
Host API Example for the ADMW1001
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
Go to the documentation of this file.
00001 /* 00002 Copyright 2019 (c) Analog Devices, Inc. 00003 00004 All rights reserved. 00005 00006 Redistribution and use in source and binary forms, with or without 00007 modification, are permitted provided that the following conditions are met: 00008 - Redistributions of source code must retain the above copyright 00009 notice, this list of conditions and the following disclaimer. 00010 - Redistributions in binary form must reproduce the above copyright 00011 notice, this list of conditions and the following disclaimer in 00012 the documentation and/or other materials provided with the 00013 distribution. 00014 - Neither the name of Analog Devices, Inc. nor the names of its 00015 contributors may be used to endorse or promote products derived 00016 from this software without specific prior written permission. 00017 - The use of this software may or may not infringe the patent rights 00018 of one or more patent holders. This license does not release you 00019 from the requirement that you obtain separate licenses from these 00020 patent holders to use this software. 00021 - Use of the software either in source or binary form, must be run 00022 on or directly connected to an Analog Devices Inc. component. 00023 00024 THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00025 IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00026 MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00027 IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00028 INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 00035 *****************************************************************************/ 00036 /*! 00037 ****************************************************************************** 00038 * @file: 00039 *----------------------------------------------------------------------------- 00040 * 00041 */ 00042 #include "mbed.h" 00043 #include "admw_api.h" 00044 #include "admw1001/admw1001_api.h" 00045 #include "admw_log.h" 00046 #include "common/utils.h" 00047 #include "common/platform.h" 00048 #include "admw1001/ADMW1001_REGISTERS.h" 00049 00050 extern ADMW_CONFIG sensor0_typeK_cjc0_config; 00051 extern ADMW_CONFIG sensor1_rtd_3w_pt100_config; 00052 extern ADMW_CONFIG sensor2_typeT_cjc0_config; 00053 extern ADMW_CONFIG sensor3_typeJ_cjc0_config; 00054 extern ADMW_CONFIG i2c0_sensirionSHT3X_config; 00055 extern ADMW_CONFIG spi0_adiAdxl362_config; 00056 extern ADMW_CONFIG multichannel_continuous_config; 00057 extern ADMW_CONFIG multichannel_multicycle_config; 00058 extern ADMW_CONFIG multichannel_singlecycle_config; 00059 extern ADMW_CONFIG sensor2_bridge_4w_load_cell_config; 00060 extern ADMW_CONFIG config; 00061 /* Change the following pointer to select any of the configurations above */ 00062 static ADMW_CONFIG *pSelectedConfig = &multichannel_continuous_config; 00063 00064 static ADMW_CONNECTION connectionInfo = PLATFORM_CONNECTION_INFO; 00065 00066 00067 int main() 00068 { 00069 ADMW_RESULT res; 00070 ADMW_STATUS status; 00071 ADMW_DEVICE_HANDLE hDevice; 00072 ADMW_MEASUREMENT_MODE eMeasurementMode = ADMW_MEASUREMENT_MODE_NORMAL ; 00073 bool bDeviceReady; 00074 00075 /* 00076 * Open an ADMW1001 device instance. 00077 */ 00078 res = admw_Open(0, &connectionInfo, &hDevice); 00079 if (res != ADMW_SUCCESS ) { 00080 ADMW_LOG_ERROR("Failed to open device instance"); 00081 return res; 00082 } 00083 00084 /* 00085 * Reset the given ADMW1001 device.... 00086 */ 00087 ADMW_LOG_INFO("Resetting ADMW1001 device, please wait..."); 00088 res = admw_Reset(hDevice); 00089 if (res != ADMW_SUCCESS ) { 00090 ADMW_LOG_ERROR("Failed to reset device"); 00091 return res; 00092 } 00093 /* 00094 * ...and wait until the device is ready. 00095 */ 00096 do { 00097 wait_ms(100); 00098 res = admw_GetDeviceReadyState(hDevice, &bDeviceReady); 00099 if (res != ADMW_SUCCESS ) { 00100 ADMW_LOG_ERROR("Failed to get device ready-state"); 00101 return res; 00102 } 00103 } while (! bDeviceReady); 00104 ADMW_LOG_INFO("ADMW1001 device ready"); 00105 /* 00106 * Write configuration settings to the device registers. 00107 * Settings are not applied until admw_ApplyConfigUpdates() is called. 00108 */ 00109 ADMW_LOG_INFO("Setting device configuration"); 00110 res = admw_SetConfig(hDevice, pSelectedConfig); 00111 if (res != ADMW_SUCCESS ) { 00112 ADMW_LOG_ERROR("Failed to set device configuration"); 00113 return res; 00114 } 00115 res = admw_ApplyConfigUpdates(hDevice); 00116 if (res != ADMW_SUCCESS ) { 00117 ADMW_LOG_ERROR("Failed to apply device configuration"); 00118 return res; 00119 } 00120 /* 00121 * Check device status after updating the configuration 00122 */ 00123 res = admw_GetStatus(hDevice, &status); 00124 deviceInformation(hDevice); 00125 if (res != ADMW_SUCCESS ) { 00126 ADMW_LOG_ERROR("Failed to retrieve device status"); 00127 return res; 00128 } 00129 if (status.deviceStatus & 00130 (ADMW_DEVICE_STATUS_ERROR | ADMW_DEVICE_STATUS_ALERT )) { 00131 utils_printStatus(&status); 00132 } 00133 00134 /* 00135 * Kick off the measurement cycle here 00136 */ 00137 ADMW_LOG_INFO("Configuration completed, starting measurement cycles"); 00138 utils_runMeasurement(hDevice, eMeasurementMode); 00139 00140 /* 00141 * Clean up and exit 00142 */ 00143 res = admw_Close(hDevice); 00144 if (res != ADMW_SUCCESS ) { 00145 ADMW_LOG_ERROR("Failed to close device instance"); 00146 return res; 00147 } 00148 00149 return 0; 00150 } 00151
Generated on Wed Jul 13 2022 03:04:54 by
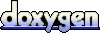