Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
ncg_vg.c
00001 /****************************************************************************** 00002 * Copyright(c) 2010-2014 Renesas Electronics Corporation. All rights reserved. 00003 * 00004 * brief : R-GPVG control functions. 00005 * 00006 * author : Renesas Electronics Corporation 00007 * 00008 * history: 2011.01.20 00009 * - Created the initial code. 00010 * 2012.08.22 00011 * - Added return value to "NCGVG_InitResourceSize" 00012 * 2013.05.13 00013 * - Added function of initialization. 00014 * - Added function of finalization. 00015 * 2013.06.10 00016 * - Applied to new VDC5 driver (Version 0.03.0046). 00017 * 2013.11.07 00018 * - Modified value of NCG_VG_SBO1_SIZE to (4U) from (0U). 00019 * 2014.02.13 00020 * - Modified the value of definitions. 00021 * NCG_VG_WB_STRIDE 00022 * NCG_VG_WB_HEIGHT 00023 * NCG_VG_SBO0_SIZE 00024 * NCG_VG_DLB_SIZE 00025 * 2014.03.25 00026 * - Modified NCGVG_Init() and NCGVG_DeInit(). 00027 * 2014.05.16 00028 * - Modified NCGVG_Init() and NCGVG_DeInit(). 00029 * 2014.12.22 00030 * - Applied to OSPL and RGA. 00031 * 00032 *******************************************************************************/ 00033 00034 /*============================================================================= 00035 * Includes 00036 */ 00037 00038 #include "Project_Config.h" 00039 #include "ncg_defs.h" 00040 #include "ncg_debug.h" 00041 #include "r_ospl.h" 00042 00043 #include "ncg_vg.h" 00044 00045 /* Depending on the build environment */ 00046 #include "r_typedefs.h" 00047 00048 #include "iodefine.h" 00049 #include "iobitmasks/cpg_iobitmask.h" 00050 00051 #ifdef RGAH_VERSION 00052 00053 /*============================================================================= 00054 * Internal definitions 00055 */ 00056 00057 /* TODO: 00058 Please change these parameters in accordance with your system. 00059 */ 00060 #define NCG_VG_WB_STRIDE (256U) 00061 #define NCG_VG_WB_HEIGHT (480U) 00062 00063 #define NCG_VG_SBO0_SIZE (16384U) 00064 #define NCG_VG_SBO1_SIZE (4U) 00065 #define NCG_VG_DLB_SIZE (5120U) 00066 00067 /*============================================================================= 00068 * Prototyping of internal functions 00069 */ 00070 00071 00072 /*============================================================================= 00073 * Private global variables and functions 00074 */ 00075 00076 00077 /*============================================================================= 00078 * Global Function 00079 */ 00080 00081 /*---------------------------------------------------------------------------- 00082 NAME : NCGVG_Init 00083 FUNCTION : Initialize R-GPVG. 00084 PARAMETERS : pVGInfo : [IN ] The pointer to the initialization information struct. 00085 RETURN : None. 00086 ------------------------------------------------------------------------------*/ 00087 NCGvoid 00088 NCGVG_Init ( 00089 PNCGVGINFO pVGInfo) 00090 { 00091 /* Depending on the build environment */ 00092 volatile uint8_t reg_value; 00093 00094 NCG_UNREFERENCED_PARAMETER( pVGInfo ); 00095 00096 NCG_DEBUG_PRINT_STRING( "[LOG] NCGVG_Init" ); 00097 00098 reg_value = CPG.STBCR10; 00099 if ( CPG.STBCR10 & CPG_STBCR10_MSTP100 ) { 00100 /* Standby control register 10 (STBCR10) : 0 : R-GPVG enable */ 00101 CPG.STBCR10 &= ~CPG_STBCR10_MSTP100; 00102 /* dummy read */ 00103 reg_value = CPG.STBCR10; 00104 00105 if ( CPG.STBREQ2 & CPG_STBREQ2_STBRQ20 ) { 00106 CPG.SWRSTCR3 |= CPG_SWRSTCR3_SRST32; 00107 /* dummy read */ 00108 reg_value = CPG.SWRSTCR3; 00109 00110 CPG.SWRSTCR3 &= ~CPG_SWRSTCR3_SRST32; 00111 /* dummy read */ 00112 reg_value = CPG.SWRSTCR3; 00113 00114 CPG.STBREQ2 &= ~CPG_STBREQ2_STBRQ20; 00115 /* dummy read */ 00116 reg_value = CPG.STBREQ2; 00117 while ( (CPG.STBACK2 & CPG_STBACK2_STBAK20) != 0 ) ; 00118 } 00119 } 00120 00121 NCG_UNREFERENCED_PARAMETER( reg_value ); /* Avoid warning of "unused-but-set-variable" of GCC_ARM */ 00122 00123 return ; 00124 } 00125 00126 /*---------------------------------------------------------------------------- 00127 NAME : NCGVG_DeInit 00128 FUNCTION : Finalize R-GPVG. 00129 PARAMETERS : pVGInfo : [IN ] The pointer to the finalization information struct. 00130 RETURN : None. 00131 ------------------------------------------------------------------------------*/ 00132 NCGvoid 00133 NCGVG_DeInit ( 00134 PNCGVGINFO pVGInfo ) 00135 { 00136 /* Depending on the build environment */ 00137 volatile uint8_t reg_value; 00138 00139 NCG_UNREFERENCED_PARAMETER(pVGInfo); 00140 00141 NCG_DEBUG_PRINT_STRING( "[LOG] NCGVG_DeInit" ); 00142 00143 if ( (CPG.STBCR10 & CPG_STBCR10_MSTP100) != CPG_STBCR10_MSTP100 ) { 00144 CPG.STBREQ2 |= CPG_STBREQ2_STBRQ20; 00145 /* dummy read */ 00146 reg_value = CPG.STBREQ2; 00147 while ( (CPG.STBACK2 & CPG_STBACK2_STBAK20) == 0 ) ; 00148 00149 /* Standby control register 10 (STBCR10) : 0 : R-GPVG disable */ 00150 CPG.STBCR10 |= CPG_STBCR10_MSTP100; 00151 /* dummy read */ 00152 reg_value = CPG.STBCR10; 00153 } 00154 00155 NCG_UNREFERENCED_PARAMETER( reg_value ); /* Avoid warning of "unused-but-set-variable" of GCC_ARM */ 00156 00157 return ; 00158 } 00159 00160 #endif 00161 /* -- end of file -- */
Generated on Tue Jul 12 2022 11:15:02 by
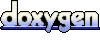