Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
locking_user.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /** 00024 * @file locking_user.h 00025 * @brief Lock related FIT BSP. User defined. 00026 * 00027 * $Module: OSPL $ $PublicVersion: 0.90 $ (=R_OSPL_VERSION) 00028 * $Rev: 35 $ 00029 * $Date:: 2014-04-15 21:38:18 +0900#$ 00030 */ 00031 00032 #ifndef LOCKING_USER_H 00033 #define LOCKING_USER_H 00034 00035 00036 /****************************************************************************** 00037 Includes <System Includes> , "Project Includes" 00038 ******************************************************************************/ 00039 #include "r_typedefs.h" 00040 #include "locking_typedef.h" 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif /* __cplusplus */ 00045 00046 00047 /****************************************************************************** 00048 Typedef definitions 00049 ******************************************************************************/ 00050 00051 /** 00052 * @struct r_ospl_user_lock_t 00053 * @brief Example of user defined lock type 00054 */ 00055 typedef struct st_r_ospl_user_lock_t r_ospl_user_lock_t; 00056 struct st_r_ospl_user_lock_t { 00057 int UserLockMember; 00058 }; 00059 00060 00061 /****************************************************************************** 00062 Macro definitions 00063 ******************************************************************************/ 00064 00065 /** 00066 * @def BSP_CFG_USER_LOCKING_TYPE 00067 * @brief C-lock (FIT BSP portable) 00068 * @par Parameters 00069 * None 00070 * @return None. 00071 * 00072 * @par Description 00073 * The value can be changed. 00074 * This is enabled, when "BSP_CFG_USER_LOCKING_ENABLED == 1". 00075 */ 00076 #define BSP_CFG_USER_LOCKING_TYPE r_ospl_user_lock_t 00077 00078 00079 /** 00080 * @def BSP_CFG_USER_LOCKING_SW_LOCK_FUNCTION 00081 * @brief Start C-lock for software module 00082 * @par Parameters 00083 * None 00084 * @return None. 00085 * 00086 * @par Description 00087 * The value can be changed. 00088 * This is enabled, when "BSP_CFG_USER_LOCKING_ENABLED == 1". 00089 */ 00090 #define BSP_CFG_USER_LOCKING_SW_LOCK_FUNCTION R_OSPL_USER_LOCK_SoftwareLock 00091 00092 00093 /** 00094 * @def BSP_CFG_USER_LOCKING_SW_UNLOCK_FUNCTION 00095 * @brief End C-lock for software module 00096 * @par Parameters 00097 * None 00098 * @return None. 00099 * 00100 * @par Description 00101 * The value can be changed. 00102 * This is enabled, when "BSP_CFG_USER_LOCKING_ENABLED == 1". 00103 */ 00104 #define BSP_CFG_USER_LOCKING_SW_UNLOCK_FUNCTION R_OSPL_USER_LOCK_SoftwareUnlock 00105 00106 00107 /** 00108 * @def BSP_CFG_USER_LOCKING_HW_LOCK_FUNCTION 00109 * @brief Start C-lock for hardware module 00110 * @par Parameters 00111 * None 00112 * @return None. 00113 * 00114 * @par Description 00115 * The value can be changed. 00116 * This is enabled, when "BSP_CFG_USER_LOCKING_ENABLED == 1". 00117 */ 00118 #define BSP_CFG_USER_LOCKING_HW_LOCK_FUNCTION R_OSPL_USER_LOCK_HardwareLock 00119 00120 00121 /** 00122 * @def BSP_CFG_USER_LOCKING_HW_UNLOCK_FUNCTION 00123 * @brief End C-lock for hardware module 00124 * @par Parameters 00125 * None 00126 * @return None. 00127 * 00128 * @par Description 00129 * The value can be changed. 00130 * This is enabled, when "BSP_CFG_USER_LOCKING_ENABLED == 1". 00131 */ 00132 #define BSP_CFG_USER_LOCKING_HW_UNLOCK_FUNCTION R_OSPL_USER_LOCK_HardwareUnlock 00133 00134 00135 /****************************************************************************** 00136 Variable Externs 00137 ******************************************************************************/ 00138 00139 /****************************************************************************** 00140 Functions Prototypes 00141 ******************************************************************************/ 00142 00143 /** 00144 * @brief Example of BSP_CFG_USER_LOCKING_SW_LOCK_FUNCTION 00145 * 00146 * @par Parameters 00147 * None 00148 * @return None. 00149 */ 00150 #if BSP_CFG_USER_LOCKING_ENABLED == 1 00151 bool_t R_OSPL_USER_LOCK_SoftwareLock( r_ospl_user_lock_t *LockObject ); 00152 #endif 00153 00154 00155 /** 00156 * @brief Example of BSP_CFG_USER_LOCKING_SW_UNLOCK_FUNCTION 00157 * 00158 * @par Parameters 00159 * None 00160 * @return None. 00161 */ 00162 #if BSP_CFG_USER_LOCKING_ENABLED == 1 00163 bool_t R_OSPL_USER_LOCK_SoftwareUnlock( r_ospl_user_lock_t *LockObject ); 00164 #endif 00165 00166 00167 /** 00168 * @brief Example of BSP_CFG_USER_LOCKING_HW_LOCK_FUNCTION 00169 * 00170 * @par Parameters 00171 * None 00172 * @return None. 00173 */ 00174 #if BSP_CFG_USER_LOCKING_ENABLED == 1 00175 bool_t R_OSPL_USER_LOCK_HardwareLock( mcu_lock_t HardwareIndex ); 00176 #endif 00177 00178 00179 /** 00180 * @brief Example of BSP_CFG_USER_LOCKING_HW_UNLOCK_FUNCTION 00181 * 00182 * @par Parameters 00183 * None 00184 * @return None. 00185 */ 00186 #if BSP_CFG_USER_LOCKING_ENABLED == 1 00187 bool_t R_OSPL_USER_LOCK_HardwareUnlock( mcu_lock_t HardwareIndex ); 00188 #endif 00189 00190 00191 /** 00192 * @brief For Test 00193 * 00194 * @par Parameters 00195 * None 00196 * @return None. 00197 */ 00198 #if BSP_CFG_USER_LOCKING_ENABLED == 1 00199 r_ospl_user_lock_t *R_OSPL_GetHardwareLockObjectForTest( mcu_lock_t HardwareIndex ); 00200 #endif 00201 00202 00203 /*********************************************************************** 00204 * End of File: 00205 ************************************************************************/ 00206 #ifdef __cplusplus 00207 } /* extern "C" */ 00208 #endif /* __cplusplus */ 00209 00210 #endif /* LOCKING_USER_H */ 00211
Generated on Tue Jul 12 2022 11:15:01 by
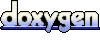