAMETERS : 00347 RETURN : None. 00348 -----------------------------------------------------------------------------*/ 00349 static void NCGVG_RGPVG_ISR_3(void) 00350 { 00351 NCGVG_RGPVG_ISR(); 00352 00353 GIC_EndInterrupt( BSP_INT_SRC_INT3 ); 00354 } 00355 00356 #endif 00357 /* -- end of file -- */
Generated on Tue Jul 12 2022 11:15:02 by
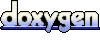