Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
jcu_api.c
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /******************************************************************************* 00024 * $FileName: jcu_api.c $ 00025 * $Module: JCU $ $PublicVersion: 1.00 $ (=JCU_VERSION) 00026 * $Rev: 35 $ 00027 * $Date:: 2014-02-26 13:18:53 +0900#$ 00028 * Description : JCU driver API 00029 ******************************************************************************/ 00030 /** 00031 * @file jcu_api.c 00032 * @brief JCU (JPEG hardware) driver API. Main Code. 00033 * 00034 * $Module: JCU $ $PublicVersion: 1.00 $ (=JCU_VERSION) 00035 * $Rev: 38 $ 00036 * $Date:: 2014-03-18 16:14:45 +0900#$ 00037 */ 00038 00039 /****************************************************************************** 00040 Includes <System Includes> , "Project Includes" 00041 ******************************************************************************/ 00042 #include <string.h> 00043 #include "r_typedefs.h" 00044 #include "iodefine.h" 00045 #include "r_ospl.h" 00046 #include "r_jcu_api.h" 00047 #include "r_jcu_local.h" 00048 #include "r_jcu_pl.h" 00049 00050 /****************************************************************************** 00051 Typedef definitions 00052 ******************************************************************************/ 00053 00054 /****************************************************************************** 00055 Macro definitions 00056 ******************************************************************************/ 00057 00058 /****************************************************************************** 00059 Imported global variables and functions (from other files) 00060 ******************************************************************************/ 00061 00062 /****************************************************************************** 00063 Exported global variables and functions (to be accessed by other files) 00064 ******************************************************************************/ 00065 /* Section: Internal Global */ 00066 00067 /** gs_jcu_internal_information */ 00068 static jcu_internal_information_t gs_jcu_internal_information; 00069 00070 00071 /** gs_jcu_i_lock */ 00072 static jcu_i_lock_t gs_jcu_i_lock; 00073 00074 00075 /****************************************************************************** 00076 Private global variables and functions 00077 ******************************************************************************/ 00078 00079 /*********************************************************************** 00080 * Class: jcu_i_lock_t 00081 ************************************************************************/ 00082 static void R_JCU_I_LOCK_Reset( jcu_i_lock_t *const self ); 00083 static bool_t R_JCU_I_LOCK_Lock( void *const self_ ); 00084 static void R_JCU_I_LOCK_Unlock( void *const self_ ); 00085 static void R_JCU_I_LOCK_RequestFinalize( void *const self_ ); 00086 static int_fast32_t R_JCU_I_LOCK_GetRootChannelNum( const void *const self_ ); 00087 00088 00089 /* Section: Global */ 00090 /** 00091 * @brief Initialize the driver 00092 * 00093 * @param NullConfig (in) NULL 00094 * @return <jcu_errorcode_t> type. 00095 */ 00096 /* ->SEC M1.1.1 API Function */ 00097 jcu_errorcode_t R_JCU_Initialize( void *const NullConfig ) 00098 /* <-SEC M1.1.1 */ 00099 { 00100 errnum_t e; 00101 jcu_errorcode_t returnValue; 00102 jcu_internal_information_t *const self = &gs_jcu_internal_information; 00103 00104 static const r_ospl_i_lock_vtable_t gs_I_LockVTable = { 00105 &( R_JCU_I_LOCK_Lock ), /* MISRA 16.9 */ 00106 &( R_JCU_I_LOCK_Unlock ), 00107 &( R_JCU_I_LOCK_RequestFinalize ), 00108 &( R_JCU_I_LOCK_GetRootChannelNum ) 00109 }; 00110 00111 R_UNREFERENCED_VARIABLE( NullConfig ); 00112 R_IT_WILL_BE_NOT_CONST( NullConfig ); 00113 00114 if ( self->I_Lock == NULL ) { 00115 jcu_i_lock_t *const i_lock = &gs_jcu_i_lock; 00116 00117 self->Is_I_LockMaster = R_JCU_I_LOCK_Replace( 00118 i_lock, &gs_I_LockVTable ); 00119 } 00120 00121 if ( IS( self->Is_I_LockMaster ) ) { 00122 R_JCU_I_LOCK_Reset( self->I_Lock ); 00123 } 00124 R_OSPL_FLAG32_InitConst( &self->AsyncStatus.InterruptFlags ); 00125 R_OSPL_FLAG32_InitConst( &self->AsyncStatus.InterruptEnables ); 00126 R_OSPL_FLAG32_InitConst( &self->AsyncStatus.CancelFlags ); 00127 self->AsyncStatus.IsEnabledInterrupt = false; 00128 00129 /* Error check */ 00130 if (self->AsyncStatus.Status != JCU_STATUS_UNDEF) { 00131 returnValue = JCU_ERROR_STATUS; 00132 goto fin; 00133 } /* end if */ 00134 00135 /* Run the User-defined function if user set the function */ 00136 e= R_JCU_OnInitialize(); 00137 IF ( e != 0 ) { 00138 returnValue = JCU_ERROR_PARAM; 00139 goto fin; 00140 } 00141 00142 /* Initialize the