Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
RGA_Port.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2013 - 2014 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /****************************************************************************** 00024 * $FileName: RGA_Port.h $ 00025 * $Module: RGA $ $PublicVersion: 1.03 $ (=RGA_VERSION) 00026 * $Rev: $ 00027 * $Date:: $ 00028 * Description: 00029 ******************************************************************************/ 00030 00031 #ifndef RGA_PORT_H 00032 #define RGA_PORT_H 00033 00034 /****************************************************************************** 00035 Includes <System Includes> , "Project Includes" 00036 ******************************************************************************/ 00037 #include "RGA_API_typedef.h " 00038 #include "RGA_Port_typedef.h " 00039 #include "clib_drivers_typedef.h " /* "R_CEIL_8U" in "R_RGA_CalcWorkBufferSize" */ 00040 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif /* __cplusplus */ 00045 00046 00047 /****************************************************************************** 00048 Typedef definitions 00049 ******************************************************************************/ 00050 /* in RGA_Port_typedef.h */ 00051 00052 /****************************************************************************** 00053 Macro definitions 00054 ******************************************************************************/ 00055 /* in RGA_Port_typedef.h */ 00056 00057 /****************************************************************************** 00058 Variable Externs 00059 ******************************************************************************/ 00060 /* in RGA_Port_typedef.h */ 00061 00062 /****************************************************************************** 00063 Functions Prototypes 00064 ******************************************************************************/ 00065 errnum_t drawCLUTImage( uint8_t *SrcAddress, const graphics_image_t *image, frame_buffer_t *frame ); 00066 00067 00068 /*********************************************************************** 00069 * Class: vram_ex_stack_t 00070 ************************************************************************/ 00071 errnum_t R_VRAM_EX_STACK_Initialize( vram_ex_stack_t *self, void *NullConfig ); 00072 errnum_t R_VRAM_EX_STACK_Alloc( vram_ex_stack_t *self, frame_buffer_t *in_out_FrameBuffer ); 00073 errnum_t R_VRAM_EX_STACK_Free( vram_ex_stack_t *self, frame_buffer_t *frame_buffer ); 00074 00075 00076 /* Section: Global */ 00077 /** 00078 * @brief Calculate size of hardware work buffer 00079 * 00080 * @param max_height_of_frame_buffer int 00081 * @return size of hardware work buffer 00082 */ 00083 #ifdef RGAH_VERSION 00084 00085 #define R_RGA_CalcWorkBufferSize( max_height_of_frame_buffer ) \ 00086 ( (size_t)( RGA_WORK_BUFFER_MAX_DISPLAY_LIST + \ 00087 RGA_WORK_BUFFER_STRIDE * R_CEIL_8U( max_height_of_frame_buffer ) * 4 * 2 ) ) 00088 R_STATIC_ASSERT_GLOBAL( RGA_WORK_BUFFER_HEIGHT_ALIGNMENT == 8, "" ); /* check of R_CEIL_8U */ 00089 00090 #endif 00091 00092 00093 /** 00094 * @brief Calculate size of hardware work buffer 00095 * 00096 * @param max_width_of_frame_buffer int 00097 * @param max_height_of_frame_buffer int 00098 * @return size of hardware work buffer 00099 */ 00100 #ifdef RGAH_VERSION 00101 00102 #define R_RGA_CalcWorkBufferSize2( max_width_of_frame_buffer, max_height_of_frame_buffer ) \ 00103 R_RGA_CalcWorkBufferSize( max_height_of_frame_buffer ) 00104 #else 00105 00106 #define R_RGA_CalcWorkBufferSize2( max_width_of_frame_buffer, max_height_of_frame_buffer ) \ 00107 ( (size_t)( (max_width_of_frame_buffer) * RGA_WORK_BUFFER_DMAC_LM_WIDTH_BYTE + \ 00108 (max_height_of_frame_buffer) * RGA_WORK_BUFFER_DMAC_LM_HEIGHT_BYTE ) ) 00109 #endif 00110 00111 00112 /** 00113 * @brief Calculate size of hardware work buffer B 00114 * 00115 * @param MaxWidthOfJPEG int 00116 * @param MaxHeightOfJPEG int 00117 * @param MaxBytePerPixelOfFrameBuffer int 00118 * @return size of hardware work buffer B 00119 */ 00120 #define R_RGA_CalcWorkBufferB_Size( MaxWidthOfJPEG, MaxHeightOfJPEG, MaxBytePerPixelOfFrameBuffer ) \ 00121 ( R_CEIL_16U( MaxWidthOfJPEG ) * R_CEIL_16U( MaxHeightOfJPEG ) * (MaxBytePerPixelOfFrameBuffer) ) 00122 R_STATIC_ASSERT_GLOBAL( RGA_JPEG_MAX_WIDTH_ALIGNMENT == 16, "" ); /* check of R_CEIL_16U */ 00123 R_STATIC_ASSERT_GLOBAL( RGA_JPEG_MAX_HEIGHT_ALIGNMENT == 16, "" ); /* check of R_CEIL_16U */ 00124 00125 00126 #ifdef __cplusplus 00127 } /* extern "C" */ 00128 #endif /* __cplusplus */ 00129 00130 /* Inline Functions */ 00131 #include "RGA_Port_inline.h" 00132 00133 #endif /* RGA_PORT_H */ 00134
Generated on Tue Jul 12 2022 11:15:04 by
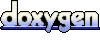