Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
jcu_pl.c
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2014 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /******************************************************************************* 00024 * $FileName: jcu_pl.c $ 00025 * $Module: JCU $ $PublicVersion: 1.00 $ (=JCU_VERSION) 00026 * $Rev: 35 $ 00027 * $Date:: 2014-02-26 13:18:53 +0900#$ 00028 * Description : JCU driver porting layer 00029 ******************************************************************************/ 00030 00031 00032 /****************************************************************************** 00033 Includes <System Includes> , "Project Includes" 00034 ******************************************************************************/ 00035 #include "r_ospl.h" 00036 #include "iodefine.h" 00037 #include "r_jcu_api.h" 00038 #include "r_jcu_pl.h" 00039 00040 /****************************************************************************** 00041 Typedef definitions 00042 ******************************************************************************/ 00043 typedef struct st_jcu_pl_t jcu_pl_t; 00044 00045 /*[jcu_pl_t]*/ 00046 struct st_jcu_pl_t { 00047 const r_ospl_caller_t *InterruptCallbackCaller; 00048 const jcu_async_status_t *Status; 00049 }; 00050 00051 /*[gs_jcu_pl]*/ 00052 static jcu_pl_t gs_jcu_pl 00053 ; 00054 00055 /****************************************************************************** 00056 Macro definitions 00057 ******************************************************************************/ 00058 enum { JCU_INT_PRI = 2 }; 00059 #define CPG_JCU_CLOCK_POWER_OFF 0x00000002u 00060 00061 /****************************************************************************** 00062 Imported global variables and functions (from other files) 00063 ******************************************************************************/ 00064 00065 /****************************************************************************** 00066 Exported global variables and functions (to be accessed by other files) 00067 ******************************************************************************/ 00068 00069 /****************************************************************************** 00070 Private global variables and functions 00071 ******************************************************************************/ 00072 static void JCU_IRQ_JEDI_Handler(void); 00073 static void JCU_IRQ_JDTI_Handler(void); 00074 00075 /*[gs_jedi_interrupt_context] JEDI interrupt context */ 00076 /*[gs_jdti_interrupt_context] JDTI interrupt context */ 00077 static const r_ospl_interrupt_t gs_jedi_interrupt_context = { BSP_INT_SRC_JEDI, 0 }; 00078 static const r_ospl_interrupt_t gs_jdti_interrupt_context = { BSP_INT_SRC_JDTI, 0 }; 00079 00080 00081 /**************************************************************************//** 00082 * Function Name: [R_JCU_SetDefaultAsync] 00083 * @retval None 00084 ******************************************************************************/ 00085 void R_JCU_SetDefaultAsync( r_ospl_async_t *const Async, r_ospl_async_type_t AsyncType ) 00086 { 00087 IF_DQ( Async == NULL ) { 00088 goto fin; 00089 } 00090 00091 if ( IS_BIT_NOT_SET( Async->Flags, R_F_OSPL_A_Thread ) ) { 00092 Async->A_Thread = NULL; 00093 } 00094 00095 if ( IS_BIT_NOT_SET( Async->Flags, R_F_OSPL_A_EventValue ) ) { 00096 if ( AsyncType == R_OSPL_ASYNC_TYPE_NORMAL ) { 00097 Async->A_EventValue = R_OSPL_A_FLAG; 00098 } else { 00099 Async->A_EventValue = R_OSPL_FINAL_A_FLAG; 00100 } 00101 } else { 00102 ASSERT_D( IS_BIT_SET( Async->Flags, R_F_OSPL_A_Thread ), R_NOOP() ); 00103 } 00104 00105 if ( IS_BIT_NOT_SET( Async->Flags, R_F_OSPL_I_Thread ) ) { 00106 Async->I_Thread = NULL; 00107 } 00108 00109 if ( IS_BIT_NOT_SET( Async->Flags, R_F_OSPL_I_EventValue ) ) { 00110 Async->I_EventValue = R_OSPL_I_FLAG; 00111 } else { 00112 ASSERT_D( IS_BIT_SET( Async->Flags, R_F_OSPL_I_Thread ), R_NOOP() ); 00113 } 00114 00115 if ( IS_BIT_NOT_SET( Async->Flags, R_F_OSPL_InterruptCallback ) ) { 00116 Async->InterruptCallback = &( R_JCU_OnInterruptDefault ); /* MISRA 16.9 */ 00117 } 00118 00119 Async->Flags = R_F_OSPL_A_Thread | R_F_OSPL_A_EventValue | 00120 R_F_OSPL_I_Thread | R_F_OSPL_I_EventValue | 00121 R_F_OSPL_InterruptCallback | R_F_OSPL_Delegate; 00122 fin: 00123 return; 00124 } 00125 00126 00127 /**************************************************************************//** 00128 * Function Name: [R_JCU_OnInitialize] 00129 * @retval Error code, 0=No error 00130 ******************************************************************************/ 00131 errnum_t R_JCU_OnInitialize(void) 00132 { 00133 errnum_t e; 00134 bsp_int_err_t eb; 00135 bsp_int_src_t const num_of_JEDI_IRQ = gs_jedi_interrupt_context.IRQ_Num; 00136 bsp_int_src_t const num_of_JDTI_IRQ = gs_jdti_interrupt_context.IRQ_Num; 00137 jcu_pl_t *const self = &gs_jcu_pl; 00138 00139 self->InterruptCallbackCaller = NULL; 00140 self->Status = NULL; 00141 00142 00143 /* Register "JEDI" */ 00144 eb= R_BSP_InterruptWrite( num_of_JEDI_IRQ, &( JCU_IRQ_JEDI_Handler ) ); /* MISRA 16.9 */ 00145 IF ( eb != 0 ) { 00146 e=E_OTHERS; 00147 goto fin; 00148 } 00149 00150 e= R_OSPL_SetInterruptPriority( num_of_JEDI_IRQ, JCU_INT_PRI ); 00151 IF ( e != 0 ) { 00152 goto fin; 00153 } 00154 00155 00156 /* Register "JDTI" */ 00157 eb= R_BSP_InterruptWrite( num_of_JDTI_IRQ, &( JCU_IRQ_JDTI_Handler ) ); /* MISRA 16.9 */ 00158 IF ( eb != 0 ) { 00159 e=E_OTHERS; 00160 goto fin; 00161 } 00162 00163 e= R_OSPL_SetInterruptPriority( num_of_JDTI_IRQ, JCU_INT_PRI ); 00164 IF ( e != 0 ) { 00165 goto fin; 00166 } 00167 00168 00169 /* start to suuply the clock for JCU */ 00170 { /* ->QAC 0306 */ 00171 uint32_t cpg_reg; 00172 cpg_reg = (uint32_t)(CPG.STBCR6) & ~CPG_JCU_CLOCK_POWER_OFF; 00173 CPG.STBCR6 =(uint8_t)cpg_reg; 00174 } /* <-QAC 0306 */ 00175 00176 e=0; 00177 fin: 00178 return e; 00179 } 00180 00181 00182 /**************************************************************************//** 00183 * Function Name: [R_JCU_OnFinalize] 00184 * @retval Error code, 0=No error and e=0 00185 ******************************************************************************/ 00186 /* ->QAC 3227 : "e" is usually changed in finalize function. */ 00187 errnum_t R_JCU_OnFinalize( errnum_t e ) 00188 /* <-QAC 3227 */ 00189 { 00190 /* stop to suuply the clock for JCU */ 00191 { /* ->QAC 0306 */ 00192 uint32_t cpg_reg; 00193 cpg_reg = (uint32_t)(CPG.STBCR6) | CPG_JCU_CLOCK_POWER_OFF; 00194 CPG.STBCR6 = (uint8_t)cpg_reg; 00195 } /* <-QAC 0306 */ 00196 00197 return e; 00198 } 00199 00200 00201 /****************************************************************************** 00202 * Function Name: [R_JCU_SetInterruptCallbackCaller] 00203 * @retval Error code, 0=No error 00204 ******************************************************************************/ 00205 errnum_t R_JCU_SetInterruptCallbackCaller( const r_ospl_caller_t *const Caller ) 00206 { 00207 jcu_pl_t *const self = &gs_jcu_pl; 00208 00209 self->InterruptCallbackCaller = Caller; 00210 00211 return 0; 00212 } 00213 00214 00215 /****************************************************************************** 00216 * Function Name: [R_JCU_OnEnableInterrupt] 00217 * @retval None 00218 ******************************************************************************/ 00219 void R_JCU_OnEnableInterrupt( jcu_interrupt_lines_t const Enables ) 00220 { 00221 bsp_int_err_t eb; 00222 00223 if ( IS_BIT_SET( Enables, JCU_INTERRUPT_LINE_JEDI ) ) { 00224 bsp_int_src_t const num_of_IRQ = gs_jedi_interrupt_context.IRQ_Num; 00225 00226 eb= R_BSP_InterruptControl( num_of_IRQ, BSP_INT_CMD_INTERRUPT_ENABLE, FIT_NO_PTR ); 00227 