variable */ 00143 self->AsyncStatus.IsPaused = false; 00144 self->AsyncStatus.SubStatusFlags = 0; 00145 self->IsCountMode = false; 00146 self->ErrorFilter = JCU_INT_ERROR_ALL; 00147 self->AsyncForFinalize = NULL; 00148 00149 /* Set the register */ 00150 returnValue = JCU_SetRegisterForInitialize(); 00151 00152 if (returnValue == JCU_ERROR_OK) { 00153 /* Set the updated status */ 00154 self->AsyncStatus.Status = JCU_STATUS_INIT; 00155 00156 /* Initialize the status for the encode */ 00157 self->Codec = JCU_CODEC_NOT_SELECTED; 00158 } /* end if */ 00159 00160 fin: 00161 return returnValue; 00162 } 00163 00164 00165 /** 00166 * @brief Terminate the driver function 00167 * 00168 * @par Parameters 00169 * None 00170 * @return <jcu_errorcode_t> type. 00171 */ 00172 /* ->SEC M1.1.1 API Function */ 00173 jcu_errorcode_t R_JCU_Terminate(void) 00174 /* <-SEC M1.1.1 */ 00175 { 00176 errnum_t e; 00177 jcu_errorcode_t ej; 00178 r_ospl_async_t async; 00179 bit_flags32_t got_flags; 00180 00181 async.Flags = R_F_OSPL_A_Thread; 00182 async.A_Thread = R_OSPL_THREAD_GetCurrentId(); 00183 00184 ej= R_JCU_TerminateAsync( &async ); 00185 IF( ej != JCU_ERROR_OK ) { 00186 goto fin; 00187 } 00188 00189 e= R_OSPL_EVENT_Wait( async.A_EventValue, &got_flags, R_OSPL_INFINITE ); 00190 IF(e!=0) { 00191 ej=JCU_ERROR_PARAM; 00192 goto fin; 00193 } 00194 00195 e= async.ReturnValue; 00196 IF(e!=0) { 00197 ej=JCU_ERROR_PARAM; 00198 goto fin; 00199 } 00200 00201 fin: 00202 return ej; 00203 } 00204 00205 00206 /** 00207 * @brief Terminate the driver function 00208 * 00209 * @param async <r_ospl_async_t> 00210 * @return <jcu_errorcode_t> type. 00211 */ 00212 /* ->SEC M1.1.1 API Function */ 00213 jcu_errorcode_t R_JCU_TerminateAsync( r_ospl_async_t *const async ) 00214 /* <-SEC M1.1.1 */ 00215 { 00216 jcu_errorcode_t returnValue = JCU_ERROR_OK; 00217 bool_t was_enabled = false; 00218 jcu_internal_information_t *const self = &gs_jcu_internal_information; 00219 00220 IF_DQ( async == NULL ) { 00221 returnValue = JCU_ERROR_PARAM; 00222 goto fin; 00223 } 00224 00225 R_JCU_SetDefaultAsync( async, R_OSPL_ASYNC_TYPE_FINALIZE ); 00226 async->ReturnValue = 0; 00227 00228 was_enabled = self->I_LockVTable->Lock( self->I_Lock ); 00229 00230 /* Check the status */ 00231 if ( self->AsyncStatus.Status == JCU_STATUS_UNDEF ) { 00232 returnValue = JCU_ERROR_OK; 00233 goto fin; 00234 } /* end if */ 00235 00236 if ( self->AsyncStatus.Status == JCU_STATUS_RUN ) { 00237 self->AsyncForFinalize = async; 00238 00239 R_OSPL_FLAG32_Set( &self->AsyncStatus.CancelFlags, R_OSPL_FINALIZE_REQUEST ); 00240 00241 /* "R_JCU_OnInterrupted" will be called */ 00242 } else { 00243 returnValue = R_JCU_TerminateStep2(); 00244 R_OSPL_EVENT_Set( async->A_Thread, async->A_EventValue ); 00245 } /* end if */ 00246 00247 fin: 00248 /* Finalize I-Lock */ 00249 if ( IS( was_enabled ) ) { 00250 self->I_LockVTable->Unlock( self->I_Lock ); 00251 00252 if ( IS( self->Is_I_LockMaster ) ) { 00253 if ( IS( gs_jcu_i_lock.IsRequestedFinalize ) ) { 00254 R_JCU_I_LOCK_Finalize(); 00255 } 00256 } 00257 } 00258 return returnValue; 00259 } 00260 00261 00262 /** 00263 * @brief Terminate the driver function 00264 * 00265 * @par Parameters 00266 * None 00267 * @return <jcu_errorcode_t> type. 00268 */ 00269 jcu_errorcode_t R_JCU_TerminateStep2(void) 00270 { 00271 errnum_t ee; 00272 jcu_errorcode_t returnValue = JCU_ERROR_OK; 00273 jcu_internal_information_t *const self = &gs_jcu_internal_information; 00274 00275 R_AVOID_UNSAFE_ALWAYS_WARNING( returnValue ); 00276 00277 00278 /* Set the updated status */ 00279 R_OSPL_FLAG32_Clear( &self->AsyncStatus.CancelFlags, R_OSPL_FLAG32_ALL_BITS ); 00280 self->AsyncStatus.Status = JCU_STATUS_UNDEF; 00281 self->I_LockVTable->RequestFinalize( self->I_Lock ); 00282 00283 00284 /* Run the additional function selected by parameter */ 00285 ee= R_JCU_OnFinalize( returnValue ); 00286 IF ( (ee != 0) && (returnValue == JCU_ERROR_OK) ) { 00287 returnValue = JCU_ERROR_PARAM; 00288 } 00289 00290 return returnValue; 00291 } 00292 00293 00294 /** 00295 * @brief Select Codec Type 00296 * 00297 * @param codec <jcu_codec_t> 00298 * @return <jcu_errorcode_t> type. 00299 */ 00300 /* ->SEC M1.1.1 API Function */ 00301 jcu_errorcode_t R_JCU_SelectCodec( 00302 const jcu_codec_t codec) 00303 /* <-SEC M1.1.1 */ 00304 { 00305 jcu_errorcode_t returnValue; 00306 bool_t was_enabled; /* = false; */ /* QAC 3197 */ 00307 jcu_internal_information_t *const self = &gs_jcu_internal_information; 00308 00309 was_enabled = self->I_LockVTable->Lock( self->I_Lock ); 00310 00311 #ifdef JCU_PARAMETER_CHECK 00312 IF ((self->AsyncStatus.Status == JCU_STATUS_UNDEF) 00313 || (self->AsyncStatus.Status == JCU_STATUS_RUN)) { 00314 returnValue = JCU_ERROR_STATUS; 00315 goto fin; 