Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
converter_wrapper.c
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /**************************************************************************//** 00024 * @file converter_wrapper.c 00025 * @version 1.00 00026 * $Rev: 1 $ 00027 * $Date:: 2015-08-06 16:33:52 +0900#$ 00028 * @brief Graphics driver wrapper function definitions in C 00029 ******************************************************************************/ 00030 00031 /****************************************************************************** 00032 Includes <System Includes> , "Project Includes" 00033 ******************************************************************************/ 00034 #include "converter_wrapper.h" 00035 #include "r_ospl.h" 00036 #include "r_jcu_api.h" 00037 #include "r_jcu_pl.h" 00038 00039 static mbed_CallbackFunc_t* SetCallback; 00040 static size_t* pEncodeSize; 00041 static int32_t EncodeCount; 00042 static int32_t EncodeCountMax; 00043 static size_t DecodeWidth; 00044 static size_t DecodeHeight; 00045 static r_ospl_async_t Async; 00046 00047 /****************************************************************************** 00048 Imported global variables and functions (from other files) 00049 ******************************************************************************/ 00050 /**************************************************************************//** 00051 * @brief Set callback function address for decode 00052 * @param[in] pSetCallbackAdr Callback function address 00053 * @param[in] width Decode data width 00054 * @param[in] height Decode data height 00055 * @retval error code 00056 ******************************************************************************/ 00057 errnum_t R_wrpper_set_decode_callback(mbed_CallbackFunc_t* pSetCallbackAdr, size_t width, size_t height) 00058 { 00059 errnum_t e; 00060 00061 Async.Flags = R_F_OSPL_InterruptCallback; 00062 Async.A_Thread = R_OSPL_THREAD_GetCurrentId(); 00063 Async.InterruptCallback = &R_wrpper_LocalDecodeCallback; 00064 SetCallback = pSetCallbackAdr; 00065 DecodeWidth = width; 00066 DecodeHeight = height; 00067 00068 e = R_JCU_StartAsync(&Async); 00069 00070 return e; 00071 } 00072 00073 /**************************************************************************//** 00074 * @brief Set callback function address for encode 00075 * @param[in] InterruptSource Interrput source data struct address 00076 * @param[in] Caller ospl caller data address 00077 * @retval error code 00078 ******************************************************************************/ 00079 errnum_t R_wrpper_LocalDecodeCallback(const r_ospl_interrupt_t *InterruptSource, const r_ospl_caller_t *Caller) 00080 { 00081 errnum_t e = 0; 00082 const jcu_async_status_t* status; 00083 jcu_image_info_t image_info; 00084 jcu_errorcode_t jcu_error; 00085 00086 e = R_JCU_OnInterruptDefault(InterruptSource, Caller); 00087 if (e != 0) { 00088 SetCallback(MBED_JCU_E_JCU_ERR ); 00089 goto fin; 00090 } 00091 R_JCU_GetAsyncStatus( &status ); 00092 if (status -> IsPaused == true) { 00093 if ((status->SubStatusFlags & JCU_SUB_INFOMATION_READY) == 0) { 00094 e = E_OTHERS; 00095 SetCallback(MBED_JCU_E_FORMA_ERR ); 00096 goto fin; 00097 } 00098 R_JCU_GetImageInfo( &image_info ); 00099 if ((image_info.width == 0u) || (image_info.height == 0u) || 00100 (image_info.width > DecodeWidth) || 00101 (image_info.height > DecodeHeight)) { 00102 e = E_OTHERS; 00103 SetCallback(MBED_JCU_E_FORMA_ERR ); 00104 goto fin; 00105 } 00106 if ((image_info.encodedFormat != JCU_JPEG_YCbCr444) && 00107 (image_info.encodedFormat != JCU_JPEG_YCbCr422) && 00108 (image_info.encodedFormat != JCU_JPEG_YCbCr420) && 00109 (image_info.encodedFormat != JCU_JPEG_YCbCr411)) { 00110 e = E_OTHERS; 00111 SetCallback(MBED_JCU_E_FORMA_ERR ); 00112 goto fin; 00113 } 00114 jcu_error = R_JCU_ContinueAsync(JCU_IMAGE_INFO, &Async); 00115 if (jcu_error != JCU_ERROR_OK) { 00116 e = E_OTHERS; 00117 SetCallback(MBED_JCU_E_JCU_ERR ); 00118 goto fin; 00119 } 00120 } else { 00121 SetCallback(MBED_JCU_E_OK ); 00122 } 00123 00124 fin: 00125 return e; 00126 00127 } 00128 00129 /**************************************************************************//** 00130 * @brief Set callback function address for encode 00131 * @param[in] pSetCallbackAdr Callback function address 00132 * @param[in] pSize Encode size input address 00133 * @param[in] count_max Encode count max num 00134 * @retval error code 00135 ******************************************************************************/ 00136 errnum_t R_wrpper_set_encode_callback( mbed_CallbackFunc_t* pSetCallbackAdr, size_t* pSize, int32_t count_max) 00137 { 00138 errnum_t e; 00139 00140 Async.Flags = R_F_OSPL_InterruptCallback; 00141 Async.A_Thread = R_OSPL_THREAD_GetCurrentId(); 00142 Async.InterruptCallback = &R_wrpper_LocalEncodeCallback; 00143 SetCallback = pSetCallbackAdr; 00144 pEncodeSize = pSize; 00145 *pEncodeSize = 0; 00146 EncodeCount = 1; 00147 EncodeCountMax = count_max; 00148 00149 e = R_JCU_StartAsync(&Async); 00150 00151 return e; 00152 } 00153 00154 /**************************************************************************//** 00155 * @brief Set callback function address for encode 00156 * @param[in] InterruptSource Interrput source data struct address 00157 * @param[in] Caller ospl caller data address 00158 * @retval error code 00159 ******************************************************************************/ 00160 errnum_t R_wrpper_LocalEncodeCallback(const r_ospl_interrupt_t *InterruptSource, const r_ospl_caller_t *Caller) 00161 { 00162 errnum_t e = 0; 00163 const jcu_async_status_t* status; 00164 jcu_errorcode_t jcu_error; 00165 00166 e = R_JCU_OnInterruptDefault(InterruptSource, Caller); 00167 if (e != 0) { 00168 SetCallback(MBED_JCU_E_JCU_ERR ); 00169 goto fin; 00170 } 00171 R_JCU_GetAsyncStatus(&status); 00172 if (status -> IsPaused == true) { 00173 if ((status->SubStatusFlags & JCU_SUB_ENCODE_OUTPUT_PAUSE) == 0) { 00174 e = E_OTHERS; 00175 goto fin; 00176 } 00177 if (EncodeCount >= EncodeCountMax) { 00178 e = E_OTHERS; 00179 SetCallback(MBED_JCU_E_JCU_ERR ); 00180 goto fin; 00181 } 00182 EncodeCount++; 00183 jcu_error = R_JCU_ContinueAsync(JCU_OUTPUT_BUFFER, &Async); 00184 if (jcu_error != JCU_ERROR_OK) { 00185 e = E_OTHERS; 00186 SetCallback(MBED_JCU_E_JCU_ERR ); 00187 goto fin; 00188 } 00189 } else { 00190 (void)R_JCU_GetEncodedSize(pEncodeSize); 00191 SetCallback(MBED_JCU_E_OK ); 00192 } 00193 00194 fin: 00195 return e; 00196 00197 }
Generated on Tue Jul 12 2022 11:15:00 by
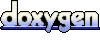