Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
JPEG_Converter.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer* 00021 * Copyright (C) 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /**************************************************************************//** 00024 * @file JPEG_Converter.h 00025 * @brief JCU API 00026 ******************************************************************************/ 00027 00028 #ifndef JPEG_CONVERTER_H 00029 #define JPEG_CONVERTER_H 00030 00031 /****************************************************************************** 00032 Includes <System Includes> , "Project Includes" 00033 ******************************************************************************/ 00034 00035 /****************************************************************************** 00036 Macro definitions 00037 ******************************************************************************/ 00038 00039 /** A class to communicate a JCU 00040 * 00041 */ 00042 class JPEG_Converter { 00043 00044 public: 00045 /*! @enum jpeg_conv_error_t 00046 @brief Error codes 00047 */ 00048 typedef enum { 00049 JPEG_CONV_OK = 0, /*!< Normal termination */ 00050 JPEG_CONV_JCU_ERR = -1, /*!< JCU driver error */ 00051 JPEG_CONV_FORMA_ERR = -2, /*!< Not support format */ 00052 JPEG_CONV_PARAM_ERR = -3, /*!< Parameter error */ 00053 JPEG_CONV_BUSY = -4, /*!< JCU Busy */ 00054 JPEG_CONV_PARAM_RANGE_ERR = -7, /*!< Parameter range error */ 00055 } jpeg_conv_error_t; 00056 00057 /*! @enum wr_rd_swa_t 00058 @brief Write/Read image pixcel frame buffer swap setting 00059 */ 00060 typedef enum { 00061 WR_RD_WRSWA_NON = 0, /*!< Not swapped: 1-2-3-4-5-6-7-8 */ 00062 WR_RD_WRSWA_8BIT = 1, /*!< Swapped in 8-bit units: 2-1-4-3-6-5-8-7 */ 00063 WR_RD_WRSWA_16BIT = 2, /*!< Swapped in 16-bit units: 3-4-1-2-7-8-5-6 */ 00064 WR_RD_WRSWA_16_8BIT = 3, /*!< Swapped in 16-bit units + 8-bit units: 4-3-2-1-8-7-6-5 */ 00065 WR_RD_WRSWA_32BIT = 4, /*!< Swapped in 32-bit units: 5-6-7-8-1-2-3-4 */ 00066 WR_RD_WRSWA_32_8BIT = 5, /*!< Swapped in 32-bit units + 8-bit units: 6-5-8-7-2-1-4-3 */ 00067 WR_RD_WRSWA_32_16BIT = 6, /*!< Swapped in 32-bit units + 16-bit units: 7-8-5-6-3-4-1-2 */ 00068 WR_RD_WRSWA_32_16_8BIT = 7, /*!< Swapped in 32-bit units + 16-bit units + 8-bit units: 8-7-6-5-4-3-2-1 */ 00069 } wr_rd_swa_t; 00070 00071 /*! @enum wr_rd_format_t 00072 @brief Write/Read image pixcel format selects 00073 */ 00074 typedef enum { 00075 WR_RD_YCbCr422 = 0x00, /*!< YCbCr422 (2byte / px) */ 00076 WR_RD_ARGB8888 = 0x01, /*!< ARGB8888 (4byte / px) */ 00077 WR_RD_RGB565 = 0x02, /*!< RGB565 (2byte / px) */ 00078 } wr_rd_format_t; 00079 00080 /*! @enum sub_sampling_t 00081 @brief Thinning output image selects 00082 */ 00083 typedef enum { 00084 SUB_SAMPLING_1_1 = 0x00, /*!< Thinning output image to 1/1 */ 00085 SUB_SAMPLING_1_2 = 0x01, /*!< Thinning output image to 1/2 */ 00086 SUB_SAMPLING_1_4 = 0x02, /*!< Thinning output image to 1/4 */ 00087 SUB_SAMPLING_1_8 = 0x03 /*!< Thinning output image to 1/8 */ 00088 } sub_sampling_t; 00089 00090 /*! @enum cbcr_offset_t 00091 @brief Cb/Cr range selects for decode 00092 */ 00093 typedef enum { 00094 CBCR_OFFSET_0 = 0x00, /*!< Cb/Cr range -128 to 127 (all format used) */ 00095 CBCR_OFFSET_128 = 0x01, /*!< Cb/Cr range 0 to 255 (Default and YCbCr format only used) */ 00096 } cbcr_offset_t; 00097 00098 /*! @struct bitmap_buff_info_t 00099 @brief Bitmap data setting struct 00100 */ 00101 typedef struct { 00102 int32_t width ; /*!< width width of bitmap data */ 00103 int32_t height ; /*!< height height of bitmap data */ 00104 wr_rd_format_t format ; /*!< format format of bitmap data */ 00105 void* buffer_address ; /*!< buffer_address address of bitmap data */ 00106 } bitmap_buff_info_t; 00107 00108 /** Constructor method of JPEG converter(encode/decode) 00109 */ 00110 JPEG_Converter(); 00111 00112 /** Destructor method of JPEG converter(encode/decode) 00113 */ 00114 virtual ~JPEG_Converter(); 00115 00116 /*! @struct DecodeOptions_t 00117 @brief Decode option setting 00118 */ 00119 struct decode_options_t { 00120 sub_sampling_t vertical_sub_sampling; /*!< Vertical sampling setting */ 00121 sub_sampling_t horizontal_sub_sampling; /*!< Horizontal sampling setting */ 00122 cbcr_offset_t output_cb_cr_offset; /*!< Output data Cb/Cr range setting */ 00123 wr_rd_swa_t output_swapsetting; /*!