00316 } /* end if */ 00317 00318 returnValue = JCU_ParaCheckSelectCodec(codec); 00319 IF (returnValue != JCU_ERROR_OK) { 00320 goto fin; 00321 } 00322 #endif /* #Ifdef JCU_PARAMETER_CHECK_ */ 00323 00324 /* Clear the count mode flag */ 00325 self->IsCountMode = false; 00326 00327 /* Register set */ 00328 JCU_SetRegisterForCodec(codec); 00329 00330 /* Set the updated status */ 00331 self->AsyncStatus.Status = JCU_STATUS_SELECTED; 00332 00333 /* Set codec type to internal information variable */ 00334 switch (codec) { 00335 case JCU_ENCODE: 00336 self->Codec = JCU_STATUS_ENCODE; 00337 break; 00338 case JCU_DECODE: 00339 self->Codec = JCU_STATUS_DECODE; 00340 break; 00341 /* ->QAC 2018 : For MISRA 15.3, SEC R3.5.2 */ 00342 default: 00343 /* <-QAC 2018 */ 00344 R_NOOP(); /* NOT REACHED */ 00345 break; 00346 } /* end switch */ 00347 00348 returnValue = JCU_ERROR_OK; 00349 fin: 00350 if ( IS( was_enabled ) ) { 00351 self->I_LockVTable->Unlock( self->I_Lock ); 00352 } 00353 return returnValue; 00354 } 00355 00356 00357 /** 00358 * @brief Start decoding or encoding. Synchronized. 00359 * 00360 * @par Parameters 00361 * None 00362 * @return <jcu_errorcode_t> type. 00363 */ 00364 /* ->SEC M1.1.1 API Function */ 00365 jcu_errorcode_t R_JCU_Start( 00366 void) 00367 /* <-SEC M1.1.1 */ 00368 { 00369 errnum_t e; 00370 jcu_errorcode_t ej; 00371 r_ospl_async_t async; 00372 bit_flags32_t got_flags; 00373 00374 async.Flags = R_F_OSPL_A_Thread; 00375 async.A_Thread = R_OSPL_THREAD_GetCurrentId(); 00376 00377 ej= R_JCU_StartAsync( &async ); 00378 IF( ej != JCU_ERROR_OK ) { 00379 goto fin; 00380 } 00381 00382 e= R_OSPL_EVENT_Wait( async.A_EventValue, &got_flags, R_OSPL_INFINITE ); 00383 IF(e!=0) { 00384 ej=e; 00385 goto fin; 00386 } 00387 00388 e= async.ReturnValue; 00389 IF(e!=0) { 00390 ej=e; 00391 goto fin; 00392 } 00393 00394 fin: 00395 return ej; 00396 } 00397 00398 00399 /** 00400 * @brief Start decoding or encoding. Asynchronized. 00401 * 00402 * @par Parameters 00403 * None 00404 * @return <jcu_errorcode_t> type. 00405 */ 00406 /* ->SEC M1.1.1 API Function */ 00407 jcu_errorcode_t R_JCU_StartAsync( 00408 r_ospl_async_t *const async) 00409 /* <-SEC M1.1.1 */ 00410 { 00411 errnum_t e; 00412 jcu_errorcode_t returnValue; 00413 bool_t was_enabled; /* = false; */ /* QAC 3197 */ 00414 jcu_internal_information_t *const self = &gs_jcu_internal_information; 00415 00416 was_enabled = self->I_LockVTable->Lock( self->I_Lock ); 00417 00418 #ifdef JCU_PARAMETER_CHECK 00419 IF (self->AsyncStatus.Status != JCU_STATUS_READY) { 00420 returnValue = JCU_ERROR_STATUS; 00421 goto fin; 00422 } /* end if */ 00423 00424 returnValue = JCU_ParaCheckStart(); 00425 IF(returnValue != JCU_ERROR_OK) { 00426 goto fin; 00427 } /* end if */ 00428 00429 IF ( async == NULL ) { 00430 returnValue = JCU_ERROR_PARAM; 00431 goto fin; 00432 } /* end if */ 00433 #endif /* #Ifdef JCU_PARAMETER_CHECK_ */ 00434 00435 R_JCU_SetDefaultAsync( async, R_OSPL_ASYNC_TYPE_NORMAL ); 00436 async->ReturnValue = 0; 00437 00438 /* Attach "Async" to interrupt */ 00439 /* ->MISRA 11.4 : Not too big "enum" is same bit count as "int" */ /* ->SEC R2.7.1 */ 00440 R_OSPL_CALLER_Initialize( &self->InterruptCallbackCaller, 00441 async, (int_fast32_t *)&self->AsyncStatus.Status, JCU_STATUS_INTERRUPTING, 00442 self->I_Lock, self->I_LockVTable ); 00443 /* <-MISRA 11.4 */ /* <-SEC R2.7.1 */ 00444 e= R_JCU_SetInterruptCallbackCaller( &self->InterruptCallbackCaller ); 00445 IF ( e != 0 ) { 00446 returnValue = JCU_ERROR_PARAM; 00447 goto fin; 00448 } 00449 R_OSPL_FLAG32_Set( &self->AsyncStatus.InterruptEnables, JCU_INTERRUPT_LINE_ALL ); 00450 00451 /* Clear event flags */ 00452 R_OSPL_EVENT_Clear( async->A_Thread, async->A_EventValue ); 00453 R_OSPL_EVENT_Clear( async->I_Thread, async->I_EventValue ); 00454 00455 /* Set the updated status when register update is finished */ 00456 /* Change the status */ 00457 self->AsyncStatus.Status = JCU_STATUS_RUN; 00458 00459 /* Register set */ 00460 JCU_SetRegisterForStart( self->ErrorFilter ); 00461 00462 returnValue = JCU_ERROR_OK; 00463 fin: 00464 if ( IS( was_enabled ) ) { 00465 self->I_LockVTable->Unlock( self->I_Lock ); 00466 } 00467 return returnValue; 00468 } 00469 00470 00471 /** 00472 * @brief Set the count mode (separate operating) parameter 00473 * 00474 * @param buffer <jcu_count_mode_param_t> 00475 * @return <jcu_errorcode_t> type. 00476 */ 00477 /* ->SEC M1.1.1 API Function */ 00478 jcu_errorcode_t R_JCU_SetCountMode( 00479 const jcu_count_mode_param_t *const buffer) 00480 /* <-SEC M1.1.1 */ 00481 { 00482 jcu_errorcode_t returnValue; 00483 bool_t was_enabled; /* = false; */ /* QAC 3197 */ 00484 jcu_internal_information_t *const self = &gs_jcu_internal_information; 00485 00486 was_enabled = self->I_LockVTable->Lock( self->I_Lock ); 00487 00488 #ifdef JCU_PARAMETER_CHECK 00489 IF (!