Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
ncg_state.h
00001 /****************************************************************************** 00002 * Copyright(c) 2010-2012 Renesas Electronics Corporation. All rights reserved. 00003 * 00004 * brief : NCG state variable control functions header. 00005 * 00006 * author : Renesas Electronics Corporation 00007 * 00008 * history: 2010.10.08 00009 * - Created the initial code. 00010 * 2012.08.22 00011 * - Updated coding format. 00012 * 00013 *******************************************************************************/ 00014 00015 #ifndef NCG_STATE_H 00016 #define NCG_STATE_H 00017 00018 #ifdef __cplusplus 00019 extern "C" { 00020 #endif 00021 00022 00023 /*============================================================================= 00024 * Definitions 00025 */ 00026 00027 /* 00028 * Mode Flags 00029 */ 00030 00031 #define NCGSYS_STATE_CALL_MASK (0x00000003U) 00032 #define NCGSYS_STATE_CALL_NORMAL (0x00000000U) 00033 #define NCGSYS_STATE_CALL_INTERRUPT (0x00000001U) 00034 #define NCGSYS_STATE_CALL_CALLBACK (0x00000002U) 00035 00036 #define NCGSYS_STATE_WAIT_MASK (0x00000004U) 00037 #define NCGSYS_STATE_WAIT_OR (0x00000004U) 00038 #define NCGSYS_STATE_WAIT_AND (0x00000000U) 00039 00040 #define NCGSYS_STATE_SET_MASK (0x00000018U) 00041 #define NCGSYS_STATE_SET_AND (0x00000000U) 00042 #define NCGSYS_STATE_SET_OR (0x00000008U) 00043 #define NCGSYS_STATE_SET_SET (0x00000010U) 00044 00045 #define NCGSYS_STATE_OR NCGSYS_STATE_SET_OR 00046 #define NCGSYS_STATE_SET NCGSYS_STATE_SET_SET 00047 #define NCGSYS_STATE_CLEAR NCGSYS_STATE_SET_AND 00048 00049 00050 /*============================================================================= 00051 * Structures 00052 */ 00053 00054 00055 /*============================================================================= 00056 * Function prototyping 00057 */ 00058 00059 /* 00060 * State control 00061 */ 00062 00063 NCGint32 00064 NCGSYS_CreateState ( 00065 NCGvoid **ppObj, 00066 NCGuint32 ui32StateID 00067 ); 00068 00069 NCGint32 00070 NCGSYS_DestroyState ( 00071 NCGvoid *pObj 00072 ); 00073 00074 NCGint32 00075 NCGSYS_SetState ( 00076 NCGvoid *pObj, 00077 NCGuint32 ui32State, 00078 NCGuint32 ui32Flags 00079 ); 00080 00081 NCGuint32 00082 NCGSYS_GetState ( 00083 NCGvoid *pObj, 00084 NCGuint32 ui32Flags 00085 ); 00086 00087 NCGint32 00088 NCGSYS_WaitState ( 00089 NCGvoid *pObj, 00090 NCGuint32 ui32State, 00091 NCGuint32 ui32Flags, 00092 NCGuint32 ui32Timeout 00093 ); 00094 00095 NCGvoid 00096 NCGSYS_SetStateEventValue ( 00097 NCGvoid *pObj, 00098 NCGuint32 ui32EventValue 00099 ); 00100 00101 NCGvoid * 00102 NCGSYS_GetLastCreatedState(void); 00103 00104 00105 #ifdef __cplusplus 00106 } 00107 #endif 00108 00109 #endif /* NCG_STATE_H */
Generated on Tue Jul 12 2022 11:15:02 by
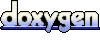