Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
locking.c
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /** 00024 * @file locking.c 00025 * @brief Lock related FIT BSP. 00026 * 00027 * $Module: OSPL $ $PublicVersion: 0.90 $ (=R_OSPL_VERSION) 00028 * $Rev: 35 $ 00029 * $Date:: 2014-04-15 21:38:18 +0900#$ 00030 */ 00031 00032 00033 /****************************************************************************** 00034 Includes <System Includes> , "Project Includes" 00035 ******************************************************************************/ 00036 #include "r_ospl.h" 00037 #if R_OSPL_IS_PREEMPTION 00038 #include "r_ospl_RTX_private.h" 00039 #endif 00040 #if IS_RZ_A1_BSP_USED 00041 #include "dma_if.h" /* R_DMA_Alloc */ 00042 #endif 00043 00044 00045 /****************************************************************************** 00046 Typedef definitions 00047 ******************************************************************************/ 00048 00049 /****************************************************************************** 00050 Macro definitions 00051 ******************************************************************************/ 00052 00053 /****************************************************************************** 00054 Imported global variables and functions (from other files) 00055 ******************************************************************************/ 00056 00057 /****************************************************************************** 00058 Exported global variables and functions (to be accessed by other files) 00059 ******************************************************************************/ 00060 00061 /****************************************************************************** 00062 Private global variables and functions 00063 ******************************************************************************/ 00064 00065 /** gs_ospl_mutex */ 00066 #if R_OSPL_IS_PREEMPTION 00067 static osMutexId gs_ospl_mutex; /* gs_OSPL_Mutex */ 00068 #endif 00069 00070 00071 /** g_bsp_Locks */ 00072 BSP_CFG_USER_LOCKING_TYPE g_bsp_Locks[ BSP_NUM_LOCKS ]; 00073 00074 00075 /*********************************************************************** 00076 * Implement: R_BSP_HardwareLock 00077 ************************************************************************/ 00078 bool_t R_BSP_HardwareLock( mcu_lock_t const HardwareIndex ) 00079 { 00080 #if BSP_CFG_USER_LOCKING_ENABLED 00081 return BSP_CFG_USER_LOCKING_HW_LOCK_FUNCTION( HardwareIndex ); 00082 #else 00083 bool_t is_success; 00084 00085 00086 #if IS_RZ_A1_BSP_USED 00087 if ( HardwareIndex >= BSP_LOCK_DMAC0 && HardwareIndex <= BSP_LOCK_DMAC15 ) { 00088 int_fast32_t channel_of_DMAC = HardwareIndex - BSP_LOCK_DMAC0; 00089 00090 channel_of_DMAC = R_DMA_Alloc( channel_of_DMAC, NULL ); 00091 IF ( channel_of_DMAC == -1 ) { 00092 is_success = false; 00093 goto fin; 00094 } 00095 00096 is_success = true; 00097 goto fin; 00098 } 00099 #endif 00100 00101 IF_D ( R_CUT_IF_ALWAYS_FALSE( HardwareIndex < 0u ||) HardwareIndex >= R_COUNT_OF( g_bsp_Locks ) ) { 00102 is_success = false; 00103 goto fin; 00104 } 00105 00106 is_success = R_BSP_SoftwareLock( &g_bsp_Locks[ HardwareIndex ] ); 00107 IF ( ! is_success ) { 00108 goto fin; 00109 } 00110 00111 is_success = true; 00112 fin: 00113 return is_success; 00114 #endif 00115 } 00116 00117 00118 /*********************************************************************** 