((self->AsyncStatus.Status == JCU_STATUS_SELECTED) 00490 || (self->AsyncStatus.Status == JCU_STATUS_READY))) { 00491 returnValue = JCU_ERROR_STATUS; 00492 goto fin; 00493 } /* end if */ 00494 00495 IF(buffer == NULL) { 00496 returnValue = JCU_ERROR_STATUS; 00497 goto fin; 00498 } /* end if */ 00499 00500 returnValue = JCU_ParaCheckSetCountMode(buffer); 00501 IF(returnValue != JCU_ERROR_OK) { 00502 goto fin; 00503 } /* end if */ 00504 #endif /* #Ifdef JCU_PARAMETER_CHECK_ */ 00505 00506 /* Set the count mode flag */ 00507 if ((buffer->inputBuffer.isEnable != false) || (buffer->outputBuffer.isEnable != false)) { 00508 self->IsCountMode = true; 00509 } else { 00510 self->IsCountMode = false; 00511 } /* end if */ 00512 00513 /* Register set */ 00514 JCU_SetRegisterForSetCountMode(buffer); 00515 00516 returnValue = JCU_ERROR_OK; 00517 fin: 00518 if ( IS( was_enabled ) ) { 00519 self->I_LockVTable->Unlock( self->I_Lock ); 00520 } 00521 return returnValue; 00522 } 00523 00524 00525 /** 00526 * @brief Restart the processing caused by count mode. Synchronized. 00527 * 00528 * @param type The target to continue 00529 * @return <jcu_errorcode_t> type. 00530 */ 00531 /* ->SEC M1.1.1 API Function */ 00532 jcu_errorcode_t R_JCU_Continue( 00533 const jcu_continue_type_t type) 00534 /* <-SEC M1.1.1 */ 00535 { 00536 errnum_t e; 00537 jcu_errorcode_t ej; 00538 r_ospl_async_t async; 00539 bit_flags32_t got_flags; 00540 00541 async.Flags = R_F_OSPL_A_Thread; 00542 async.A_Thread = R_OSPL_THREAD_GetCurrentId(); 00543 00544 ej= R_JCU_ContinueAsync( type, &async ); 00545 IF( ej != JCU_ERROR_OK ) { 00546 goto fin; 00547 } 00548 00549 e= R_OSPL_EVENT_Wait( async.A_EventValue, &got_flags, R_OSPL_INFINITE ); 00550 IF(e!=0) { 00551 ej=JCU_ERROR_PARAM; 00552 goto fin; 00553 } 00554 00555 e= async.ReturnValue; 00556 IF(e!=0) { 00557 ej=JCU_ERROR_PARAM; 00558 goto fin; 00559 } 00560 00561 fin: 00562 return ej; 00563 } 00564 00565 00566 /** 00567 * @brief Restart the processing caused by count mode. Asynchronized. 00568 * 00569 * @param type The target to continue 00570 * @return <jcu_errorcode_t> type. 00571 */ 00572 /* ->SEC M1.1.1 API Function */ 00573 jcu_errorcode_t R_JCU_ContinueAsync( 00574 const jcu_continue_type_t type, 00575 r_ospl_async_t *const async) 00576 /* <-SEC M1.1.1 */ 00577 { 00578 errnum_t e; 00579 jcu_errorcode_t returnValue; 00580 bool_t was_enabled; /* = false; */ /* QAC 3197 */ 00581 bit_flags32_t mask; 00582 jcu_internal_information_t *const self = &gs_jcu_internal_information; 00583 00584 was_enabled = self->I_LockVTable->Lock( self->I_Lock ); 00585 00586 #ifdef JCU_PARAMETER_CHECK 00587 IF (self->AsyncStatus.Status != JCU_STATUS_READY) { 00588 returnValue = JCU_ERROR_STATUS; 00589 goto fin; 00590 } /* end if */ 00591 00592 IF (self->AsyncStatus.IsPaused == false) { 00593 returnValue = JCU_ERROR_PARAM; 00594 goto fin; 00595 } /* end if */ 00596 00597 IF ( async == NULL ) { 00598 returnValue = JCU_ERROR_PARAM; 00599 goto fin; 00600 } /* end if */ 00601 #endif /* #Ifdef JCU_PARAMETER_CHECK_ */ 00602 00603 /* Clear the sub status flag */ 00604 switch ( type ) { 00605 case JCU_INPUT_BUFFER: 00606 mask = ( ( JINF_MASK | DINLF_MASK ) << JCU_SHIFT_JINTS1 ); 00607 break; 00608 case JCU_OUTPUT_BUFFER: 00609 mask = ( ( DOUTLF_MASK | JOUTF_MASK ) << JCU_SHIFT_JINTS1 ); 00610 break; 00611 default: 00612 ASSERT_R( type == JCU_IMAGE_INFO, returnValue=E_OTHERS; goto fin ); 00613 mask = INS3_MASK; 00614 break; 00615 } 00616 ASSERT_R( IS_ANY_BITS_SET( self->AsyncStatus.SubStatusFlags, mask ), returnValue=E_OTHERS; goto fin ); 00617 self->AsyncStatus.SubStatusFlags &= ~mask; 00618 00619 00620 R_JCU_SetDefaultAsync( async, R_OSPL_ASYNC_TYPE_NORMAL ); 00621 async->ReturnValue = 0; 00622 00623 /* Clear event flags */ 00624 R_OSPL_EVENT_Clear( async->A_Thread, async->A_EventValue ); 00625 R_OSPL_EVENT_Clear( async->I_Thread, async->I_EventValue ); 00626 00627 /* JCU will restart */ 00628 if( self->AsyncStatus.SubStatusFlags == 0 ) { 00629 /* Attach "Async" to interrupt */ 00630 /* ->MISRA 11.4 : Not too big "enum" is same bit count as "int" */ /* ->SEC R2.7.1 */ 00631 R_OSPL_CALLER_Initialize( &self->InterruptCallbackCaller, 00632 async, (int_fast32_t *)&self->AsyncStatus.Status, JCU_STATUS_INTERRUPTING, 00633 self->I_Lock, self->I_LockVTable ); 00634 /* <-MISRA 11.4 */ /* <-SEC R2.7.1 */ 00635 e= R_JCU_SetInterruptCallbackCaller( &self->InterruptCallbackCaller ); 00636 IF ( e != 0 ) { 00637 returnValue = JCU_ERROR_PARAM; 00638 goto fin; 00639 } 00640 R_OSPL_FLAG32_Set( &self->AsyncStatus.InterruptEnables, JCU_INTERRUPT_LINE_ALL ); 00641 00642 /* Change the status */ 00643 self->AsyncStatus.Status = JCU_STATUS_RUN; 00644 self->AsyncStatus.IsPaused = false; 00645 00646 /* Register set */ 00647 JCU_SetRegisterForContinue( type ); 00648 } 00649 00650 /* JCU will not restart */ 00651 else { 00652 /* Register set */ 00653 JCU_SetRegisterForContinue( type ); 00654 00655 /* Change the status */ 00656 self->AsyncStatus.Status = JCU_STATUS_READY; 00657 00658 /* Set event flags */ 00659 R_OSPL_EVENT_Set( async->A_Thread, async->A_EventValue ); 00660 R_OSPL_EVENT_Set( async->I_Thread, async->I_EventValue ); 00661 } 00662 00663 00664 returnValue = JCU_ERROR_OK; 00665 fin: 00666 if ( IS( was_enabled ) ) { 00667 self->I_LockVTable->Unlock( self->I_Lock ); 00668 } 00669 return returnValue; 00670 } 00671 00672 00673 /** 00674 * @brief R_JCU_GetAsyncStatus. 00675 * 00676 * @par Parameters 00677 * None 00678 * @return None 00679 */ 00680 void R_JCU_GetAsyncStatus( const jcu_async_status_t **const out_Status ) 00681 { 00682 jcu_internal_information_t *const self = &gs_jcu_internal_information; 00683 00684 IF_DQ( out_Status == NULL ) { 00685 goto fin; 00686 } 00687 00688 *out_Status = &self->AsyncStatus; 00689 00690 fin: 00691 return; 00692 } 00693 00694 00695 /** 00696 * @brief Set parameter for decode. 00697 * 00698 * @param decode Select the encode parameter for decoding. <jcu_decode_param_t> 00699 * @param buffer Buffer settings for decode. <jcu_buffer_param_t> 00700 * @return <jcu_errorcode_t> type. 00701 */ 00702 /* ->SEC M1.1.1 API Function */ 00703 jcu_errorcode_t R_JCU_SetDecodeParam( 00704 const jcu_decode_param_t *const decode, 00705 const jcu_buffer_param_t *const buffer) 00706 /* <-SEC M1.1.1 */ 00707 { 00708 jcu_errorcode_t returnValue; 00709 bool_t was_enabled; /* = false; */ /* QAC 3197 */ 00710 jcu_internal_information_t *const self = &gs_jcu_internal_information; 00711 00712 was_enabled = self->I_LockVTable->Lock( self->I_Lock ); 00713 00714 #ifdef JCU_PARAMETER_CHECK 00715 IF (!((self->AsyncStatus.Status == JCU_STATUS_READY) 00716 || (self->AsyncStatus.Status == JCU_STATUS_SELECTED))) { 00717 returnValue = JCU_ERROR_STATUS; 00718 goto fin; 00719 } /* end if */ 00720 00721 IF(self->Codec != JCU_STATUS_DECODE) { 00722 returnValue = JCU_ERROR_STATUS; 00723 goto fin; 00724 } /* end if */ 00725 00726 IF((decode == NULL) || (buffer == NULL)) { 00727 returnValue = JCU_ERROR_PARAM; 00728 goto fin; 00729 } /* end if */ 00730 00731 returnValue = JCU_ParaCheckSetDecodeParam(decode, buffer); 00732 IF(returnValue != JCU_ERROR_OK) { 00733 goto fin; 00734 } /* end if */ 00735 00736 #endif /* #Ifdef JCU_PARAMETER_CHECK_ */ 00737 00738 /* Register set */ 00739 JCU_SetRegisterForSetDecodePrm(decode, buffer); 00740 00741 /* Set the updated status */ 00742 self->AsyncStatus.Status = JCU_STATUS_READY; 00743 00744 returnValue = JCU_ERROR_OK; 00745 fin: 00746 if ( IS( was_enabled ) ) { 00747 self->I_LockVTable->Unlock( self->I_Lock ); 00748 } 00749 return returnValue; 00750 } 00751 00752 00753 /** 00754 * @brief Whether stop or not stop for R_JCU_GetImageInfo() 00755 * 00756 * @param is_pause Whether stop or not stop 00757 * @return <jcu_errorcode_t> type. 00758 */ 00759 jcu_errorcode_t R_JCU_SetPauseForImageInfo( const bool_t is_pause ) 00760 { 00761 jcu_errorcode_t returnValue; 00762 bool_t was_enabled; /* = false; */ /* QAC 3197 */ 00763 jcu_internal_information_t *const self = &gs_jcu_internal_information; 00764 00765 was_enabled = self->I_LockVTable->Lock( self->I_Lock ); 00766 00767 #ifdef JCU_PARAMETER_CHECK 00768 IF (!((self->AsyncStatus.Status == JCU_STATUS_READY) 00769 || (self->AsyncStatus.Status == JCU_STATUS_SELECTED))) { 00770 returnValue = JCU_ERROR_STATUS; 00771 goto fin; 00772 } /* end if */ 00773 00774 IF(self->Codec != JCU_STATUS_DECODE) { 00775 returnValue = JCU_ERROR_STATUS; 00776 goto fin; 00777 } /* end if */ 00778 #endif /* #Ifdef JCU_PARAMETER_CHECK_ */ 00779 00780 /* Register set */ 00781 JCU_SetRegisterForSetPause( is_pause, self->ErrorFilter ); 00782 00783 returnValue = JCU_ERROR_OK; 00784 fin: 00785 if ( IS( was_enabled ) ) { 00786 self->I_LockVTable->Unlock( self->I_Lock ); 00787 } 00788 return returnValue; 00789 } 00790 00791 00792 /** 00793 * @brief GetImageInfo 00794 * 00795 * @param buffer Image information. <jcu_image_info_t> 00796 * @return <jcu_errorcode_t> type. 00797 */ 00798 /* ->SEC M1.1.1 API Function */ 00799 jcu_errorcode_t R_JCU_GetImageInfo( 00800 jcu_image_info_t *const buffer) 00801 /* <-SEC M1.1.1 */ 00802 { 00803 jcu_errorcode_t returnValue; 00804 bool_t was_enabled; /* = false; */ /* QAC 3197 */ 00805 jcu_internal_information_t *const self = &gs_jcu_internal_information; 00806 00807 was_enabled = self->I_LockVTable->Lock( self->I_Lock ); 00808 00809 #ifdef JCU_PARAMETER_CHECK 00810 IF (self->AsyncStatus.Status != JCU_STATUS_READY) { 