
Host API Example for the ADMW1001
ADMW Host Library API
Data Structures | |
struct | ADMW_CONNECTION |
struct | ADMW_STATUS |
struct | ADMW_DATA_SAMPLE |
struct | ADMW_CONFIG_VERSION_ID |
struct | ADMW_CONFIG |
Modules | |
ADMW1001 Host Library API | |
Typedefs | |
typedef void * | ADMW_DEVICE_HANDLE |
Enumerations | |
enum | ADMW_CONNECTION_TYPE { ADMW_CONNECTION_TYPE_SPI = 1 } |
enum | ADMW_DEVICE_STATUS_FLAGS { ADMW_DEVICE_STATUS_BUSY = (1 << 0), ADMW_DEVICE_STATUS_DATAREADY = (1 << 1), ADMW_DEVICE_STATUS_ERROR = (1 << 2), ADMW_DEVICE_STATUS_ALERT = (1 << 3), ADMW_DEVICE_STATUS_FIFO_ERROR = (1 << 4), ADMW_DEVICE_STATUS_CONFIG_ERROR = (1 << 5), ADMW_DEVICE_STATUS_LUT_ERROR = (1 << 6) } |
enum | ADMW_DIAGNOSTICS_STATUS_FLAGS { ADMW_DIAGNOSTICS_STATUS_CHECKSUM_ERROR = (1 << 0), ADMW_DIAGNOSTICS_STATUS_CONVERSION_ERROR = (1 << 8), ADMW_DIAGNOSTICS_STATUS_CALIBRATION_ERROR = (1 << 9) } |
enum | ADMW_CHANNEL_ALERT_FLAGS { ADMW_CHANNEL_ALERT_TIMEOUT = (1 << 0), ADMW_CHANNEL_ALERT_UNDER_RANGE = (1 << 1), ADMW_CHANNEL_ALERT_OVER_RANGE = (1 << 2), ADMW_CHANNEL_ALERT_LOW_LIMIT = (1 << 3), ADMW_CHANNEL_ALERT_HIGH_LIMIT = (1 << 4), ADMW_CHANNEL_ALERT_SENSOR_OPEN = (1 << 5), ADMW_CHANNEL_ALERT_REF_DETECT = (1 << 6), ADMW_CHANNEL_ALERT_CONFIG_ERR = (1 << 7), ADMW_CHANNEL_ALERT_LUT_ERR = (1 << 8), ADMW_CHANNEL_ALERT_SENSOR_NOT_READY = (1 << 9), ADMW_CHANNEL_ALERT_COMP_NOT_READY = (1 << 10), ADMW_CHANNEL_ALERT_LUT_UNDER_RANGE = (1 << 13), ADMW_CHANNEL_ALERT_LUT_OVER_RANGE = (1 << 14) } |
enum | ADMW_MEASUREMENT_MODE { ADMW_MEASUREMENT_MODE_NORMAL = 0, ADMW_MEASUREMENT_MODE_OMIT_RAW } |
enum | ADMW_USER_CONFIG_SLOT |
enum | ADMW_PRODUCT_ID { ADMW_PRODUCT_ID_ADMW1001 = 0x0020 } |
Functions | |
ADMW_RESULT | admw_Open (unsigned const nDeviceIndex, ADMW_CONNECTION *const pConnectionInfo, ADMW_DEVICE_HANDLE *const phDevice) |
Open ADMW device handle and set up communication interface. | |
ADMW_RESULT | admw_Close (ADMW_DEVICE_HANDLE const hDevice) |
Close ADMW device context and free resources. | |
ADMW_RESULT | admw_GetGpioState (ADMW_DEVICE_HANDLE const hDevice, ADMW_GPIO_PIN const ePinId, bool *const pbAsserted) |
Get the current state of the specified GPIO input signal. | |
ADMW_RESULT | admw_RegisterGpioCallback (ADMW_DEVICE_HANDLE const hDevice, ADMW_GPIO_PIN const ePinId, ADMW_GPIO_CALLBACK const callbackFunction, void *const pCallbackParam) |
Register an application-defined callback function for GPIO interrupts. | |
ADMW_RESULT | admw_Shutdown (ADMW_DEVICE_HANDLE const hDevice) |
Trigger a shut down of the device. | |
ADMW_RESULT | admw_Reset (ADMW_DEVICE_HANDLE const hDevice) |
Reset the ADMW device. | |
ADMW_RESULT | admw_GetDeviceReadyState (ADMW_DEVICE_HANDLE const hDevice, bool *const pbReady) |
Check if the device is ready, following power-up or a reset. | |
ADMW_RESULT | admw_GetProductID (ADMW_DEVICE_HANDLE const hDevice, ADMW_PRODUCT_ID *const pProductId) |
