
Host API Example for the ADMW1001
ADMW Host GPIO interface functions
[ADMW Host Portability Layer]
Typedefs | |
typedef void(* | ADMW_GPIO_CALLBACK )(ADMW_GPIO_PIN ePinId, void *pArg) |
typedef void * | ADMW_GPIO_HANDLE |
Enumerations | |
enum | ADMW_GPIO_PIN { ADMW_GPIO_PIN_RESET = 0, ADMW_GPIO_PIN_ALERT_ERROR, ADMW_GPIO_PIN_DATAREADY } |
Functions | |
ADMW_RESULT | admw_GpioOpen (ADMW_PLATFORM_GPIO_CONFIG *pConfig, ADMW_GPIO_HANDLE *phDevice) |
Open the SPI interface and allocate resources. | |
void | admw_GpioClose (ADMW_GPIO_HANDLE hDevice) |
Close GPIO interface and free resources. | |
ADMW_RESULT | admw_GpioGet (ADMW_GPIO_HANDLE hDevice, ADMW_GPIO_PIN ePinId, bool *pbState) |
Get the state of the specified GPIO pin. | |
ADMW_RESULT | admw_GpioSet (ADMW_GPIO_HANDLE hDevice, ADMW_GPIO_PIN ePinId, bool bState) |
Set the state of the specified GPIO pin. | |
ADMW_RESULT | admw_GpioIrqEnable (ADMW_GPIO_HANDLE hDevice, ADMW_GPIO_PIN ePinId, ADMW_GPIO_CALLBACK callback, void *arg) |
Enable interrupt notifications on the specified GPIO pin. | |
ADMW_RESULT | admw_GpioIrqDisable (ADMW_GPIO_HANDLE hDevice, ADMW_GPIO_PIN ePinId) |
Disable interrupt notifications on the specified GPIO pin. |
Typedef Documentation
typedef void(* ADMW_GPIO_CALLBACK)(ADMW_GPIO_PIN ePinId, void *pArg) |
GPIO callback function signature
- Parameters:
-
[in] ePinId The GPIO pin which triggered the interrupt notification [in] pArg Optional opaque parameter to be passed to the callback
Definition at line 69 of file admw_gpio.h.
typedef void* ADMW_GPIO_HANDLE |
A handle used in all API functions to identify the GPIO interface context
Definition at line 74 of file admw_gpio.h.
Enumeration Type Documentation
enum ADMW_GPIO_PIN |
GPIO pin identifiers
- Enumerator:
ADMW_GPIO_PIN_RESET RESET GPIO output signal
ADMW_GPIO_PIN_ALERT_ERROR ALERT GPIO input signal
ADMW_GPIO_PIN_DATAREADY DATAREADY GPIO input signal
Definition at line 56 of file admw_gpio.h.
Function Documentation
void admw_GpioClose | ( | ADMW_GPIO_HANDLE | hDevice ) |
Close GPIO interface and free resources.
- Parameters:
-
[in] hDevice GPIO interface context handle (admw_GpioOpen)
Definition at line 251 of file admw_gpio.cpp.
ADMW_RESULT admw_GpioGet | ( | ADMW_GPIO_HANDLE | hDevice, |
ADMW_GPIO_PIN | ePinId, | ||
bool * | pbState | ||
) |
Get the state of the specified GPIO pin.
- Parameters:
-
[in] hDevice GPIO interface context handle (admw_GpioOpen) [in] ePinId GPIO pin to be read [out] pbState Pointer to return the state of the GPIO pin
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully
- ADMW_INVALID_DEVICE_NUM Invalid GPIO pin specified
Definition at line 199 of file admw_gpio.cpp.
ADMW_RESULT admw_GpioIrqDisable | ( | ADMW_GPIO_HANDLE | hDevice, |
ADMW_GPIO_PIN | ePinId | ||
) |
Disable interrupt notifications on the specified GPIO pin.
- Parameters:
-
[in] hDevice GPIO interface context handle (admw_GpioOpen) [in] ePinId GPIO pin on which to disable interrupt notifications
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully
- ADMW_INVALID_DEVICE_NUM Invalid GPIO pin specified
Definition at line 239 of file admw_gpio.cpp.
ADMW_RESULT admw_GpioIrqEnable | ( | ADMW_GPIO_HANDLE | hDevice, |
ADMW_GPIO_PIN | ePinId, | ||
ADMW_GPIO_CALLBACK | callback, | ||
void * | arg | ||
) |
Enable interrupt notifications on the specified GPIO pin.
- Parameters:
-
[in] hDevice GPIO interface context handle (admw_GpioOpen) [in] ePinId GPIO pin on which to enable interrupt notifications [in] callback Callback function to invoke when the GPIO is asserted [in] arg Optional opaque parameter to be passed to the callback
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully
- ADMW_INVALID_DEVICE_NUM Invalid GPIO pin specified
Definition at line 225 of file admw_gpio.cpp.
ADMW_RESULT admw_GpioOpen | ( | ADMW_PLATFORM_GPIO_CONFIG * | pConfig, |
ADMW_GPIO_HANDLE * | phDevice | ||
) |
Open the SPI interface and allocate resources.
- Parameters:
-
[in] pConfig Pointer to platform-specific GPIO interface details [out] phDevice Pointer to return a GPIO interface context handle
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully
- ADMW_NO_MEM Failed to allocate memory for interface context
Definition at line 180 of file admw_gpio.cpp.
ADMW_RESULT admw_GpioSet | ( | ADMW_GPIO_HANDLE | hDevice, |
ADMW_GPIO_PIN | ePinId, | ||
bool | bState | ||
) |
Set the state of the specified GPIO pin.
- Parameters:
-
[in] hDevice GPIO interface context handle (admw_GpioOpen) [in] ePinId GPIO pin to be set [in] bState The state to set for GPIO pin
- Returns:
- Status
- ADMW_SUCCESS Call completed successfully
- ADMW_INVALID_DEVICE_NUM Invalid GPIO pin specified
Definition at line 212 of file admw_gpio.cpp.
Generated on Wed Jul 13 2022 03:04:55 by
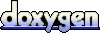