00119 * Implement: R_OSPL_LockUnlockedChannel 00120 ************************************************************************/ 00121 #if ! BSP_CFG_USER_LOCKING_ENABLED 00122 errnum_t R_OSPL_LockUnlockedChannel( int_fast32_t *out_ChannelNum, 00123 mcu_lock_t HardwareIndexMin, mcu_lock_t HardwareIndexMax ) 00124 { 00125 errnum_t e; 00126 mcu_lock_t hardware_index; 00127 #if R_OSPL_IS_PREEMPTION 00128 bool_t is_lock = false; 00129 #endif 00130 00131 #if R_OSPL_IS_PREEMPTION 00132 if ( R_OSPL_THREAD_GetCurrentId() == NULL ) { /* If interrrupt context */ 00133 e = E_NOT_THREAD; 00134 goto fin; 00135 } 00136 e= R_OSPL_Start_T_Lock(); 00137 if ( e != 0 ) { 00138 R_OSPL_RaiseUnrecoverable( e ); 00139 goto fin; 00140 } 00141 is_lock = true; /* T-Lock to "self" */ 00142 #endif 00143 00144 00145 #if IS_RZ_A1_BSP_USED 00146 if ( HardwareIndexMin == BSP_LOCK_DMAC0 ) { 00147 int_fast32_t channel_of_DMAC; 00148 00149 channel_of_DMAC = R_DMA_Alloc( DMA_ALLOC_CH, NULL ); 00150 IF ( channel_of_DMAC == -1 ) { 00151 e = E_FEW_ARRAY; 00152 goto fin; 00153 } 00154 00155 *out_ChannelNum = channel_of_DMAC; 00156 e = 0; 00157 goto fin; 00158 } 00159 #endif 00160 00161 00162 for ( hardware_index = HardwareIndexMin; hardware_index <= HardwareIndexMax; 00163 hardware_index += 1 ) { 00164 r_ospl_c_lock_t *lock = &g_bsp_Locks[ hardware_index ]; 00165 00166 if ( ! lock->IsLocked ) { 00167 lock->IsLocked = true; 00168 break; 00169 } 00170 } 00171 IF ( hardware_index > HardwareIndexMax ) { 00172 e=E_FEW_ARRAY; 00173 goto fin; 00174 } 00175 00176 00177 *out_ChannelNum = hardware_index - HardwareIndexMin; 00178 00179 00180 e=0; 00181 fin: 00182 #if R_OSPL_IS_PREEMPTION 00183 if ( IS( is_lock ) ) { 00184 R_OSPL_End_T_Lock(); 00185 } 00186 #endif 00187 00188 return e; 00189 } 00190 #endif 00191 00192 00193 /*********************************************************************** 00194 * Implement: R_BSP_HardwareUnlock 00195 ************************************************************************/ 00196 bool_t R_BSP_HardwareUnlock( mcu_lock_t const HardwareIndex ) 00197 { 00198 #if BSP_CFG_USER_LOCKING_ENABLED 00199 return BSP_CFG_USER_LOCKING_HW_UNLOCK_FUNCTION( HardwareIndex ); 00200 #else 00201 bool_t is_success; 00202 00203 00204 #if IS_RZ_A1_BSP_USED 00205 if ( HardwareIndex >= BSP_LOCK_DMAC0 && HardwareIndex <= BSP_LOCK_DMAC15 ) { 00206 int_fast32_t channel_of_DMAC = HardwareIndex - BSP_LOCK_DMAC0; 00207 int_fast32_t err2; 00208 00209 err2 = R_DMA_Free( channel_of_DMAC, NULL ); 00210 IF ( err2 != ESUCCESS ) { 00211 is_success = false; 00212 goto fin; 00213 } 00214 00215 is_success = true; 00216 goto fin; 00217 } 00218 #endif 00219 00220 00221 IF_D ( R_CUT_IF_ALWAYS_FALSE( HardwareIndex < 0u ||) HardwareIndex >= R_COUNT_OF( g_bsp_Locks ) ) { 00222 is_success = false; 00223 R_OSPL_RaiseUnrecoverable( E_FEW_ARRAY ); 00224 goto fin; 00225 } 00226 00227 is_success = R_BSP_SoftwareUnlock( &g_bsp_Locks[ HardwareIndex ] ); 00228 IF ( ! is_success ) { 00229 goto fin; 00230 } 00231 00232 is_success = true; 00233 fin: 00234 return is_success; 00235 #endif 00236 } 00237 00238 00239 /*********************************************************************** 00240 * Implement: R_BSP_SoftwareLock 00241 ************************************************************************/ 00242 bool_t R_BSP_SoftwareLock( BSP_CFG_USER_LOCKING_TYPE *const LockObject ) 00243 { 00244 #if BSP_CFG_USER_LOCKING_ENABLED 00245 return BSP_CFG_USER_LOCKING_SW_LOCK_FUNCTION( LockObject ); 00246 #else 00247 errnum_t e; 00248 #if R_OSPL_IS_PREEMPTION 00249 bool_t is_lock = false; 00250 #endif 00251 00252 #if R_OSPL_IS_PREEMPTION 00253 if ( R_OSPL_THREAD_GetCurrentId() != NULL ) { /* If not interrrupt context */ 00254 e= R_OSPL_Start_T_Lock(); 00255 IF(e!