Obtain the product ID from the device. | |
ADMW_RESULT | admw_SetConfig (ADMW_DEVICE_HANDLE const hDevice, ADMW_CONFIG *const pConfig) |
Write full configuration settings to the device registers. | |
ADMW_RESULT | admw_ApplyConfigUpdates (ADMW_DEVICE_HANDLE const hDevice) |
Apply the configuration settings currently stored in device registers. | |
ADMW_RESULT | admw_SaveConfig (ADMW_DEVICE_HANDLE const hDevice, ADMW_USER_CONFIG_SLOT const eSlotId) |
Store the configuration settings to persistent memory on the device. | |
ADMW_RESULT | admw_RestoreConfig (ADMW_DEVICE_HANDLE const hDevice, ADMW_USER_CONFIG_SLOT const eSlotId) |
Restore configuration settings from persistent memory on the device. | |
ADMW_RESULT | admw_EraseExternalFlash (ADMW_DEVICE_HANDLE const hDevice) |
Erases the external flash memory. | |
ADMW_RESULT | admw_GetExternalFlashSampleCount (ADMW_DEVICE_HANDLE const hDevice, uint32_t *nSampleCount) |
Gets the number of samples stored in the external flash memory. | |
ADMW_RESULT | admw_GetExternalFlashData (ADMW_DEVICE_HANDLE const hDevice, ADMW_DATA_SAMPLE *const pSamples, uint32_t const nIndex, uint32_t const nRequested, uint32_t *const pnReturned) |
Read measurement samples stored in the the external flash memory. | |
ADMW_RESULT | admw_SaveLutData (ADMW_DEVICE_HANDLE const hDevice) |
Store the LUT data to persistent memory on the device. | |
ADMW_RESULT | admw_RestoreLutData (ADMW_DEVICE_HANDLE const hDevice) |
Restore LUT data from persistent memory on the device. | |
ADMW_RESULT | admw_StartMeasurement (ADMW_DEVICE_HANDLE const hDevice, ADMW_MEASUREMENT_MODE const eMeasurementMode) |
Start the measurement cycles on the device. | |
ADMW_RESULT | admw_StopMeasurement (ADMW_DEVICE_HANDLE const hDevice) |
Stop the measurement cycles on the device. | |
ADMW_RESULT | admw_RunDiagnostics (ADMW_DEVICE_HANDLE const hDevice) |
Run built-in diagnostic checks on the device. | |
ADMW_RESULT | admw_RunCalibration (ADMW_DEVICE_HANDLE const hDevice) |
Run built-in calibration on the device. | |
ADMW_RESULT | admw_RunDigitalCalibration (ADMW_DEVICE_HANDLE const hDevice) |
Run built-in digital calibration on the device. | |
ADMW_RESULT | admw_GetStatus (ADMW_DEVICE_HANDLE const hDevice, ADMW_STATUS *const pStatus) |
Read the current status from the device registers. | |
ADMW_RESULT | admw_GetData (ADMW_DEVICE_HANDLE const hDevice, ADMW_MEASUREMENT_MODE const eMeasurementMode, ADMW_DATA_SAMPLE *const pSamples, uint8_t const nBytesPerSample, uint32_t const nRequested, uint32_t *const pnReturned) |
Read measurement data samples from the device registers. | |
ADMW_RESULT | admw_GetCommandRunningState (ADMW_DEVICE_HANDLE hDevice, bool *pbCommandRunning) |
Check if a command is currently running on the device. |
Detailed Description
Host library API common to the ADMW product family.