00811 returnValue = JCU_ERROR_STATUS; 00812 goto fin; 00813 } /* end if */ 00814 00815 IF (self->Codec != JCU_STATUS_DECODE) { 00816 returnValue = JCU_ERROR_STATUS; 00817 goto fin; 00818 } /* end if */ 00819 00820 IF (buffer == NULL) { 00821 returnValue = JCU_ERROR_PARAM; 00822 goto fin; 00823 } /* end if */ 00824 00825 returnValue = JCU_ParaCheckGetImageInfo(buffer); 00826 if(returnValue != JCU_ERROR_OK) { 00827 goto fin; 00828 } /* end if */ 00829 #endif /* #Ifdef JCU_PARAMETER_CHECK_ */ 00830 00831 /* Register set */ 00832 JCU_GetRegisterForGetImageInfo(buffer); 00833 00834 returnValue = JCU_ERROR_OK; 00835 fin: 00836 if ( IS( was_enabled ) ) { 00837 self->I_LockVTable->Unlock( self->I_Lock ); 00838 } 00839 return returnValue; 00840 } 00841 00842 00843 /** 00844 * @brief SetErrorFilter 00845 * 00846 * @param filter enable bit of error. <jcu_int_detail_errors_t> 00847 * @return <jcu_errorcode_t> type. 00848 */ 00849 jcu_errorcode_t R_JCU_SetErrorFilter(jcu_int_detail_errors_t filter) 00850 { 00851 jcu_errorcode_t returnValue; 00852 jcu_internal_information_t *const self = &gs_jcu_internal_information; 00853 00854 #ifdef JCU_PARAMETER_CHECK 00855 IF (!((self->AsyncStatus.Status == JCU_STATUS_INIT) 00856 || (self->AsyncStatus.Status == JCU_STATUS_READY) 00857 || (self->AsyncStatus.Status == JCU_STATUS_SELECTED))) { 00858 returnValue = JCU_ERROR_STATUS; 00859 goto fin; 00860 } /* end if */ 00861 00862 #endif /* #Ifdef JCU_PARAMETER_CHECK_ */ 00863 00864 IF ( IS_ANY_BITS_SET( filter, ~JCU_INT_ERROR_ALL ) ) { 00865 returnValue = JCU_ERROR_PARAM; 00866 goto fin; 00867 } /* end if */ 00868 00869 self->ErrorFilter = filter; 00870 00871 returnValue = JCU_ERROR_OK; 00872 fin: 00873 return returnValue; 00874 } 00875 00876 00877 /** 00878 * @brief SetQuantizationTable 00879 * 00880 * @param tableNo The table number to set the value. <jcu_table_no_t> 00881 * @param table The body of the table to set. 00882 * @return <jcu_errorcode_t> type. 00883 */ 00884 /* ->SEC M1.1.1 API Function */ 00885 jcu_errorcode_t R_JCU_SetQuantizationTable( 00886 const jcu_table_no_t tableNo, 00887 const uint8_t *const table) 00888 /* <-SEC M1.1.1 */ 00889 { 00890 jcu_errorcode_t returnValue; 00891 bool_t was_enabled; /* = false; */ /* QAC 3197 */ 00892 jcu_internal_information_t *const self = &gs_jcu_internal_information; 00893 00894 was_enabled = self->I_LockVTable->Lock( self->I_Lock ); 00895 00896 #ifdef JCU_PARAMETER_CHECK 00897 IF (!((self->AsyncStatus.Status == JCU_STATUS_READY) 00898 || (self->AsyncStatus.Status == JCU_STATUS_SELECTED))) { 00899 returnValue = JCU_ERROR_STATUS; 00900 goto fin; 00901 } /* end if */ 00902 00903 IF (self->Codec != JCU_STATUS_ENCODE) { 00904 returnValue = JCU_ERROR_STATUS; 00905 goto fin; 00906 } /* end if */ 00907 00908 IF (table == NULL) { 00909 returnValue = JCU_ERROR_PARAM; 00910 goto fin; 00911 } /* end if */ 00912 00913 returnValue = JCU_ParaCheckSetQuantizationTbl(tableNo); 00914 IF (returnValue != JCU_ERROR_OK) { 00915 goto fin; 00916 } /* end if */ 00917 #endif /* #Ifdef JCU_PARAMETER_CHECK_ */ 00918 00919 /* Register set */ 00920 JCU_SetRegisterForSetQtTable(tableNo, table); 00921 00922 returnValue = JCU_ERROR_OK; 00923 fin: 00924 if ( IS( was_enabled ) ) { 00925 self->I_LockVTable->Unlock( self->I_Lock ); 00926 } 00927 return returnValue; 00928 } 00929 00930 00931 /** 00932 * @brief Set the Huffman table 00933 * 00934 * @param tableNo The table number to set the value. <jcu_table_no_t> 00935 * @param type The type of Huffman table type (AC or DC). <jcu_huff_t> 00936 * @param table The body of the table to set 00937 * @return <jcu_errorcode_t> type. 00938 */ 00939 /* ->SEC M1.1.1 API Function */ 00940 jcu_errorcode_t R_JCU_SetHuffmanTable( 00941 const jcu_table_no_t tableNo, 00942 const jcu_huff_t type, 00943 const uint8_t *const table) 00944 /* <-SEC M1.1.1 */ 00945 { 00946 jcu_errorcode_t returnValue; 00947 bool_t was_enabled; /* = false; */ /* QAC 3197 */ 00948 jcu_internal_information_t *const self = &gs_jcu_internal_information; 00949 00950 was_enabled = self->I_LockVTable->Lock( self->I_Lock ); 00951 00952 #ifdef JCU_PARAMETER_CHECK 00953 IF (!