ASSERT_D( eb == 0, R_NOOP() ); 00228 R_UNREFERENCED_VARIABLE( eb ); /* for Release configuration */ 00229 } 00230 00231 if ( IS_BIT_SET( Enables, JCU_INTERRUPT_LINE_JDTI ) ) { 00232 bsp_int_src_t const num_of_IRQ = gs_jdti_interrupt_context.IRQ_Num; 00233 00234 eb= R_BSP_InterruptControl( num_of_IRQ, BSP_INT_CMD_INTERRUPT_ENABLE, FIT_NO_PTR ); 00235 ASSERT_D( eb == 0, R_NOOP() ); 00236 R_UNREFERENCED_VARIABLE( eb ); /* for Release configuration */ 00237 } 00238 } 00239 00240 00241 /****************************************************************************** 00242 * Function Name: [R_JCU_OnDisableInterrupt] 00243 * @retval None 00244 ******************************************************************************/ 00245 void R_JCU_OnDisableInterrupt( jcu_interrupt_lines_t const Disables1 ) 00246 { 00247 bsp_int_err_t eb; 00248 00249 if ( IS_BIT_SET( Disables1, JCU_INTERRUPT_LINE_JEDI ) ) { 00250 bsp_int_src_t const num_of_IRQ = gs_jedi_interrupt_context.IRQ_Num; 00251 00252 eb= R_BSP_InterruptControl( num_of_IRQ, BSP_INT_CMD_INTERRUPT_DISABLE, FIT_NO_PTR ); 00253 ASSERT_D( eb == 0, R_NOOP() ); 00254 R_UNREFERENCED_VARIABLE( eb ); /* for Release configuration */ 00255 } 00256 00257 if ( IS_BIT_SET( Disables1, JCU_INTERRUPT_LINE_JDTI ) ) { 00258 bsp_int_src_t const num_of_IRQ = gs_jdti_interrupt_context.IRQ_Num; 00259 00260 eb= R_BSP_InterruptControl( num_of_IRQ, BSP_INT_CMD_INTERRUPT_DISABLE, FIT_NO_PTR ); 00261 ASSERT_D( eb == 0, R_NOOP() ); 00262 R_UNREFERENCED_VARIABLE( eb ); /* for Release configuration */ 00263 } 00264 } 00265 00266 00267 /****************************************************************************** 00268 * Function Name: [R_JCU_OnInterruptDefault] 00269 * @retval Error code, 0=No error 00270 ******************************************************************************/ 00271 errnum_t R_JCU_OnInterruptDefault( const r_ospl_interrupt_t *const InterruptSource, 00272 const r_ospl_caller_t *const Caller ) 00273 { 00274 errnum_t e; 00275 errnum_t ee; 00276 r_ospl_async_t *async; 00277 00278 e = 0; 00279 R_AVOID_UNSAFE_ALWAYS_WARNING( e ); 00280 00281 IF_DQ( Caller == NULL ) { 00282 goto fin; 00283 } 00284 00285 ee= R_JCU_OnInterrupting( InterruptSource ); 00286 IF ( (ee != 0) && (e == 0) ) { 00287 e = ee; 00288 } 00289 00290 async = Caller->Async; 00291 00292 if ( async->I_Thread == NULL ) { 00293 ee= R_JCU_OnInterrupted(); 00294 IF ( (ee != 0) && (e == 0) ) { 00295 e = ee; 00296 } 00297 } else { 00298 R_OSPL_EVENT_Set( async->I_Thread, async->I_EventValue ); 00299 } 00300 00301 fin: 00302 return e; 00303 } 00304 00305 00306 /**************************************************************************//** 00307 * Function Name: [JCU_IRQ_JEDI_Handler] 00308 * @brief JEDI (JCU Encode Decode Interrupt) interrupt handler 00309 * @retval None 00310 ******************************************************************************/ 00311 static void JCU_IRQ_JEDI_Handler(void) 00312 { 00313 jcu_pl_t *const self = &gs_jcu_pl; 00314 const r_ospl_interrupt_t *const i_context = &gs_jedi_interrupt_context; 00315 00316 R_OSPL_CallInterruptCallback( self->InterruptCallbackCaller, i_context ); 00317 } 00318 00319 00320 /**************************************************************************//** 00321 * Function Name: [JCU_IRQ_JDTI_Handler] 00322 * @brief JDTI (JCU Data Transfer Interrupt) interrupt handler 00323 * @retval None 00324 ******************************************************************************/ 00325 static void JCU_IRQ_JDTI_Handler(void) 00326 { 00327 jcu_pl_t *const self = &gs_jcu_pl; 00328 const r_ospl_interrupt_t *const i_context = &gs_jdti_interrupt_context; 00329 00330 R_OSPL_CallInterruptCallback( self->InterruptCallbackCaller, i_context ); 00331 } 00332 00333
Generated on Tue Jul 12 2022 11:15:01 by
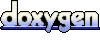