Typedef Documentation
typedef void* ADMW_DEVICE_HANDLE |
A handle used in all API functions to identify the ADMW device.
Definition at line 69 of file admw_api.h.
Enumeration Type Documentation
Bit masks (flags) for the different channel alert indicators.
- Enumerator:
Definition at line 127 of file admw_api.h.
enum ADMW_CONNECTION_TYPE |
Supported connection types for communication with the ADMW device.
Definition at line 81 of file admw_api.h.
Bit masks (flags) for the different device status indicators.
- Enumerator:
Definition at line 99 of file admw_api.h.
Bit masks (flags) for the different diagnostics status indicators.
- Enumerator:
Definition at line 117 of file admw_api.h.
Measurement mode options for the ADMW device. admw_StartMeasurement
- Enumerator:
Definition at line 194 of file admw_api.h.
enum ADMW_PRODUCT_ID |
A list of supported product identifiers
Definition at line 62 of file admw_config_types.h.
Identifiers for the user configuration slots in persistent memory.
Definition at line 204 of file admw_api.h.
Function Documentation
ADMW_RESULT admw_ApplyConfigUpdates | ( | ADMW_DEVICE_HANDLE const | hDevice ) |
Apply the configuration settings currently stored in device registers.
- Parameters:
-
[in] hDevice ADMW device context handle
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
Instructs the ADMW device to reload and apply configuration from the device configuration registers. Changes to configuration registers are ignored by the device until this function is called.
- Note:
- No other command must be running when this is called.
Definition at line 473 of file admw_1001.c.
ADMW_RESULT admw_Close | ( | ADMW_DEVICE_HANDLE const | hDevice ) |
Close ADMW device context and free resources.
- Parameters:
-
[in] hDevice ADMW device context handle
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
Definition at line 669 of file admw_1001.c.
ADMW_RESULT admw_EraseExternalFlash | ( | ADMW_DEVICE_HANDLE const | hDevice ) |
Erases the external flash memory.
- Parameters:
-
[in] hDevice ADMW device context handle
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
Sends the bulk erase instruction to the external flash device. All stored samples are deleted. It is a blocking operation and takes tens of seconds to complete.
- Note:
- No other command must be running when this is called.
ADMW_RESULT admw_GetCommandRunningState | ( | ADMW_DEVICE_HANDLE | hDevice, |
bool * | pbCommandRunning | ||
) |
Check if a command is currently running on the device.
- Parameters:
-
[in] hDevice ADMW device context handle [out] pbCommandRunning Pointer to return the command running status
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
Reads the device status register to check if a command is running.
Definition at line 409 of file admw_1001.c.
ADMW_RESULT admw_GetData | ( | ADMW_DEVICE_HANDLE const | hDevice, |
ADMW_MEASUREMENT_MODE const | eMeasurementMode, | ||
ADMW_DATA_SAMPLE *const | pSamples, | ||
uint8_t const | nBytesPerSample, | ||
uint32_t const | nRequested, | ||
uint32_t *const | pnReturned | ||
) |
Read measurement data samples from the device registers.
- Parameters:
-
[in] hDevice ADMW device context handle [in] eMeasurementMode Must be set to the same value used for admw_StartMeasurement(). [out] pSamples Pointer to return a set of requested data samples. [in] nBytesPerSample The size, in bytes, of each sample. [in] nRequested Number of requested data samples. [out] pnReturned Number of valid data samples successfully retrieved.
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
Reads the status registers and extracts the relevant information to return to the caller.
- Note:
- This is intended to be called only when the DATAREADY status signal is asserted.
Definition at line 583 of file admw_1001.c.
