Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
clib_drivers.c
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2014 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /** 00024 * @file clib_drivers.c 00025 * @brief $Module: CLibCommon $ $PublicVersion: 0.90 $ (=CLIB_VERSION) 00026 * $Rev: 30 $ 00027 * $Date:: 2014-02-13 21:21:47 +0900#$ 00028 * - Description: Common code. 00029 */ 00030 00031 00032 /****************************************************************************** 00033 Includes <System Includes> , "Project Includes" 00034 ******************************************************************************/ 00035 #include "r_ospl.h" 00036 #include "clib_drivers.h " 00037 00038 00039 /****************************************************************************** 00040 Typedef definitions 00041 ******************************************************************************/ 00042 00043 /****************************************************************************** 00044 Macro definitions 00045 ******************************************************************************/ 00046 00047 /****************************************************************************** 00048 Imported global variables and functions (from other files) 00049 ******************************************************************************/ 00050 00051 /****************************************************************************** 00052 Exported global variables and functions (to be accessed by other files) 00053 ******************************************************************************/ 00054 00055 /****************************************************************************** 00056 Private global variables and functions 00057 ******************************************************************************/ 00058 00059 /** 00060 * @brief Cast with range check 00061 * 00062 * @param input Input value 00063 * @param output Output value 00064 * @return Error Code. 0=No Error. 00065 */ 00066 errnum_t R_int32_t_to_int8_t( int32_t const input, int8_t *const output ) 00067 { 00068 errnum_t e; 00069 00070 IF ( (input < INT8_MIN) || (input > INT8_MAX) ) { 00071 e=E_OTHERS; 00072 goto fin; 00073 } 00074 IF_DQ( output == NULL ) { 00075 e=E_OTHERS; 00076 goto fin; 00077 } 00078 00079 *output = (int8_t) input; 00080 00081 e=0; 00082 fin: 00083 return e; 00084 } 00085 00086 00087 /** 00088 * @brief Cast with range check 00089 * 00090 * @param input Input value 00091 * @param output Output value 00092 * @return Error Code. 0=No Error. 00093 */ 00094 errnum_t R_int32_t_to_int16_t( int32_t input, int16_t *output ) 00095 { 00096 IF ( input < -32768 || input > 32767 ) { 00097 return E_OTHERS; 00098 } 00099 00100 *output = (int16_t) input; 00101 return 0; 00102 } 00103 00104 00105 /** 00106 * @brief Cast with range check 00107 * 00108 * @param input Input value 00109 * @param output Output value 00110 * @return Error Code. 0=No Error. 00111 */ 00112 errnum_t R_int32_t_to_uint8_t( int32_t input, uint8_t *output ) 00113 { 00114 IF ( input < 0 || input > 255 ) { 00115 return E_OTHERS; 00116 } 00117 00118 *output = (uint8_t) input; 00119 return 0; 00120 } 00121 00122 00123 /** 00124 * @brief Returns whether specified value has 1 bit only 1. 00125 * 00126 * @param Value Input value 00127 * @return Returns whether specified value has 1 bit only 1. 00128 */ 00129 bool_t Is1bitOnlyInt( uint32_t Value ) 00130 { 00131 if ( (Value & 0x0000FFFF) == 0 ) { 00132 Value >>= 16; 00133 } 00134 if ( (Value & 0x000000FF) == 0 ) { 00135 Value >>= 8; 00136 } 00137 if ( (Value & 0x0000000F) == 0 ) { 00138 Value >>= 4; 00139 } 00140 if ( (Value & 0x00000003) == 0 ) { 00141 Value >>= 2; 00142 } 00143 if ( (Value & 0x00000001) == 0 ) { 00144 Value >>= 1; 00145 } 00146 return ( Value == 1 ); 00147 } 00148 00149 00150 /** 00151 * @brief Get whether 2 "IntBoxType"s are same area. 00152 * 00153 * @param in_BoxA A box 00154 * @param in_BoxB Other box 00155 * @param out_IsSame Whether 2 "IntBoxType"s are same area. 00156 * @return Error Code. 0=No Error. 00157 */ 00158 errnum_t IntBoxType_isSame( const IntBoxType *in_BoxA, const IntBoxType *in_BoxB, bool_t *out_IsSame ) 00159 { 00160 *out_IsSame = ( 00161 in_BoxA->MinX == in_BoxB->MinX && 00162 in_BoxA->MinY == in_BoxB->MinY && 00163 in_BoxA->Width == in_BoxB->Width && 00164 in_BoxA->Height == in_BoxB->Height ); 00165 00166 return 0; 00167 } 00168 00169
Generated on Tue Jul 12 2022 11:15:00 by
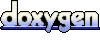