=0) { 00256 goto fin; 00257 } 00258 is_lock = true; /* T-Lock to "self" */ 00259 } 00260 #endif 00261 00262 00263 e= R_OSPL_C_LOCK_Lock( LockObject ); 00264 if ( e == E_ACCESS_DENIED ) { 00265 R_OSPL_CLEAR_ERROR(); 00266 goto fin; 00267 } 00268 IF(e) { 00269 goto fin; 00270 } 00271 00272 e=0; 00273 fin: 00274 #if R_OSPL_IS_PREEMPTION 00275 if ( IS( is_lock ) ) { 00276 R_OSPL_End_T_Lock(); 00277 } 00278 #endif 00279 00280 return (bool_t)( e == 0 ); 00281 #endif 00282 } 00283 00284 00285 /*********************************************************************** 00286 * Implement: R_BSP_SoftwareUnlock 00287 ************************************************************************/ 00288 bool_t R_BSP_SoftwareUnlock( BSP_CFG_USER_LOCKING_TYPE *const LockObject ) 00289 { 00290 #if BSP_CFG_USER_LOCKING_ENABLED 00291 return BSP_CFG_USER_LOCKING_SW_UNLOCK_FUNCTION( LockObject ); 00292 #else 00293 errnum_t e; 00294 #if R_OSPL_IS_PREEMPTION 00295 bool_t is_lock = false; 00296 #endif 00297 00298 #if R_OSPL_IS_PREEMPTION 00299 if ( R_OSPL_THREAD_GetCurrentId() != NULL ) { /* If not interrrupt context */ 00300 e= R_OSPL_Start_T_Lock(); 00301 IF ( e != 0 ) { 00302 R_OSPL_RaiseUnrecoverable( e ); 00303 goto fin; 00304 } 00305 is_lock = true; /* T-Lock to "self" */ 00306 } 00307 #endif 00308 00309 00310 e= R_OSPL_C_LOCK_Unlock( LockObject ); 00311 if ( e == E_ACCESS_DENIED ) { 00312 R_OSPL_CLEAR_ERROR(); 00313 goto fin; 00314 } 00315 IF(e) { 00316 goto fin; 00317 } 00318 00319 e=0; 00320 fin: 00321 #if R_OSPL_IS_PREEMPTION 00322 if ( IS( is_lock ) ) { 00323 R_OSPL_End_T_Lock(); 00324 } 00325 #endif 00326 00327 return (bool_t)( e == 0 ); 00328 #endif 00329 } 00330 00331 00332 /** 00333 * @brief The function callbacked from OSPL internal, when T-Lock started 00334 * 00335 * @par Parameters 00336 * None 00337 * @return Error Code. 0=No Error. 00338 */ 00339 #if R_OSPL_IS_PREEMPTION 00340 errnum_t R_OSPL_Start_T_Lock(void) 00341 { 00342 errnum_t e; 00343 osStatus es; 00344 00345 static osMutexDef( gs_ospl_mutex ); 00346 00347 if ( gs_ospl_mutex == NULL ) { 00348 gs_ospl_mutex = osMutexCreate( osMutex( gs_ospl_mutex ) ); 00349 if ( gs_ospl_mutex == NULL ) { 00350 e=E_OTHERS; 00351 goto fin; 00352 } 00353 } 00354 00355 es= osMutexWait( gs_ospl_mutex, osWaitForever ); 00356 if ( es == osErrorISR ) { 00357 es = osOK; 00358 } 00359 if ( es != osOK ) { 00360 e=E_OTHERS; 00361 goto fin; 00362 } 00363 00364 e=0; 00365 fin: 00366 return e; 00367 } 00368 #endif 00369 00370 00371 /** 00372 * @brief The function callbacked from OSPL internal, when T-Lock ended 00373 * 00374 * @par Parameters 00375 * None 00376 * @return None 00377 */ 00378 #if R_OSPL_IS_PREEMPTION 00379 void R_OSPL_End_T_Lock(void) 00380 { 00381 if ( gs_ospl_mutex != NULL ) { 00382 osStatus rs; 00383 00384 rs= osMutexRelease( gs_ospl_mutex ); 00385 if ( rs == osErrorISR ) { 00386 rs = osOK; 00387 } 00388 ASSERT_R( rs == osOK, R_OSPL_RaiseUnrecoverable( E_OTHERS ) ); 00389 } 00390 } 00391 #endif 00392 00393
Generated on Tue Jul 12 2022 11:15:01 by
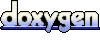