ADMW_RESULT admw_GetDeviceReadyState | ( | ADMW_DEVICE_HANDLE const | hDevice, |
bool *const | pbReady | ||
) |
Check if the device is ready, following power-up or a reset.
- Parameters:
-
[in] hDevice ADMW device context handle [out] pbReady Pointer to return true if the device is ready, or false otherwise
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
This function attempts to read a fixed-value device register via the communication interface.
Definition at line 843 of file admw_1001.c.
ADMW_RESULT admw_GetExternalFlashData | ( | ADMW_DEVICE_HANDLE const | hDevice, |
ADMW_DATA_SAMPLE *const | pSamples, | ||
uint32_t const | nIndex, | ||
uint32_t const | nRequested, | ||
uint32_t *const | pnReturned | ||
) |
Read measurement samples stored in the the external flash memory.
- Parameters:
-
[in] hDevice ADMW device context handle [out] pSamples Pointer to return a set of requested data samples. [in] nStartIndex Index of first sample to retrieve. [in] nBytesPerSample The size, in bytes, of each sample. [in] nRequested Number of requested data samples. [out] pnReturned Number of valid data samples successfully retrieved.
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
Reads the status registers and extracts the relevant information to return to the caller.
ADMW_RESULT admw_GetExternalFlashSampleCount | ( | ADMW_DEVICE_HANDLE const | hDevice, |
uint32_t * | nSampleCount | ||
) |
Gets the number of samples stored in the external flash memory.
- Parameters:
-
[in] hDevice ADMW device context handle [in] pSampleCount Address of the return value.
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
- Note:
- No other command must be running when this is called.
ADMW_RESULT admw_GetGpioState | ( | ADMW_DEVICE_HANDLE const | hDevice, |
ADMW_GPIO_PIN const | ePinId, | ||
bool *const | pbAsserted | ||
) |
Get the current state of the specified GPIO input signal.
- Parameters:
-
[in] hDevice ADMW device context handle [in] ePinId GPIO pin to query [out] pbAsserted Pointer to return the state of the status signal GPIO pin
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
- ADMW_INVALID_DEVICE_NUM Invalid GPIO pin specified.
Sets *pbAsserted to true if the status signal is asserted, or false otherwise.
Definition at line 232 of file admw_1001.c.
ADMW_RESULT admw_GetProductID | ( | ADMW_DEVICE_HANDLE const | hDevice, |
ADMW_PRODUCT_ID *const | pProductId | ||
) |
Obtain the product ID from the device.
- Parameters:
-
[in] hDevice ADMW device context handle [out] pProductId Pointer to return the product ID value
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
Reads the product ID registers on the device and returns the value.
Definition at line 944 of file admw_1001.c.
ADMW_RESULT admw_GetStatus | ( | ADMW_DEVICE_HANDLE const | hDevice, |
ADMW_STATUS *const | pStatus | ||
) |
Read the current status from the device registers.
- Parameters:
-
[in] hDevice ADMW device context handle [out] pStatus Pointer to return the status summary obtained from the device.
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
Reads the status registers and extracts the relevant information to return to the caller.
- Note:
- This may be called at any time, assuming the device is ready.
Read the current status from the device registers.
- Parameters:
-
[in] @param[out] pStatus : Pointer to CORE Status struct.
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
- ADMW_FAILURE If status register read fails.
Read the general status register for the ADMW module. Indicates Error, Alert conditions, data ready and command running.
Definition at line 308 of file admw_1001.c.
ADMW_RESULT admw_Open | ( | unsigned const | nDeviceIndex, |
ADMW_CONNECTION *const | pConnectionInfo, | ||
ADMW_DEVICE_HANDLE *const | phDevice | ||
) |
Open ADMW device handle and set up communication interface.
- Parameters:
-
[in] nDeviceIndex Zero-based index number identifying this device instance. Note that this will be used to retrieve a specific device configuration for this device (see admw_SetConfig and ADMW_CONFIG) [in] pConnectionInfo Host-specific connection details (e.g. SPI, GPIO) [out] phDevice Pointer to return an ADMW device handle
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
- ADMW_NO_MEM Failed to allocate memory resources.