((self->AsyncStatus.Status == JCU_STATUS_READY) 00954 || (self->AsyncStatus.Status == JCU_STATUS_SELECTED))) { 00955 returnValue = JCU_ERROR_STATUS; 00956 goto fin; 00957 } /* end if */ 00958 00959 IF (self->Codec != JCU_STATUS_ENCODE) { 00960 returnValue = JCU_ERROR_STATUS; 00961 goto fin; 00962 } /* end if */ 00963 00964 IF (table == NULL) { 00965 returnValue = JCU_ERROR_PARAM; 00966 goto fin; 00967 } /* end if */ 00968 00969 returnValue = JCU_ParaCheckSetHuffmanTable(tableNo, type); 00970 IF (returnValue != JCU_ERROR_OK) { 00971 goto fin; 00972 } /* end if */ 00973 #endif /* #Ifdef JCU_PARAMETER_CHECK_ */ 00974 00975 /* Register set */ 00976 JCU_SetRegisterForSetHuffTbl(tableNo, type, table); 00977 00978 returnValue = JCU_ERROR_OK; 00979 fin: 00980 if ( IS( was_enabled ) ) { 00981 self->I_LockVTable->Unlock( self->I_Lock ); 00982 } 00983 return returnValue; 00984 } 00985 00986 00987 /** 00988 * @brief Set the parameter fo encoding 00989 * 00990 * @param encode Select the encode parameter for encoding. <jcu_encode_param_t> 00991 * @param buffer Select the buffer settings for encoding. <jcu_buffer_param_t> 00992 * @return <jcu_errorcode_t> type. 00993 */ 00994 /* ->SEC M1.1.1 API Function */ 00995 jcu_errorcode_t R_JCU_SetEncodeParam( 00996 const jcu_encode_param_t *const encode, 00997 const jcu_buffer_param_t *const buffer) 00998 /* <-SEC M1.1.1 */ 00999 { 01000 jcu_errorcode_t returnValue; 01001 bool_t was_enabled; /* = false; */ /* QAC 3197 */ 01002 jcu_internal_information_t *const self = &gs_jcu_internal_information; 01003 01004 was_enabled = self->I_LockVTable->Lock( self->I_Lock ); 01005 01006 #ifdef JCU_PARAMETER_CHECK 01007 IF (!((self->AsyncStatus.Status == JCU_STATUS_READY) 01008 || (self->AsyncStatus.Status == JCU_STATUS_SELECTED))) { 01009 returnValue = JCU_ERROR_STATUS; 01010 goto fin; 01011 } /* end if */ 01012 01013 IF (self->Codec != JCU_STATUS_ENCODE) { 01014 returnValue = JCU_ERROR_STATUS; 01015 goto fin; 01016 } /* end if */ 01017 01018 IF ((encode == NULL) || (buffer == NULL)) { 01019 returnValue = JCU_ERROR_PARAM; 01020 goto fin; 01021 } /* end if */ 01022 01023 returnValue = JCU_ParaCheckEncodeParam(encode, buffer); 01024 IF (returnValue != JCU_ERROR_OK) { 01025 goto fin; 01026 } /* end if */ 01027 #endif /* #Ifdef JCU_PARAMETER_CHECK_ */ 01028 01029 /* Register set */ 01030 JCU_SetRegisterForSetEncodePrm(encode, buffer); 01031 01032 /* Set the updated status */ 01033 self->AsyncStatus.Status = JCU_STATUS_READY; 01034 01035 returnValue = JCU_ERROR_OK; 01036 fin: 01037 if ( IS( was_enabled ) ) { 01038 self->I_LockVTable->Unlock( self->I_Lock ); 01039 } 01040 return returnValue; 01041 } 01042 01043 01044 /** 01045 * @brief GetEncodedSize 01046 * 01047 * @param out_Size EncodedSize 01048 * @return <jcu_errorcode_t> type. 01049 */ 01050 /* ->SEC M1.1.1 API Function */ 01051 jcu_errorcode_t R_JCU_GetEncodedSize( 01052 size_t *const out_Size) 01053 /* <-SEC M1.1.1 */ 01054 { 01055 JCU_GetEncodedSize(out_Size); 01056 return JCU_ERROR_OK; 01057 } 01058 01059 01060 /** 01061 * @brief Set AXI bus A*CACHE bits for 2nd cache to JCU. 01062 * 01063 * @param read_cache_attribute <r_ospl_axi_cache_attribute_t> 01064 * @param write_cache_attribute <r_ospl_axi_cache_attribute_t> 01065 * @return <jcu_errorcode_t> type. 01066 */ 01067 jcu_errorcode_t R_JCU_Set2ndCacheAttribute( 01068 r_ospl_axi_cache_attribute_t const read_cache_attribute, 01069 r_ospl_axi_cache_attribute_t const write_cache_attribute ) 01070 { 01071 static const uint32_t mask_JCU = 0xFFFF0000u; 01072 jcu_errorcode_t returnValue; 01073 bool_t was_all_enabled = false; 01074 uint32_t new_value; 01075 volatile uint32_t value; 01076 jcu_internal_information_t *const self = &gs_jcu_internal_information; 01077 01078 #ifdef JCU_PARAMETER_CHECK 01079 IF (!((self->AsyncStatus.Status == JCU_STATUS_READY) 01080 || (self->AsyncStatus.Status == JCU_STATUS_SELECTED))) { 01081 returnValue = JCU_ERROR_STATUS; 01082 goto fin; 01083 } /* end if */ 01084 #endif /* #Ifdef JCU_PARAMETER_CHECK_ */ 01085 01086 01087 new_value = ( read_cache_attribute << 24 ) | ( write_cache_attribute << 16 ); 01088 01089 was_all_enabled = R_OSPL_DisableAllInterrupt(); 01090 01091 value = INB.AXIBUSCTL0; 01092 value = new_value | ( value & ~mask_JCU ); /* Mutual Exclusion from Ether */ 01093 INB.AXIBUSCTL0 = value; 01094 01095 returnValue = JCU_ERROR_OK; 01096 fin: 01097 if ( was_all_enabled ) { 01098 R_OSPL_EnableAllInterrupt(); 01099 } 01100 01101 return returnValue; 01102 } 01103 01104 01105 /** 01106 * @brief EnableInterrupt 01107 * 01108 * @par Parameters 01109 * None 01110 * @return None 01111 */ 01112 void R_JCU_EnableInterrupt(void) 01113 { 01114 jcu_internal_information_t *const self = &gs_jcu_internal_information; 01115 01116 self->AsyncStatus.IsEnabledInterrupt = true; 01117 R_JCU_OnEnableInterrupt( self->AsyncStatus.InterruptEnables.Flags ); 01118 } 01119 01120 01121 /** 01122 * @brief DisableInterrupt 01123 * 01124 * @par Parameters 01125 * None 01126 * @return Was interrupt enabled 01127 */ 01128 bool_t R_JCU_DisableInterrupt(void) 01129 { 01130 bool_t was_interrupted; 01131 jcu_internal_information_t *const self = &gs_jcu_internal_information; 01132 01133 was_interrupted = self->AsyncStatus.IsEnabledInterrupt; 01134 01135 R_JCU_OnDisableInterrupt( self->AsyncStatus.InterruptEnables.Flags ); 01136 self->AsyncStatus.IsEnabledInterrupt = false; 01137 01138 return was_interrupted; 01139 } 01140 01141 01142 /** 01143 * @brief R_JCU_GetInternalInformation 01144 * 01145 * @par Parameters 01146 * None 01147 * @return <jcu_internal_information_t> type. 01148 */ 01149 jcu_internal_information_t *R_JCU_GetInternalInformation(void) 01150 { 01151 return &gs_jcu_internal_information; 01152 } 01153 01154 01155 /** 01156 * @brief R_JCU_GetILockObject 01157 * 01158 * @par Parameters 01159 * None 01160 * @return <jcu_i_lock_t> type. 01161 */ 01162 jcu_i_lock_t *R_JCU_GetILockObject(void) 01163 { 01164 return &gs_jcu_i_lock; 01165 } 01166 01167 01168 /** 01169 * @brief Replace associated I-Lock object 01170 * 01171 * @param I_Lock I-Lock object 01172 * @param I_LockVTable V-Table 01173 * @return Whether success to replace 01174 */ 01175 bool_t R_JCU_I_LOCK_Replace( void *const I_Lock, const r_ospl_i_lock_vtable_t *const I_LockVTable ) 01176 { 01177 jcu_internal_information_t *const self = &gs_jcu_internal_information; 01178 bool_t ret = false; 01179 01180 ASSERT_R( self->AsyncStatus.Status == JCU_STATUS_UNDEF, ret = false; goto fin ); 01181 01182 if ( I_Lock != NULL ) { 01183 if ( self->I_Lock == NULL ) { 01184 self->I_Lock = I_Lock; 01185 self->I_LockVTable = I_LockVTable; 01186 ret = true; 01187 } 01188 } else { 01189 self->I_Lock = NULL; 01190 } 01191 01192 fin: 01193 return ret; 01194 } 01195 01196 01197 /** 01198 * @brief Finalize the I-Lock object 01199 * 01200 * @par Parameters 01201 * None 01202 * @return None 01203 */ 01204 void R_JCU_I_LOCK_Finalize(void) 01205 { 01206 jcu_internal_information_t *const self = &gs_jcu_internal_information; 01207 bool_t b; 01208 01209 ASSERT_D( self->Is_I_LockMaster, R_NOOP() ); 01210 01211 R_JCU_I_LOCK_Reset( self->I_Lock ); 01212 b= R_JCU_I_LOCK_Replace( NULL, NULL ); 01213 R_UNREFERENCED_VARIABLE( b ); /* QAC 3200 : This is not error information */ 01214 self->Is_I_LockMaster = false; 01215 } 01216 01217 01218 /** 01219 * @brief Reset the I-Lock object 01220 * 01221 * @param self I-Lock object 01222 * @return None 01223 */ 01224 static void R_JCU_I_LOCK_Reset( jcu_i_lock_t *const self ) 01225 { 01226 IF_DQ( self == NULL ) { 01227 goto fin; 01228 } 01229 01230 self->IsLock = false; 01231 R_JCU_OnDisableInterrupt( JCU_INTERRUPT_LINE_ALL ); 01232 JCU_ClearInterruptFlag(); 01233 self->IsRequestedFinalize = false; 01234 01235 fin: 01236 return; 01237 } 01238 01239 01240 /** 01241 * @brief Lock the I-Lock object 01242 * 01243 * @param self_ I-Lock object 01244 * @return Was interrupt enabled 01245 */ 01246 static bool_t R_JCU_I_LOCK_Lock( void *const self_ ) 01247 { 01248 bool_t is_locked; 01249 bool_t was_all_enabled; /* = false; */ /* QAC 3197 */ 01250 bool_t b; 01251 jcu_i_lock_t *const self = (jcu_i_lock_t *) self_; 01252 01253 IF_DQ( self == NULL ) { 01254 is_locked = true; 01255 goto fin; 01256 } 01257 01258 was_all_enabled = R_OSPL_DisableAllInterrupt(); 01259 01260 is_locked = self->IsLock; 01261 if ( ! is_locked ) { 01262 b= R_JCU_DisableInterrupt(); 01263 R_UNREFERENCED_VARIABLE( b ); /* QAC 3200 : This is not error information */ 01264 self->IsLock = true; 01265 } 01266 01267 if ( IS( was_all_enabled ) ) { 01268 R_OSPL_EnableAllInterrupt(); 01269 } 01270 01271 fin: 01272 return ! is_locked; 01273 } 01274 01275 01276 /** 01277 * @brief Unlock the I-Lock object 01278 * 01279 * @param self_ I-Lock object 01280 * @return None 01281 */ 01282 static void R_JCU_I_LOCK_Unlock( void *const self_ ) 01283 { 01284 bool_t was_all_enabled; /* = false; */ /* QAC 3197 */ 01285 jcu_i_lock_t *const self = (jcu_i_lock_t *) self_; 01286 01287 IF_DQ( self == NULL ) { 01288 goto fin; 01289 } 01290 01291 was_all_enabled = R_OSPL_DisableAllInterrupt(); 01292 01293 R_JCU_EnableInterrupt(); 01294 self->IsLock = false; 01295 01296 if ( IS( was_all_enabled ) ) { 01297 R_OSPL_EnableAllInterrupt(); 01298 } 01299 01300 fin: 01301 return; 01302 } 01303 01304 01305 /** 01306 * @brief Request to finalize the I-Lock object 01307 * 01308 * @param self_ I-Lock object 01309 * @return None 01310 */ 01311 static void R_JCU_I_LOCK_RequestFinalize( void *const self_ ) 01312 { 01313 jcu_i_lock_t *const self = (jcu_i_lock_t *) self_; 01314 01315 IF_DQ( self == NULL ) { 01316 goto fin; 01317 } 01318 01319 self->IsRequestedFinalize = true; 01320 01321 fin: 01322 return; 01323 } 01324 01325 01326 /** 01327 * @brief Get root channel number of I-Lock object 01328 * 01329 * @param self_ I-Lock object 01330 * @return A channel number 01331 */ 01332 static int_fast32_t R_JCU_I_LOCK_GetRootChannelNum( const void *const self_ ) 01333 { 01334 R_UNREFERENCED_VARIABLE( self_ ); 01335 01336 return 0; 01337 } 01338 01339
Generated on Tue Jul 12 2022 11:15:01 by
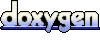