Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
RGA_Callback.c
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2013 - 2014 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /** 00024 * @file RGA_Callback.c 00025 * @brief $Module: RGA $ $PublicVersion: 1.20 $ (=RGA_VERSION) 00026 * $Rev: $ 00027 * $Date:: $ 00028 */ 00029 00030 #include <string.h> 00031 #include "RGA.h" 00032 #include "RGA_Callback_private.h " 00033 #include "clib_drivers.h " 00034 00035 00036 00037 /** gs_WorkBufferMemory */ 00038 static uint8_t *gs_WorkBufferMemory; 00039 static size_t gs_WorkBufferMemory_Size; 00040 static uint8_t *gs_WorkBufferB_Memory; 00041 static size_t gs_WorkBufferB_Memory_Size; 00042 00043 00044 /** 00045 * @struct GraphicsDefaultSubClass 00046 * @brief GraphicsDefaultSubClass 00047 */ 00048 typedef struct _GraphicsDefaultSubClass GraphicsDefaultSubClass; 00049 struct _GraphicsDefaultSubClass { 00050 uint8_t *WorkBufferMemory; 00051 size_t work_buffer_size; 00052 bool_t IsUsedWorkBuffer; 00053 uint8_t *WorkBufferB_Memory; 00054 size_t work_buffer_b_size; 00055 bool_t IsUsedWorkBufferB; 00056 }; 00057 00058 00059 /* Section: Global */ 00060 /** gs_GraphicsDefaultSub */ 00061 static GraphicsDefaultSubClass gs_GraphicsDefaultSub = { 00062 NULL, /* WorkBufferMemory */ 00063 0, /* work_buffer_size */ 00064 false, /* IsUsedWorkBuffer */ 00065 NULL, /* WorkBufferB_Memory */ 00066 0, /* work_buffer_b_size */ 00067 false, /* IsUsedWorkBufferB */ 00068 }; 00069 00070 00071 00072 /** 00073 * @brief R_GRAPHICS_STATIC_OnInitializeDefault 00074 * 00075 * @par Parameters 00076 * None 00077 * @return None. 00078 */ 00079 errnum_t R_GRAPHICS_STATIC_OnInitializeDefault( graphics_t *self, graphics_config_t *in_out_Config, 00080 void **out_CalleeDefined ) 00081 { 00082 enum { work_buffer_flags = 00083 F_GRAPHICS_WORK_BUFFER_ADDRESS | 00084 F_GRAPHICS_WORK_BUFFER_SIZE | 00085 F_GRAPHICS_MAX_WIDTH_OF_FRAME_BUFFER | 00086 F_GRAPHICS_MAX_HEIGHT_OF_FRAME_BUFFER 00087 }; 00088 00089 enum { work_buffer_B_flags = 00090 F_GRAPHICS_WORK_BUFFER_B_ADDRESS | 00091 F_GRAPHICS_WORK_BUFFER_B_SIZE 00092 }; 00093 00094 static r_ospl_c_lock_t gs_c_lock_object; 00095 00096 errnum_t e; 00097 00098 GraphicsDefaultSubClass *sub = &gs_GraphicsDefaultSub; 00099 00100 R_UNREFERENCED_VARIABLE_2( self, out_CalleeDefined ); 00101 00102 R_MEMORY_SECTION_GetMemory_RGA_WorkBuffer( &gs_WorkBufferMemory, &gs_WorkBufferMemory_Size, 00103 &gs_WorkBufferB_Memory, &gs_WorkBufferB_Memory_Size ); 00104 00105 00106 /* Set default configuration */ 00107 00108 /* Use work buffer */ 00109 if ( IS_ANY_BITS_NOT_SET( in_out_Config->flags, work_buffer_flags ) ) { 00110 ASSERT_R( IS_ALL_BITS_NOT_SET( in_out_Config->flags, work_buffer_flags ), e=E_OTHERS; goto fin ); 00111 ASSERT_R( ! sub->IsUsedWorkBuffer, e=E_OTHERS; goto fin ); 00112 00113 #if RGA_WORK_BUFFER_ADDRESS_ALIGNMENT == 64 /* Check of "R_Ceil_64u" */ 00114 sub->WorkBufferMemory = (uint8_t *) R_Ceil_64u( (uintptr_t) gs_WorkBufferMemory ); 00115 #elif RGA_WORK_BUFFER_ADDRESS_ALIGNMENT == 4 /* Check of "R_Ceil_4u" */ 00116 sub->WorkBufferMemory = (uint8_t *) R_Ceil_4u( (uintptr_t) gs_WorkBufferMemory ); 00117 #else 00118 #error 00119 #endif 00120 sub->work_buffer_size = gs_WorkBufferMemory_Size - ( sub->WorkBufferMemory - gs_WorkBufferMemory ); 00121 00122 in_out_Config->flags |= work_buffer_flags; 00123 in_out_Config->work_buffer_address = sub->WorkBufferMemory; 00124 in_out_Config->work_buffer_size = sub->work_buffer_size; 00125 in_out_Config->max_width_of_frame_buffer = MAX_WIDTH_OF_FRAME_BUFFER; 00126 in_out_Config->max_height_of_frame_buffer = MAX_HEIGHT_OF_FRAME_BUFFER; 00127 00128 sub->IsUsedWorkBuffer = true; 00129 } 00130 00131 /* Use work buffer B */ 00132 if ( IS_ANY_BITS_NOT_SET( in_out_Config->flags, work_buffer_B_flags ) ) { 00133 ASSERT_R( IS_ALL_BITS_NOT_SET( in_out_Config->flags, work_buffer_B_flags ), e=E_OTHERS; goto fin ); 00134 