Fork of Maxim's human body temp sensor library that works with mbed-os 5
Fork of MAX30205 by
MAX30205 Class Reference
Library for the MAX30205
The MAX30205 temperature sensor accurately measures temperature and provide an overtemperature alarm/interrupt/shutdown output.
More...
#include <MAX30205.h>
Data Structures | |
union | Configuration_u |
MAX30205 Configuration register bitfields. More... | |
Public Types | |
enum | Registers_e |
MAX30205 Register Addresses. More... | |
Public Member Functions | |
MAX30205 (I2C &i2c, uint8_t slaveAddress) | |
Constructor using reference to I2C object. | |
~MAX30205 (void) | |
Destructor. | |
int32_t | readTemperature (uint16_t &value) |
Read the temperature from the device into a 16 bit value. | |
int32_t | readConfiguration (Configuration_u &config) |
Read the configuration register. | |
int32_t | writeConfiguration (const Configuration_u config) |
Write the configuration register with given configuration. | |
int32_t | readTHYST (uint16_t &value) |
Read the THYST value from a specified device instance. | |
int32_t | writeTHYST (const uint16_t value) |
Write the THYST to a device instance. | |
int32_t | readTOS (uint16_t &value) |
Read the TOS value from device. | |
int32_t | writeTOS (const uint16_t value) |
Write the TOS register. | |
float | toCelsius (uint32_t rawTemp) |
Convert a raw temperature value into a float. | |
float | toFahrenheit (float temperatureC) |
Convert the passed in temperature in C to Fahrenheit. | |
Protected Member Functions | |
int32_t | writeRegister (Registers_e reg, uint16_t value) |
Write register of device at slave address. | |
int32_t | readRegister (Registers_e reg, uint16_t &value) |
Read register of device at slave address. |
Detailed Description
Library for the MAX30205
The MAX30205 temperature sensor accurately measures temperature and provide an overtemperature alarm/interrupt/shutdown output.
This device converts the temperature measurements to digital form using a high-resolution, sigma-delta, analog-to-digital converter (ADC). Accuracy meets clinical thermometry specification of the ASTM E1112 when soldered on the final PCB. Communication is through an I2C-compatible 2-wire serial interface.
#include "mbed.h" #include "max32630fthr.h" #include "MAX30205.h" MAX32630FTHR pegasus(MAX32630FTHR::VIO_3V3); //Get I2C instance I2C i2cBus(I2C1_SDA, I2C1_SCL); //Get temp sensor instance MAX30205 bodyTempSensor(i2cBus, 0x4D); //Constructor takes 7-bit slave adrs int main(void) { //use sensor }
Definition at line 67 of file MAX30205.h.
Member Enumeration Documentation
enum Registers_e |
MAX30205 Register Addresses.
Definition at line 72 of file MAX30205.h.
Constructor & Destructor Documentation
MAX30205 | ( | I2C & | i2c, |
uint8_t | slaveAddress | ||
) |
Constructor using reference to I2C object.
- Parameters:
-
i2c - Reference to I2C object slaveAddress - 7-bit I2C address
Definition at line 39 of file MAX30205.cpp.
~MAX30205 | ( | void | ) |
Destructor.
Definition at line 46 of file MAX30205.cpp.
Member Function Documentation
int32_t readConfiguration | ( | Configuration_u & | config ) |
Read the configuration register.
- Parameters:
-
config - Reference to Configuration type
- Returns:
- 0 on success, non-zero on failure
Definition at line 60 of file MAX30205.cpp.
int32_t readRegister | ( | Registers_e | reg, |
uint16_t & | value | ||
) | [protected] |
Read register of device at slave address.
- Parameters:
-
reg - Register address [out] value - Read data on success
- Returns:
- 0 on success, non-zero on failure
Definition at line 150 of file MAX30205.cpp.
int32_t readTemperature | ( | uint16_t & | value ) |
Read the temperature from the device into a 16 bit value.
- Parameters:
-
[out] value - Raw temperature data on success
- Returns:
- 0 on success, non-zero on failure
Definition at line 53 of file MAX30205.cpp.
int32_t readTHYST | ( | uint16_t & | value ) |
Read the THYST value from a specified device instance.
- Parameters:
-
[out] value - THYST register value on success
- Returns:
- 0 on success, non-zero on failure
Definition at line 85 of file MAX30205.cpp.
int32_t readTOS | ( | uint16_t & | value ) |
Read the TOS value from device.
- Parameters:
-
[out] value - TOS register value on success
- Returns:
- 0 on success, non-zero on failure
Definition at line 99 of file MAX30205.cpp.
float toCelsius | ( | uint32_t | rawTemp ) |
Convert a raw temperature value into a float.
- Parameters:
-
rawTemp - raw temperature value to convert
- Returns:
- the convereted value in degrees C
Definition at line 113 of file MAX30205.cpp.
float toFahrenheit | ( | float | temperatureC ) |
Convert the passed in temperature in C to Fahrenheit.
- Parameters:
-
temperatureC Temperature in C to convert
- Returns:
- Returns the converted Fahrenheit value
Definition at line 128 of file MAX30205.cpp.
int32_t writeConfiguration | ( | const Configuration_u | config ) |
Write the configuration register with given configuration.
- Parameters:
-
config - Configuration to write
- Returns:
- 0 on success, non-zero on failure
Definition at line 76 of file MAX30205.cpp.
int32_t writeRegister | ( | Registers_e | reg, |
uint16_t | value | ||
) | [protected] |
Write register of device at slave address.
- Parameters:
-
reg - Register address value - Value to write
- Returns:
- 0 on success, non-zero on failure
Definition at line 135 of file MAX30205.cpp.
int32_t writeTHYST | ( | const uint16_t | value ) |
Write the THYST to a device instance.
- Parameters:
-
value - 16-bit value to write
- Returns:
- 0 on success, non-zero on failure
Definition at line 92 of file MAX30205.cpp.
int32_t writeTOS | ( | const uint16_t | value ) |
Write the TOS register.
- Parameters:
-
value - 16-bit value to write
- Returns:
- 0 on success, non-zero on failure
Definition at line 106 of file MAX30205.cpp.
Generated on Thu Jul 14 2022 10:01:50 by
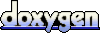