- ADMW_INVALID_DEVICE_NUM Invalid device index specified
Configure and initialise the Log interface and the SPI/GPIO communication interface to the ADMW1001 module.
Definition at line 199 of file admw_1001.c.
ADMW_RESULT admw_RegisterGpioCallback | ( | ADMW_DEVICE_HANDLE const | hDevice, |
ADMW_GPIO_PIN const | ePinId, | ||
ADMW_GPIO_CALLBACK const | callbackFunction, | ||
void *const | pCallbackParam | ||
) |
Register an application-defined callback function for GPIO interrupts.
- Parameters:
-
[in] hDevice ADMW context handle (admw_Open) [in] ePinId GPIO pin on which to enable/disable interrupts [in] callbackFunction Function to be called when an interrupt occurs. Specify NULL here to disable interrupts. [in] pCallbackParam Optional opaque parameter passed to the callback
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
- ADMW_INVALID_DEVICE_NUM Invalid GPIO pin specified.
Definition at line 245 of file admw_1001.c.
ADMW_RESULT admw_Reset | ( | ADMW_DEVICE_HANDLE const | hDevice ) |
Reset the ADMW device.
- Parameters:
-
[in] hDevice ADMW device context handle
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
Trigger a hardware-reset of the ADMW device.
- Note:
- The device may require several seconds before it is ready for use again. admw_GetDeviceReadyState may be used to check if the device is ready.
Reset the ADMW device.
- Parameters:
-
[in] hDevice - handle of ADMW device to reset.
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
- ADMW_FAILURE If reseet faisl
Toggle reset pin of the ADMW device low for a minimum of 4 usec.
Definition at line 274 of file admw_1001.c.
ADMW_RESULT admw_RestoreConfig | ( | ADMW_DEVICE_HANDLE const | hDevice, |
ADMW_USER_CONFIG_SLOT const | eSlotId | ||
) |
Restore configuration settings from persistent memory on the device.
- Parameters:
-
[in] hDevice ADMW device context handle [in] eSlotId User configuration slot in persistent memory
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
Instructs the ADMW device to restore the contents of its device configuration registers from non-volatile memory.
- Note:
- No other command must be running when this is called.
Definition at line 534 of file admw_1001.c.
ADMW_RESULT admw_RestoreLutData | ( | ADMW_DEVICE_HANDLE const | hDevice ) |
Restore LUT data from persistent memory on the device.
- Parameters:
-
[in] hDevice ADMW device context handle
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
Instructs the ADMW device to restore the contents of its LUT data, previously stored with admw_SaveLutData, from non-volatile memory.
- Note:
- No other command must be running when this is called.
Definition at line 563 of file admw_1001.c.
ADMW_RESULT admw_RunCalibration | ( | ADMW_DEVICE_HANDLE const | hDevice ) |
Run built-in calibration on the device.
- Parameters:
-
[in] hDevice ADMW device context handle
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
Instructs the ADMW device to execute its self-calibration routines, on any enabled measurement channels, according to the current applied configuration settings. Device status registers will be updated to indicate if any errors were detected.
- Note:
- No other command must be running when this is called.
ADMW_RESULT admw_RunDiagnostics | ( | ADMW_DEVICE_HANDLE const | hDevice ) |
Run built-in diagnostic checks on the device.
- Parameters:
-
[in] hDevice ADMW device context handle
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
Instructs the ADMW device to execute its built-in diagnostic tests, on any enabled measurement channels, according to the current applied configuration settings. Device status registers will be updated to indicate if any errors were detected by the diagnostics.
- Note:
- No other command must be running when this is called.
ADMW_RESULT admw_RunDigitalCalibration | ( | ADMW_DEVICE_HANDLE const | hDevice ) |
Run built-in digital calibration on the device.