ASSERT_R( ! sub->IsUsedWorkBufferB, e=E_OTHERS; goto fin ); 00135 00136 #if RGA_WORK_BUFFER_B_ADDRESS_ALIGNMENT == 32 /* Check of "R_Ceil_32u" */ 00137 sub->WorkBufferB_Memory = (uint8_t *) R_Ceil_32u( (uintptr_t) gs_WorkBufferB_Memory ); 00138 #elif RGA_WORK_BUFFER_B_ADDRESS_ALIGNMENT == 4 /* Check of "R_Ceil_4u" */ 00139 sub->WorkBufferB_Memory = (uint8_t *) R_Ceil_4u( (uintptr_t) gs_WorkBufferB_Memory ); 00140 #else 00141 #error 00142 #endif 00143 sub->work_buffer_b_size = gs_WorkBufferB_Memory_Size - ( sub->WorkBufferB_Memory - gs_WorkBufferB_Memory ); 00144 00145 in_out_Config->flags |= work_buffer_B_flags; 00146 in_out_Config->work_buffer_b_address = sub->WorkBufferB_Memory; 00147 in_out_Config->work_buffer_b_size = sub->work_buffer_b_size; 00148 00149 sub->IsUsedWorkBufferB = true; 00150 } 00151 00152 #ifdef WORK_SIZE_FOR_LIB_PNG 00153 in_out_Config->flags |= F_GRAPHICS_WORK_SIZE_FOR_LIBPNG; 00154 in_out_Config->work_size_for_libPNG = WORK_SIZE_FOR_LIB_PNG; 00155 #endif 00156 00157 R_OSPL_MEMORY_Flush( R_OSPL_FLUSH_WRITEBACK_INVALIDATE ); 00158 /* Avoid write back by initialization to global variables of buffers */ 00159 00160 /* Lock object */ 00161 in_out_Config->flags |= F_GRAPHICS_LOCK_OBJECT; 00162 in_out_Config->lock_object = &gs_c_lock_object; 00163 00164 e=0; 00165 fin: 00166 return e; 00167 } 00168 00169 00170 00171 /** 00172 * @brief R_GRAPHICS_STATIC_OnFinalizeDefault 00173 * 00174 * @par Parameters 00175 * None 00176 * @return None. 00177 */ 00178 errnum_t R_GRAPHICS_STATIC_OnFinalizeDefault( graphics_t *self, void *CalleeDefined, errnum_t e ) 00179 { 00180 GraphicsDefaultSubClass *sub = &gs_GraphicsDefaultSub; 00181 00182 R_UNREFERENCED_VARIABLE_2( self, CalleeDefined ); 00183 sub->IsUsedWorkBuffer = false; 00184 sub->IsUsedWorkBufferB = false; 00185 return e; 00186 } 00187 00188 00189 /** 00190 * @brief drawCLUTImage 00191 * 00192 * @par Parameters 00193 * None 00194 * @return None. 00195 */ 00196 errnum_t drawCLUTImage( uint8_t *SrcAddress, const graphics_image_t *image, frame_buffer_t *frame ) 00197 { 00198 errnum_t e; 00199 int_t image_w; 00200 int i; 00201 uint32_t ImageWidthPoint; 00202 uint8_t *DestAddress; 00203 graphics_image_properties_t image_prop; 00204 00205 DestAddress = frame->buffer_address[ frame->draw_buffer_index ]; 00206 00207 switch( frame->pixel_format ) { 00208 case PIXEL_FORMAT_CLUT8: 00209 ImageWidthPoint = 1; 00210 break; 00211 case PIXEL_FORMAT_CLUT4: 00212 ImageWidthPoint = 2; 00213 break; 00214 case PIXEL_FORMAT_CLUT1: 00215 ImageWidthPoint = 8; 00216 break; 00217 default: 00218 /* never comes here */ 00219 ImageWidthPoint = 1; 00220 break; 00221 } 00222 00223 e = R_GRAPHICS_IMAGE_GetProperties( image, &image_prop ); 00224 IF(e)goto fin; 00225 ASSERT_R( image_prop.pixelFormat == frame->pixel_format , e=E_NOT_SUPPORTED_PIXEL_FORMAT; goto fin ); 00226 ASSERT_R( ( image->width % ImageWidthPoint ) == 0 , e=E_OTHERS; goto fin ); 00227 ASSERT_R( image->width <= frame->width , e=E_OTHERS; goto fin ); 00228 ASSERT_R( image->height <= frame->height , e=E_OTHERS; goto fin ); 00229 ASSERT_R( frame->stride % 32 == 0 , e=E_OTHERS; goto fin ); 00230 00231 image_w = image_prop.width; 00232 switch( frame->pixel_format ) { 00233 case PIXEL_FORMAT_CLUT8: 00234 SrcAddress += sizeof( uint32_t ) * 256; 00235 break; 00236 case PIXEL_FORMAT_CLUT4: 00237 image_w = image_w / 2; 00238 SrcAddress += sizeof( uint32_t ) * 16; 00239 break; 00240 case PIXEL_FORMAT_CLUT1: 00241 image_w = image_w / 8; 00242 SrcAddress += sizeof( uint32_t ) * 2; 00243 break; 00244 default: 00245 e=E_OTHERS; 00246 goto fin; 00247 } 00248 for( i = 0; i < image->height ; i++ ) { 00249 memcpy(( void *) DestAddress, ( void *)SrcAddress, (size_t)image_w ); 00250 DestAddress += frame->stride; 00251 SrcAddress += image_w; 00252 } 00253 e = 0; 00254 fin: 00255 return e; 00256 } 00257 00258
Generated on Tue Jul 12 2022 11:15:04 by
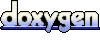