< Output data swap setteing */ 00124 int32_t alpha; /*!< alpha setting for ARGB8888 */ 00125 bool check_jpeg_format; /*!< Flag check JPEG data format : Setting NULL does not check JPEG format when decoding */ 00126 void (* p_DecodeCallBackFunc)(jpeg_conv_error_t err_code); /*!< Callback function address */ 00127 00128 decode_options_t() { 00129 vertical_sub_sampling = SUB_SAMPLING_1_1 ; 00130 horizontal_sub_sampling = SUB_SAMPLING_1_1 ; 00131 output_cb_cr_offset = CBCR_OFFSET_128 ; 00132 output_swapsetting = WR_RD_WRSWA_8BIT ; 00133 alpha = 0; 00134 check_jpeg_format = false; 00135 p_DecodeCallBackFunc = NULL; 00136 } 00137 }; 00138 00139 /*! @struct encode_options_t 00140 @brief Encode option setting 00141 */ 00142 struct encode_options_t { 00143 int32_t DRI_value ; /*!< DRI setting */ 00144 int32_t width ; /*!< Encode width */ 00145 int32_t height ; /*!< Encode height */ 00146 cbcr_offset_t input_cb_cr_offset ; /*!< Input data Cb/Cr range setting */ 00147 wr_rd_swa_t input_swapsetting ; /*!< Input data swap setteing */ 00148 size_t encode_buff_size ; /*!< Encode buffer size : Setting 0 does not the buffer size confirmation of when encoding */ 00149 void* quantization_table_Y ; /*!< Quantization table address(Y) */ 00150 void* quantization_table_C ; /*!< Quantization table address(C) */ 00151 void* huffman_table_Y_DC ; /*!< Huffman Table address(Y DC) */ 00152 void* huffman_table_C_DC ; /*!< Huffman Table address(C DC) */ 00153 void* huffman_table_Y_AC ; /*!< Huffman Table address(Y AC) */ 00154 void* huffman_table_C_AC ; /*!< Huffman Table address(C AC) */ 00155 void (* p_EncodeCallBackFunc )(jpeg_conv_error_t err_code); /*!< Callback function address */ 00156 00157 encode_options_t() { 00158 DRI_value = 0; 00159 width = 0; 00160 height = 0; 00161 input_cb_cr_offset = CBCR_OFFSET_128 ; 00162 input_swapsetting = WR_RD_WRSWA_8BIT ; 00163 encode_buff_size = 0; 00164 quantization_table_Y = NULL; 00165 quantization_table_C = NULL; 00166 huffman_table_Y_DC = NULL; 00167 huffman_table_C_DC = NULL; 00168 huffman_table_Y_AC = NULL; 00169 huffman_table_C_AC = NULL; 00170 p_EncodeCallBackFunc = NULL; 00171 } 00172 }; 00173 00174 /** Decode JPEG to rinear data 00175 * 00176 * @param[in] void* pJpegBuff : Input JPEG data address 00177 * @param[in/out] bitmap_buff_info_t* psOutputBuff : Output bitmap data address 00178 * @param[in] decode_options_t* pOptions : Decode option(Optional) 00179 * @return JPEG_CONV_OK = success 00180 * JPEG_CONV_JCU_ERR = failure (JCU error) 00181 * JPEG_CONV_FORMA_ERR = failure (data format error) 00182 * JPEG_CONV_PARAM_ERR = failure (input parameter error) 00183 * JPEG_CONV_PARAM_RANGE_ERR = failure (input parameter range error) 00184 */ 00185 JPEG_Converter::jpeg_conv_error_t decode(void* pJpegBuff, bitmap_buff_info_t* psOutputBuff ); 00186 JPEG_Converter::jpeg_conv_error_t decode(void* pJpegBuff, bitmap_buff_info_t* psOutputBuff, decode_options_t* pOptions ); 00187 00188 /** Encode rinear data to JPEG 00189 * 00190 * @param[in] bitmap_buff_info_t* psInputBuff : Input bitmap data address 00191 * @param[out] void* pJpegBuff : Output JPEG data address 00192 * @param[out] size_t* pEncodeSize : Encode size address 00193 * @param[in] encode_options_t* pOptions[IN] : Encode option(Optional) 00194 * @return JPEG_CONV_OK = success 00195 * JPEG_CONV_JCU_ERR = failure (JCU error) 00196 * JPEG_CONV_FORMA_ERR = failure (data format error) 00197 * JPEG_CONV_PARAM_ERR = failure (input parameter error) 00198 * JPEG_CONV_PARAM_RANGE_ERR = failure (input parameter range error) 00199 */ 00200 JPEG_Converter::jpeg_conv_error_t encode(bitmap_buff_info_t* psInputBuff, void* pJpegBuff, size_t* pEncodeSize ); 00201 JPEG_Converter::jpeg_conv_error_t encode(bitmap_buff_info_t* psInputBuff, void* pJpegBuff, size_t* pEncodeSize, encode_options_t* pOptions ); 00202 00203 /** Set encode quality 00204 * 00205 * @param[in] uint8_t qual : Encode quality (1 <= qual <= 100) 00206 * @return JPEG_CONV_OK = success 00207 * JPEG_CONV_PARAM_RANGE_ERR = failure (input parameter range error) 00208 */ 00209 JPEG_Converter::jpeg_conv_error_t SetQuality(const uint8_t qual); 00210 00211 private: 00212 00213 }; 00214 00215 #endif /* JPEG_CONVERTER_H */
Generated on Tue Jul 12 2022 11:15:01 by
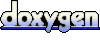