- Parameters:
-
[in] hDevice ADMW device context handle
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
Instructs the ADMW device to execute its calibration routines, on any enabled digital channels, according to the current applied configuration settings. Device status registers will be updated to indicate if any errors were detected.
- Note:
- No other command must be running when this is called.
ADMW_RESULT admw_SaveConfig | ( | ADMW_DEVICE_HANDLE const | hDevice, |
ADMW_USER_CONFIG_SLOT const | eSlotId | ||
) |
Store the configuration settings to persistent memory on the device.
- Parameters:
-
[in] hDevice ADMW device context handle [in] eSlotId User configuration slot in persistent memory
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
Instructs the ADMW device to save the current contents of its device configuration registers to non-volatile memory.
- Note:
- No other command must be running when this is called.
- Do not power down the device while this command is running.
Definition at line 516 of file admw_1001.c.
ADMW_RESULT admw_SaveLutData | ( | ADMW_DEVICE_HANDLE const | hDevice ) |
Store the LUT data to persistent memory on the device.
- Parameters:
-
[in] hDevice ADMW device context handle
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
Instructs the ADMW device to save the current contents of its LUT data buffer, set using admw_SetLutData, to non-volatile memory.
- Note:
- No other command must be running when this is called.
- Do not power down the device while this command is running.
Definition at line 553 of file admw_1001.c.
ADMW_RESULT admw_SetConfig | ( | ADMW_DEVICE_HANDLE const | hDevice, |
ADMW_CONFIG *const | pConfig | ||
) |
Write full configuration settings to the device registers.
- Parameters:
-
[in] hDevice ADMW device context handle [out] pConfig Pointer to the configuration data structure
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
Translates configuration details provided into device-specific register settings and updates device configuration registers.
- Note:
- Settings are not applied until admw_ApplyConfigUpdates() is called
Definition at line 2261 of file admw_1001.c.
ADMW_RESULT admw_Shutdown | ( | ADMW_DEVICE_HANDLE const | hDevice ) |
Trigger a shut down of the device.
- Parameters:
-
[in] hDevice ADMW device context handle
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
Instructs the ADMW device to initiate a shut down, typically used to conserve power when the device is not in use. The device may be restarted by calling admw_Reset(). Note that active configuration settings are not preserved during shutdown and must be reloaded after the device has become ready again.
- Note:
- No other command must be running when this is called.
Definition at line 466 of file admw_1001.c.
ADMW_RESULT admw_StartMeasurement | ( | ADMW_DEVICE_HANDLE const | hDevice, |
ADMW_MEASUREMENT_MODE const | eMeasurementMode | ||
) |
Start the measurement cycles on the device.
- Parameters:
-
[in] hDevice ADMW device context handle [in] eMeasurementMode Allows a choice of special modes for the measurement. See ADMW_MEASUREMENT_MODE for further information.
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
Instructs the ADMW device to start executing measurement cycles according to the current applied configuration settings. The DATAREADY status signal will be asserted whenever new measurement data is published, according to selected settings. Measurement cycles may be stopped by calling admw_StopMeasurement.
- Note:
- No other command must be running when this is called.
Start the measurement cycles on the device.
- Parameters:
-
[out]
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
- ADMW_FAILURE
Sends the latch config command. Configuration for channels in conversion cycle should be completed before this function. Channel enabled bit should be set before this function. Starts a conversion and configures the format of the sample.
Definition at line 494 of file admw_1001.c.
ADMW_RESULT admw_StopMeasurement | ( | ADMW_DEVICE_HANDLE const | hDevice ) |
Stop the measurement cycles on the device.
- Parameters:
-
[in] hDevice ADMW device context handle
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully.
Instructs the ADMW device to stop executing measurement cycles. The command may be delayed until the current conversion, if any, has been completed and published.
- Note:
- To be used only if a measurement command is currently running.
Definition at line 573 of file admw_1001.c.
Generated on Wed Jul 13 2022 03:04:55 by
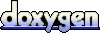